A Beginner's Guide to Pointers in Go

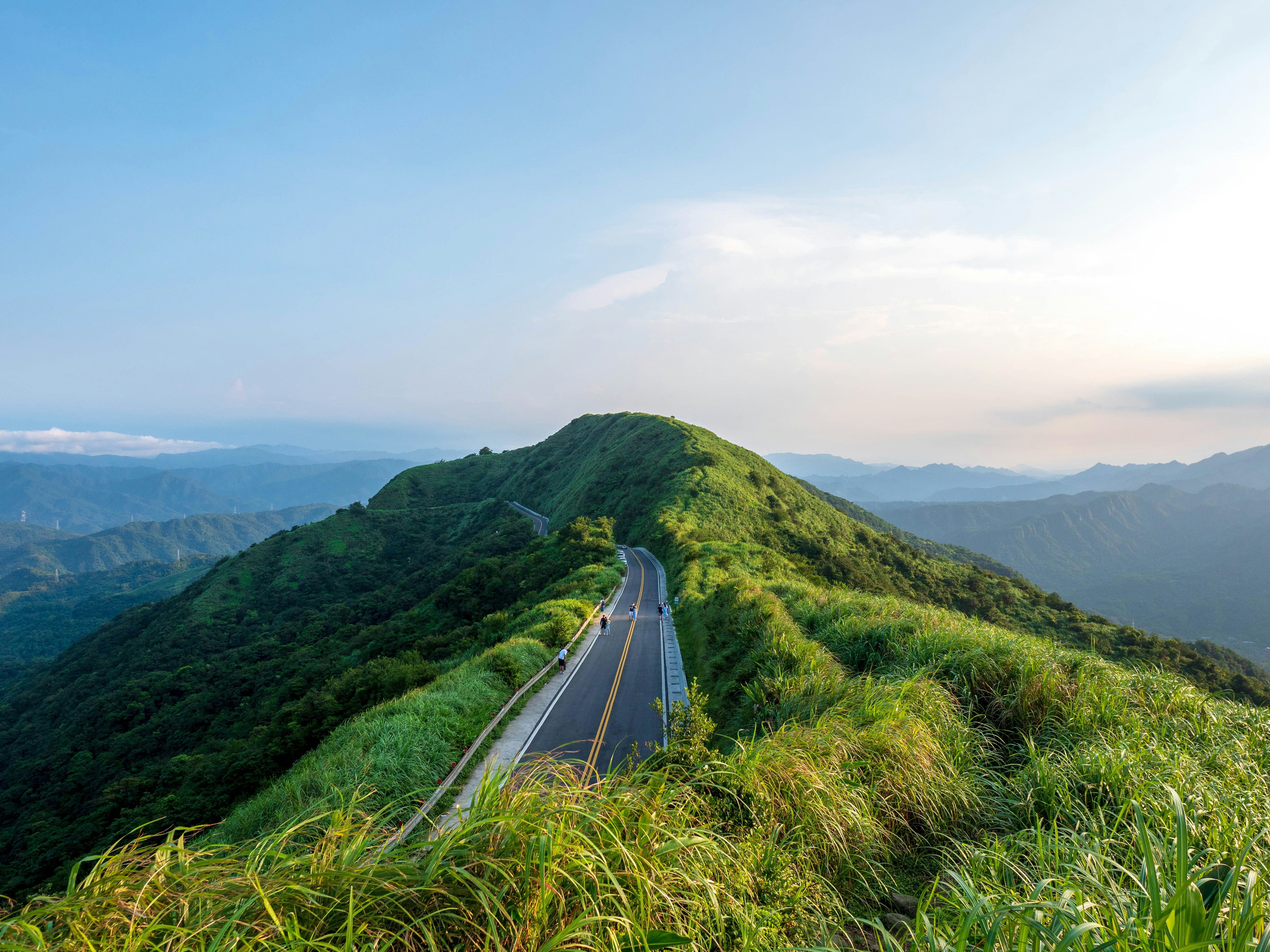
When working with Go, understanding memory management and pointers can help you write more efficient and optimized code. In this article, we’ll explain pointers, their role in memory management, and how to use them effectively.
