How to Configure Tenancy for Laravel Using the Modules Package

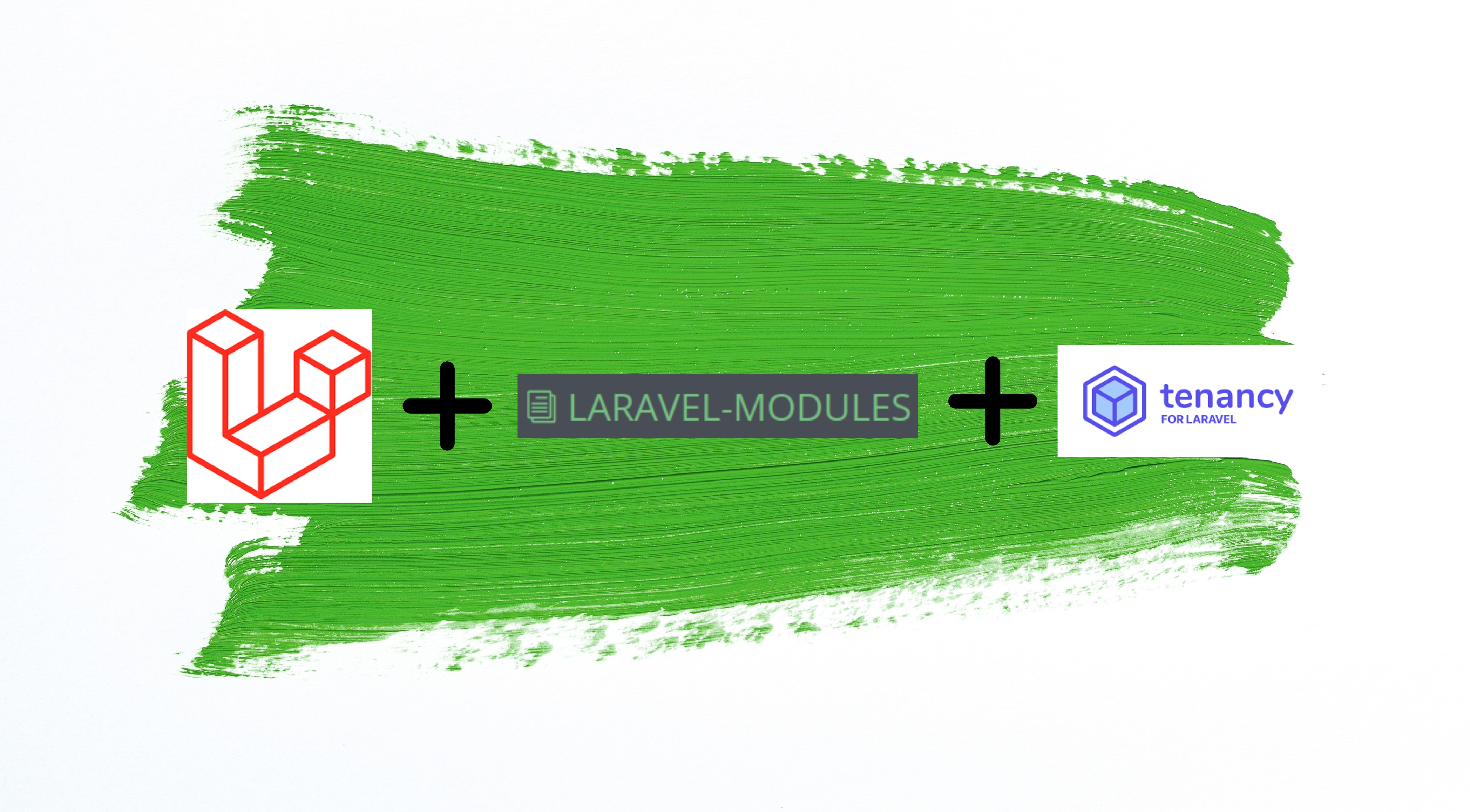
First, install Laravel fresh project or pick your existing project where already has nwidart/laravel-modules.
If you haven’t install any Laravel project then let’s start and explore it -
composer create-project laravel/laravel:^10.0 example-app
Now integrate Laravel Modules Package -
composer require nwidart/laravel-modules
Also, integrate Tenancy for Laravel Package -
composer require stancl/tenancy
php artisan tenancy:install
// bootstrap/providers.php
return [
App\Providers\AppServiceProvider::class,
App\Providers\TenancyServiceProvider::class, // <-- here
];
First Configure all requirements for tenancy from Tenancy Docs. After preparing tenancy in the project create a fresh module like Blog -
php artisan module:make Blog
After creating a fresh Blog module there are all the essential files for Laravel app. Now we want to modify this for our tenant setup.
