Mastering WebSockets in Node.js: Advanced Techniques
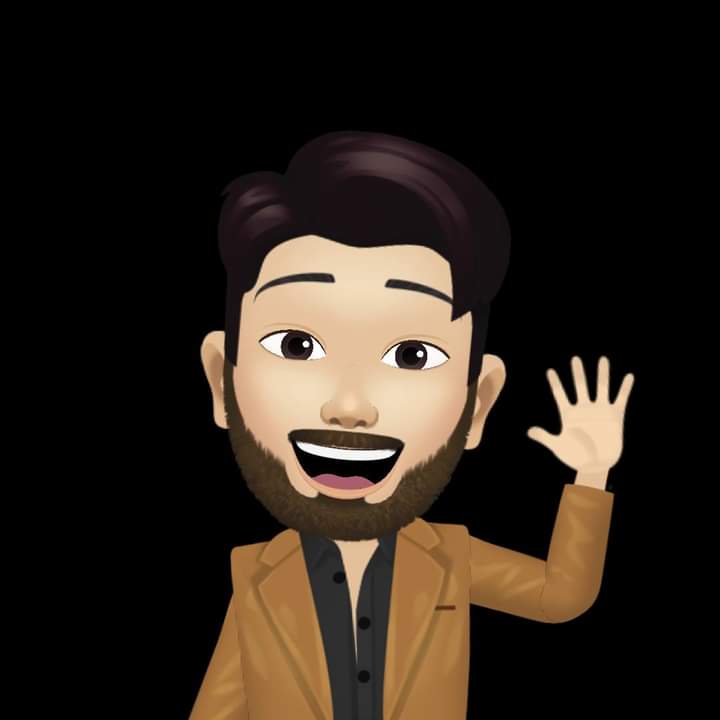
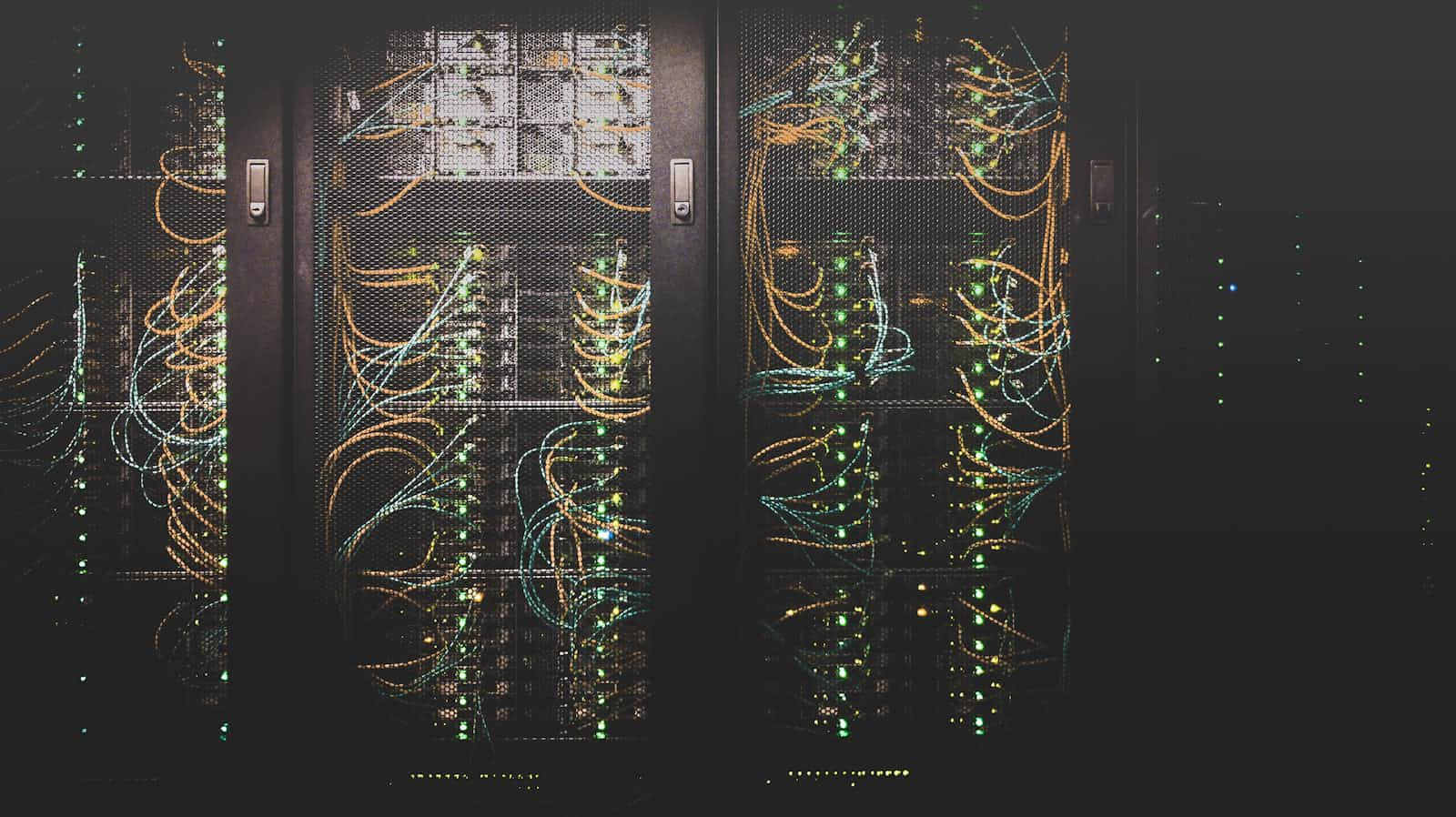
WebSockets have emerged as a key technology for real-time web applications, enabling bidirectional communication between clients and servers over a single, persistent connection. While WebSockets are not new, their potential is still vastly underutilized. In this blog post, we’ll explore the advanced usage of WebSockets in Node.js, why WebSockets are beneficial for certain types of applications, and how you can leverage their power to build scalable, real-time systems.
