How Multi-Tenant Sites Work in Next.js

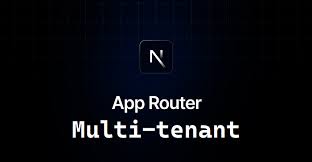
Introduction
Definition of Multi-Tenancy in Web Applications
Multi-tenancy refers to a software architecture where a single instance of an application serves multiple customers, known as "tenants." Each tenant has its own isolated data, settings, and possibly even a custom interface, all within the same application instance. This contrasts with single-tenancy, where each customer has a dedicated instance of the application.
Importance of Multi-Tenancy in Modern SaaS Applications
In modern SaaS (Software as a Service) applications, multi-tenancy plays a vital role in cost efficiency, scalability, and maintainability. It enables providers to serve many customers with fewer resources by pooling common infrastructure and resources. For developers, multi-tenancy allows for easier updates, centralized management, and customization per tenant, making it an essential architecture for SaaS businesses.
Overview of Next.js as a Web Development Framework
Next.js is a powerful React framework that provides built-in features like server-side rendering (SSR), static site generation (SSG), and API routes. It simplifies the development of scalable and optimized applications, making it an ideal choice for building multi-tenant sites. With its flexibility and ease of use, Next.js allows developers to efficiently manage routing, state, and data segregation in a multi-tenant environment.
Architectural Overview of Multi-Tenant Sites
Explanation of Single-Tenancy vs. Multi-Tenancy
Single-Tenancy: In a single-tenant architecture, each customer gets their own instance of the application, meaning they have isolated resources and databases.
Multi-Tenancy: A multi-tenant architecture serves multiple customers using the same application instance. However, data and configurations are kept isolated per tenant, ensuring privacy and security.
Benefits of a Multi-Tenant Architecture
Cost Efficiency: Fewer resources are needed to run multiple customer instances.
Centralized Management: Easier to maintain, update, and scale the system.
Faster Deployment: Roll out changes and new features to all tenants simultaneously.
Resource Optimization: Share infrastructure and resources while still providing tenant-specific customizations.
Challenges Associated with Multi-Tenancy
Data Isolation: Ensuring each tenant's data is separate and secure.
Customizability: Providing enough flexibility for each tenant to have a unique experience.
Scalability: Ensuring the application can handle increasing loads as the number of tenants grows.
Performance: Balancing the demands of multiple tenants while ensuring fast load times.
Implementing Multi-Tenancy in Next.js
Directory Structure for Multi-Tenant Applications
When building multi-tenant applications in Next.js, the directory structure plays an essential role in organizing the codebase and managing tenant-specific assets. Here's an example of a possible structure:
/pages
/[tenant] # Dynamic route for each tenant
/index.js # Landing page for a tenant
/dashboard.js # Tenant-specific dashboard
/api
/[tenant] # API routes specific to each tenant
/components
/common # Shared components across tenants
/tenant-specific # Components unique to certain tenants
/styles
/global.css # Global styles for all tenants
/[tenant].css # Tenant-specific styles
Organizing Codebase
The main challenge in a multi-tenant system is maintaining a balance between shared and tenant-specific resources. Common functionality like authentication, navigation, and API handling should be separated and reused across tenants. Tenant-specific code (e.g., UI elements, data, or custom behavior) should be modular and easy to manage.
Tenant-Specific Pages and Components
Each tenant might have specific pages, components, and configurations. In Next.js, dynamic routing can be leveraged to load tenant-specific content. For example, the pages/[tenant]
folder can be used to dynamically generate pages based on the tenant's subdomain or path.
Dynamic Routing in Next.js
Next.js makes dynamic routing easy with its file-based routing system. For multi-tenant applications, dynamic routes are used to load content specific to each tenant based on the URL.
Example of Dynamic Routing Configuration
To handle different tenants, you can configure a dynamic route in the pages/[tenant]
directory. For example:
// pages/[tenant]/index.js
import { useRouter } from 'next/router'
const TenantHomePage = () => {
const router = useRouter()
const { tenant } = router.query
return <h1>Welcome to {tenant}'s homepage</h1>
}
export default TenantHomePage
This will dynamically load a page for each tenant based on their subdomain or URL.
Handling Tenant-Specific Data
In a multi-tenant system, data segregation is critical to ensuring that each tenant's data remains private. This can be achieved through various methods:
Database Design: One database for all tenants, with tenant-specific identifiers to isolate data.
