Day 8 : Shell Scripting Challenge
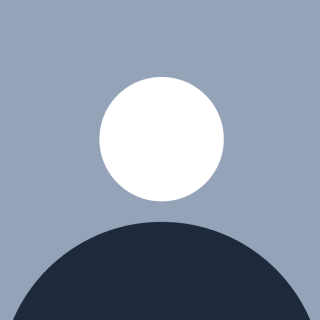
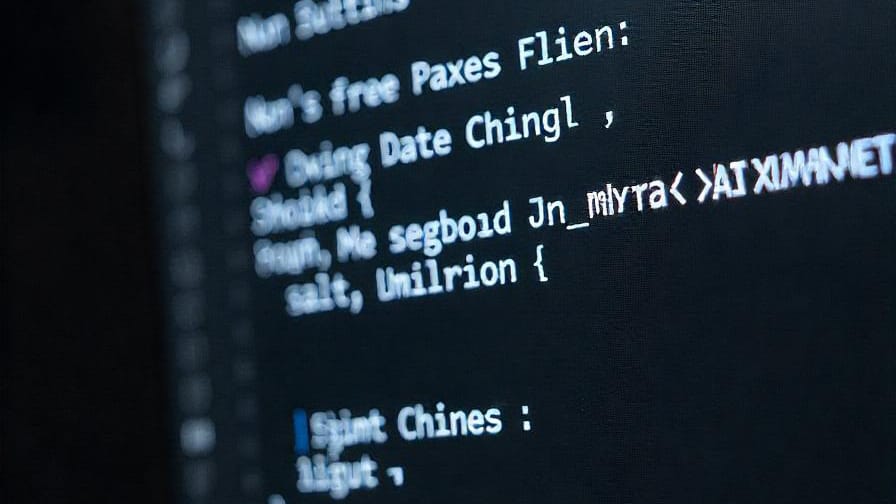
Today we have some tasks where we will know about Shell Scripting. What is Comments, echo, variables, wildcards.
Tasks :
Task 1 : Comments : In bash scripts, comments are used to add explanatory notes or disable certain lines of code.
- Your task is to create a bash script with comments explaining what the script does.
Task 2 : Echo : The echo command is used to display messages on the terminal.
- Your task is to create a bash script that uses echo to print a message of your choice.
Task 3 : Variables : Variables in bash are used to store data and can be referenced by their name.
- Your task is to create a bash script that declares variables and assigns values to them.
Task 4 : Using Variables : Now that you have declared variables, let's use them to perform a simple task.
- Create a bash script that takes two variables (numbers) as input and prints their sum using those variables.
Task 5 : Using Built-in Variables : Bash provides several built-in variables that hold useful information.
- Your task is to create a bash script that utilizes at least three different built-in variables to display relevant information.
Task 6 : Wildcards : Wildcards are special characters used to perform pattern matching when working with files.
- Your task is to create a bash script that utilizes wildcards to list all the files with a specific extension in a directory.
Solutions :
All Solutions are in a sinle script, read the following script :
#!/bin/bash
# Task 1 Solution :
# In this script, We will know about Comments, Echo, Variables and Wildcards.
# First of all what is comments?
# You are reading this line after '#' is a single line comment.
<< note
This is a multi-line comment. We use this when we need to write so many lines
as a comment. We write '<<' in the beginning and give some name like 'note' and
end it with the same name 'note'. In between these, whatever you write is a
Multi-line comment.
Comments are non executable lines in a script/code.
note
# Task 2 Solution :
# Echo is a command that display/print something like for Eg.
echo "This script contains Basics of Shell Scripting."
echo ""
# This line will be display after you execute this script. That blank 'echo ""' will skip a
# line between two commands
# Task 3 Solution :
# Variables are the thing which stores some values, values can be Numbers, Characters, etc.
variable1="Hello!"
# 'variable1' is variable name, you can give name as per your choice. '=' is to assign
# values to the varable. And "Hello!" is the value that is assigned to a variable.
# Task 4 Solution :
# Lets take two variables and get values from user
read -p "Enter 1st No : " num1
read -p "Enter 2nd No : " num2
sum=$((num1 + num2))
echo ""
echo "The Addition is : $sum"
echo ""
# 'read -p' accept value from user with displaying message and store in 'num1' 'num2' variable
# Then addition of both nums stored in 'sum' variable
# Task 5 Solution :
# Some built-in variables :
echo ""
echo "Logged in as : $USER"
echo "Home directory is : $HOME"
echo "Present working directory is : $PWD"
echo ""
# Task 6 Solution :
# Some common wildcards are '*' '?' '[]'
# Suppose if I want to display details about all files which have '.txt' extension at end
# So we write
ls -l *.txt
# '*' means whatever name of file but with extension '.txt'
# Suppose we write 'file_name.*' it means 'file_name' file with whatever extension
After giving execute permission by sudo chmod +x
and executing by ./Shell_Scripting_Basics.sh
you will be able to see like this.
so here we completed our today’s task. Follow and keep learning with us.
LinkedIn | GitHub | Hashnode
Subscribe to my newsletter
Read articles from Saad Asif Mujawar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by