Understanding Django's Auto-discovery: A Deep Dive
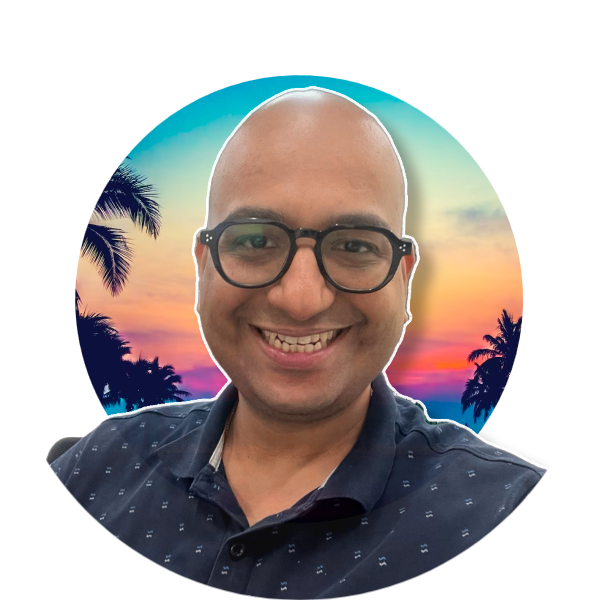
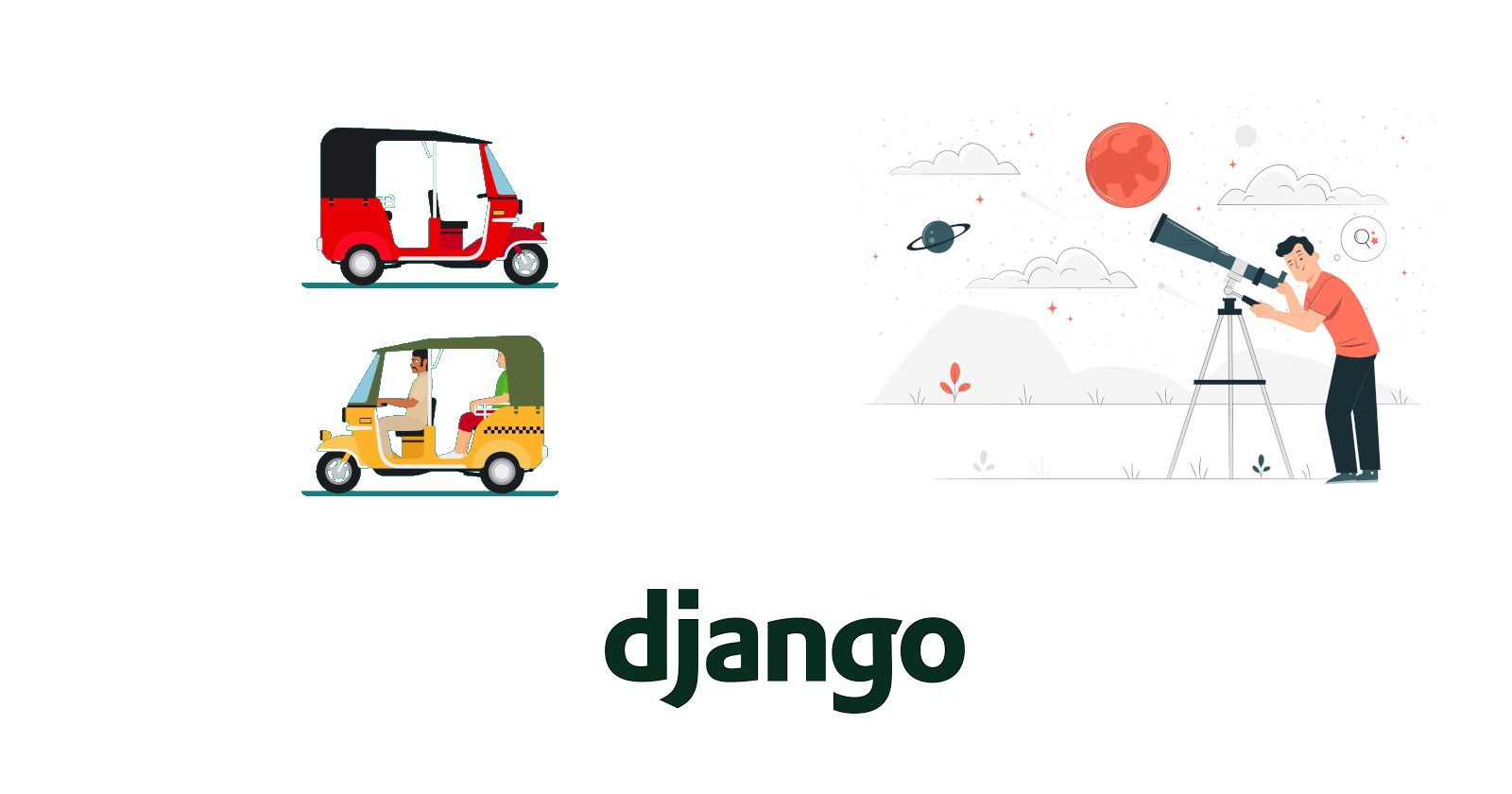
Django's auto-discovery feature is one of those magical elements that makes the framework both powerful and developer-friendly. While many developers use it daily, few understand how it works under the hood or how to leverage it effectively in their applications. In this comprehensive guide, we'll explore Django's auto-discovery mechanism, its common use cases, and how you can implement custom auto-discovery in your projects.
What is Auto-discovery?
