Switching Your SaaS Project from Stripe to Adyen: A Comprehensive Guide
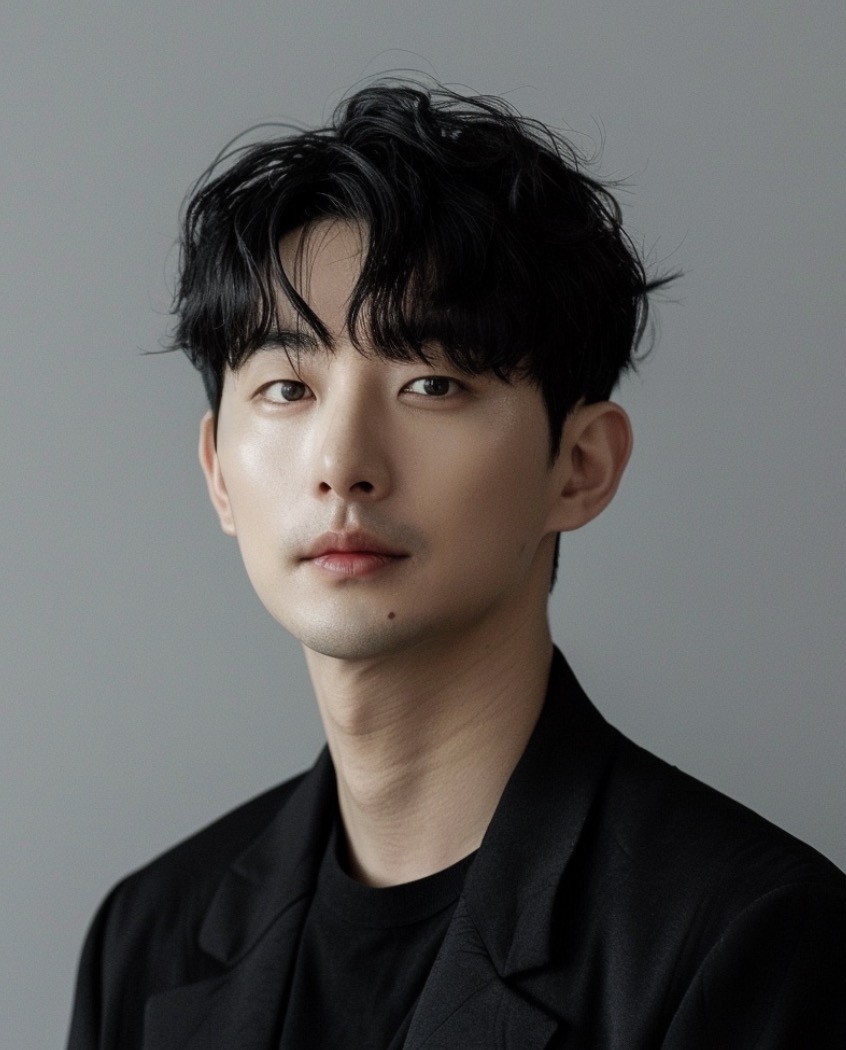

Discover how to seamlessly transition your SaaS project from Stripe to Adyen for payments, utilizing Next.js. Explore expert tips and insights on integrating Adyen's API to optimize your payment processing capabilities.
To replace the Stripe API with Adyen in your SaaS project built on Next.js, you can follow these steps. Adyen provides a comprehensive API and Drop-in component to handle payments, making the transition straightforward. Below is a step-by-step guide based on the resources provided.
Steps to Replace Stripe with Adyen
1. Install the Adyen Node.js SDK
Install the official Adyen API library for Node.js, which will allow you to interact with Adyen's APIs.
npm install @adyen/api-library
2. Set Up Environment Variables
Obtain your API key and merchant account from the Adyen Customer Area and store them in a .env.local
file for secure access.
