🔥 JavaScript Handbook
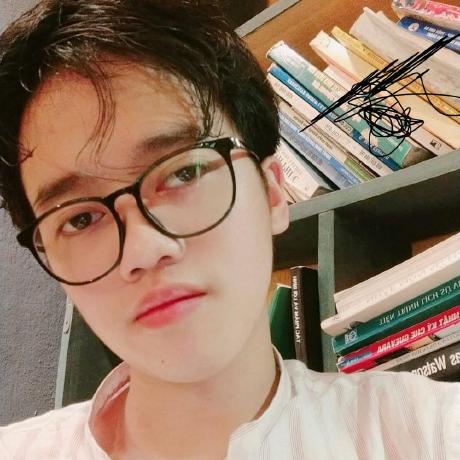
Table of contents
- Advanced JavaScript cheatsheet
- JavaScript Under the Hood
- Shallow Copy vs Deep Copy
- Hoisting in JavaScript with let and const - and how it differs from var
- Closure
- JavaScript Visualized: Event Loop
- JavaScript Visualized: Prototypal Inheritance
- JavaScript Visualized: Promise and Async/Await
- Map
- Symbols for hidden values
- JavaScript Memory Leaks
- AbortController
- Advanced OOP Concepts
- this Keyword
- Advanced JavaScript Functions To Improve Code Quality
- 8 Modern Javascript Reactive Patterns
- The Regular Expressions
- Stop Using .forEach: Transform your code with for…of Loop
- Making Good Use of Console
- Structured Cloning With structuredClone()
- Self-Invoking Functions
- Tagged Template Literals
- 12 Javascript Web APIs to build Futuristic Websites You didn’t know
- The Mysteries of bind(), call(), and apply()
- Explore the hidden power of Javascript Generators
- Advanced Uses Of Promises
- Conclusion
- References

When we take our first steps into the wonderful world of programming, we see for ourselves what it does for millions of people. Thanks to programming, the lives of so many people are made easier just by pressing a few keys on their devices. (This is magic.)
Programming is another kind of superpower, but as Uncle Ben said to his nephew with great power comes great responsibility. In the world of programming, our greatest responsibility is to ensure that the code we write is both easily testable and will remain maintainable over time.
In this blog post, we will discuss some recently added features to the language and some advanced concepts that you could use in your daily coding journey to improve your Javascript coding.
Advanced JavaScript cheatsheet
Let’s get down to the root causes that lie at the heart of JavaScript. We will be dealing with some of the more advanced and less well-known sides of JavaScript, such as functions and the this
keyword, as often happens in the JavaScript programming language. We will go into the more interesting and complex parts of JavaScript using some practical examples and an in-depth exploration of the topics. Consequently, we will develop a very comprehensive guide designed to be useful to all levels of JS developers.
JavaScript Under the Hood
Every browser has a JavaScript engine. The most popular engine is Google’s V8 engine, which powers Google Chrome and Node.js. Of course, all other browsers have their own JavaScript engines.
A JavaScript engine will always consist of a Call Stack and a Memory Heap and will run on one thread. The JavaScript engine itself runs in several threads (processes) that do different things: compressors, garbage collection, etc.
Compilation, Interpretation, and Just-In-Time Compilation
Computers fundamentally understand machine code, which can be represented as assembly language. Therefore, all software must eventually be converted into a format that a computer can execute immediately. These are three common approaches to this conversion process:
compilation
interpretation
Just-in-Time compilation
Compilation (Ahead of time compilation)
In this method, all the code is converted to machine language at once and then written to a file in assembly so that the computer can run the software, which can happen even a long time after the file was created.
Interpretation
In this method, the Interpreter goes through the code in an initial pass and then executes it line by line. During the runtime, while running line by line, the code is also compiled into machine language.
In the past, JavaScript was primarily an interpreted language, which led to certain performance limitations. As web developers discovered, interpreting JavaScript line by line made it challenging to implement optimizations effectively. This is partly due to JavaScript’s dynamic nature, where data types can change at runtime. For example, if a variable is constantly used as a Boolean, an interpreter may still allocate more memory than necessary because it can not make assumptions about the variable’s type.
Over time, advances in javascript engines, such as just-in-time complication (JIT), have been introduced to address these inefficiencies, resulting in significant performance improvements.
