A Practical Guide for Software Engineers to Understand and Tackle Complex Business Domains
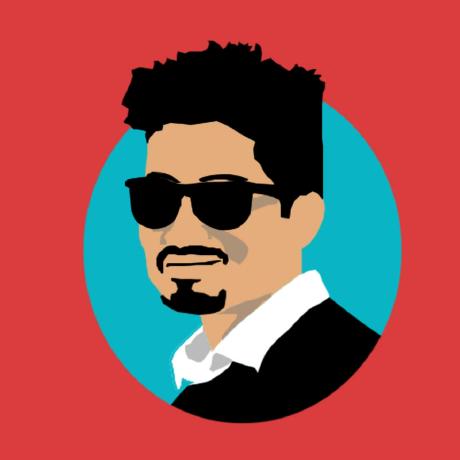

The complexity of modern software systems is an undeniable challenge for developers and organizations alike. As systems scale, they must accommodate evolving requirements, integrate with disparate technologies, and remain adaptable. Without a robust approach to managing this complexity, teams risk creating fragile architectures, accumulating technical debt, and missing the mark on delivering value to the business.
Enter Domain-Driven Design (DDD)—a methodology introduced by Eric Evans that emphasizes the creation of software systems deeply aligned with the business domains they serve. DDD provides tools, strategies, and philosophies to ensure that software reflects the intricate realities of its domain, all while fostering collaboration between technical and non-technical stakeholders.
By the end of this guide, you’ll have an actionable understanding of how to design systems that thrive in the face of complexity.
What is Domain-Driven Design?
Domain-Driven Design is a philosophy and set of practices for tackling complexity in software systems. At its core, it focuses on modeling the business domain accurately and collaboratively, ensuring that software serves as a faithful representation of business processes.
Key Objectives
Simplify Complexity: Focus on understanding and explicitly modeling the domain.
Build a Shared Understanding: Create a ubiquitous language shared by developers, stakeholders, and domain experts.
Focus on the Core: Prioritize areas of the system that directly drive business value.
Foundational Principles of DDD
1. Ubiquitous Language
In DDD, a ubiquitous language bridges the gap between technical and business stakeholders. It is a shared vocabulary that evolves alongside the domain model, ensuring clarity and consistency in all aspects of the software.
Why It Matters
Reduces ambiguity in requirements and communication.
Aligns code with business terminology, making it more understandable.
Serves as a foundation for collaboration and continuous refinement.
Example in Python
Consider an e-commerce platform where we use the terms Order
, OrderStatus
, and OrderTotal
. These terms form the ubiquitous language and appear consistently across documentation, conversations, and code.
class OrderStatus:
PENDING = "Pending"
SHIPPED = "Shipped"
DELIVERED = "Delivered"
class Order:
def __init__(self, order_id, customer, items):
self.order_id = order_id
self.customer = customer
self.items = items
self.status = OrderStatus.PENDING
def calculate_total(self):
return sum(item.price * item.quantity for item in self.items)
def mark_as_shipped(self):
self.status = OrderStatus.SHIPPED
def mark_as_delivered(self):
self.status = OrderStatus.DELIVERED
By using domain-specific terms (OrderStatus
, calculate_total
, mark_as_shipped
), the code becomes a living part of the ubiquitous language.
2. Bounded Contexts
A bounded context defines the scope within which a particular domain model applies. It ensures consistency in terminology and rules within the context while allowing for different models in other contexts.
Key Benefits
Avoids ambiguity by clearly defining where specific terms and rules apply.
Enables modularity by allowing teams to focus on one context at a time.
Simplifies integration through clearly defined boundaries.
Example
In an e-commerce platform:
Ordering Context: Handles placing and managing customer orders.
Shipping Context: Focuses on delivery logistics.
Code Example
# Ordering Context
class Order:
def __init__(self, order_id, items):
self.order_id = order_id
self.items = items
# Shipping Context
class Shipment:
def __init__(self, shipment_id, order_id):
self.shipment_id = shipment_id
self.order_id = order_id
self.status = "In Transit"
While the Order
and Shipment
entities both refer to an order ID, their meanings and responsibilities differ across contexts.
