Mastering File I/O in Go: A Beginner's Journey to Efficient File Handling

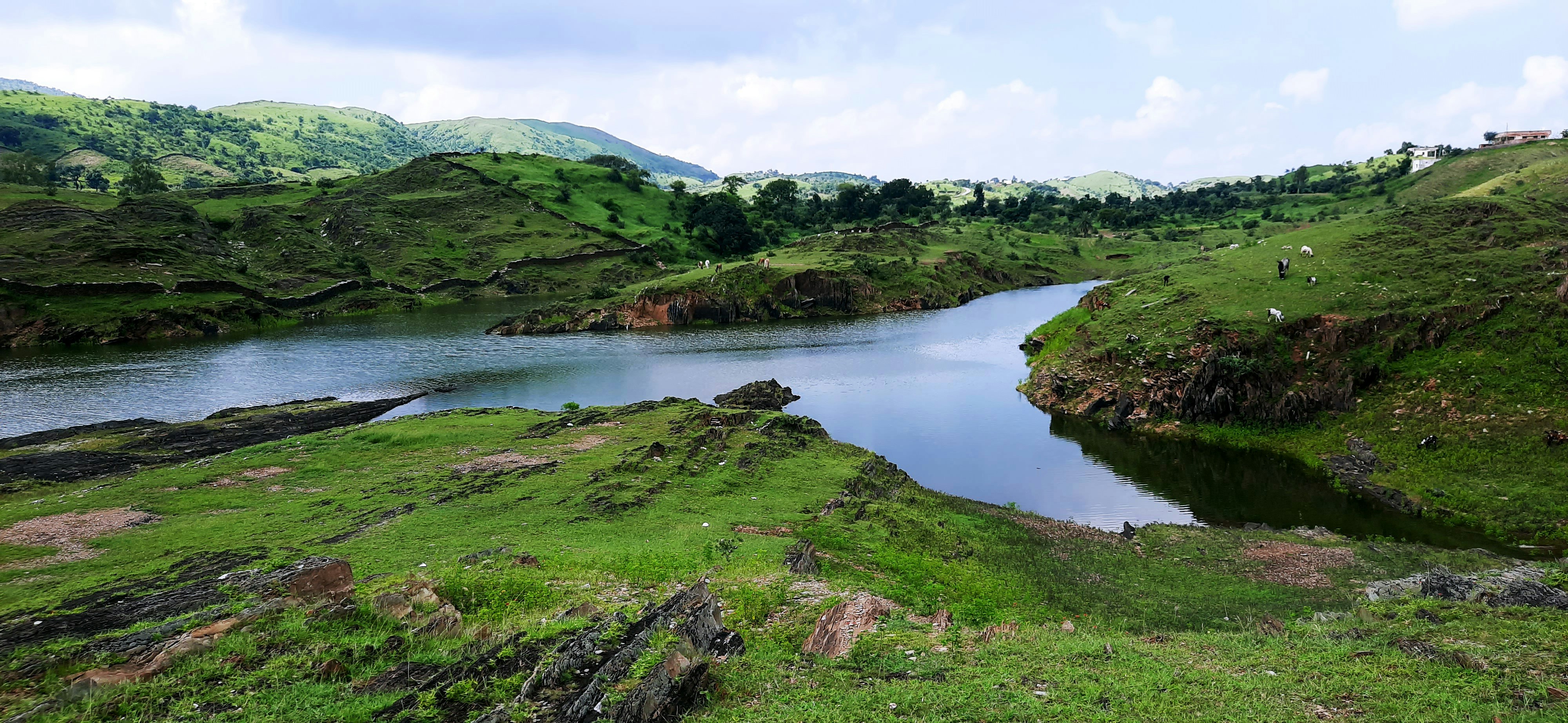
File handling is one of the essential skills for programmers. In Go, you can easily read from and write to files, manipulate them, and even handle structured data like JSON and CSV. This guide will explain everything in a simple, step-by-step manner, so even if you’re new to Go, you’ll understand it easily.
We will cover:
Reading and writing files.
File manipulation with the
os
package.Working with JSON and CSV files.
1. Reading and Writing Files
Writing to a File
Writing to a file means creating a file and saving some content in it. Think of it like writing a note and saving it in a folder.
