Flutter Chips Widget: A Comprehensive Guide with Examples
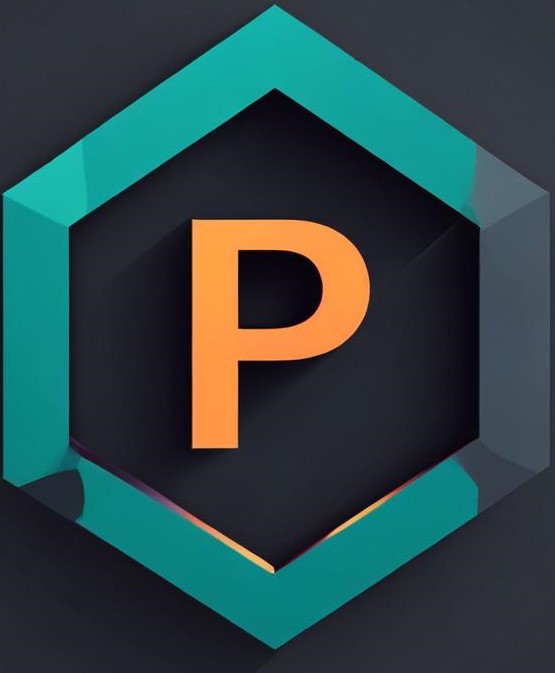
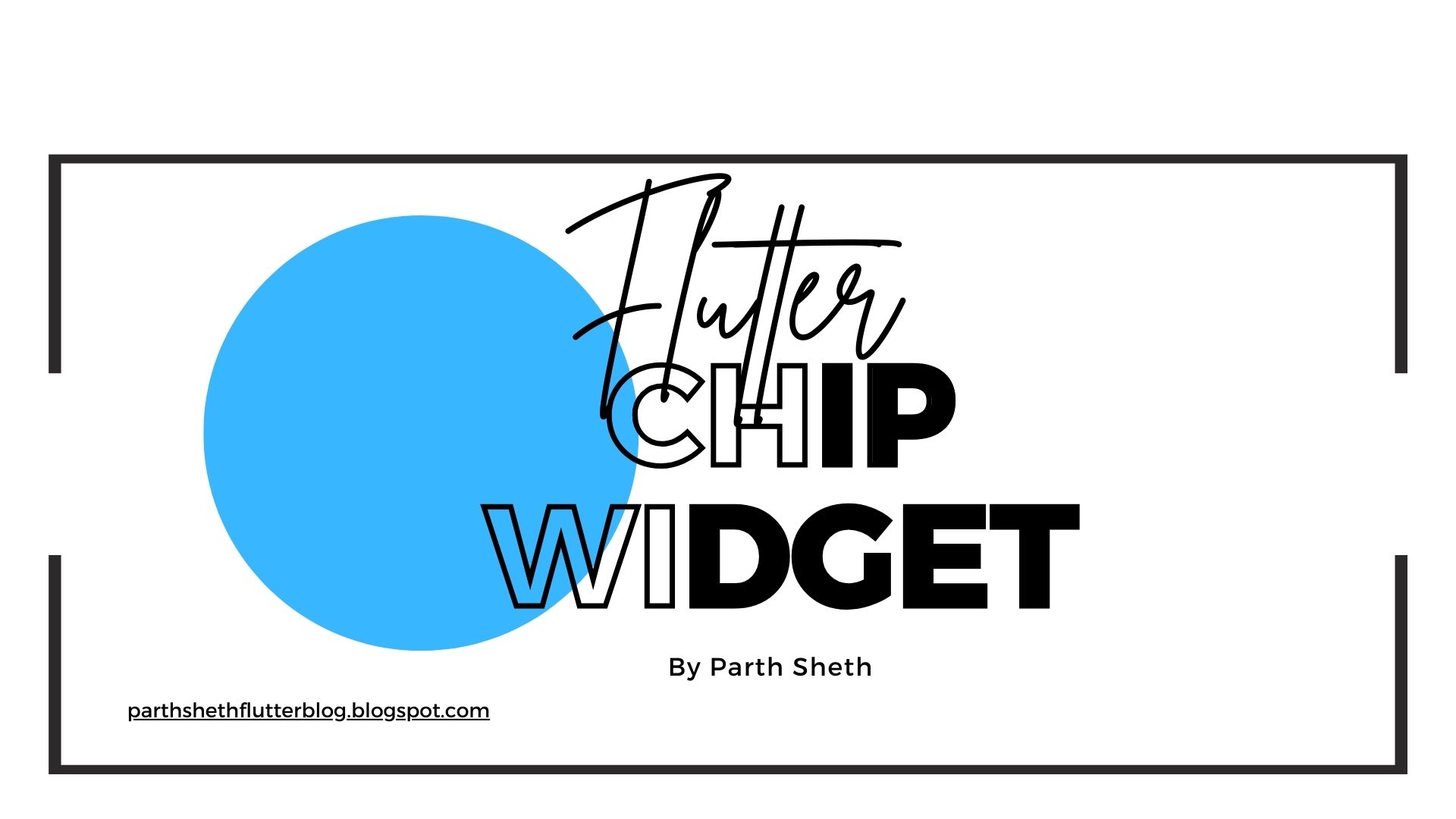
In Flutter, the Chip
widget is an excellent way to display compact elements, such as tags, categories, or interactive buttons. It allows you to present information in a clean, concise manner while offering a variety of customization options. Chips can also be interactive, allowing users to perform actions like selection or deletion.
