Argon2: The Gold Standard for Password Hashing

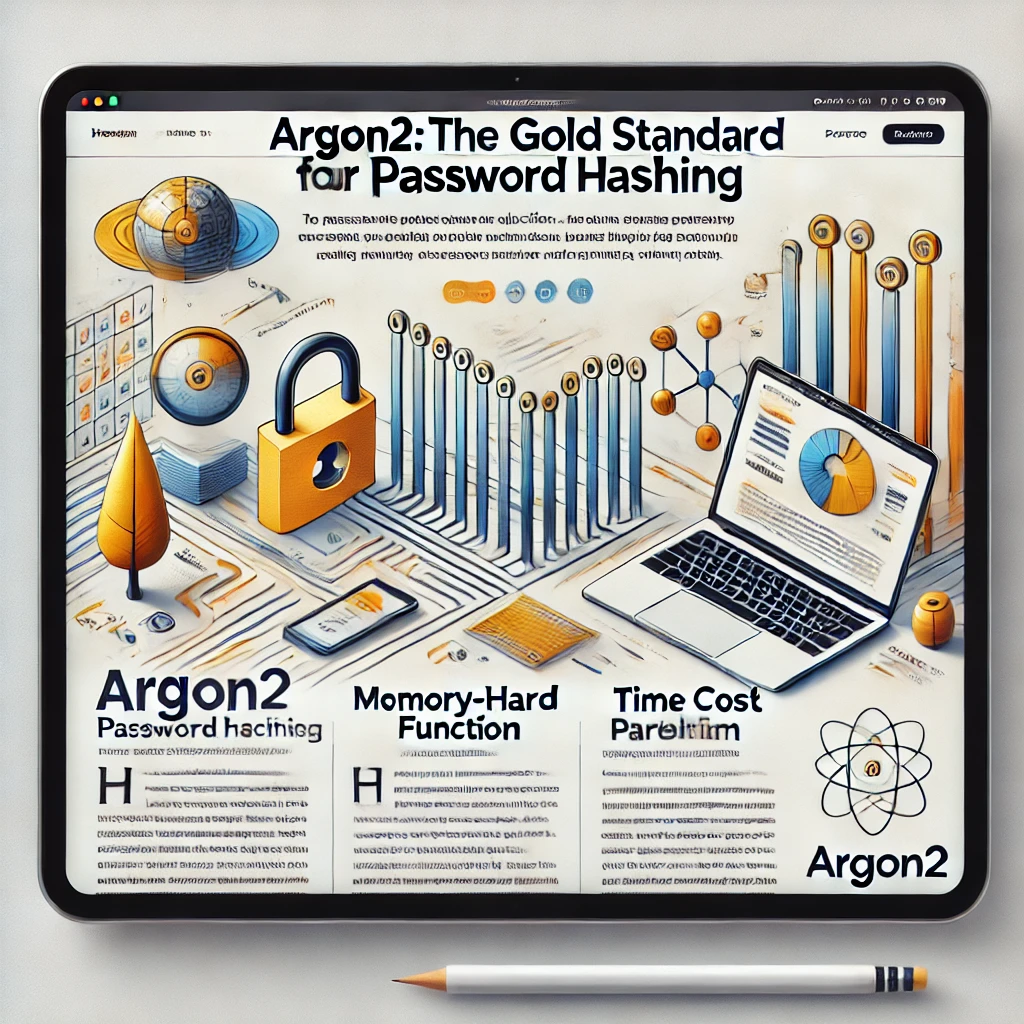
In an era where data breaches and cyberattacks are increasingly common, the importance of securing user passwords cannot be overstated. Password hashing is a critical component of this security, ensuring that even if an attacker gains access to stored credentials, the passwords remain protected. Among the myriads of hashing algorithms available, Argon2 stands out as a modern, robust, and highly secure solution.
This article explores Argon2, its workings, why it’s considered the best choice for password hashing, and how you can implement it in your projects.
What is Argon2?
Argon2 is a password hashing algorithm designed to provide the highest level of security against brute force attacks. Developed in 2015, it won the Password Hashing Competition (PHC), beating out other contenders to become the new standard for password hashing.
Argon2 comes in three variants:
Argon2d: Designed for resistance against GPU-based brute force attacks.
Argon2i: Optimized for environments vulnerable to timing attacks.
Argon2id: Combines the strengths of both Argon2d and Argon2i and is the recommended variant for general use.
