Understanding Functions, Modules, and Packages in Python
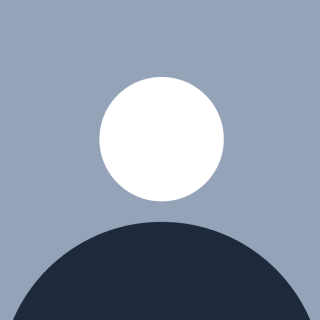
Functions in Python
Functions are reusable blocks of code that perform a specific task. They help in making the code more readable, modular, and easier to debug. Here's a simple example of a function in Python:
def add(a, b):
return a + b
result = add(2, 3)
print("Addition:", result)
Advantages of Using Functions:
Readability: Code is easier to read and understand.
Reusability: Functions can be reused across different parts of the program.
Debugging: Easier to isolate and fix issues.
Modules in Python
A module is a file containing Python definitions and statements. It can include functions, classes, and variables. Modules help in organizing code logically.
Creating a Module:
Suppose you have a file calculator.py
with the following content:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
You can import this module in another Python file:
import calculator
result = calculator.add(5, 3)
print("Addition:", result)
Packages in Python
A package is a collection of modules. It allows for a hierarchical structuring of the module namespace using dot notation. Packages are directories containing a special __init__.py
file.
Example of a Package Structure:
mypackage/
__init__.py
module1.py
module2.py
Virtual Environments
Virtual environments are a way to create isolated Python environments. They allow different projects to have their own dependencies, regardless of what dependencies every other project has.
Creating a Virtual Environment:
python -m venv myenv
source myenv/bin/activate # On Windows use `myenv\Scripts\activate`
Conclusion
Understanding functions, modules, and packages is essential for writing efficient and maintainable Python code, especially in a DevOps context. Virtual environments further enhance this by managing dependencies effectively.
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by