What is the Flutter Chip Widget?
A Chip is a small, interactive UI element that typically displays a label (text) and can optionally include an icon or an action (e.g., delete or select). It is often used for representing tags, contacts, or choices in a compact form.
Basic Syntax of the Chip Widget
Here’s a simple example of the Chip
widget:
Chip(
label: Text('Flutter Chip'),
)
This basic chip consists of just a label with the text "Flutter Chip." The Chip
widget is used for displaying simple pieces of information, often within lists, forms, or filters.
Types of Chips in Flutter
Flutter provides several types of chips, each serving different use cases:
Basic Chip: A simple chip that displays a label.
Action Chip: A chip that triggers an action when clicked.
Choice Chip: A chip used for making selections, like choosing from a set of options.
Filter Chip: A chip that allows the user to select multiple options for filtering.
Input Chip: A chip representing user input, such as an email address or tag.
Example 1: Basic Chip
The most basic form of the Chip
widget is a chip with a label, representing simple tags or categories.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Chips Example'),
),
body: Center(
child: Chip(
label: Text('Technology'),
),
),
),
);
}
}
Properties of Chip
The Chip
widget has several properties that can be customized:
label: A widget to display the chip's content, typically a
Text
widget.avatar: An optional widget, usually an icon or image, displayed at the start of the chip.
deleteIcon: An icon that appears on the chip when it can be deleted, typically shown on the right side.
onDeleted: A callback function triggered when the delete icon is tapped.
backgroundColor: The background color of the chip.
labelStyle: Customizes the style of the text within the chip.
padding: Adjusts the padding around the label inside the chip.
Example 2: Action Chip
An Action Chip can trigger an action when clicked. This is useful when you want a chip to perform an action like opening a dialog or making an API call.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Action Chip Example'),
),
body: Center(
child: ActionChip(
label: Text('Delete Item'),
onPressed: () {
// Action triggered when the chip is pressed
print('Item deleted!');
},
),
),
),
);
}
}
Properties of ActionChip
The ActionChip
widget has a few properties to customize its behavior:
label: The content inside the chip, typically a
Text
widget.onPressed: The callback that’s called when the chip is clicked.
backgroundColor: The background color of the chip.
labelStyle: A style to customize the label's appearance.
elevation: Defines the shadow of the chip when it's displayed.
Example 3: Choice Chip
A Choice Chip is used when you want users to select one option from a list. These chips are often used for toggling between options.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
bool isSelected = false;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Choice Chip Example'),
),
body: Center(
child: ChoiceChip(
label: Text('Select Option'),
selected: isSelected,
onSelected: (bool selected) {
setState(() {
isSelected = selected;
});
},
),
),
),
);
}
}
Properties of ChoiceChip
The ChoiceChip
widget is specifically used for toggling between two states (selected or unselected). Its properties include:
label: A widget, typically a
Text
widget, representing the label for the chip.selected: A boolean value that determines if the chip is selected or not.
onSelected: A callback that updates the selected state when the chip is tapped.
backgroundColor: The background color of the chip when it's unselected.
selectedColor: The background color of the chip when it's selected.
labelStyle: Customizes the text style of the label.
elevation: Adds shadow to the chip when it's selected.
