Understanding Acceptance Scenarios: Bridging Requirements and Testing with Cucumber
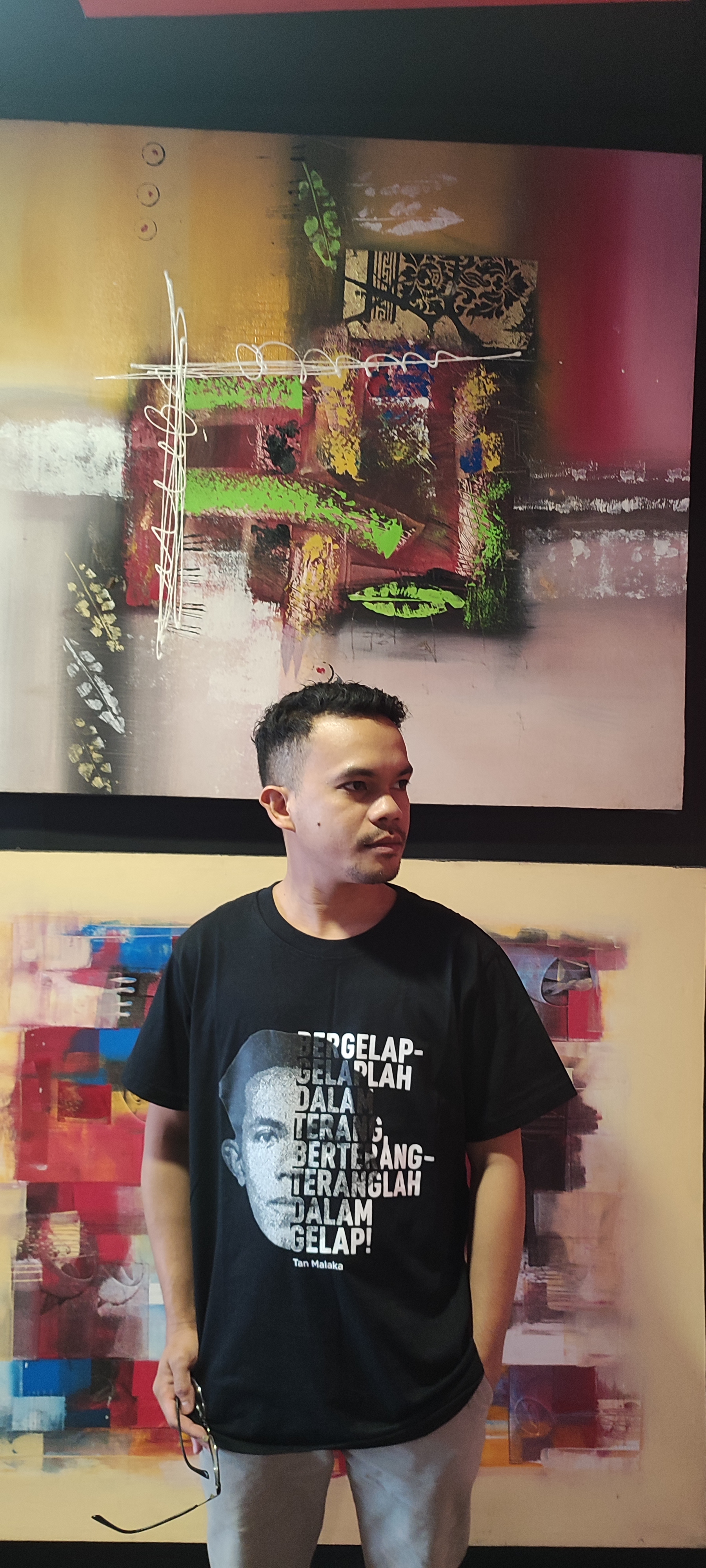

In software development, delivering a product that meets client expectations hinges on clear communication, precise requirements, and effective testing. The Software Development Life Cycle (SDLC) provides a structured approach through phases like planning, requirement analysis, design, implementation, testing, deployment, and maintenance. Meanwhile, Agile methodology introduces flexibility and iterative progress, allowing teams to adapt quickly to changes and continuously deliver value.
At the core of both SDLC and Agile is the Requirement Analysis phase, where developers, QA engineers, and clients collaborate to define what the software should achieve. This collaboration is crucial for eliminating knowledge gaps and ensuring everyone shares a common understanding. Central to this understanding is the concept of the Acceptance Scenario, which we'll explore in depth.
Acceptance Scenarios: The Bridge Between Requirements and Testing
An Acceptance Scenario is a detailed description of how a system should behave in a specific situation from the user's perspective. It serves as a concrete example to illustrate requirements, ensuring that developers, testers, and clients are aligned on expected outcomes. Acceptance Scenarios:
Describe Behavior: Outline the functionality based on user interactions.
Provide Clarity: Help eliminate ambiguities in requirements.
Guide Testing: Serve as the basis for creating test cases and scenarios.
Importance of Acceptance Scenarios
Align Expectations: Ensure all stakeholders have a shared vision of the feature.