Just-in-time compilation
In this approach, the entire code is translated into machine language in a single step and executed immediately afterward. During the conversion process, no intermediate files are created; instead, the code is directly converted to machine language and executed without delay. This method streamlines the execution process by combining the compilation and execution steps, thereby enhancing the overall efficiency of code processing and execution.
Today, JavaScript employs just-in-time (JIT) compilation, which combines the benefits of both interpretation and traditional compilation. This real-time compilation process is faster than interpretation because it eliminates the overhead associated with interpreting code line by line. JIT compilation also offers advantages over ahead-of-time compilation, as it can perform optimizations based on runtime information that would not be available during a traditional compilation process. As a result, code executed using JIT compilation is often faster and more efficient than code executed through either interpretation or ahead-of-time compilation alone.
Just-in-time compilation in JavaScript
Now we will talk about how just-in-time compilation is performed in real time in JavaScript.
Parsing
In the first step of processing JavaScript code, parsing is performed, which involves reading the code and creating an Abstract Syntax Tree(AST). During this process, the code is broken down into meaningful components according to JavaScript’s syntax, such as significant keywords like const, function, or new. These components are then organized into a structured tree. This stage also includes checking the code for any syntax errors. The resulting AST serves as the basis for converting the JavaScript source code into machine code, which can be executed by the JavaScript engine in subsequent stages.
Say we have a simple primitive variable like this:
So this is what the AST looks like for this single line of code. Besides the simple things, such as the name, the value, and the type, there is a lot more data, which is not our concern. Understanding the exact structure of the AST is not crucial, and there is no need to delve into its details. This information is provided merely for general knowledge purposes.
Important note: Despite both being referred to as “trees“, the AST and the DOM Tree are entirely separate concepts and should not be confused due to the shared terminology. The AST is a representation of all the code inside the JavaScript engine.
Compilation and Execution
The subsequent stage is the compilation phase, where the generated AST is converted into machine code. Following this, the machine code is executed immediately, as modern Javascript engines employ JIT compilation, as previously mentioned. We will soon discuss the execution stage occurring within the call stack. However, the story doesn’t end there. Modern JavaScript engines, such as the V8 engine, employ sophisticated optimization strategies. Initially, they generate an inefficient version of the machine code to enable prompt execution, and then, in the background, the code undergoes an optimization process. After each optimization, the code is recompiled in the optimized version, with the previous code being replaced without disrupting or halting the execution. This process is what makes modern engines so fast.
These processes of compression, compilation, or optimization occur within specialized threads (processes) inside the engine, which are inaccessible to us. This thread operates independently from the main thread where the call stack runs and our code executes.
While different engines may implement this process in various ways, the fundamentals remain the same for modern runtime compilation.
The JavaScript runtime environment
Next, we will discuss the JavaScript runtime environment in the browser, which is crucial to understand.
Picture the runtime environment as a container encompassing everything needed to run JavaScript within the browser. At the core of the runtime environment lies the JavaScript engines, which we explored in the previous environment. Without an engine, there is no runtime, and without a runtime, JavaScript cannot function.
However, the engine alone is insufficient. For it to operate correctly within the browser, access to Web APIs is necessary. Web APIs encompass everything related to the DOM, timers, and numerous other APIs that we can access in the browser.
For instance, console.log, which we frequently use, is not a part of the language itself but an implementation of an API that JavaScript utilizes. Web APIs are interfaces that offer functionality to the engine in the browser environment but are not part of the JavaScript language itself. JavaScript accesses these APIs through the global Window object in the browser.
As mentioned earlier, Web APIs are not part of the engine, but they are components of the runtime environment. The runtime environment, as discussed, is a container that holds everything JavaScript needs to function in the browser. The JavaScript runtime also comprises the Callback Queue, a data structure containing all the callback functions that are ready to execute. For example, when attaching an Event Listener to a DOM element like a button, it responds to a click event. We will see this in action later.
The function passed to the event listener is a Callback function. When the event occurs, such as when the button is clicked, the callback function is invoked. What happens is that the callback function is first moved to the Callbacks Queue, and once the Call Stack is empty, the Callback Function transitions to the Call Stack for execution.
