The Pragmatic Programming for Modern Developers
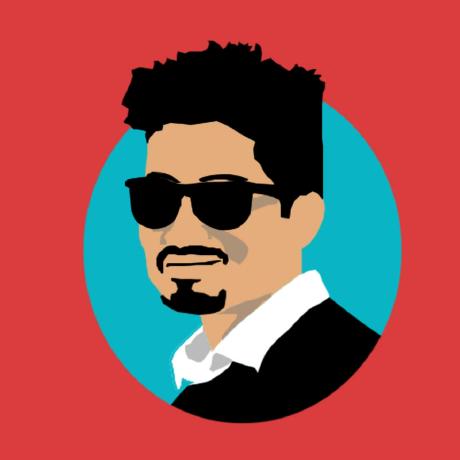
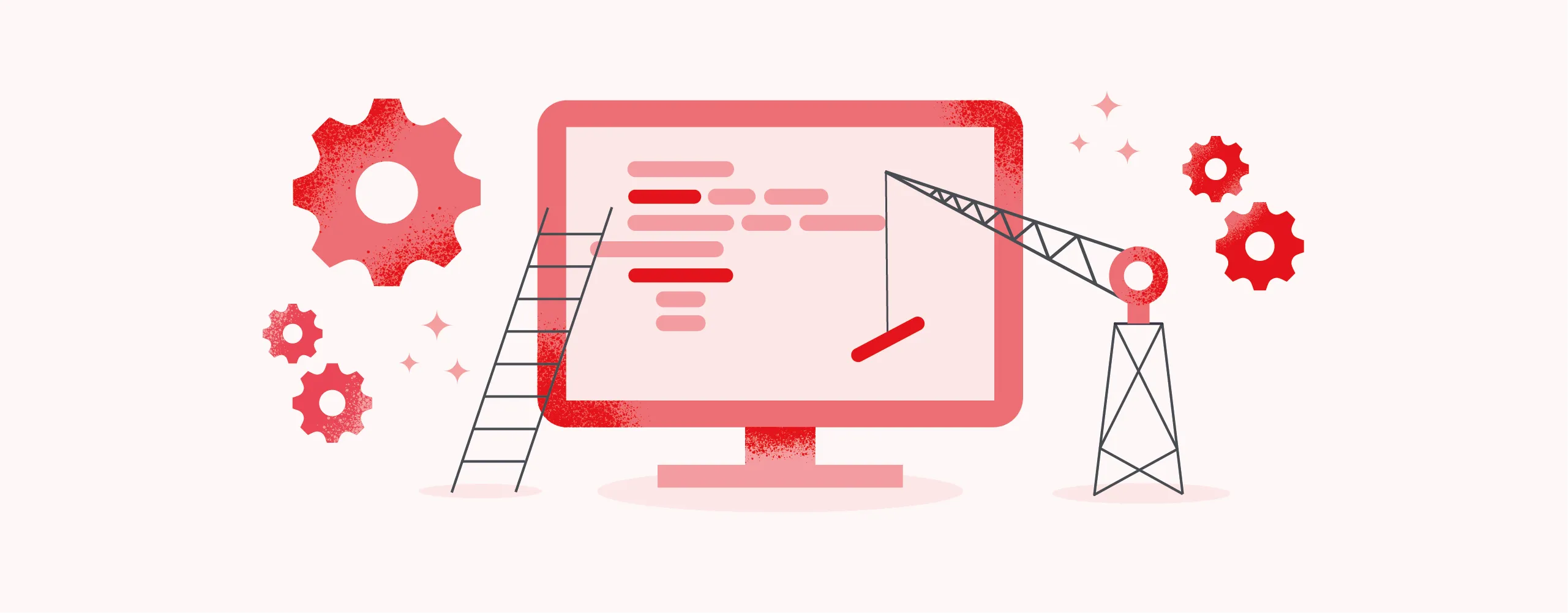
“The greatest challenges for a software developer today aren’t purely technical—they’re about navigating complexity, adapting to change, and creating lasting impact. ”
Software development has come a long way since The Pragmatic Programmer was first published in 1999. Yet, the principles outlined by Andrew Hunt and David Thomas remain strikingly relevant. As experienced developers, our challenges today include managing complex systems, adapting to rapidly evolving technologies, and mentoring the next generation of programmers.
