Why Test-Driven Development Matters: A Guide for Developers and Businesses
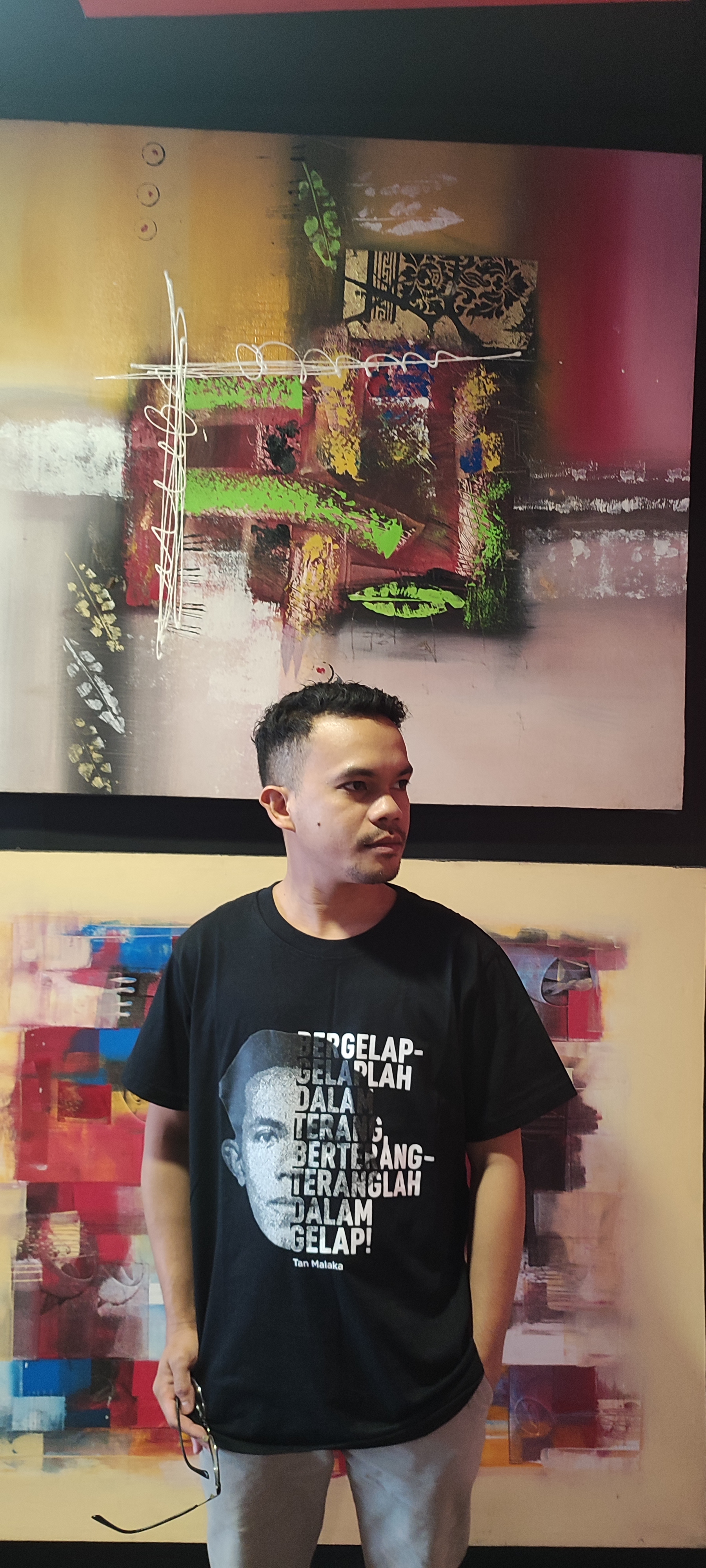

In the fast-paced world of software development, delivering high-quality software efficiently is crucial. One methodology that has gained significant traction in achieving this goal is Test-Driven Development (TDD). But what exactly is TDD, and why should both developers and businesses care about it?
This article aims to demystify TDD, highlight its significance for developers and businesses alike, and provide a simple use case using JavaScript with Jest to illustrate how it works in practice.
What Is Test-Driven Development?
Test-Driven Development is a software development approach where you write tests before writing the actual code. At first glance, this might seem counterintuitive, but this methodology ensures that developers thoroughly understand the desired functionality before implementation.
