React Roadmap for Beginners ๐ค

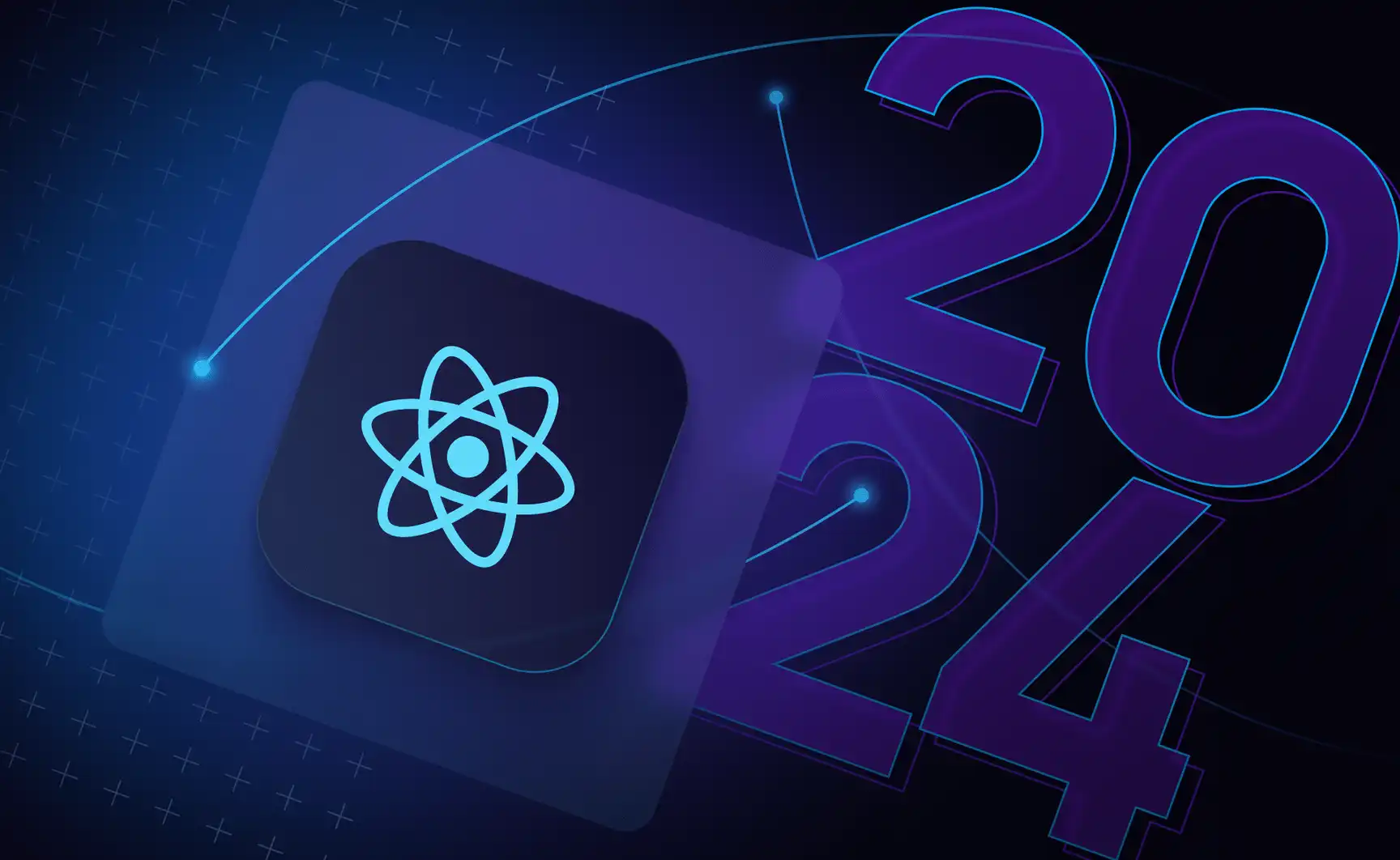
React is a JavaScript library used for building user interfaces, primarily for web applications. It was developed and is maintained by Meta (formerly Facebook) and has become one of the most popular tools for front-end development.
Key Features of React ๐ :
Component-Based Architecture ๐๏ธ:
React applications are built using reusable, independent components.
Each component can have its own logic and design, making development and maintenance easier.
Declarative UI ๐จ :
React uses a declarative programming style to describe how the UI should look for different states.
You focus on "what" the UI should do, and React takes care of the "how."
๐ Virtual DOM :
React uses a Virtual DOM (a lightweight copy of the actual DOM) to improve performance.
When changes occur, React updates only the necessary parts of the actual DOM, making it efficient.
JSX (JavaScript XML) ๐ :
- React uses JSX, a syntax extension that allows you to write HTML-like code within JavaScript.
const element = <h1>Hello, world!</h1>;
Unidirectional Data Flow ๐ :
- Data flows in a single direction (from parent to child components), making the application's state easier to manage and debug.
Rich Ecosystem and Community Support ๐๐ค:
React has a vast ecosystem of tools, libraries, and plugins to extend its functionality.
It is widely supported with a large community and extensive documentation.
Why Use React? ๐
Performance: The Virtual DOM ensures fast updates and rendering.
Reusability: Component-based structure encourages code reuse.
Flexibility: Can be used for single-page applications, mobile apps (React Native), and even server-rendered apps.
Tooling: Rich tools like React Developer Tools and integration with libraries like Redux for state management.
Example: A Simple React Component ๐:
import React from 'react';
function Greeting() {
return <h1>Hello, Vishal Singh!</h1>;
}
export default Greeting;
This example defines a simple React component that displays a greeting. ๐
React Learning Topics :
Basics of React ๐ :
Introduction to React
Setting up a React Application
JSX Syntax and Basics
Components and Props
State and useState Hook
Handling Events
Intermediate React ๐โจ:
Lifecycle Methods and useEffect Hook
React Router Basics
Forms and Controlled Components
Conditional Rendering
Lists and Keys in React
Styling Approaches (CSS, SCSS, Tailwind CSS)
Fetching Data with APIs
Advanced React ๐ :
Context API
Advanced State Management (Redux, Zustand)
Performance Optimization
Custom Hooks
Lazy Loading and Code Splitting
Error Boundaries
Ecosystem and Integration ๐๐ :
Type Checking with PropTypes or TypeScript
Server-Side Rendering (Next.js, Remix)
Testing React Applications (Jest, React Testing Library)
GraphQL Integration (Apollo Client)
Specialized Topics ๐ :
Progressive Web Apps (PWAs)
React Native for Mobile Development
Building Reusable Components and Libraries
Deployment and Best Practices ๐โจ :
Deploying React Applications
Best Practices for React Development
Debugging and Troubleshooting React Applications
Check out my React blogs to learn these topics and dive into other exciting topics in Rera development! ๐๐กโจ
Subscribe to my newsletter
Read articles from Vishal Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