This occurs through the so-called Event Loop, which takes Callback Functions from the Callback Queue and places them onto the Call Stack for execution. In this manner, the JavaScript runtime environment implements the Nonblocking Concurrency Model, a non-blocking parallel model. Although JavaScript runs on a single thread, it employs multiple processes using other components in the runtime environment. We will discuss this further later and explain why it makes the entire JavaScript runtime non-blocking.
We have discussed how JavaScript operates in the browser, but it is important to remember that JavaScript can also exist and run outside of browsers, such as in Node.js.
The Node.js runtime environment is quite similar to the browser’s runtime environment. However, since there is no browser, Web APIs are not present, and there is no browser to provide them. Instead, we have C++ Bindings and a Thread Pool. These details are not our primary concern. The essential point to remember is that different Javascript runtimes exist.
The Execution Context
So, how is JavaScript code executed? We will now delve deeper into the topic, starting with the Execution Context. While the Execution Context is an abstract concept, it can be defined as an environment where segments of JavaScript code are executed. It serves as a container that holds all the necessary information for executing JavaScript code, such as local variables or arguments passed to functions. There will always be only one Execution Context in operation, and the default Execution Context is the Global Execution Context, where the top-level code is executed.
Once the top-level code execution is completed, functions begin to execute. For each function call, a new Execution Context is created, containing all the information required to run that particular function. The same principle applies to methods, as they are merely functions attached to keys within objects.
After all functions have finished executing, the engine waits for the arrival of the Callback Function to run them, such as those linked to Click Events. As a reminder, it is the Event Loop that supplies these Callback Functions from the Callback Queue when the Call Stack is emptied.
The Components of the Execution Context
The first thing we have in the Execution Context is the Variable Environment.
In this environment, all variables and function declarations are stored. Additionally, a special “arguments“ object is present. As the name suggests, the object contains all the arguments passed to the function currently in the Execution Context, provided the Execution Context belongs to a function. As mentioned earlier, each function has its own Execution Context, so all variables declared by the function will be located in the function’s variable environment. Furthermore, a function can access variables outside of itself due to the Scope Chain. In short, the Scope Chain contains references to all external variables accessible by the function. Lastly, each Execution Context also receives a special variable called the “this” keyword.
In summary, the Execution Context comprises the variable environment, the Scope Chain, and the ‘this‘ Keyword. These components are created during the Creation Phase, which occurs before the execution.
It’s worth mentioning that the Arrow Function does not possess its own ‘arguments‘ object or ‘this‘ Keyword. Rather, they make use of the ‘arguments‘ object and ‘this‘ keyword from their nearest function or, alternatively, refer to the Global Scope.
The Execution Context in Practice
Let’s look at an example to understand the topic better:
Initially, a Global Execution Context is created for the Top-level code, which is any code not contained within a function. So, at first, only this code is executed. This makes sense because functions only run when they are executed or triggered. In this example, the ‘first‘ variable is in the TOP Level code, so it’s executed in the Global Execution Context. It’s known that the value of ‘first’ is 5, ‘second’ and ‘third’ are functions, and the value of ‘fourth’ is still unknown.
In line 14, to get the value of ‘fourth’, the ‘second’ function is activated, and its Execution Context is created. Here, the value of ‘a’ is known to be 1, but the value of ‘b’ is still unknown, as it must be obtained from the ‘third’ function. So, the ‘third’ function runs, and its Execution Context is created with the value of ‘c’ being 3 and its ‘arguments’ object containing 1 and 2, which were passed when the function was executed. Now, ‘third’ returns 6 (1 + 2 + 3), we return to the Execution Context of ‘second’, and the value of ‘b’ is now 6. Finally, ‘second’ returns 7 (6 + 1), and the value of ‘fourth’ is determined as 7 in the Global Execution Context.
In this small and seemingly simple example, we observed a rather complex process. This raises the question of how the JavaScript engine knows when to execute functions and which Execution Context should be the current one, especially in more intricate code examples. This is where the Call Stack comes into play.
The Call Stack
As previously mentioned, the JavaScript runtime includes the JavaScript engine, which itself comprises the Memory Heap and the Call Stack. So, what exactly is the Call Stack?
The Call Stack is where Execution Contexts are stacked on top of one another to keep track of the program’s execution. At any given moment, the topmost Execution Context is the one being executed, following the Last-In-First-Out (LIFO) method.
