Laravel Broken Authentication: How to Detect and Fix It

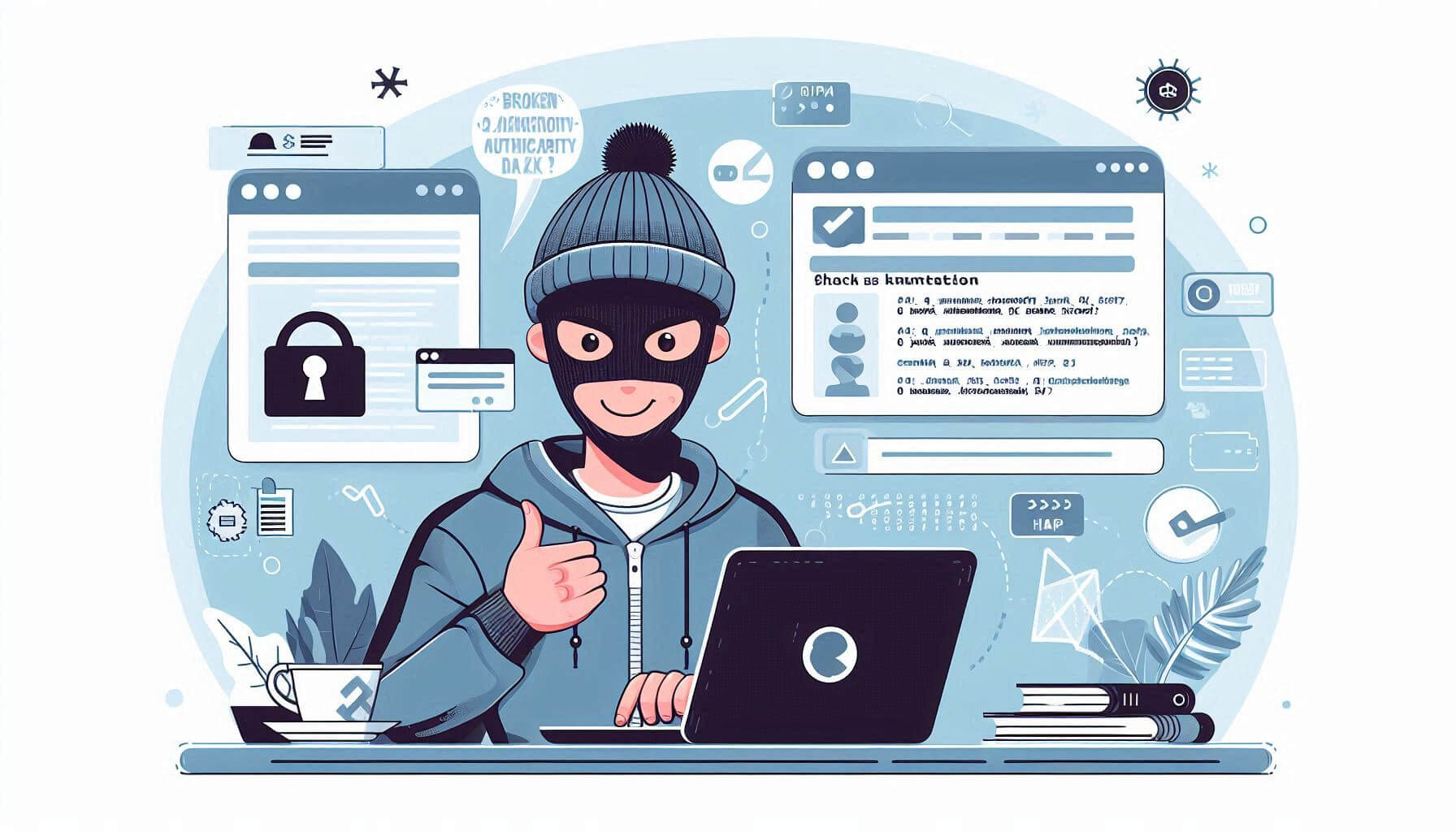
Broken authentication is a critical security vulnerability that exposes web applications to unauthorized access. For Laravel developers, ensuring robust authentication mechanisms is vital to safeguard user data and maintain trust.
In this post, we’ll explore:
What is broken authentication?
How it impacts Laravel applications.
How to detect it using our free Website Security Scanner.
A coding example to mitigate it.
What is Broken Authentication?
Broken authentication occurs when a web application's authentication process can be bypassed or exploited due to flaws such as:
Weak password policies.
Exposed session IDs.
Poor implementation of multi-factor authentication (MFA).
In Laravel applications, the misconfiguration of authentication routes or weak password hashing can be the primary culprits.
Impacts of Broken Authentication
Failing to address broken authentication can lead to:
Unauthorized access to sensitive data.
Privilege escalation attacks.
Compromised user accounts.
Let’s delve into how to detect these vulnerabilities and fix them.
Detecting Vulnerabilities with Free Tools
Before diving into code, it's essential to analyze your Laravel application for broken authentication vulnerabilities. Use our Website Security Checker Tool to perform a comprehensive assessment.
Security Checker Tool Overview
This tool scans your website for vulnerabilities, including issues related to authentication mechanisms. After running the scan, you’ll receive a detailed report highlighting areas needing attention.
Coding Example: Mitigating Broken Authentication in Laravel
Below is an example of how to enforce stricter authentication practices in Laravel using bcrypt for hashing passwords and implementing session management.
Secure Password Hashing
Ensure passwords are hashed securely using Laravel’s bcrypt
:
use Illuminate\Support\Facades\Hash;
// Storing hashed password
$user->password = Hash::make('your_password');
$user->save();
// Verifying password during login
if (Hash::check('your_password', $user->password)) {
// Login successful
} else {
// Authentication failed
}
Enforcing Multi-Factor Authentication (MFA)
Add MFA to strengthen authentication:
// Example using Laravel package for OTP (One Time Password)
use OTPAuth;
$otp = new OTPAuth\TOTP('Label', 'SecretKey');
echo 'Your OTP is: ' . $otp->now();
Session Management
To prevent session hijacking, secure your sessions in the config/session.php
:
'secure' => env('SESSION_SECURE_COOKIE', true),
'http_only' => true,
'same_site' => 'strict',
Using Vulnerability Assessment Reports for Improvements
After implementing these changes, re-scan your website using our Website Security Checker.
Vulnerability Assessment Report
The report will confirm whether your fixes have addressed the identified vulnerabilities.
Conclusion
Securing Laravel applications against broken authentication is crucial for protecting your users and business reputation. By combining robust coding practices with tools like our free Website Security Checker, you can effectively identify and mitigate these vulnerabilities.
Ready to secure your application? Scan your website for free today!
Subscribe to my newsletter
Read articles from Pentest_Testing_Corp directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pentest_Testing_Corp
Pentest_Testing_Corp
Pentest Testing Corp. offers advanced penetration testing to identify vulnerabilities and secure businesses in the USA and UK, helping safeguard data and strengthen defenses against evolving cyber threats. Visit us at free.pentesttesting.com now to get a Free Website Security Check.