Introduction to WebSockets
Before diving into advanced concepts, let’s quickly review what WebSockets are and how they work.
A WebSocket is a protocol that allows for full-duplex communication channels over a single TCP connection. Unlike traditional HTTP, where the client sends a request and the server sends a response, WebSockets enable both the client and server to send messages to each other asynchronously. Once the connection is established, data can flow freely in both directions, with no need for additional handshakes or reconnections.
Node.js, being a non-blocking, event-driven environment, is particularly well-suited for handling WebSocket connections. The combination of WebSockets and Node.js allows developers to build applications where low-latency communication and real-time interactions are crucial.
Why WebSockets are Ideal for Certain Scenarios
WebSockets shine in applications that require real-time communication and low-latency updates. Here are some key scenarios where WebSockets are particularly beneficial:
Real-time messaging applications: Think of chat apps like Slack or WhatsApp, where users expect immediate delivery of messages.
Live sports updates: For applications that provide live score updates, WebSockets ensure that data is pushed to users without constant polling.
Collaborative tools: Tools like Google Docs or Figma, where multiple users interact with the same document or project in real-time.
Online gaming: Multiplayer games need low-latency, real-time communication between players and servers.
Stock market tracking: Financial applications require real-time updates on stock prices and market trends.
In all these cases, WebSockets allow for instantaneous, bidirectional communication, reducing the overhead associated with HTTP polling or long-polling techniques.
Getting Started with WebSockets in Node.js
Setting Up a Basic WebSocket Server
First, let's start by setting up a basic WebSocket server using Node.js. For this, we’ll use the popular ws
library, which provides a simple and efficient WebSocket implementation.
Install the ws
library via npm:
npm install ws
Now, let's create a simple WebSocket server:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
console.log('A new client connected');
// Send a welcome message to the client
ws.send('Hello, Client!');
// Listen for incoming messages from clients
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
});
// Handle connection closure
ws.on('close', () => {
console.log('Client disconnected');
});
});
console.log('WebSocket server is running on ws://localhost:8080');
In this example, the WebSocket server listens on port 8080
. When a client connects, the server sends a "Hello, Client!" message to the client, and it listens for any messages sent from the client. Upon disconnection, the server logs the event.
Simple WebSocket Client
Let’s create a basic WebSocket client that connects to the server we just created. You can use this client in a browser console or as part of a Node.js application.
const socket = new WebSocket('ws://localhost:8080');
// When the connection is established
socket.onopen = () => {
console.log('Connected to the WebSocket server');
socket.send('Hello, Server!');
};
// Handle incoming messages from the server
socket.onmessage = (event) => {
console.log('Received from server:', event.data);
};
// Handle errors
socket.onerror = (error) => {
console.error('WebSocket error:', error);
};
// Handle connection closure
socket.onclose = () => {
console.log('Disconnected from the server');
};
In this client code, we connect to the WebSocket server, send a message, and handle any responses or errors.
Advanced WebSocket Features
Broadcasting to Multiple Clients
One of the most powerful features of WebSockets is the ability to broadcast messages to multiple clients at once. This is essential for applications like live chats or real-time notifications.
Let’s say we want to send a message to all connected clients every time a new user joins. We can modify our WebSocket server like this:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
let clients = [];
wss.on('connection', (ws) => {
console.log('A new client connected');
clients.push(ws); // Add the client to the array
// Send a welcome message to the new client
ws.send('Welcome, new user!');
// Broadcast to all connected clients when a new user joins
clients.forEach((client) => {
if (client !== ws && client.readyState === WebSocket.OPEN) {
client.send('A new user has joined!');
}
});
// Listen for incoming messages from clients
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
});
// Handle connection closure
ws.on('close', () => {
console.log('Client disconnected');
clients = clients.filter(client => client !== ws); // Remove the client from the array
});
});
console.log('WebSocket server is running on ws://localhost:8080');
In this version, every time a new client connects, the server broadcasts a message to all other connected clients. The clients
array stores all connected WebSocket clients, and we filter it when a client disconnects to ensure the list stays up-to-date.
