Python for DevOps: Understanding Data Types and String Handling
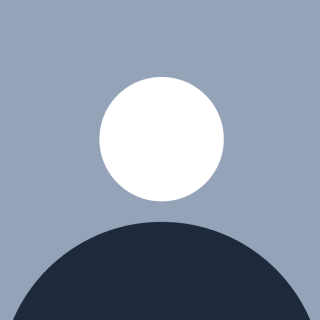
Introduction
In this article, we'll explore key Python concepts for DevOps engineers, focusing on data types, string handling, and practical examples that demonstrate Python's utility in real-world scenarios.
Key Takeaways
What are Data Types?
Data types help compilers/interpreters understand the nature of data
Python is a dynamically typed language
Main data type categories include:
Strings
Numeric types (Integers, Floats)
Sequence types (Lists, Tuples)
Mapping types (Dictionaries)
Boolean
Sets
String Handling in Python
Declaring Strings
Use single or double quotes
Example:
name = "Abishek"
Common String Functions
Split Function
Separates strings based on a delimiter
Useful for extracting specific parts of strings
arn = "aws:iam::123456789:user/username"
username = arn.split("/")[1]
Uppercase Conversion
name = "abishek" uppercase_name = name.upper() # Returns "ABISHEK"
Concatenation
string1 = "Hello" string2 = "World" full_string = string1 + " " + string2 # "Hello World"
Length Function
text = "This is a string" length = len(text) # Returns 16
Numeric Types
Integers: Whole numbers (e.g., 10, -5)
Floats: Decimal numbers (e.g., 3.14, 2.5)
Numeric Functions
round()
: Rounds floating-point numbersabs()
: Returns absolute value
Regular Expressions
Used for pattern matching in strings
Helpful in log file analysis
Example use cases:
Finding specific error patterns in log files
Validating string patterns
DevOps Practical Example
In AWS, you might use string manipulation to extract usernames from ARNs (Amazon Resource Names):
arn = "arn:aws:iam::123456789:user/devops_engineer"
username = arn.split("/")[1] # Extracts "devops_engineer"
Learning Tips
Practice using built-in functions
Experiment with different string manipulations
Always look for built-in functions before writing custom code
Conclusion
Understanding data types and string handling is crucial for DevOps engineers. Python provides powerful, intuitive tools to manipulate and process data efficiently.
Resources
Python Official Documentation
DevOps with Python courses
Practice coding platforms
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by