Understanding CRUD Operations
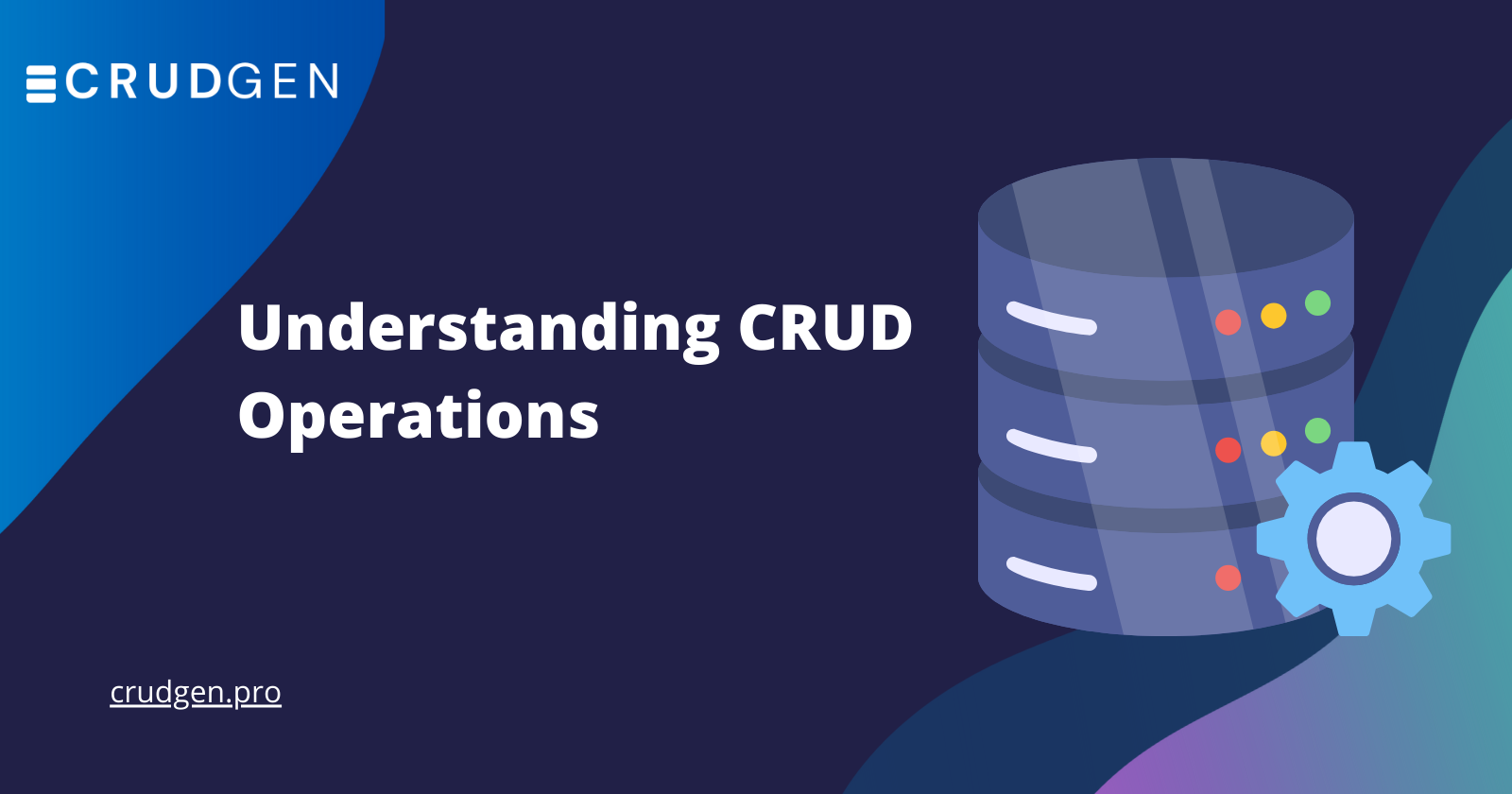
CRUD operations are fundamental to the functioning of modern software applications, particularly those that involve persistent data storage. These operations—Create, Read, Update, and Delete—form the backbone of data manipulation in various programming contexts, including databases and web applications. This article aims to provide an in-depth exploration of CRUD operations, their significance, and practical implementation across different technologies.
CRUD is an acronym that encapsulates the four essential functions that a software application must perform when interacting with data. Each operation corresponds to a specific action that users or systems can take on data stored in a database or similar storage solution.
Create: This operation involves adding records or data entries into a database. For example, when a user signs up for an account, their information is created and stored in the user database.
Read: This operation allows users to retrieve and view existing data. Reading data is crucial for applications that need to display information, such as user profiles or product listings.
Update: This operation enables users to modify existing records. For instance, if a user changes their email address, the update operation will alter the relevant entry in the database.
Delete: This operation removes records from the database. If a user decides to delete their account, the delete operation will ensure that their information is removed from the system.
Understanding these operations is vital for developers, as they form the basis for data management in most applications.
The Importance of CRUD in Software Development
CRUD operations are not just technical necessities; they play a crucial role in enhancing user experience and application functionality. Here are some reasons why CRUD operations are essential in software development:
Data Management
CRUD operations provide a structured approach to managing data. By defining clear actions for data manipulation, developers can create applications that are easier to maintain and extend. This structure also helps in ensuring data integrity and consistency.
User Interaction
Applications that implement CRUD operations can offer a more interactive and dynamic user experience. Users can create new entries, view existing data, make updates, and delete unwanted information—all of which contribute to a more engaging interface.
