Python for DevOps: Keywords, Variables, and Best Practices
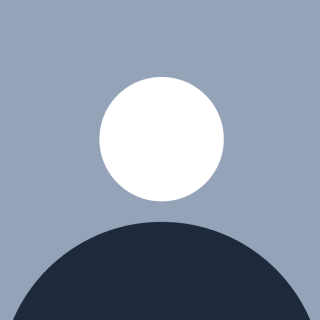
Introduction
In the third day of the Python for DevOps series, Abhishek Veeramalla delves into two fundamental concepts that are crucial for any Python programmer: keywords and variables. This article breaks down the key learnings from the session.
Keywords: The Building Blocks of Python
What are Keywords?
Keywords are reserved words in Python that have special meanings and purposes. They are essential for writing any Python program, serving as the foundation for creating logic and implementing functionality.
Importance of Keywords
Abhishek highlights four strong pillars of programming languages:
Understanding keywords
Learning data types
Mastering operators
Developing logical reasoning skills
Common Keywords for DevOps Engineers
Some popular keywords include:
and
,or
,not
(logical operators)if
,else
,elif
(conditional statements)while
,for
(loops)try
,except
,finally
(error handling)def
,return
(function definitions)class
(object-oriented programming)import
,from
(module imports)True
,False
,None
(boolean and null values)
Variables: Flexible and Dynamic
What are Variables?
Variables are containers for storing data values. In Python, they are dynamically typed, meaning you don't need to declare the type of variable before using it.
Variable Declaration
# Simple variable declaration
name = "Abhishek"
age = 30
is_developer = True
Advantages of Using Variables
Easy Updates: Change a value in one place, and it updates everywhere in the code
Reduced Errors: Minimize mistakes by centralizing value declarations
Code Readability: Make code more understandable and maintainable
Scope of Variables
Global Variables: Declared outside functions, accessible everywhere
Local Variables: Declared inside functions, accessible only within that function
Naming Conventions
Use lowercase for variable names
Use snake_case or camelCase for multi-word variables
Be descriptive in naming
# Good variable naming
ec2_instance_name = "Project XYZ"
full_name = "Abhishek Bala"
Conclusion
Understanding keywords and variables is crucial for writing effective Python code. Practice and consistent application of these concepts will help you become proficient in Python programming
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by