An Introduction to beginner friendly Flask for beginner developers

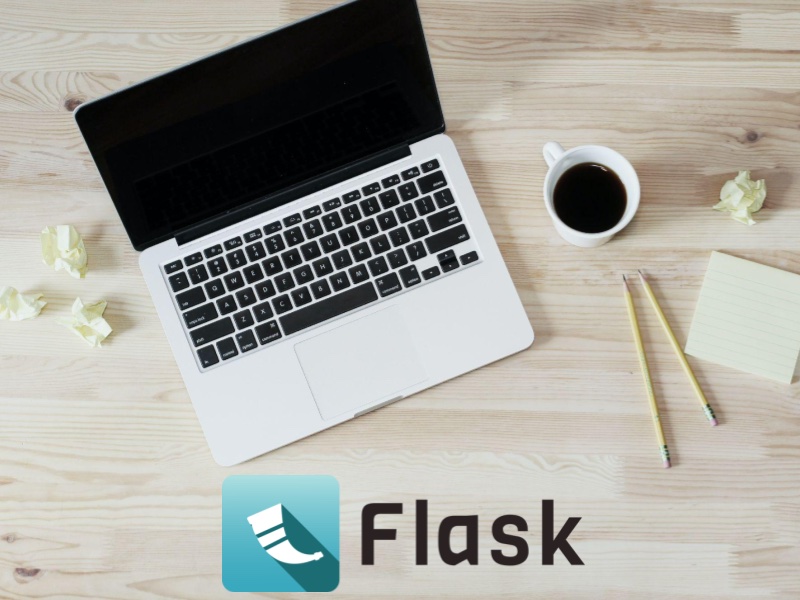
Flask is a very lightweight and flexible python web framework
, designed to help developers to build web applications quickly and efficiently.
Framework?
Some of the major advantages of Flask
Simplicity
Flask is straightforward and has very intuitive syntax, A new beginner developer can use it with ease even with minimal experience of python.
Modularity
Flask’s modular design means we can integrate only the components we need, avoiding excessive codebases. Developers can start with the very basics and add extentions (like database or authentication) as the project grows.
Extensibility
Flask has a diverse extensions such as Flask-SQLAlchemy (for databases), Flask-WTF (for forms), Flask-RESTful (for APIs).
Defination and History
Flask is considered as a “micro-framework”
.Unlike larger frameworks like Django, Flask does not come with built-in tools like an ORM(Object-Relational Mapper) or authentication system. Instead, it gives developers the freedom to chose and integrate what they really need.
micro-framework?
Flask was first released on 2010, It has very simple philosophy i.e, “Simple is better than complex”.
Flask is built on top of two libraries
Jinja2: A templating engine for dynamic HTML generation
Werkzeug: A library for handling HTTP requests.
Setting Up Your Flask Environment
Setting up flask environment is an easy-peasy task. Tools needed: Python, pip, virtual environment.
- Installation of Flask
pip install flask
- file structure for your project
my_flask_app/
├── app.py
├── static/
├── templates/
└── venv/
Let’s try to build our first Flask App
- A simplest example, initiate the app
from flask import Flask
app = Flask(__name__)
@app.route("/")
def home():
return "Namaste! everyone"
if __name__ == "__main__":
app.run(debug=True)
lets try to understand each component
from flask import Flask
this imports theFlask
class from the Flask library, which is the foundation for building a Flask application.app = Flask(__name__)
Flask
is a class used to create a Flaskapplication instance
.The
__name__
variable is a special Python variable that represents the name fo the current module(the file being executed).Flask uses this name to determine the location of the application (useful for finding resources like templates and static files).
application instance
In Flask, the application instance is the central object that represents your web application. It's created by instantiating theFlask
class, and it serves as the entry point for defining your app's behavior, such as handling requests, routing, and configuring settings.@app.route(“/”)
this is a decorator that maps the URL path / (the root of your website, like http://localhost:5000/) to the home function.def home():
this defines a Python function calledhome
.return “Namaste! everyone”:
this specifies the response that will be sent back to the user’s browser when they visit the/
route. In this case, the browser will display Namaste! everyoneif name == “__main__”:
this ensures that the code inside this block only runs when the script is executed directly(e.g.python app.py
) and not when it’s imported as a module in another script.app.run(debug=True)
this starts the Flask development server to handle incoming requests.debug=True
enables debug mode, which automatically restarts the server when code changes and provides detailed error messages for easier debugging.
- Adding Templates and Static files
<html>
<head>
<title>Flask App</title>
</head>
<body>
<h1>Welcome to Flask!</h1>
</body>
</html>
Templates
Jinja2
Key features in Jinja2:
{{ variable }}: inserts dynamic content (e.g., {{ username }}.
{% for …%}: loops through data
{% if…%}: Adds conditonal logic
- Handling Forms and routes
from flask import request
@app.route("/submit, methods=["POST"])
def submit():
name = request.form.get("name")
return f"Hello, {name}!"
methods=[“POST”]:
By default, Flask routes handleGET
requests. The methods parameter specifies which HTTP methods are allowed for the route. Here,POST
is specifies, meaning this route will only respond toPOST
requestdef submit():
This is the Python function that will execute when the/submit
route is accessed via aPOST
request.name = request.form.get(“name”)
request
Object: the request object in Flask provides access to the data sent by the client
request.form
: the form attribute contains the form data sent in the body of a POST request. It’s a dictionary-like object.
.get(“name”):
this retrieves the value associated with the key“name”
from the form data. If no value exists for “name”, it returnsNone
by default.
Connecting to a Database(Optional):
You can connect Database like SQLite with Flask-SQLAlchemy for storing data.Now you can run your application.
if you have initiated your app in the app.py file, then you can run your application by runnig the scriptpython app.py
in the terminal.
Now that you have got the basics of Flask, it’s time to create your own project! Start small- perhaps a personal homepage or a basic app that displays quotes.
Don’t be afraid to make mistakes, every error is a step closer to mastery. Happy coding!
Resources for further Learning:
Official Flask documentation
Subscribe to my newsletter
Read articles from NIRAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