This comprehensive guide revisits The Pragmatic Programmer through the lens of a modern developer. It integrates timeless wisdom with contemporary practices, providing an all-in-one reference for mastering the craft of software development in today’s world.
Why Pragmatism Matters More Than Ever
Pragmatism is the art of making thoughtful, practical decisions. It’s not about following rules blindly or cutting corners—it’s about balancing efficiency, quality, and impact. For modern developers, pragmatism is essential for:
Navigating Complexity: Distributed systems, microservices, and cloud-native architectures make even simple tasks intricate.
Managing Change: Frameworks and tools evolve at breakneck speed. Pragmatism helps you choose the right technologies for the long term.
Maximizing Impact: It’s about focusing on what truly matters—delivering value to users and stakeholders.
Core Principles of Pragmatic Programming
1. DRY (Don’t Repeat Yourself)
“Every piece of knowledge must have a single, unambiguous, authoritative representation within a system.”
Modern Applications of DRY
In Code: Avoid duplicating logic. Extract reusable modules, libraries, or services.
In CI/CD: Use templates for deployment pipelines to ensure consistency.
In Documentation: Maintain centralized, single-source-of-truth documentation.
When to Break DRY
Performance optimizations may require duplication (e.g., caching strategies).
Microservices might duplicate logic to reduce coupling and ensure independence.
Pro Tips
Use tools like Terraform modules for reusable infrastructure as code.
Centralize error handling and logging frameworks across services.
2. Orthogonality
“Keep things independent. When you change one thing, you shouldn’t have to change another.”
Modern Examples
In API Design: Decouple internal implementations from external contracts using tools like OpenAPI or gRPC.
In Microservices: Separate data stores and business logic for individual services to avoid ripple effects.
In Infrastructure: Use containerization to isolate dependencies.
Practical Implementation
Feature flags allow teams to release code without coupling it to the release process.
Service meshes (e.g., Istio) abstract network-level concerns from application logic.
3. Tracer Bullets
“Tracer bullets let you find your target incrementally by delivering small, functional increments.”
Tracer bullets contrast with prototypes by being real, functional implementations that evolve into the final product.
Modern Use Cases
Canary Deployments: Test features with a subset of users before a full rollout.
Feature Flags: Use tools like LaunchDarkly to test incomplete features in production.
Iterative Development: Start with end-to-end functionality, then refine.
Pro Tips
Treat initial implementations as production-ready, even if they’re incomplete.
Collect metrics and feedback early to guide further development.
The Pragmatic Toolkit for Modern Developers
1. Master Your Tools
“A craftsman is only as good as their tools.”
Essential Modern Tools
Editors and IDEs: Master tools like JetBrains IntelliJ, VS Code, or Vim. Customize them with plugins and shortcuts.
Version Control: Leverage advanced Git workflows like rebasing, cherry-picking, and subtree merging.
Debugging Distributed Systems: Use tools like Jaeger, OpenTelemetry, and Sentry for observability.
Pro Tips
Automate repetitive tasks with custom scripts.
Learn CLI tools (e.g., jq, grep, sed) to process logs and JSON efficiently.
2. Automate Everything
“Anything you do more than twice should be automated.”
Automation reduces toil and increases consistency. From builds to monitoring, automation is essential in modern development.
What to Automate
CI/CD Pipelines: Use Jenkins, GitHub Actions, or GitLab CI for automated testing and deployments.
Infrastructure: Provision and manage resources using Terraform or Pulumi.
Incident Response: Automate runbooks and alerts with tools like PagerDuty and Opsgenie.
Pro Tips
Use GitOps to manage infrastructure declaratively.
Implement automated chaos testing to ensure system resilience.
Pragmatic Coding Practices
1. Refactor Ruthlessly
“Leave the code cleaner than you found it.”