At its core, auto-discovery is Django's way of automatically finding and loading certain components from your installed applications without requiring explicit registration. This feature follows Python's "convention over configuration" principle, making it easier to maintain a clean and organized code-base while reducing boilerplate code.
Common Use Cases
1. Admin Module Auto-discovery
The most familiar example of auto-discovery is Django's admin interface. When you include the admin URLs in your project, Django automatically searches for and registers models from each app's admin.py
:
# urls.py
from django.contrib import admin
from django.urls import path
admin.autodiscover() # This line triggers admin autodiscovery
urlpatterns = [
path('admin/', admin.site.urls),
]
In your app's admin.py
:
from django.contrib import admin
from .models import Product, Category
@admin.register(Product)
class ProductAdmin(admin.ModelAdmin):
list_display = ('name', 'price', 'category')
search_fields = ('name',)
@admin.register(Category)
class CategoryAdmin(admin.ModelAdmin):
list_display = ('name', 'created_at')
2. Management Commands
Django automatically discovers custom management commands placed in the management/commands
directory of your apps. This allows you to create powerful CLI commands without additional registration:
myapp/
└── management/
└── commands/
├── __init__.py
└── import_data.py
3. Template Tags and Filters
Custom template tags and filters are automatically discovered from the templatetags
directory:
myapp/
└── templatetags/
├── __init__.py
└── custom_filters.py
How Auto-discovery Works
Under the hood, Django's auto-discovery mechanism follows these steps:
App Configuration: Django reads the
INSTALLED_APPS
setting to get a list of all installed applications.Module Search: For each app, Django looks for specific module names (e.g.,
admin.py
,signals.py
).Import Process: When found, these modules are imported, executing any registration code they contain.
Registration: The discovered components are registered with their respective systems (admin site, URL patterns, etc.).
Implementing Custom Auto-discovery
You can implement your own auto-discovery mechanism for custom components. Here's a practical example:
# utils/discovery.py
from django.utils.module_loading import autodiscover_modules
def autodiscover_handlers():
"""
Automatically discover and register handlers from all installed apps.
"""
autodiscover_modules('handlers')
# handlers.py (in your app)
from .base import BaseHandler
class CustomHandler(BaseHandler):
def handle(self, data):
# Handler implementation
pass
# Register the handler
registry.register(CustomHandler)
Best Practices and Tips
Module Organization
Keep auto-discovered modules focused and single-purpose
Use clear naming conventions for auto-discovered files
Include
__init__.py
files in all directories
Performance Considerations
Auto-discovery happens at startup, so keep initialization code lightweight
Use lazy loading when possible to defer expensive operations
Consider caching mechanisms for frequently accessed components
Error Handling
from django.utils.module_loading import autodiscover_modules try: autodiscover_modules('custom_module') except ImportError as e: logger.warning(f"Failed to load custom module: {e}")
Advanced Auto-discovery Patterns
1. Selective Loading
You can implement selective auto-discovery based on settings or environment:
from django.conf import settings
from django.utils.module_loading import autodiscover_modules
def conditional_autodiscover():
if settings.ENABLE_CUSTOM_HANDLERS:
autodiscover_modules('handlers')
2. Custom Registry Pattern
Create a registry system for your auto-discovered components:
class Registry:
def __init__(self):
self._registry = {}
def register(self, name, component):
self._registry[name] = component
def get_component(self, name):
return self._registry.get(name)
registry = Registry()
Common Pitfalls and Solutions
Circular Imports
Use lazy imports or move imports inside functions
Structure your code to avoid circular dependencies
Load Order Issues
Use Django's app config and ready() method for initialization
Implement proper dependency handling in your auto-discovery code
Missing Modules
Implement proper error handling for missing modules
Provide clear error messages for debugging
Conclusion
Django's auto-discovery feature is a powerful tool that can significantly improve code organization and maintainability. By understanding how it works and following best practices, you can leverage it effectively in your projects. Whether you're using built-in auto-discovery features or implementing custom ones, this pattern helps maintain a clean and scalable Django application.
Remember that while auto-discovery is powerful, it should be used judiciously. Always consider the trade-offs between convenience and explicit registration, especially in larger applications where clear dependency management becomes crucial.
By mastering Django's auto-discovery, you'll be better equipped to build more maintainable and scalable Django applications while reducing boilerplate code and improving overall code organization.
Image attribution
Django Logo
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
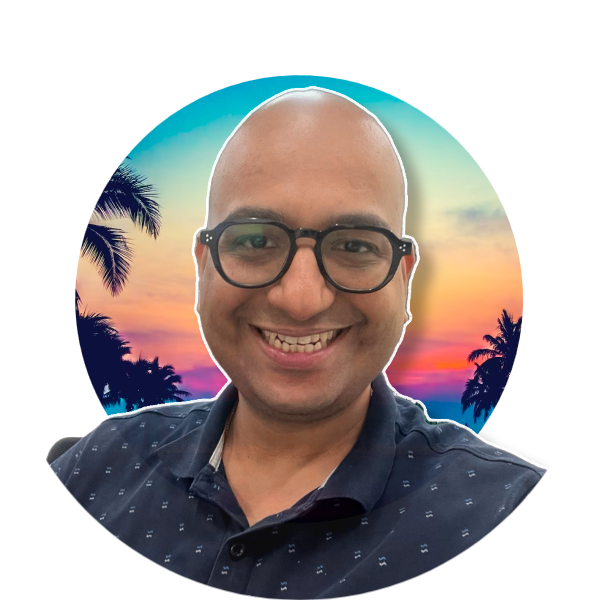
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.