Chapter 37:Exception Handling in Java(Part 4)
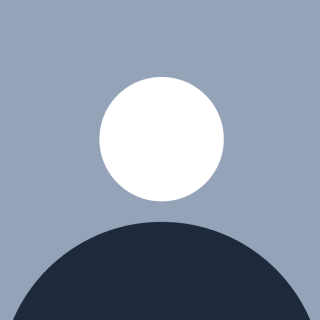
Table of contents
- Overview of Exception Handling in Java
- Importance of Managing Resources Efficiently
- Key Topics Covered
- 2. Try with Resources
- 3. Multi-Catch Blocks
- 4. Rules of Overriding Associated with Exceptions
- 5. Topics Left by Nithin Sir
- 6. File Concepts in Java
- 7. Advanced Concepts and Rules
- 8. Common Scenarios and FAQs
- 9. Diagrams and Visual Aids
- 10. Conclusion

Overview of Exception Handling in Java
Exception handling is a critical concept in Java, allowing developers to manage runtime errors and maintain the smooth execution of applications. By using structured mechanisms like try-catch
blocks, Java ensures that unexpected situations can be managed gracefully without abrupt application termination. Exception handling also aids in debugging and prevents resource leaks.
Importance of Managing Resources Efficiently
Efficient resource management is essential for applications to run reliably and perform well. Resources such as file streams, database connections, or network sockets must be properly managed to prevent memory leaks or other performance issues. Traditionally, resource handling in Java involved significant boilerplate code, particularly when closing resources explicitly in finally
blocks. With enhancements in Java 1.7, modern techniques simplify this process.
Key Topics Covered
Try with Resources
A feature introduced in Java 1.7 to simplify resource management by automatically closing resources at the end of thetry
block.Multi-Catch Blocks
Enables handling multiple exceptions in a singlecatch
block, improving code readability and reducing redundancy.Rules of Overriding with Exceptions
Discusses the constraints when overriding methods that throw exceptions, focusing on compile-time and runtime considerations.instanceOf
vs.isInstanceOf(Object obj)
Explores the difference between the Javainstanceof
operator and any custom implementations of type-checking methods.User-Defined Packages
Covers the creation and usage of custom packages in real-time projects to organize code effectively and ensure reusability..
2. Try with Resources
2.1 Background
Pre-JDK 1.7 resource management.
Problems with the traditional try-catch-finally approach.
Introduction to "boilerplate code" and why itโs problematic.
2.2 How Try with Resources Solves the Problem
Automatic resource management.
No need for explicit closing in finally blocks.
Simplified syntax and reduced complexity.
2.3 Syntax and Implementation
Code snippets demonstrating try-with-resources.
Comparing traditional vs try-with-resources approaches.
Handling multiple resources in a single try block.
2.4 Rules for Try with Resources
Requirement for resources to implement
AutoCloseable
.Understanding implicit finality of resources.
Practical examples and common pitfalls.
2.5 Real-World Scenarios
Managing multiple resources in a project.
Examples from real-time systems like file handling, database connections, etc.
3. Multi-Catch Blocks
3.1 Traditional Exception Handling
Separate catch blocks for different exceptions.
Code verbosity and maintenance issues.
3.2 Introduction to Multi-Catch Blocks
Syntax and implementation.
Reducing redundancy and improving readability.
3.3 Rules and Limitations
Restriction on catching unrelated exceptions together.
Use of the
|
operator for grouping exceptions.Best practices and scenarios.
4. Rules of Overriding Associated with Exceptions
4.1 Basics of Overriding
What is method overriding in Java?
Rules for overriding methods.
4.2 Exception Handling in Overridden Methods
Checked vs unchecked exceptions.
Rules for declaring exceptions in overridden methods.
Examples to demonstrate scenarios and compile-time behaviors.
4.3 Common Mistakes
Declaring incompatible exceptions.
Misunderstanding exception hierarchies.
5. Topics Left by Nithin Sir
5.1 instanceOf
vs isInstanceOf(Object obj)
Understanding
instanceOf
operator.Differentiating
instanceOf
from customisInstanceOf
methods.Use cases and examples.
5.2 User-Defined Packages
Steps to create a package in Java.
Using packages in real-time projects.
Advantages of modular programming with packages.
6. File Concepts in Java
6.1 Overview
File handling and its importance.
Structure of files in a hard disk.
6.2 Working with FileReader and BufferedReader
Code walkthroughs.
Explaining the medium of communication between
.java
and.txt
files.Handling common file-handling errors.
6.3 Real-World Applications
Example projects involving file reading/writing.
Error handling and debugging file I/O issues.
7. Advanced Concepts and Rules
7.1 Behind the Scenes of Try with Resources
Role of
AutoCloseable
andCloseable
interfaces.How JVM manages resources under the hood.
7.2 Implicit Finality of Resources
Explaining why resources in try-with-resources are implicitly final.
Examples and compile-time error scenarios.
7.3 Resource Leak Prevention
Consequences of not closing resources.
JVMโs role in preventing leaks with try-with-resources.
8. Common Scenarios and FAQs
Handling legacy code without try-with-resources.
Mixing try-with-resources and traditional try-catch-finally blocks.
Troubleshooting compile-time and runtime errors.
9. Diagrams and Visual Aids
10. Conclusion
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
๐ Tech Enthusiast | Full Stack Developer | System Design Explorer ๐ป Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iโm Rohit Gawande! ๐I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iโm Currently Doing ๐น Writing an in-depth System Design Series to help developers master complex design concepts.๐น Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.๐น Exploring advanced Java concepts and modern web technologies. What You Can Expect Here โจ Detailed technical blogs with examples, diagrams, and real-world use cases.โจ Practical guides on Java, System Design, and Full Stack Development.โจ Community-driven discussions to learn and grow together. Letโs Connect! ๐ GitHub โ Explore my projects and contributions.๐ผ LinkedIn โ Connect for opportunities and collaborations.๐ LeetCode โ Check out my problem-solving journey. ๐ก "Learning is a journey, not a destination. Letโs grow together!" Feel free to customize or add more based on your preferences! ๐