Facilitate Communication: Bridge the gap between technical and non-technical team members.
Enhance Quality: Lead to more thorough and focused testing, reducing defects.
Implementing Acceptance Scenarios with Given-When-Then and Feature Files
To formalize Acceptance Scenarios, we use the Given-When-Then format within Feature Files, utilizing the Gherkin language. This approach is central to Behavior-Driven Development (BDD).
Understanding Given-When-Then and Feature Files
Given-When-Then Format
The Given-When-Then format is a simple and structured way to write down test scenarios:
Given: Describes the initial context or preconditions before an action is taken.
When: Specifies the action or event that triggers the scenario.
Then: Details the expected outcome or result after the action.
This format helps in breaking down requirements into clear, testable statements that are easy to understand by all stakeholders, including non-technical team members.
Feature Files and Gherkin Language
Feature Files are text files with a .feature
extension that contain one or more scenarios written in the Given-When-Then format using the Gherkin language. Gherkin is a business-readable, domain-specific language that lets you describe software's behavior without detailing how that functionality is implemented.
Key characteristics of Gherkin and Feature Files:
Human-Readable: Written in plain English (or other natural languages), making them accessible to non-technical stakeholders.
Structured: Follows a specific syntax and structure, which allows tools like Cucumber to parse and execute them.
Executable Specifications: Serve both as documentation and as tests that can be run to validate the software's behavior.
Easy-to-Grasp Example of Given-When-Then
Let's consider a simple scenario where a user wants to log into an application.
Example:
Feature: User Login
Scenario: Successful login with valid credentials
Given the user is on the login page
When the user enters a valid username and password
And clicks the login button
Then the user should be redirected to the dashboard
And a welcome message should be displayed
Explanation:
Feature: Describes the functionality under test—in this case, "User Login."
Scenario: Represents a specific situation or use case.
Given: Sets up the initial state—the user is on the login page.
When: Specifies the action—the user enters valid credentials and clicks login.
Then: Describes the expected outcome—the user is redirected to the dashboard, and a welcome message is displayed.
By writing scenarios in this way, everyone involved in the project can understand what is expected, and developers can implement the functionality to meet these specifications.
Practical Implementation Using Cucumber
Cucumber is a testing tool that supports BDD by running automated tests written in plain language. Here's how you can implement Acceptance Scenarios using Cucumber.
Step 1: Define the Acceptance Scenario
Scenario: User logs in successfully with valid credentials.
Step 2: Write the Feature File
Create a file named login.feature
:
Feature: User Login
Scenario: Successful login with valid credentials
Given the user is on the login page
When the user enters a valid username and password
And clicks the login button
Then the user should be redirected to the dashboard
And a welcome message should be displayed
Step 3: Set Up the Project Environment
We'll use JavaScript with Node.js and Cucumber.js.
Install Dependencies
npm init -y
npm install @cucumber/cucumber --save-dev
Step 4: Write the Step Definitions
Create a folder features/step_definitions
and add a file login.steps.js
:
// features/step_definitions/login.steps.js
const { Given, When, Then } = require('@cucumber/cucumber');
const assert = require('assert');
let currentPage;
let user;
Given('the user is on the login page', function () {
currentPage = 'login';
});
When('the user enters a valid username and password', function () {
user = { username: 'testuser', password: 'password123' };
this.enteredUsername = user.username;
this.enteredPassword = user.password;
});
When('clicks the login button', function () {
if (
this.enteredUsername === 'testuser' &&
this.enteredPassword === 'password123'
) {
currentPage = 'dashboard';
this.welcomeMessage = `Welcome, ${this.enteredUsername}!`;
} else {
currentPage = 'login';
this.errorMessage = 'Invalid credentials';
}
});
Then('the user should be redirected to the dashboard', function () {
assert.strictEqual(currentPage, 'dashboard');
});
Then('a welcome message should be displayed', function () {
assert.strictEqual(this.welcomeMessage, `Welcome, ${this.enteredUsername}!`);
});
Step 5: Configure Cucumber
Create a cucumber.js
configuration file:
// cucumber.js
module.exports = {
default: `--publish-quiet --require features/step_definitions/*.js`,
};
Step 6: Run the Tests
Execute the tests using the Cucumber CLI:
npx cucumber-js
Step 7: Review the Test Results
You should see an output indicating that all steps have passed:
1 scenario (1 passed)
5 steps (5 passed)
Conclusion
Acceptance Scenarios are a vital tool for ensuring that software features meet the needs and expectations of all stakeholders. By utilizing the Given-When-Then format and Feature Files with the Gherkin language, teams can create clear, testable requirements that bridge the gap between clients, developers, and testers.
Implementing these scenarios with tools like Cucumber allows for automated testing, increasing efficiency and software quality. This practice not only improves the development process but also fosters collaboration and transparency.
Next Steps
Start Writing Acceptance Scenarios: Collaborate with your team to define scenarios for upcoming features.
Adopt the Given-When-Then Format: Use this structure to clarify requirements.
Leverage Feature Files: Document scenarios in a way that is both readable and executable.
