Fine-Tuning Llama 3.2 for Targeted Performance: A Step-by-Step Guide
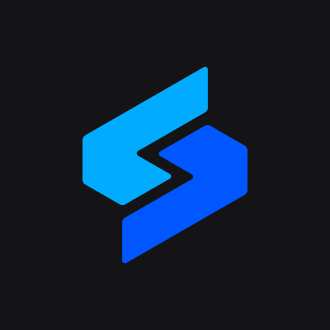
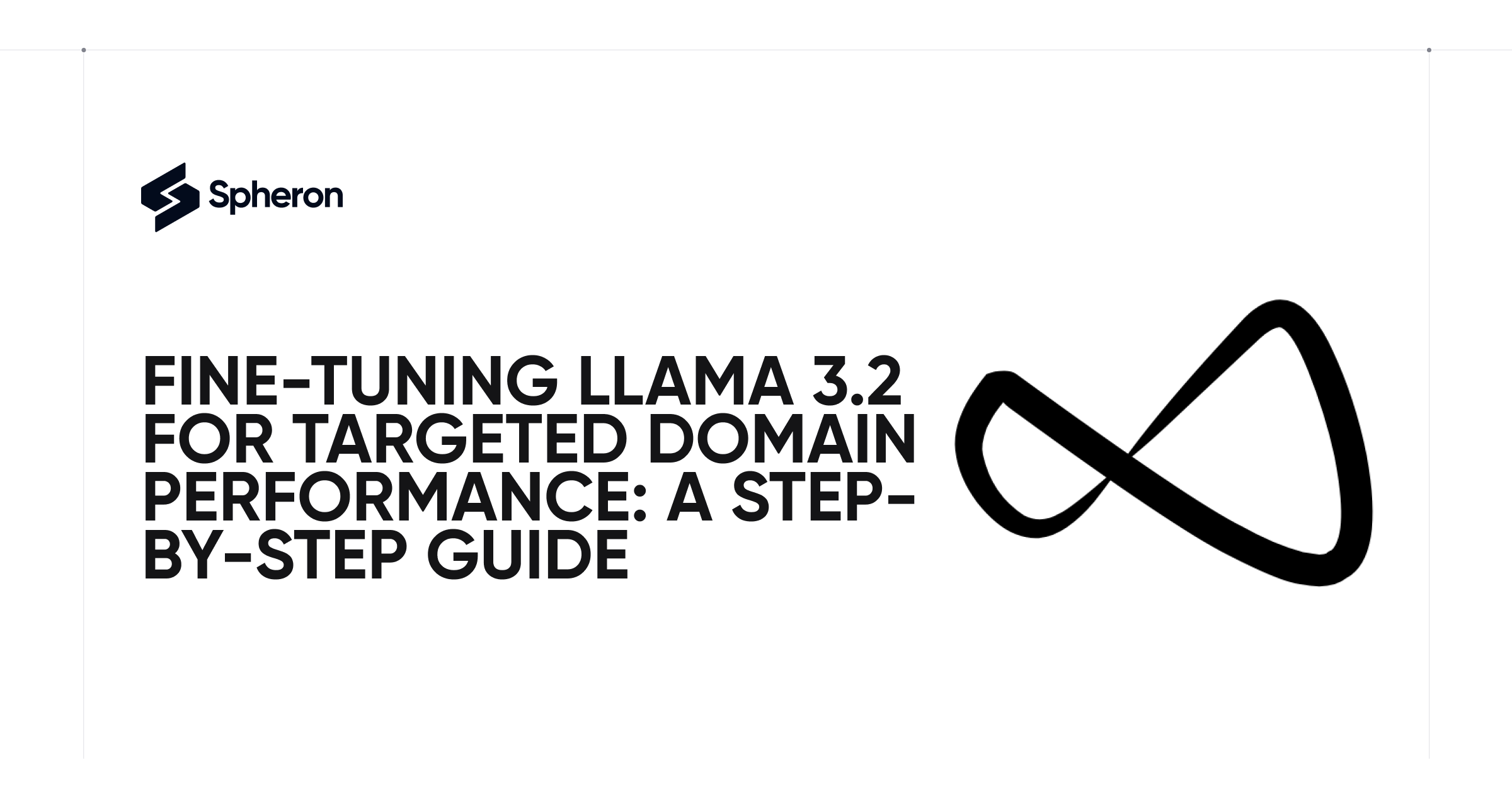
With the release of Meta’s Llama 3.2, fine-tuning large language models to perform well on targeted domains is increasingly feasible. This article provides a comprehensive guide on fine-tuning Llama 3.2 to elevate its performance on specific tasks, making it a powerful tool for machine learning engineers and data scientists looking to specialize their models.
