Bcrypt: The Unsung Hero of Password Security
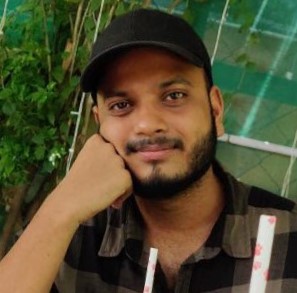
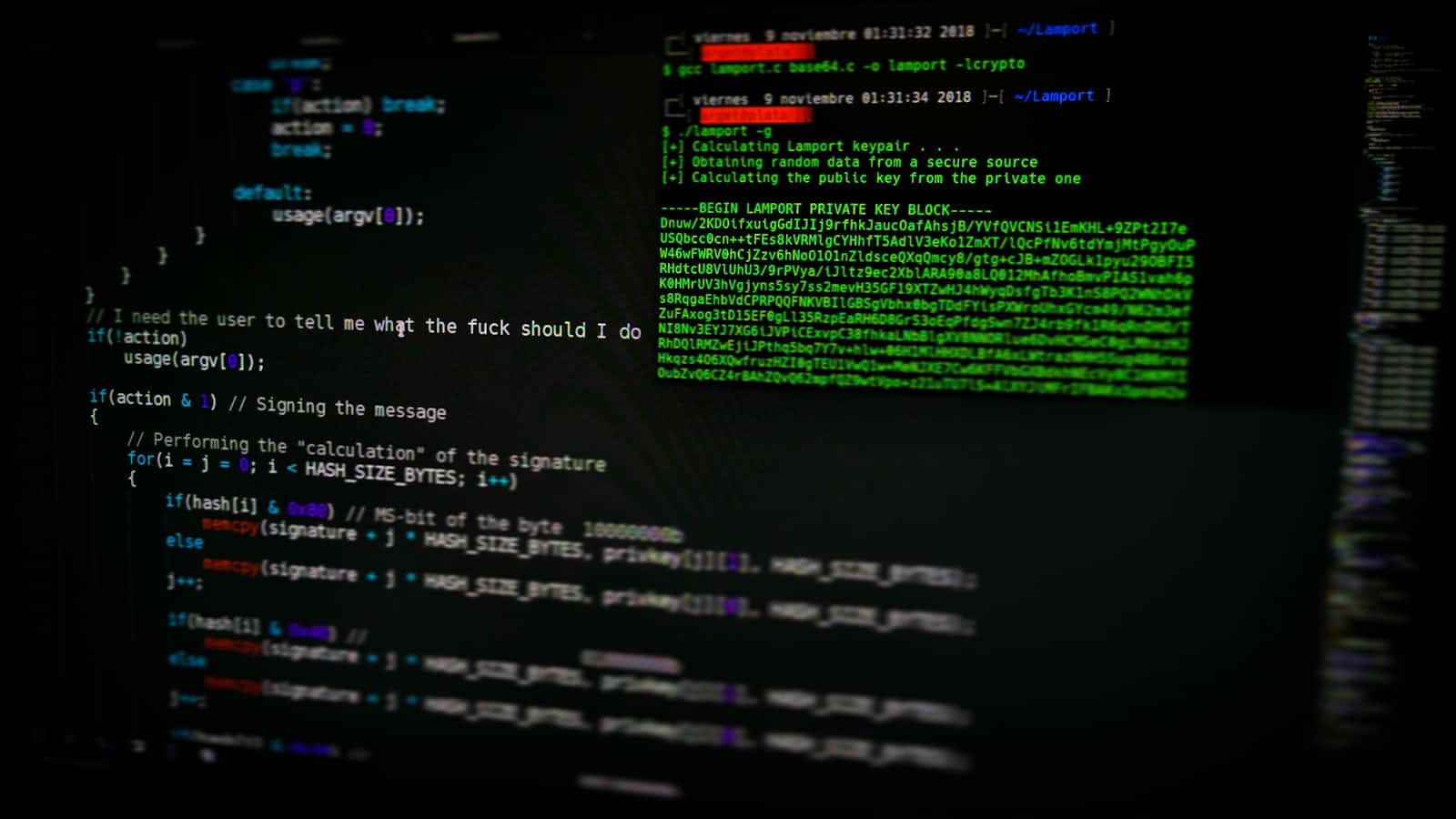
In the digital age, where data breaches are as common as coffee spills, one cryptographic algorithm stands out for its robustness in securing our passwords: bcrypt. Let's dive into the world of bcrypt hashing, uncovering its mechanics, the role of salting, and how it keeps our credentials safe from prying eyes.