ADYEN_API_KEY=<YOUR_API_KEY>
ADYEN_MERCHANT_ACCOUNT=<YOUR_MERCHANT_ACCOUNT>
NEXT_PUBLIC_ADYEN_CLIENT_KEY=<YOUR_CLIENT_KEY>
NEXT_PUBLIC_ADYEN_ENVIRONMENT=TEST # Use "LIVE" for production
3. Create an API Endpoint for Session Creation
Adyen requires a session to initialize the Drop-in component. Create a new endpoint (pages/api/session.ts
) to handle session creation.
import { NextApiRequest, NextApiResponse } from 'next';
import { Client, Config, CheckoutAPI } from '@adyen/api-library';
const config = new Config({
apiKey: process.env.ADYEN_API_KEY,
environment: 'TEST', // Use 'LIVE' for production
});
const client = new Client({ config });
const checkout = new CheckoutAPI(client);
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const merchantAccount = process.env.ADYEN_MERCHANT_ACCOUNT ?? '';
try {
const response = await checkout.sessions({
amount: { currency: 'EUR', value: 10000 }, // Amount in minor units (e.g., 100€ = 10000)
reference: 'YOUR_ORDER_REFERENCE',
returnUrl: 'http://localhost:3000/checkout-success',
merchantAccount,
});
res.status(200).json(response);
} catch (error) {
console.error(error);
res.status(500).json({ error: error.message });
}
}
4. Integrate the Adyen Drop-in Component on the Frontend
Use Adyen's Drop-in component to handle payment details securely. Install the necessary frontend library:
npm install @adyen/adyen-web
Add the Drop-in component to your checkout page (e.g., pages/checkout.tsx
).
import React, { useEffect, useRef } from 'react';
import AdyenCheckout from '@adyen/adyen-web';
const CheckoutPage = () => {
const paymentContainer = useRef(null);
useEffect(() => {
const fetchSession = async () => {
const response = await fetch('/api/session');
const session = await response.json();
const checkout = await AdyenCheckout({
environment: process.env.NEXT_PUBLIC_ADYEN_ENVIRONMENT,
clientKey: process.env.NEXT_PUBLIC_ADYEN_CLIENT_KEY,
session,
onPaymentCompleted: (result) => {
console.log('Payment completed:', result);
},
onError: (error) => {
console.error('Payment error:', error);
},
});
if (paymentContainer.current) {
checkout.create('dropin').mount(paymentContainer.current);
}
};
fetchSession();
}, []);
return <div ref={paymentContainer}></div>;
};
export default CheckoutPage;
5. Handle Webhooks for Payment Confirmation
Adyen sends payment status updates via webhooks. Create another API endpoint (pages/api/webhooks.ts
) to handle these notifications.
import { NextApiRequest, NextApiResponse } from 'next';
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const notification = req.body;
// Verify webhook signature here if needed
if (notification.eventCode === 'AUTHORISATION' && notification.success === 'true') {
console.log('Payment authorized:', notification.pspReference);
// Update order status in your database
}
res.status(200).send('[accepted]');
}
6. Update Your Payment Flow
Replace Stripe-specific logic in your backend and frontend with the Adyen session creation and Drop-in integration.
Ensure that your order management system updates based on Adyen's webhook notifications.
Key Considerations
Security: Ensure sensitive keys like
ADYEN_API_KEY
are stored securely and not exposed in frontend code.Testing: Use Adyen's test environment (
environment: TEST
) during development.Compliance: Verify compliance with PCI DSS since Adyen handles payment details securely via its Drop-in component.
Customization: The Drop-in component can be customized to match your branding.
By following these steps, you can successfully transition from Stripe to Adyen for payment processing in your Next.js SaaS project. For further details, refer to [Magnus Wahlstrand's guide] or the [Adyen Node.js SDK documentation].
What are the main differences between Stripe and Adyen APIs
Here is a detailed comparison of the main differences between Stripe and Adyen APIs, based on the provided information:
Stripe vs. Adyen: Key Differences
Feature | Stripe | Adyen |
Target Audience | Ideal for small to medium-sized businesses, startups, and e-commerce platforms. | Best suited for large enterprises with complex, omnichannel payment needs. |
Payment Methods | Supports 100+ payment methods, including major credit cards and local payment options in 25+ countries. | Supports over 250 payment methods globally, including many alternative and local options. |
Global Reach | Available in 35+ countries, focusing on major e-commerce markets like the U.S., Europe, and Australia. | Operates in 40+ countries with broader coverage, including regions like South America, Africa, and the Middle East. |
Integration & APIs | Developer-friendly API with extensive documentation, pre-built checkout pages, and simplified onboarding. | Offers robust APIs but requires more technical expertise for setup; focuses on end-to-end solutions. |
Customization | Highly customizable checkout flows and features via APIs; suitable for tech-savvy businesses. | Limited customization compared to Stripe; emphasizes a unified payment platform. |
Omnichannel Support | Primarily online payments; supports in-person payments via card readers but lacks full POS integration out-of-the-box. | Seamlessly integrates online and offline payments for a unified commerce experience. |
Pricing Model | Flat-rate pricing (e.g., 2.9% + $0.30 per transaction); transparent fee structure. | Interchange-plus pricing tailored to payment methods and regions; better for high-volume merchants. |
Fraud Prevention | Machine learning-based fraud detection (Stripe Radar). | Advanced fraud protection, including tokenization and encryption, optimized for global transactions. |
Customer Support | Comprehensive support with 24/7 availability and extensive educational resources (e.g., guides, videos). | Limited customer support options; focuses more on enterprise-level assistance. |
Additional Features | - Subscription billing (Stripe Billing) - Virtual/physical card issuing - Tax automation and revenue recognition tools. | - Payment routing and recovery (Adyen Revenue Optimization) - Donation prompts at checkout (Adyen Impact) - Flexible cash advances (Adyen Capital). |
Summary of Key Points
Stripe is ideal for startups and small to medium-sized businesses due to its ease of integration, developer-friendly APIs, flat-rate pricing, and extensive customization options.
