Sorting Algorithms.
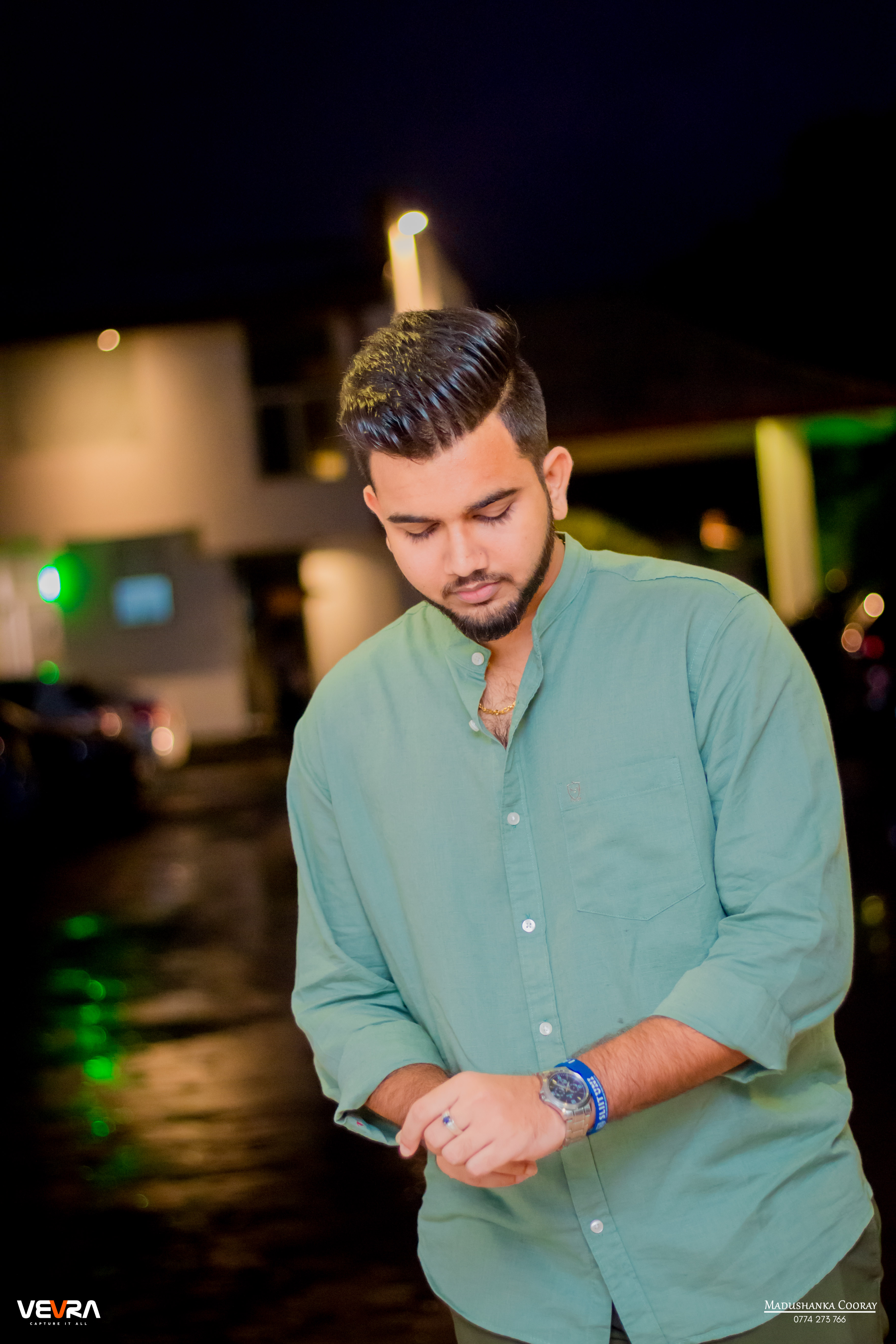

Sorting algorithms are used to arrange data in a specific order, typically ascending or descending. There are four basic sorting algorithms Bubble Sort, Selection Sort, Merge Sort, and Quick Sort
1. Bubble Sort
Bubble Sort is one of the simplest sorting algorithms. The algorithm repeatedly steps through the list, compares adjacent items, and swaps them if they are in the wrong order. The process is repeated until the list is sorted.
How It Works:
Compare adjacent elements.
If the first element is greater than the second, swap them.
Repeat the process until no more swaps are needed.
2. Selection Sort
Selection Sort works by repeatedly selecting the smallest element from the unsorted portion of the list and moving it to the sorted portion.
How It Works:
Find the smallest element in the unsorted part of the list.
Swap it with the first unsorted element.
Move the boundary of the sorted portion forward.
Repeat the process until the list is sorted.
