In-Depth Guide on Generators, Continuations, and Virtual Threads in Java with Practical Examples.
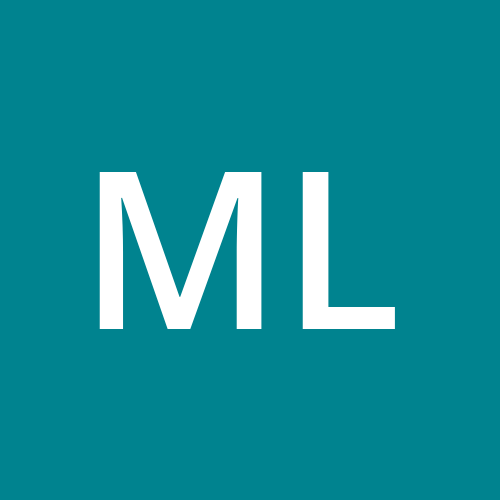
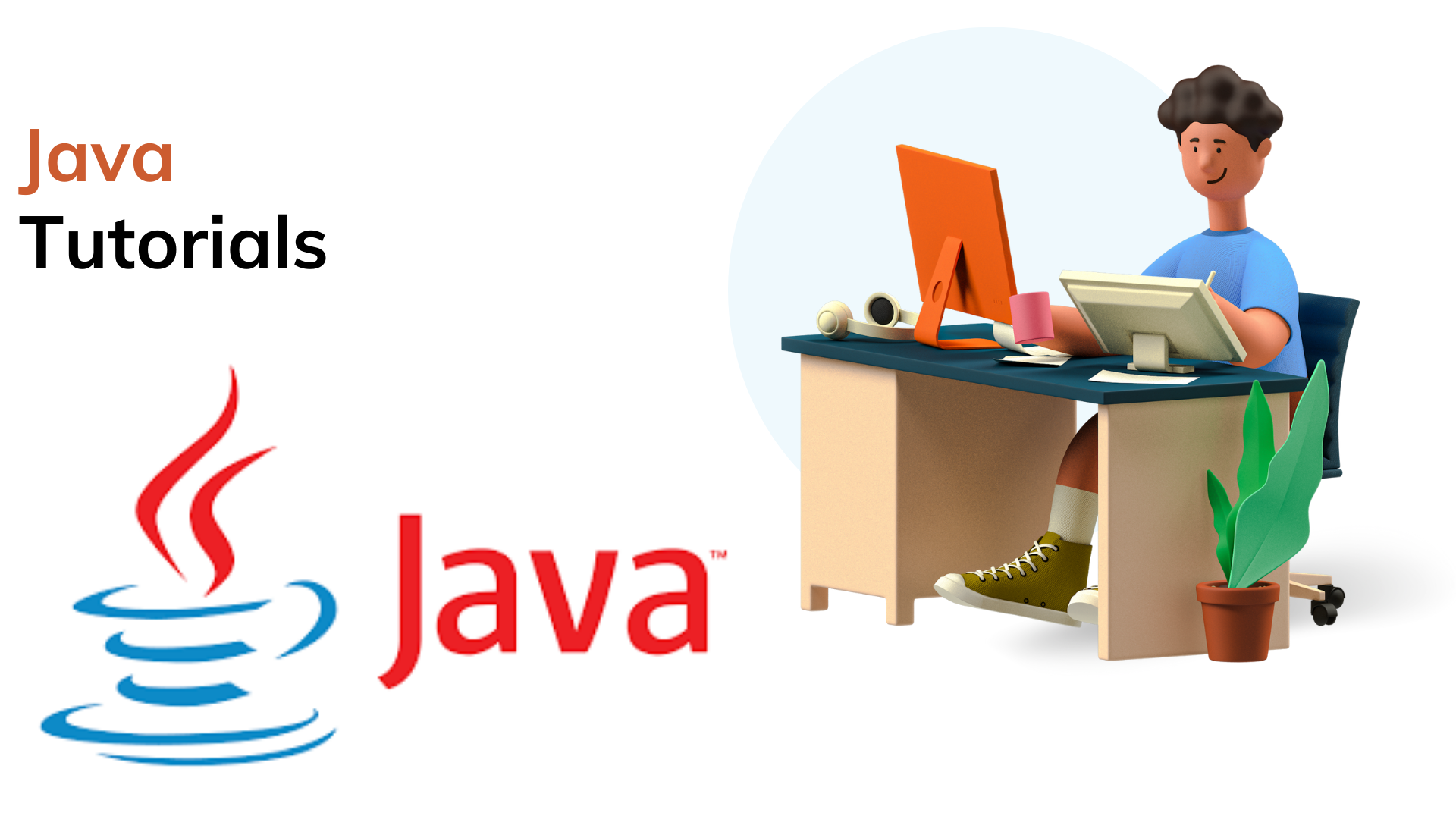
Java is continuously evolving with new features aimed at improving the language's concurrency and performance. Three such advanced features introduced in recent versions are Generators, Continuations, and Virtual Threads. These features, though relatively new, offer a powerful way to manage concurrency in Java applications. In this guide, we will dive deep into each of these concepts, explaining their importance and providing practical examples to illustrate how they work in Java.