Adyen is better suited for large enterprises needing a unified solution for online and offline payments, global reach with diverse payment methods, and advanced fraud prevention.
Can I use the same frontend code with both Stripe and Adyen
Yes, you can use the same frontend code for both Stripe and Adyen to some extent, but there are key differences in how the two platforms handle payment flows and APIs that may require adjustments, especially in backend integration and certain frontend configurations. Here's a detailed breakdown:
Frontend Code Similarities
Both Stripe and Adyen support modern frontend integrations using JavaScript SDKs or Drop-in components. If your frontend is designed to interact with a backend API for session creation and token handling, much of the frontend logic can remain the same. For example:
Both platforms use client-side SDKs to securely collect payment details.
Both provide pre-built UI components (e.g., Stripe Elements and Adyen Drop-in) that simplify payment form creation.
Both platforms allow you to handle payment events (e.g., success, failure) in a similar manner.
Frontend Code Differences
While the general structure of your frontend code can be shared, there are specific differences that may require modifications:
Aspect | Stripe | Adyen |
SDK/Library | Uses @stripe/stripe-js for client-side interactions and Stripe Elements for pre-built UI components. | Uses @adyen/adyen-web for client-side interactions and Adyen Drop-in for pre-built UI components. |
Session Handling | Relies on a PaymentIntent or SetupIntent object created on the backend and passed to the frontend. | Requires a session object created on the backend, which is used to initialize the Drop-in component. |
UI Customization | Highly customizable via Stripe Elements (e.g., custom fields for card input). | Limited customization with Adyen Drop-in; focuses on providing a unified UI for all payment methods. |
Event Handling | Handles events like onSuccess , onError , and onCancel through Stripe's JavaScript library. | Similar event handling but uses Adyen-specific callbacks like onPaymentCompleted and onError . |
Tokenization | Uses Stripe's tokenization system (token , source , or paymentMethod ) to securely transmit payment details. | Uses Adyen's encrypted payment data or tokens generated via the Drop-in component. |
Backend Adjustments
While much of the frontend code can remain similar, the backend logic will need significant changes because:
Session Creation:
Stripe uses objects like
PaymentIntent
orSetupIntent
.Adyen requires creating a session object via its API.
Webhook Handling:
Stripe relies on webhooks to confirm payment status (e.g.,
payment_intent.succeeded
).Adyen also uses webhooks but with different event types (e.g.,
AUTHORISATION
).
Example: Frontend Code Comparison
Stripe Integration:
import { loadStripe } from '@stripe/stripe-js';
const stripePromise = loadStripe('your-publishable-key');
async function handlePayment() {
const stripe = await stripePromise;
const { error } = await stripe.redirectToCheckout({ sessionId: 'your-session-id' });
if (error) {
console.error(error);
}
}
Adyen Integration:
import AdyenCheckout from '@adyen/adyen-web';
async function handlePayment() {
const response = await fetch('/api/session'); // Backend creates Adyen session
const session = await response.json();
const checkout = await AdyenCheckout({
environment: 'test',
clientKey: 'your-client-key',
session,
onPaymentCompleted: (result) => console.log('Payment completed:', result),
onError: (error) => console.error('Payment error:', error),
});
checkout.create('dropin').mount('#dropin-container');
}
Key Considerations
Shared Frontend Logic: If your frontend is modularized to call backend APIs for session creation, switching between Stripe and Adyen will primarily involve swapping SDKs and adjusting initialization logic.