3. Merge Sort
Merge Sort is a more efficient sorting algorithm that uses the divide and conquer approach. It divides the list into smaller sub-lists, sorts them, and then merges them back together in the correct order.
How It Works:
Split the list into two halves.
Recursively sort both halves.
Merge the sorted halves back together.
4. Quick Sort
Quick Sort is another efficient sorting algorithm that uses the divide and conquer strategy. It works by selecting a pivot element, partitioning the list into elements less than the pivot and greater than the pivot, and recursively sorting the two partitions.
How It Works:
Select a pivot element.
Partition the list such that elements less than the pivot are on the left, and elements greater than the pivot are on the right.
Recursively sort the left and right parts.
Bubble Sort and Selection Sort are simple but inefficient for large datasets (O(n²) time complexity).
Merge Sort and Quick Sort are much faster with O(n log n) time complexity in the average case.
Subscribe to my newsletter
Read articles from Akash De Alwis directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
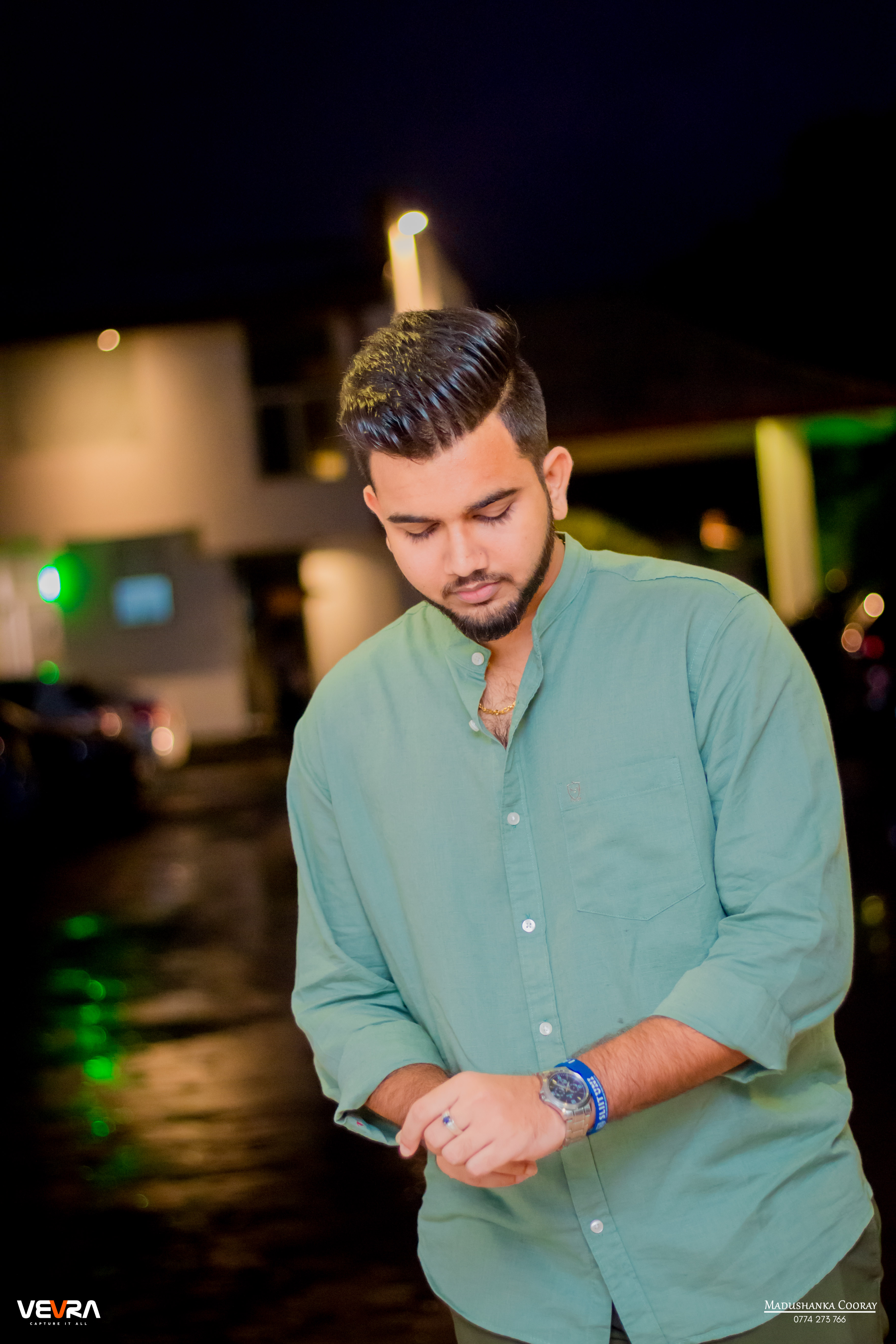
Akash De Alwis
Akash De Alwis
Undergraduate student šš¤