What Are Pointers?
A pointer is a variable that stores the memory address of another variable. Instead of holding a value directly, pointers "point" to the location in memory where the value is stored.
Why Use Pointers?
Efficient Memory Usage: Instead of copying large amounts of data, you can pass a pointer to the data.
Shared Access: Multiple functions can modify the same data using a pointer.
Dynamic Memory Management: Pointers help allocate and manage memory dynamically.
Basics of Pointers in Go
Here’s how you declare and use pointers in Go:
Declaring a Pointer
To declare a pointer, use the *
operator.
var ptr *int // Declares a pointer to an integer
Getting a Pointer
Use the &
operator to get the memory address of a variable.
num := 42
ptr := &num // ptr now points to the address of num
Dereferencing a Pointer
To access or modify the value stored at the memory address a pointer points to, use the *
operator (dereferencing).
fmt.Println(*ptr) // Prints the value stored at ptr's address
Example: Basic Pointer Usage
package main
import "fmt"
func main() {
num := 42
ptr := &num // Get pointer to num
fmt.Println("Value of num:", num) // Prints 42
fmt.Println("Address of num:", ptr) // Prints the address
fmt.Println("Value via pointer:", *ptr) // Dereferences ptr to get 42
// Modify num via the pointer
*ptr = 100
fmt.Println("Modified num:", num) // Prints 100
}
Explanation
&num
: Gets the address ofnum
.ptr
: Stores the memory address ofnum
.*ptr
: Dereferences the pointer to access or modify the value stored at the address.
Memory Management and Pointers
Pointers play a significant role in memory management, as they allow you to control how and where memory is allocated.
Stack vs. Heap
Stack:
Stores local variables and function calls.
Automatically deallocated when the function exits.
Heap:
Stores dynamically allocated data.
Requires pointers to access and manage.
Using new
for Dynamic Memory Allocation
The new
function allocates memory and returns a pointer to the zero-initialized value.
package main
import "fmt"
func main() {
ptr := new(int) // Allocates memory for an integer
fmt.Println("Value:", *ptr) // Prints 0 (zero-initialized)
*ptr = 50 // Modify value via pointer
fmt.Println("Updated Value:", *ptr) // Prints 50
}
Explanation
new(int)
: Allocates memory for an integer on the heap.Pointer Returned: You can access and modify the value using the returned pointer.
Sharing Memory Between Functions
Using pointers, you can share and modify data across functions without copying it.
package main
import "fmt"
// Modify value via pointer
func updateValue(ptr *int) {
*ptr = 100
}
func main() {
num := 42
fmt.Println("Before:", num)
updateValue(&num) // Pass the address of num
fmt.Println("After:", num)
}
Explanation
Passing by Pointer: The address of
num
is passed toupdateValue
.Modify via Pointer: The function updates the value stored at that address.
Common Mistakes and Tips
Avoid Dangling Pointers:
A dangling pointer points to memory that has been deallocated. Go’s garbage collector minimizes this risk by managing memory for you.Be Cautious with Aliasing:
Multiple pointers to the same memory can cause unintended side effects.Nil Pointers:
A pointer with no address is called a nil pointer. Always check for nil before dereferencing.var ptr *int if ptr != nil { fmt.Println(*ptr) } else { fmt.Println("Pointer is nil") }
Advantages of Pointers
Performance: Reduces memory copying for large data structures.
Flexibility: Enables dynamic memory allocation and efficient parameter passing.
Control: Provides more control over how memory is used and managed.
Pointers in Go vs. Other Languages
Unlike C, Go does not support pointer arithmetic (e.g.,
ptr++
) to prevent memory access errors.The garbage collector in Go automatically frees unused memory, reducing manual pointer management.
Conclusion
Pointers are a powerful tool in Go, enabling efficient memory usage, data sharing, and dynamic memory allocation. Understanding how pointers work and their role in memory management helps you write better, more efficient code.
In upcoming tutorials, we’ll explore advanced memory concepts like slices, maps, and the role of pointers in struct management. Keep practicing, and happy coding! 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
