Variables in JavaScript: A Beginner's Guide
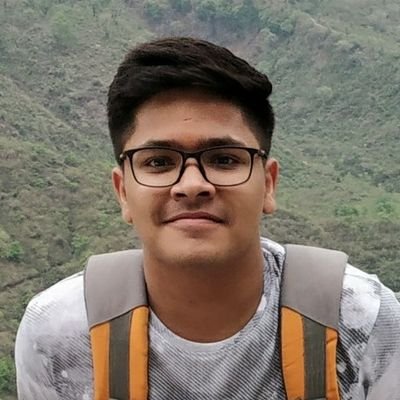

Hey folks, variables are used in every programming language for storing different values and making our tasks easy. They are like containers that can store values of different types. There are some naming conventions we must follow when declaring our variables. Let’s dive into it.
What is a variable?
A variable is a named reference to a memory location that stores a value. They allow us to manage and manipulate data dynamically within our programs. Depending on the keyword used to declare them (var
, let
, or const
), variables have specific scoping rules and behaviors. Variables are crucial for storing data like strings, numbers, objects, or even functions and are fundamental to building any dynamic application.
How Variables Enhance Your Code: When and Why to Use Them
They are essential for creating flexible and reusable programs. They let you store information that can change, such as user inputs or results of calculations. For example, instead of writing "price = 100"
multiple times, you store price
in a variable and update or reuse it as needed. Use variables whenever you need to handle values that might change.
// Without variables:
console.log("The price is $100");
console.log("If you buy two, the total is $200");
// With variables:
let price = 100; // Store the price in a variable
console.log(`The price is $${price}`);
console.log(`If you buy two, the total is $${price * 2}`); // price is 200 as initial value is multiplied by 2
// Updating the price:
price = 150; // Change the value only once
console.log(`The updated price is $${price}`);
The difference between var
and let
Both var
and let
declare variables, but they differ significantly in behavior:
var:
Has function scope, meaning it’s accessible throughout the entire function in which it’s declared, or globally if outside a function.
Does not respect block scope (e.g., within if or for blocks), you can access it through out the program.
NOTE:var
is function-scoped, not block-scoped.if(true){ var insider = 5 } console.log(insider); // 5
Allows redeclaration within the same scope.
var car = "Delorean" var car = "BumbleBee" console.log(car) // BumbleBee
NOTE: Avoid redeclaring variables in the same scope to prevent confusion for your future self.
Hoisted to the top of its scope but initialized with undefined.
let:
Has block scope, meaning it’s restricted to the block in which it’s declared.
if(true){ let insider = 5 } console.log(insider); // undefined
Cannot be redeclared within the same scope.
let car = "Delorean" let car = "BumbleBee" // SyntaxError: Identifier 'car' has already been declared
Hoisted but not initialized, leading to a ReferenceError if accessed before declaration (Temporal Dead Zone).
console.log(name); // ReferenceError: Cannot access 'name' before initialization let name = "Marty McFly";
Choosing the Right Tool: Why let
is Preferred
let
is generally preferred over var
in modern JavaScript because of its predictable scoping behavior and reduced likelihood of bugs. The block-scoping of let
makes it safer and more consistent in handling variables, especially in complex codebases.
What is const
and why should we use it?
const
is used to declare variables whose value cannot be changed or reassigned after being set. However, if the value is an object or an array, you can still change what's inside it (like adding or changing properties or elements). This is because only the reference to the object is fixed, not the content inside it.
A
const
variable must be initialized at the time of declaration.Has block scope.
It’s ideal for values that shouldn’t change during program execution, like configuration settings or constants.
const pi = 3.14159;
pi = 4 // TypeError: Assignment to constant variable.
const
locks the "container" (reference), but not the items inside the container if it's an object or array.
const obj = {
charName = "Marty McFly"
}
obj.charName = "Linda McFly"; // Allowed (mutating object property)
obj = {}; // TypeError (reassignment)
Naming Conventions for Variables
Using consistent and meaningful variable names enhances the readability and maintainability of your code. Here are some recommended conventions to follow:
Allowed Characters
Names should only contain letters, digits,
$
, and_
.- ✅
fluxCapacitor
,$plutoniumLevel
,_88mph
- ✅
Limitation: Symbols like
-
or@
and starting a name with a digit are invalid.- 🚫
flux-capacitor
,@timeMachine
,2ndTimeline
- 🚫
Stick to universally compatible characters to avoid syntax errors across different environments.
Readable and Descriptive Names
Choose names that clearly indicate their purpose.
✅
deloreanSpeed
,timeTravelDestination
🚫
d
,ttd
(What in the space-time continuum does that mean?)
Invest time in crafting meaningful names that communicate intent they are your friends.
Reserved Words
Don’t mess with reserved keywords like
return
orclass
.✅
returnTo1985
,className
🚫
let = 1.21
,class = "DeLorean"
Case Sensitivity
Variable names are case-sensitive, don’t confuse them.
✅
doc
≠Doc
≠DOC
🚫 Using similar names with different cases is like creating a time paradox.
Conclusion
At the end of the day, coding with variables is a bit like time traveling—small changes today can have big impacts down the line. Naming your variables clearly, using proper scope, and choosing the right declaration style will help your code stay smooth, just like a hoverboard ride through the years.
So remember, use let
and const
wisely, avoid re-declaring, and always keep your variable names crystal clear to avoid creating any unexpected time paradoxes in your code!
Subscribe to my newsletter
Read articles from Pranav Goel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
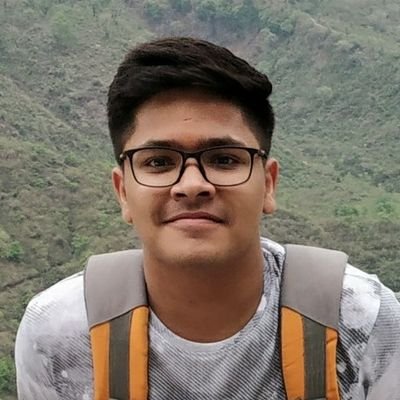
Pranav Goel
Pranav Goel
Web Developer | Open Source | Student