Advanced Integration
Bounded contexts often integrate through anticorruption layers or domain events. For example, when an order is marked as shipped in the Ordering Context, an event triggers the creation of a shipment in the Shipping Context.
class OrderShippedEvent:
def __init__(self, order_id):
self.order_id = order_id
3. Focus on the Core Domain
Not all parts of a system are equally critical. DDD emphasizes identifying and focusing on the core domain—the area that provides the most significant business value.
Subdomains
Core Domain: The heart of the business; requires the most attention.
Supporting Subdomains: Secondary systems that assist the core domain.
Generic Subdomains: Common areas that can often be outsourced or commoditized.
Example
In a ride-sharing platform:
Core Domain: Trip management (matching riders and drivers).
Supporting Subdomains: Notifications, ratings, and promotions.
Generic Subdomains: Payment processing.
Implementation in Python
Core domains typically have custom logic and are highly optimized for the business.
class Trip:
def __init__(self, trip_id, rider, driver):
self.trip_id = trip_id
self.rider = rider
self.driver = driver
self.status = "Scheduled"
def start_trip(self):
self.status = "In Progress"
def complete_trip(self):
self.status = "Completed"
Building Blocks of DDD
1. Entities
Entities have unique identities and lifecycles, making them central to the domain model.
Example
A Customer
entity in an e-commerce platform:
class Customer:
def __init__(self, customer_id, name, email):
self.customer_id = customer_id
self.name = name
self.email = email
2. Value Objects
Value objects represent domain attributes and are immutable.
Example
An Address
value object in a shipping context:
class Address:
def __init__(self, street, city, postal_code):
self.street = street
self.city = city
self.postal_code = postal_code
3. Aggregates and Aggregate Roots
Aggregates ensure consistency by grouping related entities and value objects under an aggregate root.
Example
An Order
aggregate with OrderItem
entities:
class OrderItem:
def __init__(self, product_id, quantity, price):
self.product_id = product_id
self.quantity = quantity
self.price = price
class Order:
def __init__(self, order_id, customer):
self.order_id = order_id
self.customer = customer
self.items = []
def add_item(self, product_id, quantity, price):
self.items.append(OrderItem(product_id, quantity, price))
def calculate_total(self):
return sum(item.price * item.quantity for item in self.items)
4. Domain Events
Domain events capture significant occurrences within the domain.
Example
An OrderPlacedEvent
:
class OrderPlacedEvent:
def __init__(self, order_id, customer_id):
self.order_id = order_id
self.customer_id = customer_id
5. Repositories
Repositories abstract persistence, enabling developers to interact with aggregates without worrying about storage.
Example
class OrderRepository:
def __init__(self):
self.orders = {}
def save(self, order):
self.orders[order.order_id] = order
def find_by_id(self, order_id):
return self.orders.get(order_id)
Advanced Topics
1. Event Sourcing
Store domain events as the source of truth.
class EventStore:
def __init__(self):
self.events = []
def append(self, event):
self.events.append(event)
def get_events(self):
return self.events
2. Command Query Responsibility Segregation (CQRS)
Separate read and write operations for scalability.
Example
class OrderQueryService:
def __init__(self, repository):
self.repository = repository
def get_order_details(self, order_id):
order = self.repository.find_by_id(order_id)
return {
"order_id": order.order_id,
"status": order.status,
"total": order.calculate_total()
}
Conclusion
Domain-Driven Design provides a comprehensive framework for managing complexity in software development. By focusing on the core domain, fostering collaboration through ubiquitous language, and using strategic patterns like aggregates and bounded contexts, DDD enables the creation of scalable, maintainable, and meaningful systems.
As you implement these principles, remember that the goal of DDD is not just technical elegance but creating software that truly meets the needs of the business.
For any queries reach out to me on AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
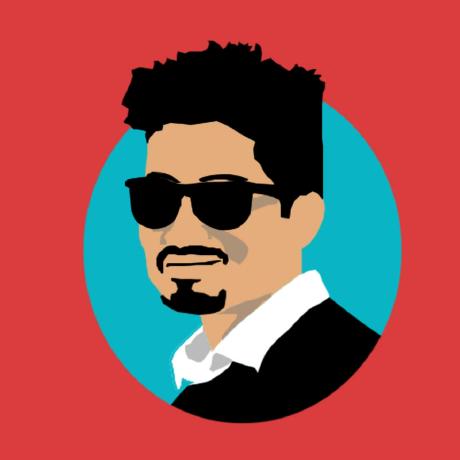