Well, first, we’re going to configure migrations for the tenant in the Modules/Blog/Database/migrations path. In this path, all migrations are for the central system. Now, create a tenant folder in that folder, like (Modules/Blog/Database/migrations/tenant), and configure it on the service provider. For this feature, we want a service provider, so create a service provider in the Blog Module.
php artisan module:make-provider TenantBlogServiceProvider Blog
<?php
namespace Modules\Blog\App\Providers;
use Illuminate\Support\ServiceProvider;
class TenantBlogServiceProvider extends ServiceProvider
{
/**
* Register the service provider.
*/
public function register(): void
{
// Register module tenant migrations
$this->app->booted(function () {
config()->push(
'tenancy.migration_parameters.--path',
module_path('Blog', 'Database/migrations/tenant')
);
});
}
/**
* Get the services provided by the provider.
*/
public function provides(): array
{
return [];
}
}
Register the provider in the BlogServiceProvider.php file
/**
* Register the service provider.
*/
public function register(): void
{
$this->app->register(RouteServiceProvider::class);
$this->app->register(TenantBlogServiceProvider::class); // Register tenant service provider for this module
}
Now we’re going to config routes for tenants -
We know all routes are web.php for web guard and api.php for API guard. But for central system routes boundary we should configure RouteServiceProvider in Blog Module -
/**
* Define the "web" routes for the application.
*
* These routes all receive session state, CSRF protection, etc.
*/
protected function mapWebRoutes(): void
{
foreach ($this->centralDomains() as $domain) {
Route::middleware('web')
->namespace($this->moduleNamespace)
->domain($domain)
->group(module_path('Blog', '/routes/web.php'));
}
}
/**
* Define the "api" routes for the application.
*
* These routes are typically stateless.
*/
protected function mapApiRoutes(): void
{
foreach ($this->centralDomains() as $domain) {
Route::prefix('api')
->middleware('api')
->namespace($this->moduleNamespace)
->domain($domain)
->group(module_path('Blog', '/routes/api.php'));
}
}
/**
* Define the "tenant" routes for the application.
*
* These routes are typically stateless.
*/
protected function mapTenantRoutes(): void
{
$this->app->booted(function () {
if (file_exists(module_path('Blog', '/routes/tenant.php'))) {
Route::namespace($this->moduleNamespace)
->group(module_path('Blog', '/routes/tenant.php'));
}
});
}
/**
* Define the routes for the application.
*/
protected function centralDomains(): array
{
return config('tenancy.central_domains');
}
Finally RouteServiceProvider.php for Blog Module
<?php
namespace Modules\Blog\App\Providers;
use Illuminate\Support\Facades\Route;
use Illuminate\Foundation\Support\Providers\RouteServiceProvider as ServiceProvider;
class RouteServiceProvider extends ServiceProvider
{
/**
* The module namespace to assume when generating URLs to actions.
*/
protected string $moduleNamespace = 'Modules\Blog\App\Http\Controllers';
/**
* Called before routes are registered.
*
* Register any model bindings or pattern based filters.
*/
public function boot(): void
{
parent::boot();
}
/**
* Define the routes for the application.
*/
public function map(): void
{
$this->mapApiRoutes();
$this->mapWebRoutes();
// Register tenant routes
$this->mapTenantRoutes();
}
/**
* Define the "web" routes for the application.
*
* These routes all receive session state, CSRF protection, etc.
*/
protected function mapWebRoutes(): void
{
foreach ($this->centralDomains() as $domain) {
Route::middleware('web')
->namespace($this->moduleNamespace)
->domain($domain)
->group(module_path('Blog', '/routes/web.php'));
}
}
/**
* Define the "api" routes for the application.
*
* These routes are typically stateless.
*/
protected function mapApiRoutes(): void
{
foreach ($this->centralDomains() as $domain) {
Route::prefix('api')
->middleware('api')
->namespace($this->moduleNamespace)
->domain($domain)
->group(module_path('Blog', '/routes/api.php'));
}
}
/**
* Define the "tenant" routes for the application.
*
* These routes are typically stateless.
*/
protected function mapTenantRoutes(): void
{
$this->app->booted(function () {
if (file_exists(module_path('Blog', '/routes/tenant.php'))) {
Route::namespace($this->moduleNamespace)
->group(module_path('Blog', '/routes/tenant.php'));
}
});
}
/**
* Define the routes for the application.
*/
protected function centralDomains(): array
{
return config('tenancy.central_domains');
}
}
Now try it after the composer dump-autoload command.
php artisan route:list
php artisan serve
Subscribe to my newsletter
Read articles from Syed Shazeedul Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