Handling Authentication with WebSockets
In real-world applications, you often need to authenticate users before allowing them to interact via WebSocket. One common approach is to use a token-based authentication system (e.g., JWT).
Here's how you could handle authentication before establishing a WebSocket connection:
const WebSocket = require('ws');
const jwt = require('jsonwebtoken');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws, req) => {
// Get token from query parameters (or headers)
const token = req.url.split('?token=')[1];
try {
// Verify token
const decoded = jwt.verify(token, 'your-secret-key');
console.log(`User ${decoded.username} connected`);
// If authenticated, allow connection
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
});
ws.send('Authentication successful!');
} catch (error) {
// If token is invalid, close the connection
console.log('Authentication failed:', error);
ws.close(1000, 'Authentication failed');
}
});
console.log('WebSocket server is running on ws://localhost:8080');
In this example, the WebSocket server expects a token
query parameter. The token is then verified using JWT. If the token is valid, the server allows communication; otherwise, it closes the connection.
Scaling WebSocket Servers
In production environments, scaling WebSocket servers can be tricky because WebSockets rely on long-lived connections. If your app grows and needs to handle thousands or millions of concurrent users, you need to consider how to scale your WebSocket server horizontally.
One approach to scaling is to use a message broker like Redis to synchronize state between multiple WebSocket server instances. Here's how you can use Redis Pub/Sub to broadcast messages across multiple server instances:
- Install the necessary dependencies:
npm install ws redis
- Create a scaled WebSocket server using Redis:
const WebSocket = require('ws');
const redis = require('redis');
const wss = new WebSocket.Server({ port: 8080 });
const pub = redis.createClient();
const sub = redis.createClient();
sub.subscribe('broadcast'); // Subscribe to the broadcast channel
wss.on('connection', (ws) => {
console.log('A new client connected');
// Send a welcome message
ws.send('Welcome to the WebSocket server!');
// Listen for incoming messages from clients
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
// Broadcast the message to all other clients
pub.publish('broadcast', message);
});
// Handle connection closure
ws.on('close', () => {
console.log('Client disconnected');
});
});
// Broadcast messages to all connected clients
sub.on('message', (channel, message) => {
wss.clients.forEach(client => {
if (client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
});
console.log('WebSocket server with Redis Pub/Sub is running on ws://localhost:8080');
In this example, we use Redis Pub/Sub to broadcast messages to all WebSocket clients, even across different instances of the WebSocket server. This ensures that no matter which server instance a client is connected to, they will still receive broadcast messages.
Conclusion
WebSockets offer significant advantages for building real-time applications, allowing for fast, bidirectional communication between clients and servers. In this post, we’ve covered the basics of setting up a WebSocket server in Node.js, as well as more advanced features
like broadcasting messages, handling authentication, and scaling WebSocket servers using Redis. By leveraging WebSockets effectively, you can create applications that feel more interactive and responsive, which is essential in today’s real-time, user-centric web.
Whether you're building a chat app, a collaborative platform, or a live data dashboard, WebSockets can help you deliver an engaging, real-time experience to your users.
Subscribe to my newsletter
Read articles from JealousGx directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
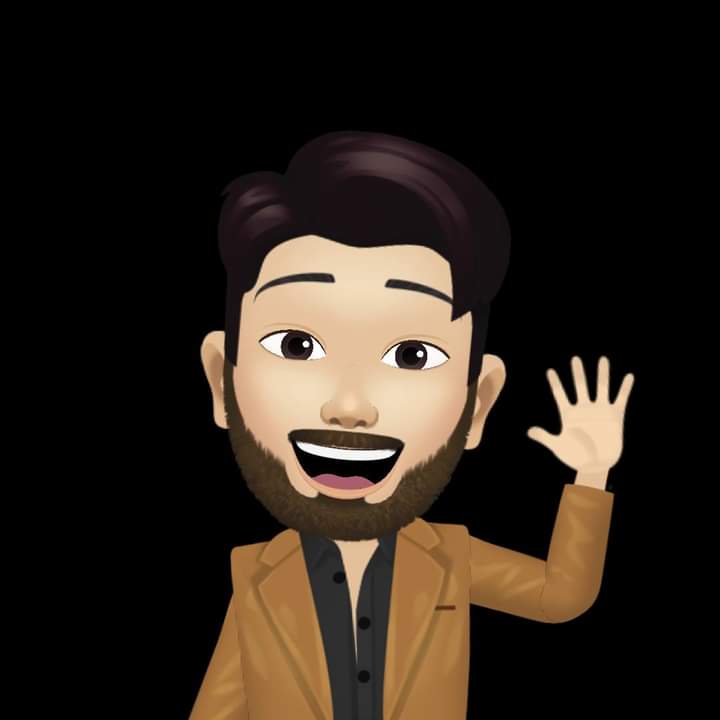
JealousGx
JealousGx
Hello, I'm a highly skilled full stack web developer with a rich background in creating and maintaining dynamic websites that drive online engagement and brand awareness. My expertise extends beyond WordPress to include a versatile skill set.