Separate Databases: A different database for each tenant, ensuring total isolation.
Tenant-Specific APIs: Using tenant identifiers in API requests to return only the relevant data.
Tenant Identification and Authentication
To serve personalized content, tenants need to be identified. This can be done by:
Subdomains: Each tenant has a unique subdomain (e.g., tenant1.yoursite.com).
Tenant ID in URL: A unique tenant identifier in the URL path (e.g., www.yoursite.com/tenant1).
Authentication: Use middleware in Next.js to ensure users are authenticated for their specific tenant.
Environment Configuration for Tenants
Managing tenant-specific configurations, such as branding, features, or settings, can be achieved using environment variables or a custom configuration file.
// next.config.js
module.exports = {
env: {
NEXT_PUBLIC_TENANT_NAME: process.env.TENANT_NAME,
NEXT_PUBLIC_TENANT_THEME: process.env.TENANT_THEME,
},
}
This allows you to easily customize settings based on the tenant.
State Management Across Tenants
Introduction to State Management in Next.js
In multi-tenant applications, managing state becomes more complex, as each tenant’s state must be isolated. For this, libraries like Redux or Zustand can be used to manage global state, ensuring that each tenant's data remains separate.
Sharing Code and Resources Between Tenants
Shared resources such as UI components, utilities, or API integrations can be reused across tenants. This reduces redundancy and ensures consistency across the application.
Maintaining Isolated State Per Tenant
For isolated tenant state, Next.js’s built-in support for cookies, localStorage, or server-side session management can be used to store tenant-specific data while ensuring that the state doesn’t bleed between tenants.
Security Considerations for Multi-Tenant Next.js Sites
Data Isolation and Protection
It’s crucial to ensure that tenant data is isolated and secure. Using proper database access controls and ensuring that each API request only accesses the data of the authenticated tenant will help maintain data privacy.
Implementing Authentication and Authorization Mechanisms
Authentication should be designed to authenticate users for their specific tenant, and authorization mechanisms should ensure that users can only access resources they are permitted to. This can be done using token-based authentication like JWT or OAuth.
Best Practices for Securing a Multi-Tenant Application
Implement strong role-based access control (RBAC).
Secure all API routes using authentication and authorization middleware.
Regularly audit your system for vulnerabilities and patch accordingly.
Performance Optimization for Multi-Tenant Applications
Leveraging Next.js Built-in Features for Scalability
Next.js offers features like Incremental Static Regeneration (ISR), which allows static pages to be updated without rebuilding the entire site. This is especially useful for multi-tenant applications that need to handle large amounts of traffic across different tenants.
Caching Strategies to Reduce Load Times
Using Next.js’s built-in caching mechanisms, such as image optimization, server-side caching, and static site generation, can significantly reduce the load time for each tenant.
Monitoring and Profiling Tenant-Specific Performance
With multiple tenants sharing the same application instance, it’s crucial to monitor performance to ensure no tenant experiences delays or downtimes. Tools like Next.js’s built-in analytics and external monitoring services can help.
Case Study/Examples
Real-World Examples of Multi-Tenant Applications Built with Next.js
Several real-world applications have been built using multi-tenant architectures with Next.js. For instance, platforms that offer white-labeled solutions to different organizations can use multi-tenancy to serve each organization with a custom experience while maintaining a shared backend.
Lessons Learned and Key Takeaways
Key takeaways from existing implementations include:
Tenant-specific configurations are critical for customization.
Data segregation is non-negotiable for security.
Scalability can be managed with Next.js's built-in features, like ISR and static site generation.
Conclusion
Recap of Multi-Tenancy Concepts and Next.js Capabilities
Multi-tenancy is a powerful architecture for modern web applications, especially in SaaS solutions. Next.js provides the tools necessary to build scalable, secure, and efficient multi-tenant applications with its support for dynamic routing, environment variables, and state management.
Future of Multi-Tenancy in Web Development
As SaaS platforms continue to grow, the need for efficient multi-tenant architectures will increase. Next.js will likely remain a key framework for building such applications due to its flexibility, scalability, and developer-friendly features.
Encouragement for Exploring Next.js for Multi-Tenant Applications
If you’re planning to build a multi-tenant web application, Next.js is an excellent choice due to its robust capabilities and vast ecosystem. Start experimenting with multi-tenant now
Subscribe to my newsletter
Read articles from Vignesh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