1. What Are Generators in Java?
A generator is a special type of function that produces a sequence of results, instead of a single value. Generators in Java are usually implemented using iterators, but Java has recently introduced more powerful features like yield to make generator-style functions easier to write. In simpler terms, a generator is a way of creating values on-demand, without having to store them all in memory at once.
Example: Using Generators with yield
The yield
keyword allows you to write a generator-style function in Java. However, this feature is available only through Java's Project Loom and is still evolving.
public class GeneratorExample {
public static void main(String[] args) {
// Using a generator to produce a sequence of numbers
for (var num : generateNumbers()) {
System.out.println(num);
}
}
// Generator function using yield to create a sequence of numbers
public static Iterable<Integer> generateNumbers() {
return () -> new java.util.Iterator<>() {
private int current = 1;
@Override
public boolean hasNext() {
return current <= 5;
}
@Override
public Integer next() {
return current++;
}
};
}
}
Explanation: The method generateNumbers
acts like a generator that yields numbers starting from 1. Using yield
, the generator provides the next value only when requested. This approach is memory-efficient because we don’t generate all the numbers at once.
2. What Are Continuations in Java?
Continuations are a low-level concurrency mechanism that allows you to pause the execution of a program at a certain point and resume it later. This can be useful in situations where you need to manage a process that involves waiting for some other resource, such as I/O operations or network requests.
Java does not have direct support for continuations in the traditional sense, but Project Loom introduces continuations through the virtual threads API. A continuation allows a virtual thread to be paused and resumed at a later time, enabling better performance in highly concurrent applications.
Example: Continuations via Virtual Threads
Project Loom introduces the concept of virtual threads, which can be used to implement continuation-like behavior in Java.
public class ContinuationExample {
public static void main(String[] args) throws InterruptedException {
// Creating and starting a virtual thread that simulates a continuation
var thread = Thread.ofVirtual().start(() -> {
System.out.println("Start of virtual thread");
try {
// Simulating a continuation point (pause)
Thread.sleep(1000);
System.out.println("Resumed after 1 second");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
// Wait for the virtual thread to finish
thread.join();
}
}
Explanation: In this example, a virtual thread simulates a continuation by pausing for 1 second before resuming. This approach allows Java applications to handle many lightweight threads efficiently without the overhead associated with traditional threads.
3. What Are Virtual Threads in Java?
Virtual threads are a new kind of thread introduced by Project Loom that are extremely lightweight and designed to handle a large number of concurrent tasks more efficiently than traditional threads. Traditional threads are often heavy due to the resources they consume, but virtual threads are designed to be far more lightweight and less resource-intensive.
The key benefit of virtual threads is that they allow you to create thousands or even millions of concurrent threads with minimal impact on performance. This makes them ideal for applications that require handling a large number of tasks simultaneously, such as web servers or network applications.