The TDD cycle typically follows these steps:
Write a Test: Start by writing a test for a small piece of functionality.
Run the Test: The test should fail since the functionality isn't implemented yet.
Write Code: Write the minimal amount of code needed to make the test pass.
Run Tests Again: Ensure that the test (and any other tests) pass.
Refactor: Clean up the code, improving its structure without changing its behavior.
Repeat: Move on to the next piece of functionality.
This cycle is often referred to as Red-Green-Refactor:
Red: Write a test that fails.
Green: Write code to make the test pass.
Refactor: Improve the code while ensuring tests still pass.
Why Is TDD Significant for Developers?
1. Improves Code Quality
By writing tests first, developers are encouraged to think critically about the requirements and possible edge cases, leading to:
Better Design: Encourages modular, loosely coupled code.
Early Bug Detection: Identifies issues before they become more complex.
2. Facilitates Refactoring
With a suite of tests, developers can confidently refactor code, knowing that any unintended changes will be caught immediately.
3. Enhances Productivity
Though it may seem slower initially, TDD can speed up development in the long run by reducing debugging time and preventing regressions.
4. Provides Documentation
Tests serve as live documentation, illustrating how the code is supposed to work and how it should be used.
Why Is TDD Important for Businesses?
1. Reduces Costs
Lower Maintenance Costs: Cleaner code is easier and cheaper to maintain.
Fewer Bugs in Production: Early detection of issues reduces the cost associated with fixing bugs after release.
2. Accelerates Time-to-Market
Efficient Development: Streamlined processes get products to market faster.
Competitive Advantage: Being first can capture market share.
3. Improves Product Quality
Customer Satisfaction: Higher quality leads to happier customers and better reviews.
Brand Reputation: Reliable products enhance brand image and trust.
4. Enhances Collaboration
Clear Communication: Tests clarify requirements for all stakeholders.
Alignment: Developers, testers, and business analysts work towards common goals.
A Simple Use Case: Building a Calculator Function with JavaScript and Jest
Let's walk through a basic example of TDD in action using JavaScript and Jest: creating a simple calculator function.
Step 1: Write a Test
First, we'll write a test that defines the expected behavior of an add
function.
// calculator.test.js
const { add } = require('./calculator');
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
Step 2: Run the Test
Since the add
function doesn't exist yet, the test will fail.
$ jest
FAIL ./calculator.test.js
● Test suite failed to run
Cannot find module './calculator' from 'calculator.test.js'
Step 3: Write Minimal Code
Create the add
function to make the test pass.
// calculator.js
function add(a, b) {
return a + b;
}
module.exports = { add };
Step 4: Run Tests Again
Run the test to see if it passes.
$ jest
PASS ./calculator.test.js
✓ adds 1 + 2 to equal 3 (5ms)
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Step 5: Refactor
The code is already simple, but if we had more complexity, we could refactor now. For demonstration, let's add another test for subtraction.
// calculator.test.js
const { add, subtract } = require('./calculator');
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
test('subtracts 5 - 2 to equal 3', () => {
expect(subtract(5, 2)).toBe(3);
});
Run the tests again.
$ jest
FAIL ./calculator.test.js
● Test suite failed to run
TypeError: subtract is not a function
Step 6: Repeat the Cycle
Implement the subtract
function.
// calculator.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
module.exports = { add, subtract };
Run the tests.
$ jest
PASS ./calculator.test.js
✓ adds 1 + 2 to equal 3 (2ms)
✓ subtracts 5 - 2 to equal 3
Test Suites: 1 passed, 1 total
Tests: 2 passed, 2 total
Step 7: Refactor
If needed, refactor the code to improve its structure or efficiency, ensuring all tests still pass.
Conclusion
Test-Driven Development is a powerful methodology that benefits both developers and businesses. For developers, it leads to better-designed, maintainable code. For businesses, it results in higher-quality software, reduced costs, and faster delivery times.
By adopting TDD, you invest in building robust software foundations, leading to greater confidence in your code and increased satisfaction among your users.
Ready to Embrace TDD?
Whether you're a developer looking to enhance your coding practices or a business aiming to improve software quality, starting with TDD can be transformative. Begin with small projects or features and experience the positive impact on your development process.