How Argon2 Works
At its core, Argon2 is a memory-hard function, meaning it requires a significant amount of memory to compute. This property makes it expensive for attackers to perform brute force attacks, particularly when using specialized hardware like GPUs or ASICs.
Key Features:
Memory Usage: Argon2 allows developers to specify how much memory the algorithm will consume.
Iterations (Time Cost): The number of passes the algorithm makes over the memory.
Parallelism: The degree of parallel threads Argon2 will use.
These parameters can be adjusted to balance security and performance, ensuring your application remains both fast and secure.
Why Use Argon2?
Security Advantages:
Memory Hardness: Makes attacks costly in terms of both time and hardware resources.
Resilience to Side-Channel Attacks: Protects against techniques like timing attacks.
Customizable for Future Needs: Easily adapts to evolving hardware capabilities.
Comparison to Older Algorithms:
Bcrypt: While secure, Bcrypt has limited memory usage and is slower to adapt to modern hardware.
PBKDF2: While widely used, it’s less secure against modern GPU and ASIC attacks.
Argon2 surpasses both by offering stronger protection and more customization options.
How to Implement Argon2
Example in PHP
PHP provides native support for Argon2 via the password_hash()
and password_verify()
functions. Here’s how to use them:
Hashing a Password:
$password = "securepassword123";
$hash = password_hash($password, PASSWORD_ARGON2ID);
echo "Hashed Password: $hash";
Verifying a Password:
$storedHash = '$argon2id$v=19$m=65536,t=4,p=1$...'; // Example hash
if (password_verify($password, $storedHash)) {
echo "Password is correct!";
} else {
echo "Invalid password.";
}
Customizing Argon2 Options:
$options = [
'memory_cost' => 1 << 17, // 128 MB
'time_cost' => 4, // Iterations
'threads' => 2 // Parallelism
];
$hash = password_hash($password, PASSWORD_ARGON2ID, $options);
Example in Python
Here’s how to use Argon2 for password hashing in Python. The argon2-cffi
library is a popular choice for this purpose. If you haven’t already installed it, you can do so using:
pip install argon2-cffi
Hashing a Password in Python
from argon2 import PasswordHasher
# Initialize the PasswordHasher
ph = PasswordHasher()
# Hash a password
password = "securepassword123"
hashed_password = ph.hash(password)
print("Hashed Password:", hashed_password)
Verifying a Password in Python
try:
# Verifying the password
if ph.verify(hashed_password, password):
print("Password is correct!")
except Exception as e:
print("Invalid password:", str(e))
Rehashing a Password in Python
To ensure passwords are hashed with updated parameters, you can check if rehashing is needed.
if ph.check_needs_rehash(hashed_password):
hashed_password = ph.hash(password)
print("Password rehashed:", hashed_password)
Customizing Argon2 Parameters
You can customize the Argon2 parameters by configuring the PasswordHasher
class.
ph = PasswordHasher(
time_cost=3, # Number of iterations
memory_cost=65536, # Memory usage in KB
parallelism=2 # Number of parallel threads
)
# Hash a password with custom parameters
hashed_password = ph.hash(password)
print("Custom Hashed Password:", hashed_password)
Real-World Applications
Argon2 is increasingly used in modern authentication systems, password managers, and even cryptocurrency wallets. Its adoption by platforms like PHP’s password hashing API shows its growing importance in the developer community.
Challenges and Considerations
While Argon2 is a powerful tool, it’s not without challenges:
Performance Costs: Overly aggressive memory or iteration settings can impact application performance.
Compatibility: Ensure your hosting environment supports Argon2. For example, Argon2 requires PHP 7.2 or higher.
Testing and benchmarking are essential to find the right balance for your specific use case.
Conclusion
Argon2 is a game-changer in password hashing, providing unmatched security and flexibility for modern applications. Its memory-hard design and configurability make it a future-proof choice for developers aiming to protect user data.
By implementing Argon2 today, you’re not just securing passwords—you’re taking a stand against the evolving threats of tomorrow.
Start implementing Argon2 in your projects now, and let security be your competitive advantage!
Subscribe to my newsletter
Read articles from Douglas Sabwa Indumwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Douglas Sabwa Indumwa
Douglas Sabwa Indumwa
I am a full-stack software developer driven by the goal of creating scalable solutions to automate business processes. Throughout my career, I have successfully developed web applications that serve thousands of users, both for-profit and non-profit. I am currently focusing on expanding my skills in DevSecOps.