Referring back to the previous example, the Global Execution Context initially occupies the Call Stack. When the ‘second’ function is called, it moves to the top of the Call Stack, followed by the ‘third’ function when it is called. Once the ‘third’ function finishes and returns a value, it is removed from the Call Stack. Similarly, when the ‘second’ function concludes, it is also removed, leaving the Global Execution Context with all the values it was waiting for.
It’s crucial to understand that while any Execution Context is running and positioned at the top of the Call Stack, all other Execution Contexts are suspended and waiting for the current one to be completed. This occurs because JavaScript operates on a single thread, requiring operations to be performed sequentially rather than in parallel. The Call Stack is an essential component of the JavaScript engine itself and not a separate part of the runtime environment.
In this way, the Call Stack maintains the execution order of the Execution Context, ensuring that the sequence of execution is always preserved.
Shallow Copy vs Deep Copy
There are two ways to clone objects in JavaScript:
Shallow copy: means that only the first level of the object is copied. Deeper levels are referenced.
Deep copy: means that all levels of the object are copied. This is a true copy of the object.
Shallow Copy
A shallow copy can be achieved by using the spread operator (…) or using Object.assign()
:
const obj = { name: 'Version 1', additionalInfo: { version: 1 } };
const shallowCopy1 = { ...obj };
const shallowCopy2 = Object.assign({}, obj);
const shallowCopy3 = obj;
shallowCopy1.name = 'Version 2';
shallowCopy1.additionalInfo.version = 2;
shallowCopy2.name = 'Version 2';
shallowCopy2.additionalInfo.version = 2;
console.log(obj); // { name: 'Version 1', additionalInfo: { version: 2 } }
console.log(shallowCopy1); // { name: 'Version 2', additionalInfo: { version: 2 } }
console.log(shallowCopy2); // { name: 'Version 2', additionalInfo: { version: 2 } }
shallowCopy3.name = "Version 3";
console.log(obj); // { name: 'Version 3', additionalInfo: { version: 2 } }
console.log(shallowCopy3); // { name: 'Version 3', additionalInfo: { version: 2 } }
As you can see in this code snippet:
After updating a property in the first level of the cloned object, the original property is not updated.
After updating a property on a deeper level, the original property is also updated. This happens because, in this case, deeper levels are referenced, not copied.
Deep Copy
A deep copy can be achieved by using JSON.parse()
+ JSON.stringify()
:
const obj = { name: 'Version 1', additionalInfo: { version: 1 } };
const deepCopy = JSON.parse(JSON.stringify(obj));
deepCopy.name = 'Version 2';
deepCopy.additionalInfo.version = 2;
console.log(obj); // { name: 'Version 1', additionalInfo: { version: 1 } }
console.log(deepCopy); // { name: 'Version 2', additionalInfo: { version: 2 } }
As you can see in this code snippet:
After updating the property in the first level of the cloned objects, the original object property is not updated.
After updating the property on a deeper level, the original property is not updated. This happens, because, in this case, deeper levels are also copied.
Performance
For obvious reasons, shallow copies are a lot faster than deep copies. But that doesn’t mean that you should always use a shallow copy because sometimes you will also need a copy of the nested objects. So, which option should I use?
if the depth of your object is equal to one, use a shallow copy.
if the depth of your object is bigger than one, use a deep copy.
Hoisting in JavaScript with let
and const
- and how it differs from var
I used to think that hoisting only happened with the variables declared with var
. But recently, I learned that it also happens with the variables declared with let
and const
.
How Hoisting Works With var
in Javascript.
Here’s how hoisting works on variables declared with var
:
console.log(number)
// undefined
var number = 10
console.log(number)
// 10
The number variable is hoisted to the top of the global scope. This makes it possible to access the variable before the line it was declared, without errors.
But what you will notice here is that only the variable declaration (var number
) is hoisted – the initialization (= 10
) isn't. So when you try to access number
before it is declared, you get the default initialization that happens with var, which is undefined
.
How Hoisting Works With let/const in JavaScript
If you try to do the same thing with let
and const
, here is what happens:
console.log(number)
let number = 10
// or const number = 10
console.log(number)
You get an error that says: ReferenceError: Cannot access ‘number’ before initialization.
