Enhance Your Development Skills with SOLID Principles

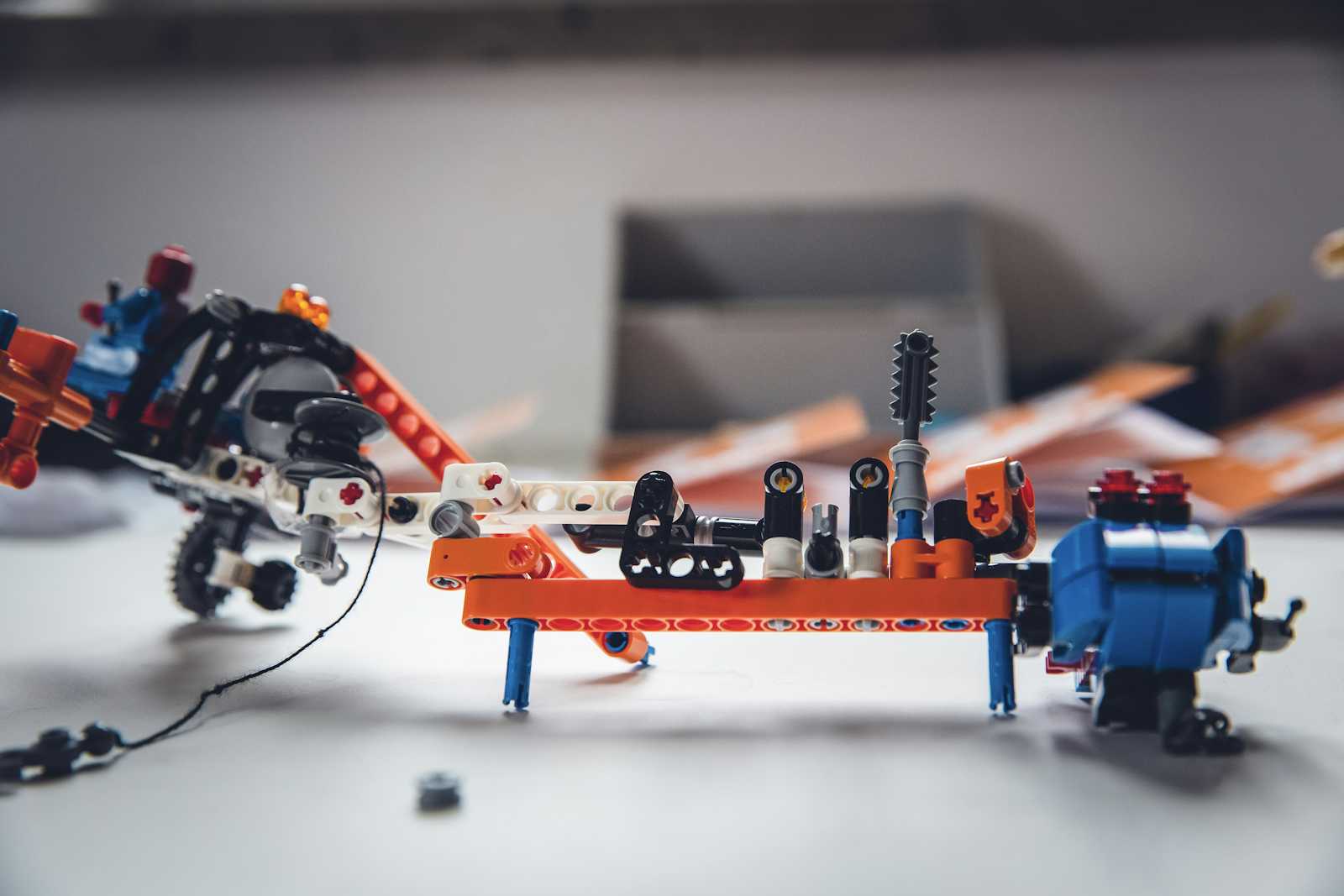
Introduction
Hey there, young coder! ๐ Today, we're going to learn about some super cool principles that help us write better code. These principles are called SOLID. Imagine SOLID principles as the rules of a game that make sure everyone has fun and the game runs smoothly. Let's dive in and see how we can use these principles in front-end development with Node.js and JavaScript!