Example: Writing Text to a File
package main
import (
"fmt"
"os"
)
func main() {
// Step 1: Create or open a file for writing
file, err := os.Create("example.txt") // Create a file named "example.txt"
if err != nil {
// If there’s an error, print it and exit
fmt.Println("Error creating file:", err)
return
}
defer file.Close() // Ensure the file closes after the program is done
// Step 2: Write some text to the file
_, err = file.WriteString("Hello, Go File I/O!\n") // Write text to the file
if err != nil {
// If there’s an error, print it
fmt.Println("Error writing to file:", err)
return
}
// Step 3: Confirm the operation
fmt.Println("File written successfully")
}
Explanation:
os.Create()
: This function creates a file if it doesn’t exist or clears it if it does.defer file.Close()
: Ensures the file is properly closed once the program finishes.file.WriteString()
: Writes the string"Hello, Go File I/O!\n"
to the file.
Output:
File written successfully
When you open the example.txt
file, it will contain:
Hello, Go File I/O!
Reading from a File
Reading a file is like opening a saved document and looking at its content.
Example: Reading Text from a File
package main
import (
"fmt"
"io/ioutil"
)
func main() {
// Step 1: Read the content of the file
data, err := ioutil.ReadFile("example.txt") // Read the file "example.txt"
if err != nil {
// If there’s an error, print it
fmt.Println("Error reading file:", err)
return
}
// Step 2: Print the file content
fmt.Println("File content:")
fmt.Println(string(data)) // Convert bytes to string for printing
}
Explanation:
ioutil.ReadFile()
: Reads the entire file and returns its content as bytes.string(data)
: Converts the byte data into a readable string.
Output:
File content:
Hello, Go File I/O!
2. File Manipulation with the os
Package
The os
package in Go provides tools to manage files, such as renaming and deleting them.
Renaming a File
Renaming a file is like changing its name in your computer's file explorer.
Example: Renaming a File
package main
import (
"fmt"
"os"
)
func main() {
// Rename the file "example.txt" to "renamed_example.txt"
err := os.Rename("example.txt", "renamed_example.txt")
if err != nil {
// Print error if renaming fails
fmt.Println("Error renaming file:", err)
return
}
fmt.Println("File renamed successfully")
}
Explanation:
os.Rename()
: Changes the name of the file.If the operation succeeds, the file’s name will be updated.
Output:
File renamed successfully
Deleting a File
Deleting a file is like throwing it in the trash.
Example: Deleting a File
package main
import (
"fmt"
"os"
)
func main() {
// Delete the file "renamed_example.txt"
err := os.Remove("renamed_example.txt")
if err != nil {
// Print error if deleting fails
fmt.Println("Error deleting file:", err)
return
}
fmt.Println("File deleted successfully")
}
Explanation:
os.Remove()
: Deletes the specified file.If successful, the file will no longer exist.
Output:
File deleted successfully
3. Working with JSON Files
JSON (JavaScript Object Notation) is a format used to store structured data. For example, it can store a person’s name and age together.
Writing JSON to a File
Example
package main
import (
"encoding/json"
"fmt"
"os"
)
type Person struct {
Name string `json:"name"` // Field names in JSON
Age int `json:"age"`
}
func main() {
person := Person{Name: "Alice", Age: 30} // Create a Person struct
// Create a JSON file
file, err := os.Create("person.json")
if err != nil {
fmt.Println("Error creating file:", err)
return
}
defer file.Close()
// Write JSON data to the file
encoder := json.NewEncoder(file)
err = encoder.Encode(person)
if err != nil {
fmt.Println("Error encoding JSON:", err)
return
}
fmt.Println("JSON file written successfully")
}
Explanation:
Struct Definition: The
Person
struct represents the data to be saved.json.NewEncoder()
: Encodes the struct into JSON format and writes it to the file.
Output:
JSON file written successfully
Content of person.json
:
{
"name": "Alice",
"age": 30
}
Reading JSON from a File
Example
package main
import (
"encoding/json"
"fmt"
"os"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
func main() {
// Open the JSON file
file, err := os.Open("person.json")
if err != nil {
fmt.Println("Error opening file:", err)
return
}
defer file.Close()
// Read and decode the JSON data
var person Person
decoder := json.NewDecoder(file)
err = decoder.Decode(&person)
if err != nil {
fmt.Println("Error decoding JSON:", err)
return
}
fmt.Printf("Read JSON: %+v\n", person)
}
Explanation:
Struct Definition: Same as before to match the JSON structure.
json.NewDecoder()
: Reads the JSON file and decodes it into aPerson
struct.
Output:
Read JSON: {Name:Alice Age:30}
Summary
In this article, we learned:
Reading and Writing Files: How to create, write to, and read from text files.
File Manipulation: Renaming and deleting files with the
os
package.Working with JSON: Saving and loading structured data in JSON format.
File handling is an essential skill, and Go makes it simple and efficient. Practice these examples to build a strong foundation in file handling!
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