So you can access the variable declared with var before the declaration without errors, but you can’t do the same thing with let and const.
That is why I had always thought that hoisting only happens with var, it doesn’t happen with let and const.
But as I said, I learned recently that variables declared with let or const are also hoisted. Let me explain.
Take a look at this example:
console.log(number2)
let number = 10
I logged a variable called number2 to the console, and I declared and initialized a variable called number
.
Running this code produces this error: ReferenceError: number2 is not defined
What do you notice between the previous error and this error? The previous error says ReferenceError: Cannot access 'number' before initialization, while this new error says ReferenceError: number2 is not defined.
Here's the difference. The former says "cannot access before initialization," while the latter says "is not defined".
What the latter means is that JavaScript has no idea about the number2
because it’s not defined - and indeed we didn’t define it. We only defined number
.
But the former doesn't say "is not defined", instead, it says, "cannot access before initialization". Here's the code again:
console.log(number)
// ReferenceError: Cannot access 'number' before initialization
let number = 10
console.log(number)
That means JavaScript knows about the number variable. How does it know? Because number
is hoisted to the top of the global scope.
But why does an error occur? Well, this clarifies the difference between the hoisting behavior with var and let/const.
Variables declared with let
or const
are hoisted WITHOUT a default initialization. So accessing them before the line they were declared throws ReferenceError: Cannot access 'variable' before initialization.
But variables declared with var
are hoisted WITH a default initialization of undefined. So accessing them before the line they were declared returns undefined
.
There is a name for the period during execution where let/const variables are hoisted but not accessible: It’s called the Temporal Dead Zone.
Closure
In JavaScript, functions can be nested within other functions, creating a hierarchy of scopes. Each function has its own local scope, and nested functions have access to the variables declared in their scope as well as the scopes of their outer functions. This concept is known as “nested function scope.“.
Let’s explore nested function scope with an example:
function outerFunction() {
var outerVariable = "I am from the outer function";
function innerFunction() {
var innerVariable = "I am from the inner function";
console.log("Inside innerFunction:", outerVariable);
// Accesses outerVariable
outerVariable = "Modified in innerFunction";
// Modifies outerVariable
console.log("Inside innerFunction:", innerVariable);
// Accesses innerVariable
}
innerFunction(); // Calls innerFunction
console.log("After calling innerFunction:", outerVariable);
// Shows modified outerVariable
// Uncommenting the next line will throw an error since
// innerVariable is not accessible here
// console.log("Outside innerFunction:", innerVariable);
}
outerFunction();
A closure is a feature that allows a function to retain access to variables from its outer(enclosing) scope even when the outer function has finished executing. This means that the inner function “closes over“ the variables it references, and they remain accessible when the outer function has completed its execution. Closures are a powerful and fundamental concept in JavaScript. Here is a detailed example to illustrate closures:
// Outer function that returns an inner function
function outerFunction(outerVariable) {
// Inner function defined inside the outer function
function innerFunction(innerVariable) {
// Accessing variables from both the outer and inner functions
console.log("Outer variable:", outerVariable);
console.log("Inner variable:", innerVariable);
}
// Returning the inner function (creating a closure)
return innerFunction;
}
// Creating closures by calling outerFunction with different arguments
var cls1= outerFunction("cls 1");
var cls2 = outerFunction("cls 2");
// Invoking the inner functions created by the closures
cls("Inner 1");// Output: Outer variable: cls 1, Inner variable: Inner 1
cls2("Inner 2");// Output: Outer variable: cls 2, Inner variable: Inner 2
JavaScript Visualized: Event Loop
Oh, boy, the event loop. It’s one of those things that every JavaScript developer has to deal with in one way or another, but it can be a bit confusing to understand at first. I am a visual learner, so I thought I would try to help you by explaining it in a visual way through low-res gifs because it’s 2024 and gifs are somehow still pixelated and blurry.
But first, what is the event loop, and why should you care?
JavaScript is single-threaded: only one task can run at a time. Usually, that is no big deal, but now imagine you are running a task that takes 30 seconds. During that task, we wait for 30 seconds before anything else happens (JavaScript runs on the browser’s main thread by default, so the Ui is stuck). It’s 2024, no one wants slow, unresponsive websites.
