Distributed Tracing with OpenTelemetry and Spring Boot 3!
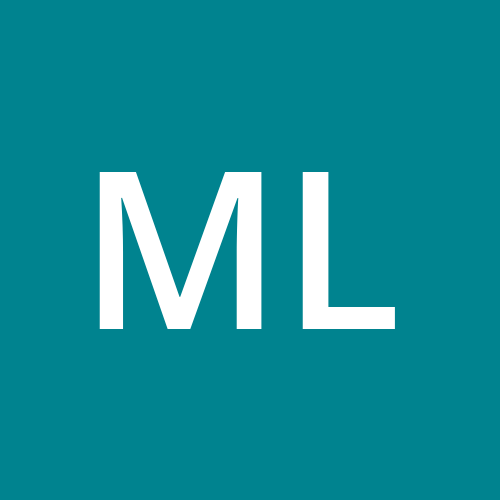

As microservices become the backbone of modern applications, understanding and monitoring the flow of requests across distributed systems is crucial. Distributed tracing helps visualize this flow, diagnose bottlenecks, and debug issues effectively. OpenTelemetry, an open-source observability framework, is a powerful tool to implement distributed tracing in Spring Boot 3 applications.
In this guide, you’ll learn:
What distributed tracing and OpenTelemetry are.
How to integrate OpenTelemetry with a Spring Boot 3 application.
How to visualize trace data using tools like Jaeger or Zipkin.
What Is Distributed Tracing?
Distributed tracing tracks a request as it travels through multiple services in a distributed system.
Key Benefits:
Visualizing end-to-end request flows.
Pinpointing performance bottlenecks.
Identifying errors in complex microservice architectures.
A trace consists of:
Spans: Individual units of work.
Context: Information shared between spans.
What Is OpenTelemetry?
OpenTelemetry (OTel) is an observability framework that standardizes the collection, processing, and exporting of telemetry data (traces, metrics, and logs).
Why Use OpenTelemetry?
Vendor-neutral and supports multiple backends like Jaeger, Zipkin, Prometheus, etc.
Integrates seamlessly with Spring Boot 3.
Provides instrumentation libraries for popular technologies.
Setting Up OpenTelemetry with Spring Boot 3
Follow these steps to implement distributed tracing in your Spring Boot 3 application.
1. Add Dependencies
Include the following dependencies in your pom.xml
:
<dependency>
<groupId>io.opentelemetry.instrumentation</groupId>
<artifactId>opentelemetry-spring-boot-starter</artifactId>
<version>1.27.0</version>
</dependency>
<dependency>
<groupId>io.opentelemetry</groupId>
<artifactId>opentelemetry-exporter-otlp</artifactId>
<version>1.27.0</version>
</dependency>
The
opentelemetry-spring-boot-starter
provides auto-configuration for tracing.The
opentelemetry-exporter-otlp
sends trace data to backends like Jaeger or Zipkin.
2. Configure OpenTelemetry
Add the required configuration in application.properties
:
# Exporter settings
otel.traces.exporter=otlp
otel.exporter.otlp.endpoint=http://localhost:4317
otel.resource.attributes=service.name=MySpringBootApp
# Sampling configuration
otel.traces.sampler=always_on
- Replace
http://localhost:4317
with the endpoint of your tracing backend.
3. Instrument Your Application
With OpenTelemetry, most Spring Boot components are auto-instrumented, but you can add custom spans for detailed tracing.
Example: Adding a Custom Span
import io.opentelemetry.api.trace.Span;
import io.opentelemetry.api.trace.Tracer;
import io.opentelemetry.api.GlobalOpenTelemetry;
@RestController
@RequestMapping("/orders")
public class OrderController {
private final Tracer tracer = GlobalOpenTelemetry.getTracer("OrderService");
@GetMapping("/{id}")
public String getOrder(@PathVariable String id) {
Span span = tracer.spanBuilder("getOrder").startSpan();
try {
// Business logic
span.setAttribute("order.id", id);
return "Order details for ID: " + id;
} finally {
span.end();
}
}
}
4. Visualize Trace Data
Install Jaeger or Zipkin
Run Jaeger in Docker:
docker run -d --name jaeger \
-e COLLECTOR_OTLP_ENABLED=true \
-p 16686:16686 \
-p 4317:4317 \
jaegertracing/all-in-one:latest
- Access Jaeger UI at
http://localhost:16686
.
5. Test the Setup
Run your Spring Boot application and make a request:
curl http://localhost:8080/orders/123
Go to the Jaeger UI to visualize the trace. You should see the spans and their relationships.
Advanced Configurations
Use Context Propagation Across Services
In microservices, trace context (e.g., trace ID, span ID) must be propagated. OpenTelemetry handles this automatically with HTTP headers like:
traceparent
: Carries trace information.tracestate
: Contains vendor-specific trace data.
Integrate Metrics and Logs
You can also collect and export metrics and logs alongside traces using OpenTelemetry libraries for unified observability.
Benefits of Distributed Tracing with OpenTelemetry
Vendor-Neutral: Flexibility to choose observability backends.
Reduced Complexity: Auto-instrumentation simplifies setup.
End-to-End Visibility: Identify bottlenecks and failures across services.
Conclusion
Distributed tracing is essential for monitoring modern microservices. With OpenTelemetry and Spring Boot 3, implementing tracing is both straightforward and effective. By visualizing traces in tools like Jaeger or Zipkin, you can gain deep insights into your application's behavior and resolve issues faster.
Start integrating OpenTelemetry in your Spring Boot applications today and take your observability to the next level!
More such articles:
https://www.youtube.com/@maheshwarligade
Subscribe to my newsletter
Read articles from Maheshwar Ligade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
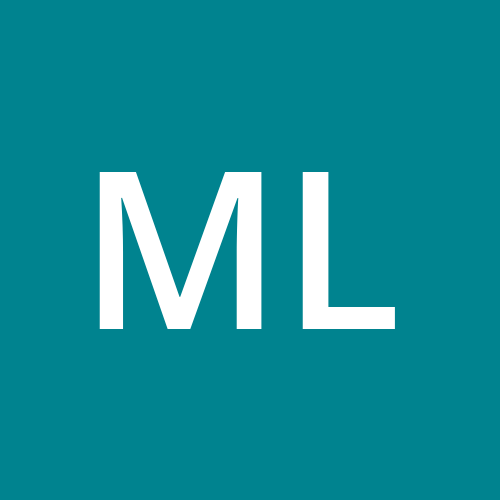
Maheshwar Ligade
Maheshwar Ligade
Learner, Love to make things simple, Full Stack Developer, StackOverflower, Passionate about using machine learning, deep learning and AI