Example 4: Filter Chip
A Filter Chip is a special type of chip that can represent one or more selected filter options. It is used in scenarios like search filtering, where the user selects multiple filters from a list.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
List<String> selectedTags = [];
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Filter Chip Example'),
),
body: Center(
child: Wrap(
spacing: 10,
children: ['Flutter', 'Dart', 'React', 'JavaScript'].map((tag) {
return FilterChip(
label: Text(tag),
selected: selectedTags.contains(tag),
onSelected: (bool selected) {
setState(() {
selected
? selectedTags.add(tag)
: selectedTags.remove(tag);
});
},
);
}).toList(),
),
),
),
);
}
}
Properties of FilterChip
The FilterChip
widget is used for multiple selection:
label: A widget that represents the label of the chip, typically a
Text
widget.selected: A boolean that determines if the chip is selected.
onSelected: A callback function triggered when the chip is selected or deselected.
backgroundColor: The color of the chip when it’s unselected.
selectedColor: The color of the chip when it’s selected.
labelStyle: Customizes the style of the label.
avatar: Adds an optional avatar (icon/image) to the chip.
elevation: Sets the shadow effect when the chip is in the selected state.
Common Chip Properties
Here’s a summary of the most common properties for chips in Flutter:
label: The content inside the chip (typically a
Text
widget).avatar: Adds an icon or image to the left of the chip's label.
deleteIcon: An icon that is shown when the chip is deletable.
onDeleted: A callback function for when the delete icon is pressed.
backgroundColor: The background color of the chip.
selected: A boolean that defines whether the chip is selected or not (used in
ChoiceChip
andFilterChip
).selectedColor: The background color when the chip is selected.
labelStyle: Customizes the style of the label text inside the chip.
shape: Defines the shape of the chip, such as a rounded or circular shape.
elevation: Controls the shadow depth for the chip, giving it a lifted effect.
padding: Adjusts the padding around the label inside the chip.
Conclusion
The Chip
widget in Flutter is a versatile tool for displaying compact, interactive elements like tags, filters, or action buttons. By customizing properties such as label
, backgroundColor
, avatar
, and onDeleted
, you can easily adapt the chip to suit your app’s needs.
Whether you're displaying simple tags, providing selectable options, or offering interactive actions, chips help create a clean, efficient interface in Flutter apps. Experiment with the different chip types and their properties to build intuitive and engaging user experiences!
Happy coding with Flutter! 🚀
Subscribe to my newsletter
Read articles from Parth Sheth directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
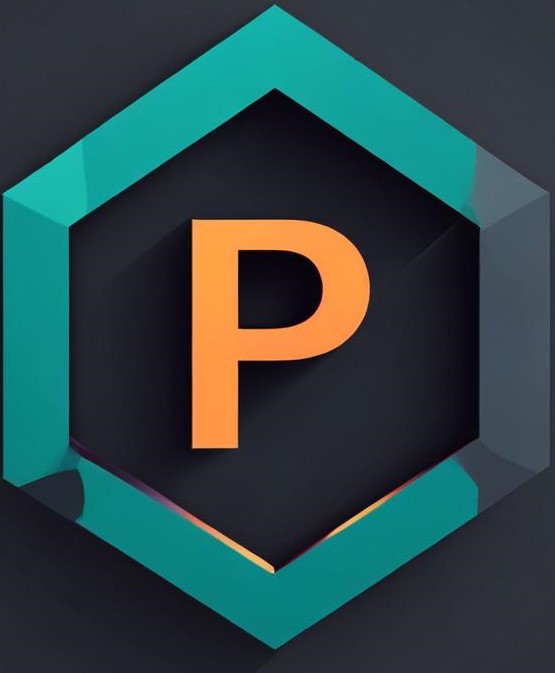
Parth Sheth
Parth Sheth
"🚀 Fluttering through the digital cosmos, I am Parth Sheth, a passionate architect of interactive marvels in the realm of Flutter development. With a fervent love for coding and an insatiable curiosity for the ever-evolving tech landscape, I embark on a perpetual quest to redefine the boundaries of innovation. From sleek UI designs to seamless user experiences, I orchestrate symphonies of code that resonate with elegance and functionality. 💻✨ My journey in Flutter is more than just a profession; it's a thrilling odyssey where creativity meets craftsmanship. Armed with a keen eye for detail and an arsenal of cutting-edge tools, I navigate the complexities of app development with finesse, crafting solutions that not only meet but exceed expectations. 🎨🔧 But beyond the lines of code lies a deeper commitment—to inspire, empower, and elevate. Through my words, my work, and my unwavering dedication to excellence, I strive to ignite the spark of imagination in others, fostering a community where innovation knows no bounds. 🌟💡 So come, fellow adventurers, and join me on this exhilarating expedition into the unknown. Together, let's sculpt the future, one pixel at a time, and unleash the full potential of Flutter's boundless possibilities. Connect with me, and let's embark on this extraordinary voyage—together, we shall chart new horizons and redefine what's possible in the digital landscape. 🌍🔍"