API Development
In the context of web development, CRUD operations are integral to RESTful APIs. These APIs rely on HTTP methods to perform CRUD actions, allowing different systems to communicate and exchange data seamlessly. For instance, a RESTful API might use POST for creating resources, GET for reading, PUT or PATCH for updating, and DELETE for removing resources.
Scalability
Applications designed with CRUD operations in mind can scale more effectively. As the volume of data grows, the structured approach to data manipulation allows for better performance tuning and optimisation, ensuring that applications remain responsive under increasing loads.
Breaking Down Each CRUD Operation
Create Operation
The Create operation is the first step in data management, allowing users to add new entries to a database. This operation is typically implemented using the SQL INSERT statement or through API endpoints that accept POST requests.
How It Works
When a user submits a form to create a new record, the application processes the input data and sends a request to the server to insert this data into the relevant database table. Here’s an example of how this might look in SQL:
INSERT INTO users (username, email, password) VALUES ('john_doe', '
john@example.com
', 'securepassword');
In a RESTful API context, a POST request might look like this:
POST /api/users Content-Type: application/json { "username": "john_doe", "email": "
john@example.com
", "password": "securepassword" }
Read Operation
The Read operation is essential for retrieving data from a database. This operation is commonly executed using the SQL SELECT statement or through API endpoints that accept GET requests.
How It Works
When a user wants to view data, the application sends a request to the server to read the relevant records. For example, to retrieve user information, the SQL statement might look like this:
SELECT * FROM users WHERE username = 'john_doe';
In a RESTful API, a GET request would resemble:
GET /api/users/john_doe
Update Operation
The Update operation allows users to modify existing records. This operation is implemented using the SQL UPDATE statement or through API endpoints that accept PUT or PATCH requests.
How It Works
When a user updates their information, the application sends a request to the server to change the relevant data. For example, to update a user's email address, the SQL statement could be:
UPDATE users SET email = '
new_email@example.com
' WHERE username = 'john_doe';
In a RESTful API context, a PATCH request might look like this:
PATCH /api/users/john_doe Content-Type: application/json { "email": "
new_email@example.com
" }
Delete Operation
The Delete operation is responsible for removing records from a database. This operation is typically executed using the SQL DELETE statement or through API endpoints that accept DELETE requests.
How It Works
When a user decides to delete their account, the application sends a request to the server to remove the relevant record. The SQL statement might look like:
DELETE FROM users WHERE username = 'john_doe';
In a RESTful API, a DELETE request would appear as follows:
DELETE /api/users/john_doe
Implementing CRUD Operations in Different Technologies
CRUD operations can be implemented across various programming languages and frameworks. Here’s a brief overview of how CRUD can be executed in some popular technologies.
CRUD in SQL
SQL databases are the most common environments for implementing CRUD operations. Here’s a summary of how each operation is executed:
Operation SQL Command Create INSERT Read SELECT Update UPDATE Delete DELETE CRUD in Node.js
Node.js applications often use frameworks like Express to implement CRUD operations. Below is an example of how CRUD operations can be defined in a simple Node.js application:
const express = require('express'); const app = express(); const bodyParser = require('body-parser'); app.use(bodyParser.json()); let users = [];
// Create
app.post
('/users', (req, res) => { const user = req.body; users.push(user); res.status(201).send(user); });
// Read
app.get('/users', (req, res) => { res.send(users); });
// Update
app.put('/users/:username', (req, res) => { const username = req.params.username; const updatedUser = req.body; users =
users.map
(user => (user.username === username ? updatedUser : user)); res.send(updatedUser); });
// Delete
app.delete('/users/:username', (req, res) => { const username = req.params.username; users = users.filter(user => user.username !== username); res.status(204).send(); });
Best Practices for CRUD Operations
Implementing CRUD operations effectively requires adherence to best practices that enhance performance, security, and maintainability. Here are some recommendations:
Use Prepared Statements
To prevent SQL injection attacks, always use prepared statements or parameterised queries. This approach ensures that user input is treated as data rather than executable code.
Validate User Input
Before processing any CRUD operation, validate user input to ensure it meets the required format and constraints. This step helps avoid errors and ensures data integrity.
Implement Error Handling
Graceful error handling is essential for a good user experience. Ensure that your application can handle errors gracefully and provide meaningful feedback to users.
Optimise Database Queries
Optimise your database queries to improve performance. Use indexing where appropriate and avoid unnecessary complexity in your SQL statements.
Secure APIs
When exposing CRUD operations through APIs, implement authentication and authorisation mechanisms to protect sensitive data and ensure that only authorised users can perform actions.
Conclusion
CRUD operations are a cornerstone of modern software development, enabling efficient data management and user interaction. Understanding how to implement these operations effectively is crucial for developers looking to create robust applications. By adhering to best practices and leveraging appropriate technologies, developers can ensure that their applications are secure, performant, and user-friendly.
In summary, CRUD operations encompass the essential functions of creating, reading, updating, and deleting data. Mastery of these operations is vital for any developer aiming to build effective applications that interact with persistent data storage. Whether through SQL databases, RESTful APIs, or various programming languages, CRUD remains a fundamental concept that underpins the functionality of countless software solutions.
Subscribe to my newsletter
Read articles from Kim Majali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Kim Majali
Kim Majali
CEO at EITO & Web AI Ltd., 16 years entrepreneur, 22 years of web development experience (full-stack developer), 18 years of digital marketing experience, 6 years cloud architect experience.