Refactoring isn’t a one-time event; it’s a daily habit. Regular refactoring reduces technical debt and keeps code maintainable.
Modern Tools for Refactoring
IDEs with refactoring support (e.g., IntelliJ, PyCharm).
Static analysis tools like SonarQube to identify problem areas.
Linters and formatters like ESLint and Prettier for consistent code styles.
Pro Tips
Focus on small, incremental improvements.
Prioritize high-impact areas when refactoring.
2. Test Early, Test Often
“Testing is integral to development, not an afterthought.”
A robust test suite is your safety net in an ever-changing codebase.
Modern Test Strategies
Unit Tests: Test individual components for correctness.
Integration Tests: Validate interactions between modules.
End-to-End Tests: Simulate real user flows.
Tools to Use
UI Testing: Cypress, Playwright.
API Testing: Postman, REST-assured.
Load Testing: k6, Locust.
Pro Tips
Shift testing left in the development cycle.
Use test coverage metrics wisely—don’t aim for 100% coverage if it doesn’t add value.
Pragmatic Leadership and Mentorship
As a senior developer, your role extends beyond writing code. You’re a mentor, a decision-maker, and a cultural influencer.
1. Be a Mentor
Share knowledge generously.
Encourage junior developers to take ownership.
Provide actionable, constructive feedback.
2. Manage Technical Debt
Treat technical debt like financial debt—track, prioritize, and pay it down regularly.
Use tools like CodeScene to visualize and manage debt.
Scaling Pragmatism Across Teams
1. Build Orthogonal Teams
Foster cross-functional teams with clear responsibilities.
Use retrospectives to continuously improve team processes.
2. Communicate Clearly
Translate technical challenges into business terms for stakeholders.
Document decisions to avoid miscommunication.
The Pragmatic Programmer’s Career Compass
Pragmatism isn’t just about systems—it’s about your career. As a senior developer, continuous learning is non-negotiable.
1. Build Your Knowledge Portfolio
Invest in new technologies, but focus on concepts over tools.
Experiment with side projects to stay sharp.
2. Avoid Burnout
Set boundaries to maintain work-life balance.
Delegate tasks that don’t require your expertise.
Conclusion: Pragmatism for Life
The principles of The Pragmatic Programmer transcend coding. They’re a philosophy for solving problems, making decisions, and creating value. In an industry that’s constantly changing, these principles are your anchor.
By embracing pragmatism, you’ll not only write better code—you’ll build better systems, better teams, and a better career.
Appendices
1. Tools and Frameworks Cheat Sheet
Debugging: Jaeger, Sentry.
Testing: Cypress, Pytest.
Automation: Jenkins, Terraform.
2. Glossary of Pragmatic Concepts
DRY: Don’t Repeat Yourself.
Orthogonality: Independence between components.
Tracer Bullets: Incremental, functional development.
3. Further Reading
Clean Code by Robert C. Martin.
Accelerate by Nicole Forsgren, Jez Humble, and Gene Kim.
This comprehensive guide serves as your one-stop reference to the pragmatic principles every modern developer needs. Bookmark it, share it, and live by it—it’s a map to navigate the complexity of modern software development with confidence and clarity.
To discuss things like these over a cup of tea, feel free to reach out to me at AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
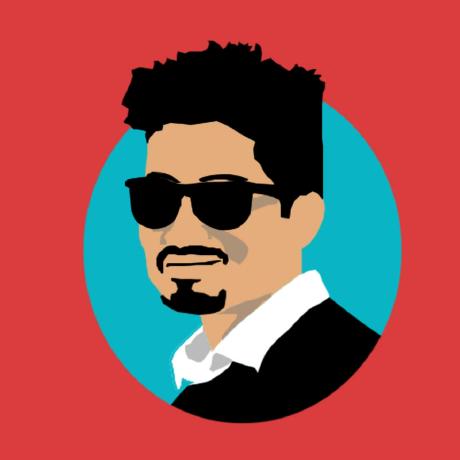