Docker Compose Made Easy: Spring Boot Banking App in No Time!

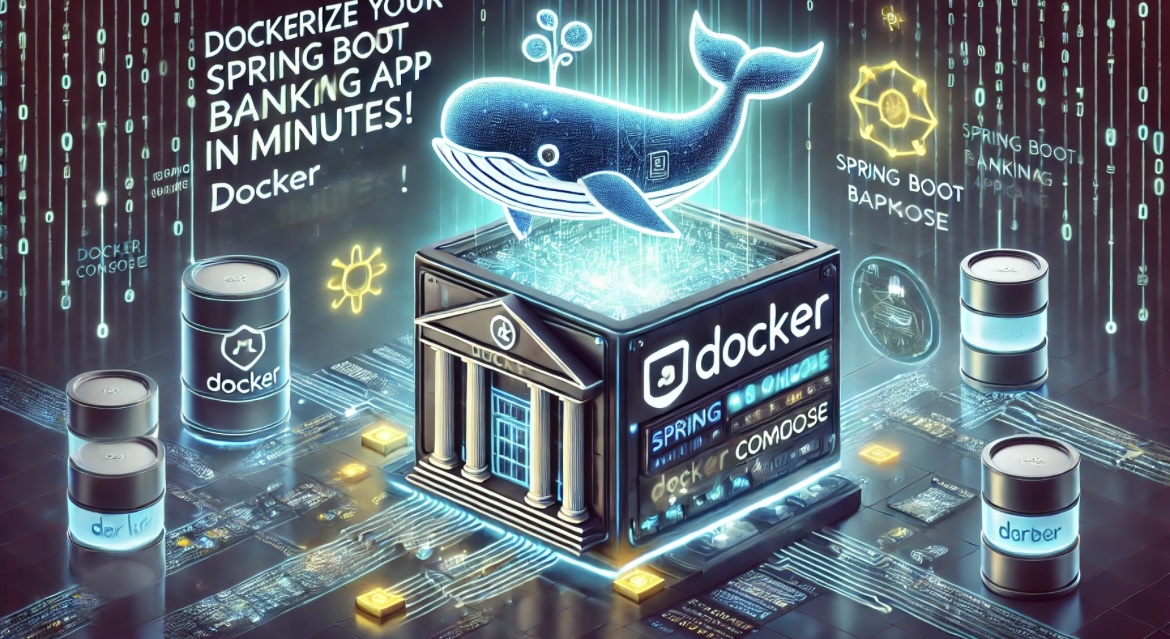
Prerequisite:
It is recommended to read this blog before proceeding with the current one: Banking App and then Docker Image Push Publicly .
I have added this project on my: GitHub Repo you can fork this project.
Steps to Dockerize a Spring Boot Banking App:
- Create a
docker-compose.yml
file
version: "3.8"
services:
mysql:
container_name: mysql
image: mysql:latest
ports:
- 3306:3306
environment:
MYSQL_ROOT_PASSWORD: Test@123
MYSQL_DATABASE: BankDB
networks:
- bankapp
volumes:
- mysql-data:/var/lib/mysql
healthcheck:
test: ["CMD","mysqladmin","ping","-h","localhost","-uroot","-pTest@123"]
interval: 10s
timeout: 5s
retries: 5
start_period: 60s
restart: always
bankapp:
container_name: bankapp
image: chetan0103/springboot-bankapp:latest
ports:
- 8080:8080
environment:
SPRING_DATASOURCE_USERNAME: root
SPRING_DATASOURCE_URL: "jdbc:mysql://mysql:3306/BankDB?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=UTC"
SPRING_DATASOURCE_PASSWORD: Test@123
networks:
- bankapp
depends_on:
- mysql
restart: always
volumes:
mysql-data:
networks:
bankapp:
(This concept involves deleting all the images.)
#To list all the images
docker images -aq
#Forcefully delete all the image id's
docker rmi -f $(docker images -aq)
#List images and you can see no images
docker images
- To install Docker Compose on your virtual machine, follow these steps:
sudo apt-get install docker-compose-v2
- Now, execute Docker Compose to run the application, and the image of the Spring Boot app will be pulled from Docker Hub.
docker compose up -d
#-d is use our docker compose to run in the background
- You can now copy the public IP from your EC2 instance and paste it into your browser, using port 8080. This will allow you to see your application running.
Register a new user, and then you can access all the features.
Even if we stop our container, our data will remain saved because we have added a volume in our configuration.
- Now, we can access MySQL to check if our data is stored in the volume.
docker exec -it "container_id" bash
#Run docker ps to get container id for particular container
- To log in to MySQL, follow these steps:
mysql -u root -p
#It will ask password (Password which you use to create MySQL-> Test@123)
- To perform tasks within the MySQL container, follow these steps:
use BankDB;
show tables;
select * from account;
select * from transaction;
This guide explains how to Dockerize a Spring Boot Banking App using Docker Compose in a few simple steps.
Happy Learning :)
Chetan Mohod
For more DevOps updates, you can follow me on LinkedIn.
Subscribe to my newsletter
Read articles from Chetan Mohanrao Mohod directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chetan Mohanrao Mohod
Chetan Mohanrao Mohod
DevOps Engineer focused on automating workflows, optimizing infrastructure, and building scalable efficient solutions.