Let’s dive into the fine-tuning process, requirements, setup steps, and how to test your model for optimal performance.
Why Fine-Tune Llama 3.2?
While large language models (LLMs) like Llama 3.2 and GPT-4 have powerful generalization capabilities, fine-tuning a model tailors its behavior to meet specialized requirements. For example, a fine-tuned model trained for a customer support domain can provide more accurate responses than a general-purpose model. Fine-tuning allows LLMs to outperform general models by optimizing them for specific fields, which is essential for tasks requiring domain-specific knowledge.
In this guide, we’ll cover how to fine-tune Llama 3.2 locally and use it to solve math problems as a simple example of fine-tuning. By following these steps, you’ll be able to experiment on a smaller scale before scaling up your fine-tuning efforts.
Preliminary Setup: Running Llama 3.2 on Windows
If you’re working on Windows, fine-tuning Llama 3.2 comes with some setup requirements, especially if you want to leverage a GPU for training. Follow these steps to get your environment ready:
Install Windows Subsystem for Linux (WSL): WSL enables you to use a Linux environment on Windows. Search for “WSL” in the Microsoft Store, download an Ubuntu distribution, and open it to access a Linux terminal.
Configure GPU Access: You’ll need an NVIDIA driver to enable GPU access through WSL. To confirm GPU availability, use:
nvidia-smi
If this command shows GPU details, the driver is installed correctly. If not, download the necessary NVIDIA driver from their official site.
Install Necessary Tools:
C Compiler: Run the following commands to install essential build tools.
sudo apt-get update sudo apt-get install build-essential
Python-Dev Environment: Install Python development dependencies for compatibility.
sudo apt-get update && sudo apt-get install python3-dev
Completing these setup steps will prepare you to start working with the Unsloth library on a Windows machine using WSL.
Creating a Dataset for Fine-Tuning
A key component of fine-tuning is having a relevant dataset. For this example, we’ll create a dataset to train Llama 3.2 to answer simple math questions with only the numeric result as the answer. This will serve as a quick, targeted task for the model.
Generate the Dataset: Use Python to create a list of math questions and answers:
import pandas as pd import random def create_math_question(): num1, num2 = random.randint(1, 1000), random.randint(1, 1000) answer = num1 + num2 return f"What is {num1} + {num2}?", str(answer) dataset = [create_math_question() for _ in range(10000)] df = pd.DataFrame(dataset, columns=["prompt", "target"])
Format the Dataset: Convert each question and answer pair into a structured format compatible with Llama 3.2.
formatted_data = [ [{"from": "human", "value": prompt}, {"from": "gpt", "value": target}] for prompt, target in dataset ] df = pd.DataFrame({'conversations': formatted_data}) df.to_pickle("math_dataset.pkl")
Load Dataset for Training: Once formatted, this dataset is ready for fine-tuning.
Setting Up the Training Script for Llama 3.2
With your dataset ready, setting up a training script will allow you to fine-tune Llama 3.2. The training process leverages the Unsloth library, simplifying fine-tuning with LoRA (Low-Rank Adaptation) by selectively updating key model parameters. Let’s begin with package installation and model loading.