Integrate Cucumber for Testing: Automate your acceptance tests to ensure continuous validation.
By focusing on Acceptance Scenarios, you enhance communication, reduce misunderstandings, and deliver software that truly meets user needs.
References
Cucumber Documentation: https://cucumber.io/docs
Gherkin Language Guide: https://cucumber.io/docs/gherkin/
Behavior-Driven Development Overview: https://dannorth.net/introducing-bdd
Agile Manifesto: http://agilemanifesto.org
Note: The code examples are simplified for demonstration purposes. In a real-world application, you would interact with actual web pages using tools like Selenium WebDriver or Cypress.
Subscribe to my newsletter
Read articles from Eklemis Santo Ndun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
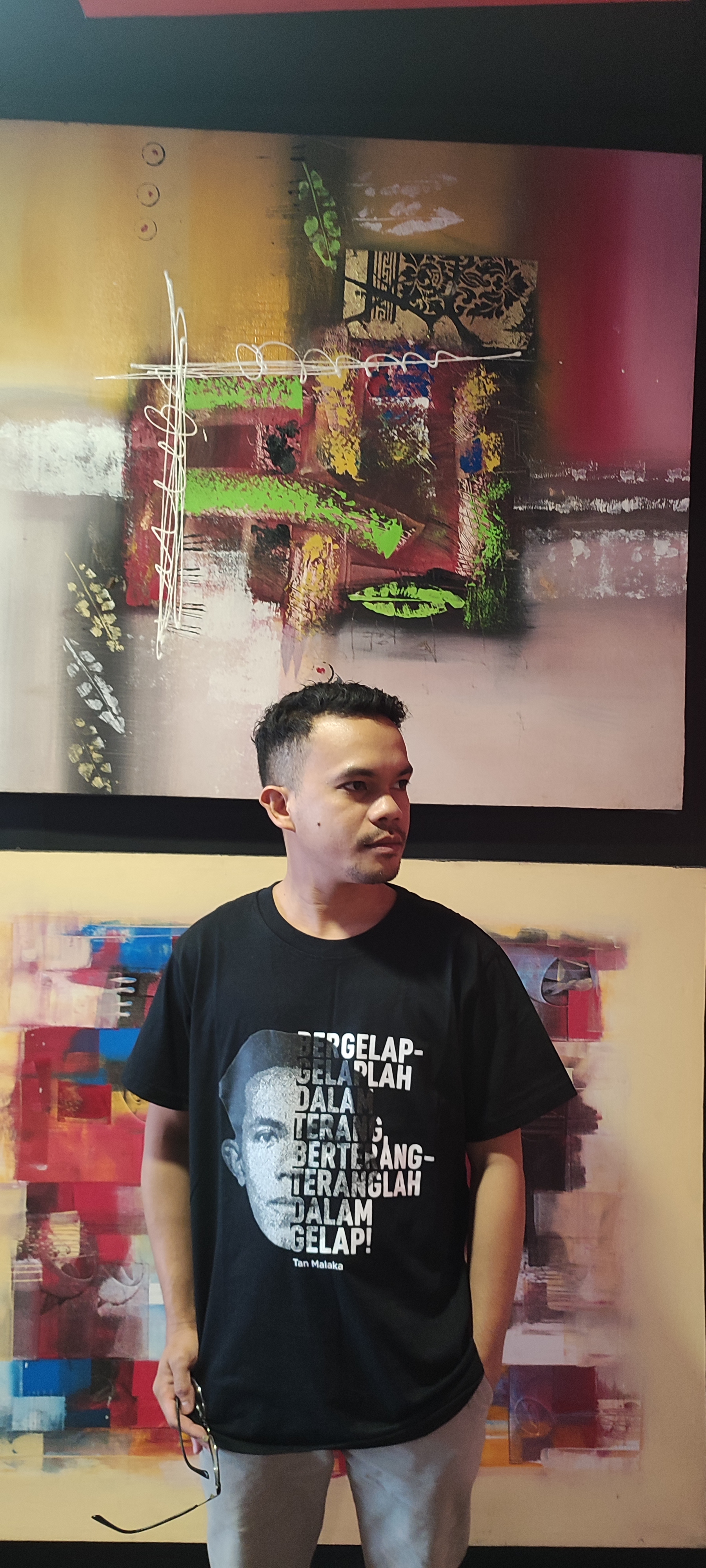
Eklemis Santo Ndun
Eklemis Santo Ndun
Hi there, I'm a developer from Indonesia. I love learning new things especially technology. Now, not only do i love learning new things, but also to write about what i've learnt. In regular work day, i deal a lot with Images files, SQL Server, MS Access and Excel as well. To make my daily target reached with high quality and shorter time, i automate many of my task with help of Python. In some months where my regular task is not much, i spent time develop web apps to automate my colleagues regular task as well. Out of regular work time, i spend time learning and creating project with Javascript (and Html and CSS), learn UI/UX design with Figma, code Python scripts(Machine Learning), and Rust programming language. To help better remembering all things i've learnt, now i learn to write about them as well.