Subscribe to my newsletter
Read articles from Eklemis Santo Ndun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
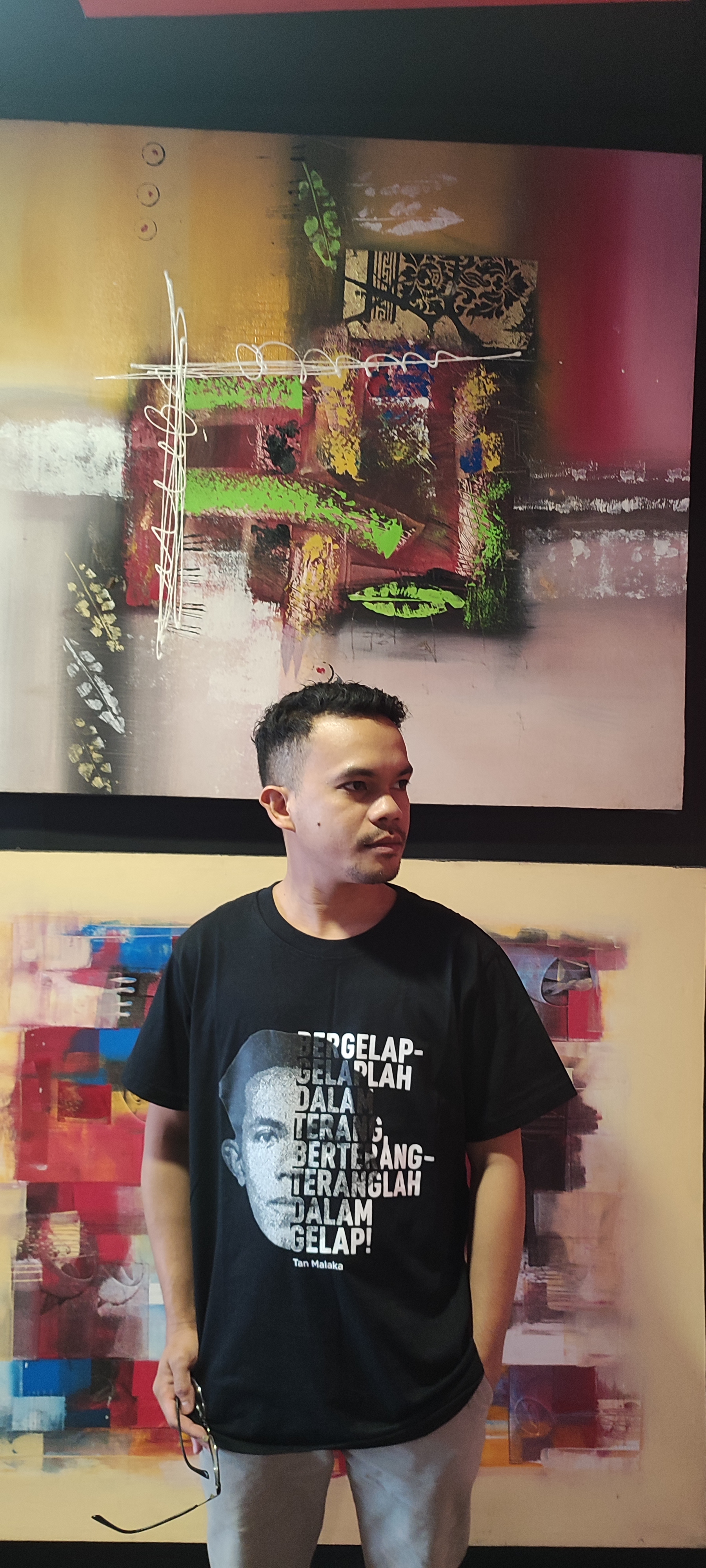
Eklemis Santo Ndun
Eklemis Santo Ndun
Hi there, I'm a developer from Indonesia. I love learning new things especially technology. Now, not only do i love learning new things, but also to write about what i've learnt. In regular work day, i deal a lot with Images files, SQL Server, MS Access and Excel as well. To make my daily target reached with high quality and shorter time, i automate many of my task with help of Python. In some months where my regular task is not much, i spent time develop web apps to automate my colleagues regular task as well. Out of regular work time, i spend time learning and creating project with Javascript (and Html and CSS), learn UI/UX design with Figma, code Python scripts(Machine Learning), and Rust programming language. To help better remembering all things i've learnt, now i learn to write about them as well.