Install Required Packages:
pip install "unsloth[colab-new] @ git+https://github.com/unslothai/unsloth.git" pip install --no-deps "xformers<0.0.27" "trl<0.9.0" peft accelerate bitsandbytes
Load the Model: Here, we load a smaller version of Llama 3.2 to optimize memory usage.
from unsloth import FastLanguageModel model, tokenizer = FastLanguageModel.from_pretrained( model_name="unsloth/Llama-3.2-1B-Instruct", max_seq_length=1024, load_in_4bit=True, )
Load Dataset and Prepare for Training: Format the dataset in alignment with the model’s expected structure.
from datasets import Dataset import pandas as pd df = pd.read_pickle("math_dataset.pkl") dataset = Dataset.from_pandas(df)
Begin Training: With all components in place, start fine-tuning the model.
from trl import SFTTrainer from transformers import TrainingArguments trainer = SFTTrainer( model=model, tokenizer=tokenizer, train_dataset=dataset, max_seq_length=1024, args=TrainingArguments( learning_rate=3e-4, per_device_train_batch_size=4, num_train_epochs=1, output_dir="output", ), ) trainer.train()
After training, your model is now fine-tuned for concisely answering math questions.
Testing and Evaluating the Fine-Tuned Model
After fine-tuning, evaluating the model’s performance is essential to ensure it meets expectations.
Generate Test Set: Create a new set of questions for testing.
test_set = [create_math_question() for _ in range(1000)] test_df = pd.DataFrame(test_set, columns=["prompt", "gt"]) test_df.to_pickle("math_test_set.pkl")
Run Inference: Compare responses from the fine-tuned model against the baseline.
test_responses = [] for prompt in test_df["prompt"]: input_data = tokenizer(prompt, return_tensors="pt").to("cuda") response = model.generate(input_data["input_ids"], max_new_tokens=50) test_responses.append(tokenizer.decode(response[0], skip_special_tokens=True)) test_df["fine_tuned_response"] = test_responses
Evaluate Results: Compare responses from the fine-tuned model with the expected answers to gauge accuracy. The fine-tuned model should provide short, accurate answers aligned with the test set, verifying the success of the fine-tuning process.
Fine-Tuning Benefits and Limitations
Fine-tuning offers significant benefits, like improved model performance on specialized tasks. However, in some cases, prompt tuning (providing specific instructions in the prompt itself) may achieve similar results without needing a complex setup. Fine-tuning is ideal for repeated, domain-specific tasks where accuracy is essential and prompt tuning alone is insufficient.
Conclusion
Fine-tuning Llama 3.2 enables the model to perform better in targeted domains, making it highly effective for domain-specific applications. This guide walked through the process of preparing, setting up, training, and testing a fine-tuned model. In our example, the model learned to provide concise answers to math questions, illustrating how fine-tuning modifies model behavior for specific needs.
For tasks that require targeted domain knowledge, fine-tuning unlocks the potential for a powerful, specialized language model tailored to your unique requirements.
FAQs
Is fine-tuning better than prompt tuning for specific tasks?
Fine-tuning can be more effective for domain-specific tasks requiring consistent accuracy, while prompt tuning is often faster but may not yield the same level of precision.What resources are needed for fine-tuning Llama 3.2?
Fine-tuning requires a good GPU, sufficient training data, and compatible software packages, particularly if working on a Windows setup with WSL.Can I run fine-tuning on a CPU?
Fine-tuning on a CPU is theoretically possible but impractically slow. A GPU is highly recommended for efficient training.Does fine-tuning improve model responses in all domains?
Fine-tuning is most effective for well-defined domains where the model can learn specific behaviors. General improvement in varied domains would require a larger dataset and more complex fine-tuning.How does LoRA contribute to efficient fine-tuning?
LoRA reduces the memory required by focusing on modifying only essential parameters, making fine-tuning feasible on smaller hardware setups.
Subscribe to my newsletter
Read articles from Spheron Network directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
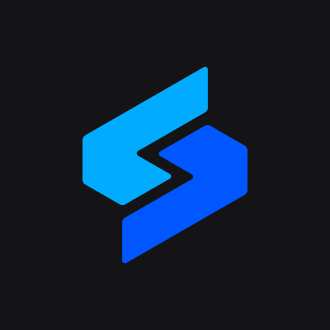
Spheron Network
Spheron Network
On-demand DePIN for GPU Compute