this in JavaScript: A Comedy of Contexts
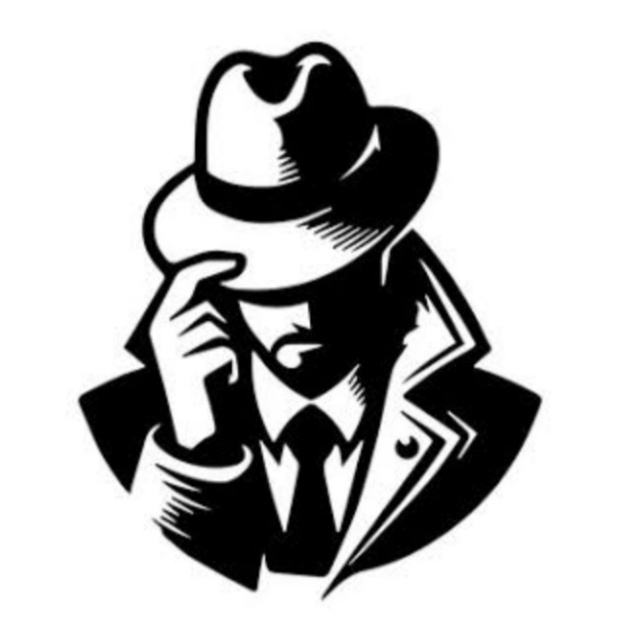

Ah, JavaScript. The land of quirky rules, unexpected behaviors, and, of course, the enigmatic this
. Let’s dive into the various personalities of this
and see why it’s both your best friend and the source of your debugging nightmares.
What Exactly is this
?
In JavaScript, this
is like a method actor — its identity changes depending on the scene it’s in. It usually refers to an object, but which object? That depends on the circumstances. Let’s break it down:
In an Object Method:
this
is the object that’s doing the talking.Alone in the Code:
this
is the global object (orundefined
in strict mode).In a Regular Function:
Non-strict mode:
this
is the global objectStrict mode:
this
isundefined
In Event Handlers:
this
is the element that got clicked, poked, or prodded.When Called with
call()
,apply()
, orbind()
:this
gets a custom-tailored suit and does whatever you explicitly tell it to.
1. this
in Object Methods
Imagine this
as the VIP of an object’s methods — it’s always pointing back to the object.
Example:
const car = {
brand: "Toyota",
getBrand: function () {
return this.brand; // It's me, the car!
},
};
console.log(car.getBrand()); // Outputs: Toyota
Here, this
is the car object itself.
2. this
in the Global Context
Out in the open, this
likes to go big or go home.
Non-strict mode:
console.log(this); // Outputs: window
Strict mode:
"use strict"; console.log(this); // Outputs: undefined (strict mode doesn’t play games)
Here, this
is either your browser’s window
object or… a blank stare from the void.
3. this
in Regular Functions
When it comes to regular functions, this
behaves like a well-meaning but clueless intern — it just defaults to the global object. Unless you’re in strict mode, in which case this
has no idea what’s going on.
Example:
function showThis() {
console.log(this);
}
showThis(); // Outputs: global object or undefined (in strict mode)
“Who, me? No idea what I’m doing here!”
4. this
in Arrow Functions
Arrow functions? They’re the laid-back millennials of the JavaScript world. They don’t bother with their own this
— they just borrow it from their surroundings.
Example:
const obj = {
name: "Alice",
arrowFunc: () => {
console.log(this.name); // Not me, buddy.
},
};
obj.arrowFunc(); // Outputs: undefined (inherits global or undefined in strict mode)
Arrow functions say, “I’m not getting involved. Whatever this
you have, I’m good with it.”
5. this
in Event Handlers
Event handlers are like chameleons — this
changes to match the element receiving the event.
Example:
document.getElementById("btn").addEventListener("click", function () {
console.log(this); // Outputs: the button element
});
But throw an arrow function into the mix, and it’s like hiring a babysitter who refuses to leave their couch:
document.getElementById("btn").addEventListener("click", () => {
console.log(this); // Outputs: global object or undefined
});
Arrow functions in event handlers? They’re like, “Nah, I don’t do buttons.”
6. Taking Control of this
with call()
, apply()
, and bind()
When JavaScript gets too free-spirited with this
, you can reign it in using call()
, apply()
, or bind()
.
call()
You politely ask this
to be someone else — and it agrees.
function greet(greeting) {
console.log(`${greeting}, I am ${this.name}`);
}
const person = { name: "John" };
greet.call(person, "Hello"); // Outputs: Hello, I am John
apply()
Same deal as call()
, but the arguments arrive in bulk, like Costco.
greet.apply(person, ["Hi"]); // Outputs: Hi, I am John
bind()
You tie this
down for later use. It’s commitment-phobic, but you’ve got a workaround.
const boundGreet = greet.bind(person, "Hey");
boundGreet(); // Outputs: Hey, I am John
“Bound and ready to go!”
Key Takeaways
this
is the ultimate shapeshifter. Its value depends entirely on where and how it’s invoked.If
this
starts acting out, you can discipline it withcall()
,apply()
, orbind()
.Arrow functions are the Zen monks of JavaScript — they simply borrow
this
from their surroundings and refuse to argue.
Mastering this
takes patience, practice, and maybe a few dozen consolatory cups of coffee, but once you do, you’ll feel like a JavaScript ninja. Or at least like someone who knows where they are in the grand cosmic joke that is this
.
Subscribe to my newsletter
Read articles from Maneeshwar Karne directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
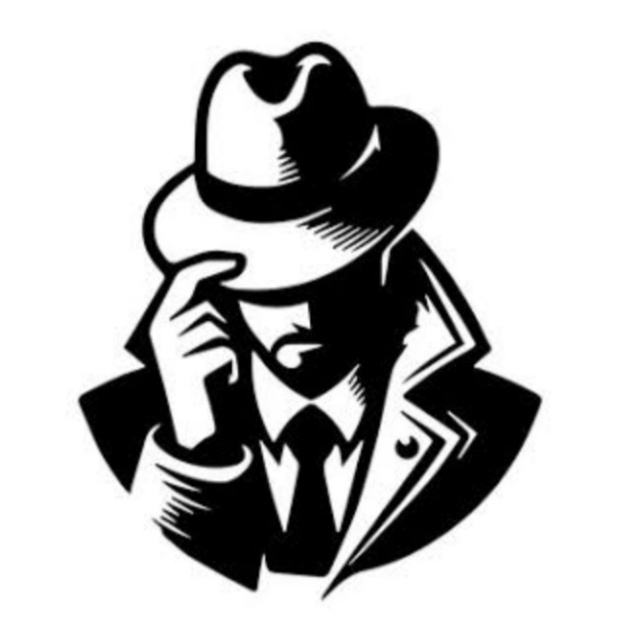
Maneeshwar Karne
Maneeshwar Karne
Learning web devlelopment