Custom Checkout UI: If you use custom UI components instead of pre-built ones (like Stripe Elements or Adyen Drop-in), you can reuse most of your code by handling tokenization/encryption differently.
Event Handling: Ensure that your event handlers are updated to match the specific callbacks provided by each platform.
Conclusion
You can reuse much of your frontend code if it's designed generically to interact with a backend API for payment processing. However, you'll need to adapt specific parts of the integration—such as SDK initialization, session handling, and event callbacks—to accommodate differences between Stripe and Adyen APIs.
What are the security implications of switching from Stripe to Adyen
Switching from Stripe to Adyen for payment processing in your SaaS project has several security implications. Both platforms are highly secure and compliant with industry standards, but they differ in their approaches to data protection, fraud prevention, and compliance responsibilities. Below is a detailed analysis of the security implications:
1. PCI DSS Compliance
Stripe: Stripe is a PCI DSS Level 1 Service Provider, the highest level of compliance. It handles sensitive payment data through tokenization and encryption, minimizing merchants' PCI scope. Merchants do not have to store or process cardholder data directly, reducing compliance burdens.
Adyen: Adyen is also a PCI DSS Level 1 Service Provider but offers additional flexibility with its end-to-end encryption (E2EE) and point-to-point encryption (P2PE) solutions. While Adyen reduces PCI scope significantly, merchants are still responsible for securing cardholder data before it reaches Adyen's systems.
Implication: Adyen may require more effort on your part to ensure PCI compliance at the integration points, whereas Stripe abstracts more of this responsibility.
2. Encryption and Tokenization
Stripe: Uses AES-256 encryption for payment data and tokenization to replace sensitive card information with non-sensitive tokens. This ensures that merchants never handle raw payment details.
Adyen: Implements advanced encryption methods like E2EE and P2PE, securing cardholder data from the point of entry to processing. Adyen also uses tokenization but emphasizes its ability to handle multi-channel payments securely.
Implication: Both platforms provide robust encryption, but Adyen's E2EE and P2PE solutions may offer enhanced security for omnichannel payments.
3. Fraud Prevention
Stripe: Offers Stripe Radar, an AI-driven fraud detection system that leverages global transaction data to identify suspicious activities. It includes tools like 3D Secure authentication and customizable fraud rules.
Adyen: Provides RevenueProtect, a machine-learning-based risk management system that detects fraud using custom risk rules, biometric authentication, and multi-factor authentication (MFA). It also supports advanced fraud detection for global transactions.
Implication: Both platforms offer strong fraud prevention tools, but Adyen's RevenueProtect may be better suited for enterprises with complex global operations.
4. Data Privacy and Compliance
Stripe: Complies with GDPR, CCPA, PSD2, and other regional regulations. It acts as both a data controller and processor, ensuring compliance with privacy laws while using aggregated transaction data to improve its services.
Adyen: Focuses on strict compliance with GDPR and other global privacy regulations. It provides tools for merchants to meet regional legal requirements but does not use aggregated data for service improvements in the same way Stripe does.
Implication: If privacy concerns around aggregated data usage are critical for your business, Adyen might align better with your needs.
5. Webhook Security
Stripe: Relies heavily on webhooks for payment updates (e.g.,
payment_intent.succeeded
). Webhook endpoints must be secured using secret keys or signature verification.Adyen: Also uses webhooks but requires merchants to validate notifications using HMAC signatures. This adds an extra layer of security.
Implication: Adyen's webhook validation process may provide stronger security against spoofing attacks compared to Stripe's standard implementation.
6. Multi-Channel Payment Security
Stripe: Primarily focuses on online payments but supports in-person payments via card readers.
Adyen: Offers seamless integration across online, in-app, and in-person payments with unified security measures like P2PE.
Implication: If your SaaS business involves omnichannel payments (e.g., online and physical stores), Adyen provides more comprehensive security across channels.
7. Chargeback Management
Stripe: Includes tools like Chargeback Protection to help merchants manage disputes effectively.
Adyen: Offers a similar feature through RevenueProtect but also provides advanced tools tailored for high-risk industries.
Implication: Both platforms are effective at managing chargebacks, but Adyen's tools may be better suited for enterprises handling high volumes of disputes.