What is Bcrypt?
Bcrypt is a password hashing function designed by Niels Provos and David Mazières, based on the Blowfish cipher. Its primary goal? To protect passwords in storage against offline attacks. Here's why bcrypt isn't just another algorithm:
Adaptive Hashing: bcrypt allows for variable computational cost, meaning you can increase its security over time as computing power advances.
Built-in Salt: Each password hash includes a unique salt, reducing the effectiveness of rainbow tables and ensuring that identical passwords have different hashes.
The Structure of a Bcrypt Hash
$2a$10$N9qo8uLOickgx2ZMRZoMyeIjZAgcfl7p92ldGxad68LJZdL17lhWy
2a : This is the version of bcrypt. Think of it as the type of bread for your sandwich.
10: Represents the cost factor. It's like deciding how much effort goes into making the sandwich. A higher number means more rounds of hashing, making it tougher for someone to crack.
Salt: N9qo8uLOickgx2ZMRZoMye - This is the unique seasoning. Each password gets its own special blend, ensuring no two sandwiches taste the same.
Hash: IjZAgcfl7p92ldGxad68LJZdL17lhWy - This is the actual hashed password, the main ingredient after being processed with the salt.
How Does Bcrypt Work?
Here's a step-by-step breakdown:
Password Input: When you type in your password, it's like ordering a custom sandwich.
password := []byte("yourpassword")
Hashing with Salt: bcrypt generates a salt for each password. This is like the chef adding a secret ingredient.
hashedPassword, err := bcrypt.GenerateFromPassword(password, bcrypt.DefaultCost) if err != nil { log.Fatal(err) }
Storing the Hash: The hash, including the salt, is stored in your database. When someone tries to log in:
storedHash := bcryptHashFromDatabase // This would be read from your storage
Password Verification:
The salt from storedHash is extracted (like reading the recipe).
The provided password is hashed using this salt.
The two hashes are compared in constant time to prevent timing attacks.
err := bcrypt.CompareHashAndPassword(storedHash, providedPassword)
if err == nil {
fmt.Println("Login successful")
} else {
fmt.Println("Login failed")
}
Engaging with Bcrypt
To make this more visual, think of bcrypt like cooking:
Salting: Just like adding spices to a dish, bcrypt adds a unique flavor (salt) to each password, making each hash unique.
Slow Cooking: bcrypt intentionally takes time to hash (the cost factor). This slow cooking process is a security feature, making brute-force attacks less feasible.
Taste Test: When you try to log in, bcrypt isn't just checking if the password is right; it's ensuring the dish (hash) matches the original recipe (stored hash), including the secret ingredient (salt).
Why Not Just Store Passwords Plainly?
Storing passwords in plain text is like leaving your front door unlocked. Here are a few reasons why bcrypt is better:
Salting: Prevents pre-computed hash attacks.
Adaptive Difficulty: As hardware improves, you can increase the cost factor to stay ahead of computational advances.
Secure Against Timing Attacks: Constant-time comparison prevents hackers from deducing information from how long comparisons take.
Conclusion
Bcrypt turns the simple act of password hashing into an art form, ensuring that even if someone gets their hands on your database, cracking those passwords would be like trying to reverse-engineer a gourmet recipe from a single bite of a dish.
Next time you type in your password, give a nod to bcrypt for keeping your digital life secure. And remember, always use strong, unique passwords for each service, because even the best seasoning can't protect a poorly chosen ingredient.
Subscribe to my newsletter
Read articles from Ashish maurya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
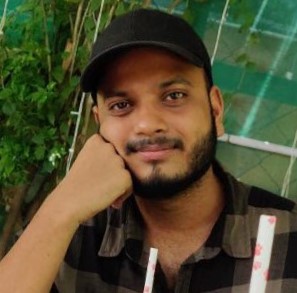
Ashish maurya
Ashish maurya
I am a developer from India with a good eye for frontend logic and design. I am also well acquainted with the backend and business logic so that's add to my ability to create a good frontend while making it easily integratable with backend.