Example: Virtual Threads for Concurrency
public class VirtualThreadExample {
public static void main(String[] args) throws InterruptedException {
// Creating 1000 virtual threads to simulate concurrent processing
for (int i = 0; i < 1000; i++) {
final int taskId = i;
Thread.ofVirtual().start(() -> {
try {
// Simulate some work
System.out.println("Processing task: " + taskId);
Thread.sleep(500); // Simulate task processing time
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
// Sleep to allow virtual threads to finish
Thread.sleep(2000);
}
}
Explanation: In this example, we are creating 1000 virtual threads that simulate concurrent tasks. Virtual threads are ideal for handling a large number of tasks without significant performance penalties. This approach is lightweight compared to using traditional threads, as each virtual thread consumes very little memory.
4. How Virtual Threads Improve Performance in Concurrency
Virtual threads enable applications to achieve a high degree of concurrency without the usual overhead associated with managing traditional threads. Here’s why they improve performance:
Lightweight: Virtual threads consume much less memory and CPU compared to traditional threads.
Scalability: Virtual threads allow developers to easily scale applications to handle a large number of concurrent tasks without running into issues like thread pool exhaustion or system resource limits.
Simplified Concurrency: With virtual threads, developers can write more natural, sequential code instead of dealing with complex callback-based concurrency mechanisms like
CompletableFuture
or explicit thread management.
Example: Comparing Virtual Threads with Traditional Threads
public class ThreadComparison {
public static void main(String[] args) throws InterruptedException {
// Traditional thread example
long startTime = System.nanoTime();
for (int i = 0; i < 1000; i++) {
new Thread(() -> {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}).start();
}
System.out.println("Traditional threads executed in: " + (System.nanoTime() - startTime) / 1_000_000 + " ms");
// Virtual thread example
startTime = System.nanoTime();
for (int i = 0; i < 1000; i++) {
Thread.ofVirtual().start(() -> {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
System.out.println("Virtual threads executed in: " + (System.nanoTime() - startTime) / 1_000_000 + " ms");
}
}
Explanation: In this example, we compare the execution time of creating 1000 traditional threads versus 1000 virtual threads. The virtual threads will typically show much faster execution times due to their lightweight nature.
5. When to Use Virtual Threads in Your Java Application
Virtual threads are a great tool, but they are not always the best choice for every use case. Here are some scenarios where they shine:
Handling I/O-bound tasks: Virtual threads are perfect for applications that handle many I/O-bound tasks, such as web servers or database queries, as they allow you to scale easily without using excessive memory.
Task-based parallelism: If your application involves managing many small tasks that can be executed concurrently, virtual threads will provide a much more efficient solution than traditional threads.
Reactive programming: Virtual threads are a natural fit for reactive programming models, where you need to process many events concurrently.
However, if your tasks are CPU-bound and require heavy computation, traditional threads might be a better option.
Conclusion
Java’s Generators, Continuations, and Virtual Threads offer exciting new ways to handle concurrency and improve performance in Java applications. By leveraging these features, you can write more efficient, scalable, and maintainable code.
Generators simplify the process of working with sequences of values.
Continuations, through virtual threads, allow you to pause and resume execution without blocking resources.
Virtual Threads provide a highly efficient mechanism for managing large numbers of concurrent tasks.
As these features become more stable and widely adopted, they will play a significant role in shaping the future of Java development.
More such articles:
https://www.youtube.com/@maheshwarligade
Subscribe to my newsletter
Read articles from Maheshwar Ligade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
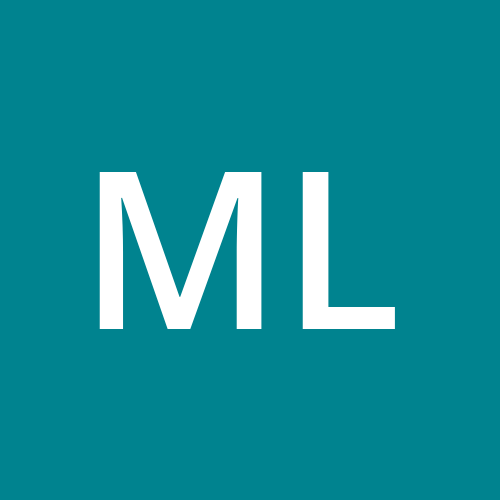
Maheshwar Ligade
Maheshwar Ligade
Learner, Love to make things simple, Full Stack Developer, StackOverflower, Passionate about using machine learning, deep learning and AI