Summary of Security Implications:
Aspect | Stripe | Adyen |
PCI DSS Compliance | Abstracts most responsibilities; minimal merchant effort required | Requires securing data before it reaches Adyen |
Encryption | AES-256 encryption; tokenization | E2EE/P2PE encryption; tokenization |
Fraud Prevention | Stripe Radar (AI-driven) | RevenueProtect (customizable risk rules) |
Data Privacy | Aggregates transaction data for service improvement | Strict GDPR compliance without aggregated data usage |
Webhook Security | Signature-based webhook verification | HMAC signature validation |
Multi-Channel Security | Online-first focus | Unified security across online and offline channels |
Chargeback Tools | Stripe Radar + Chargeback Protection | RevenueProtect tailored for enterprises |
Conclusion:
Switching from Stripe to Adyen introduces some additional responsibilities for securing cardholder data before it reaches Adyen's systems. However, Adyen offers advanced encryption methods (E2EE/P2PE), superior omnichannel support, and customizable fraud prevention tools that can benefit larger enterprises or businesses operating globally. If your SaaS project prioritizes ease of integration and minimal compliance burden, Stripe may remain a better fit; if you need enterprise-grade security features or omnichannel capabilities, Adyen is worth considering.
Subscribe to my newsletter
Read articles from Ewan Mak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
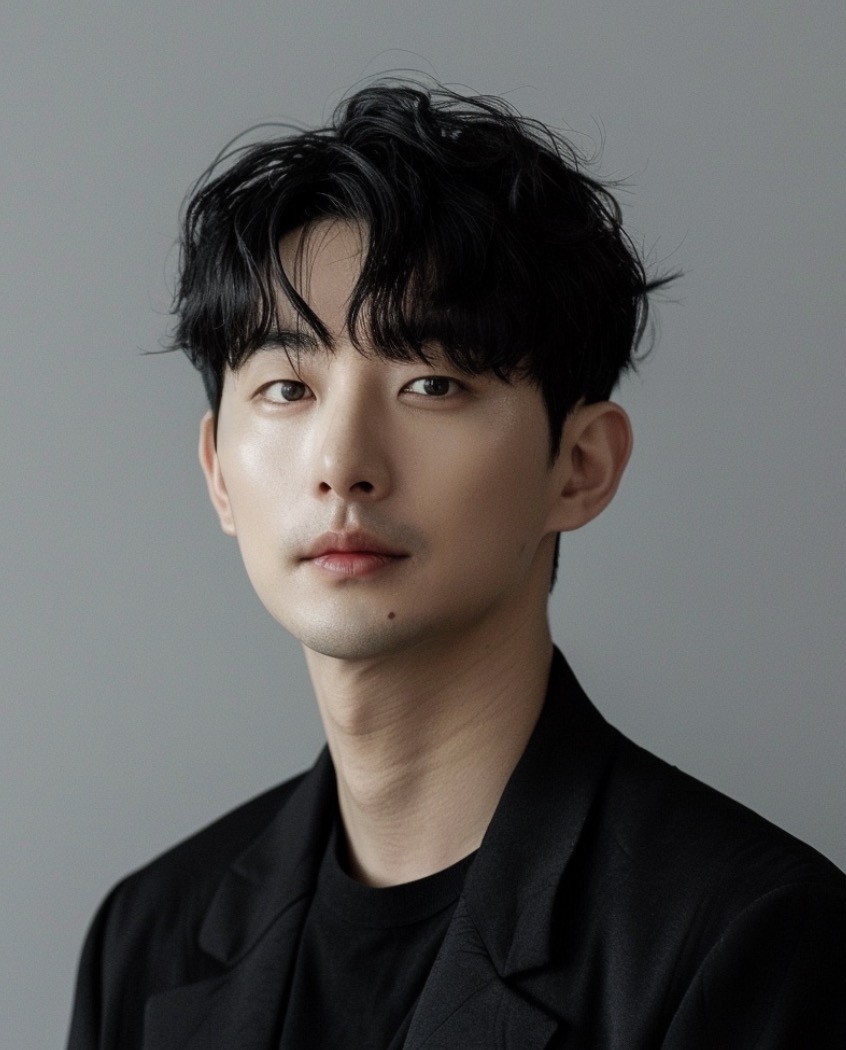
Ewan Mak
Ewan Mak
Crafting seamless user experiences with a passion for headless CMS, Vercel deployments, and Cloudflare optimization. I'm a Full Stack Developer with expertise in building modern web applications that are blazing fast, secure, and scalable. Let's connect and discuss how I can help you elevate your next project!