Single Responsibility Principle (SRP)
Analogy: Imagine you have a toy robot that can walk, talk, and dance. If one part of the robot breaks, you don't want the whole robot to stop working, right? Each part should have its own job.
In Code: In JavaScript, this means each function or module should do one thing and do it well. For example, if you have a function that fetches data and another that displays it, keep them separate.
// Fetching data
async function fetchData(url) {
const response = await fetch(url);
return response.json();
}
// Displaying data
function displayData(data) {
console.log(data);
}
// Using the functions
fetchData('https://api.example.com/data')
.then(data => displayData(data));
Open/Closed Principle (OCP)
Analogy: Think of your favorite LEGO set. You can add new pieces to build something new without changing the original pieces.
In Code: This means your code should be open for extension but closed for modification. You can add new features without changing existing code.
// Base class
class Animal {
speak() {
console.log('Animal speaks');
}
}
// Extended class
class Dog extends Animal {
speak() {
console.log('Dog barks');
}
}
// Using the classes
const myDog = new Dog();
myDog.speak(); // Dog barks
Liskov Substitution Principle (LSP)
Analogy: Imagine you have a toy car and a toy truck. Both can move forward and backward. You can replace the car with the truck, and it should still work the same way.
In Code: This means that objects of a superclass should be replaceable with objects of a subclass without affecting the functionality.
class Bird {
fly() {
console.log('Bird flies');
}
}
class Sparrow extends Bird {
fly() {
console.log('Sparrow flies');
}
}
function makeBirdFly(bird) {
bird.fly();
}
const mySparrow = new Sparrow();
makeBirdFly(mySparrow); // Sparrow flies
Interface Segregation Principle (ISP)
Analogy: Imagine you have different toys for different activities: a ball for playing catch and a puzzle for solving. You don't want a toy that tries to do everything but doesn't do anything well.
In Code: This means that a class should not be forced to implement interfaces it doesn't use. Instead, create specific interfaces for specific tasks.
// Interface for flying
class Flyable {
fly() {
throw new Error('This method should be overridden');
}
}
// Interface for swimming
class Swimmable {
swim() {
throw new Error('This method should be overridden');
}
}
// Class that implements Flyable
class Eagle extends Flyable {
fly() {
console.log('Eagle flies');
}
}
// Class that implements Swimmable
class Fish extends Swimmable {
swim() {
console.log('Fish swims');
}
}
Dependency Inversion Principle (DIP)
Analogy: Think of a remote-controlled car. The remote (high-level module) should work with any car (low-level module) as long as the car follows the rules set by the remote.
In Code: This means high-level modules should not depend on low-level modules. Both should depend on abstractions.
// Abstraction
class Engine {
start() {
throw new Error('This method should be overridden');
}
}
// Low-level module
class ElectricEngine extends Engine {
start() {
console.log('Electric engine starts');
}
}
// High-level module
class Car {
constructor(engine) {
this.engine = engine;
}
start() {
this.engine.start();
}
}
// Using the modules
const myEngine = new ElectricEngine();
const myCar = new Car(myEngine);
myCar.start(); // Electric engine starts
Conclusion
By following these SOLID principles, we can write code that is easier to understand, maintain, and extend. Just like building with LEGO bricks or playing with different toys, these principles help us create amazing things without breaking what we already have. Happy coding! ๐
Subscribe to my newsletter
Read articles from Pawan Gangwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pawan Gangwani
Pawan Gangwani
Iโm Pawan Gangwani, a passionate Full Stack Developer with over 12 years of experience in web development. Currently serving as a Lead Software Engineer at Lowes India, I specialize in modern web applications, particularly in React and performance optimization. Iโm dedicated to best practices in coding, testing, and Agile methodologies. Outside of work, I enjoy table tennis, exploring new cuisines, and spending quality time with my family.