Luckily, the browser gives us some features that the JavaScript itself doesn’t provide: a Web API. This includes a DOM API, setTimeout, HTTP requests, and so on. This can help us create some sync and non-blocking behavior.
For more details, refer to this article.
JavaScript Visualized: Prototypal Inheritance
Ever wondered why we can use built-in methods such as .length
, .split()
, .join()
on our strings, arrays, or objects? We never explicitly specified them, where do they come from? Don’t say “It’s JavaScript lol no one knows, it’s magic“, it’s actually because of something called prototypal inheritance. It’s pretty awesome, and you use it more often than you realize.
You often have to create many objects of the same type. Say we have a website where people can browse dogs.
For every dog, we need objects that represent that dog! Instead of writing a new object each time, we will use a constructor function (I know what you are thinking, we will cover ES6 classes later on) from which we can create Dog instances using the new keyword(this post isn’t really about explaining constructor functions though, so I won't talk much about that)
Every dog has a name, a breed, a color, and a function to bark.
Check out this article to explore amazing things about prototypal inheritance.
Difference between prototype and __proto__
The prototype
property is an attribute associated with constructor functions in JavaScript. Constructor functions are used to create objects in JavaScript. When you define a constructor function, you can also attach properties and methods to its prototype
property. These properties and methods then become accessible to all instances of objects created from that constructor. Thus, the prototype
property serves as the common repository for methods and properties that are shared among instances.
Consider the following code snippet:
// Constructor function
function Person(name) {
this.name = name;
}
// Adding a method to the prototype
Person.prototype.sayHello = function() {
console.log(`Hello, my name is ${this.name}.`);
};
// Creating instances
const person1 = new Person("Haider Wain");
const person2 = new Person("Omer Asif");
// Calling the shared method
person1.sayHello(); // Output: Hello, my name is Haider Wain.
person2.sayHello(); // Output: Hello, my name is Omer Asif.
In this example, we have a constructor function named Person
. By extending the Person.prototype
with a method like sayHello
, we are adding this method to the prototype chain of all Person
instances. This allows each instance of Person
to access and utilize the shared method. Instead, each instance has its own copy of the method.
On the other hand, the __proto__
property, often pronounced as "dunder proto," exists in every JavaScript object. In JavaScript, everything, except primitive types, can be treated as an object. Each of these objects has a prototype, which serves as a reference to another object. The __proto__
property is simply a reference to this prototype object. The prototype object is used as a fallback source for properties and methods when the original object doesn’t possess them. By default, when you create an object, its prototype is set to Object.prototype
.
When you attempt to access a property or method on an object, JavaScript follows a lookup process to find it. This process involves two main steps:
Object’s Own Properties: JavaScript first checks if the object itself directly possesses the desired property or method. If the property is found within the object, it’s accessed and used directly.
Prototype Chain Lookup: If the property is not found in the object itself, JavaScript looks at the object’s prototype (referenced by the
__proto__
property) and searches for the property there. This process continues recursively up the prototype chain until the property is found or until the lookup reaches theObject.prototype
.
If the property is not found even in the Object.prototype
, JavaScript returns undefined
, indicating that the property does not exist.
JavaScript Visualized: Promise and Async/Await
Have you ever had to deal with JS code that just… didn’t run the way you expected it to? Maybe it seemed like functions got executed at random, unpredictable times, or the execution got delayed. There is a chance you were dealing with a cool new feature that ES6 introduced: Promises!
My curiosity from many years ago has paid off, and my sleepless nights have once given me the time to make some animations. Time to talk about Promises: why would you use them? How do they work “under the hood“, and how can we write them in the most modern ways?
All of those questions will be answered in this article.
Map
In earlier JavaScript, developers often used plain objects to map keys to values. However, this approach has limitations, especially when keys are not strings or symbols. Plain objects can only use strings or symbols as keys, so if you need to map non-primitive objects (like arrays or other objects) to values, it becomes cumbersome and error-prone.
const obj = {};
const key = { id: 1 };
// Trying to use a non-primitive object as a key
obj[key] = 'value';
console.log(obj); // Object automatically converts key to a string: '[object Object]: value'
Advice: Use Map
when you need to map non-primitive objects or when a more robust data structure is required. Unlike plain objects, Map
allows any type of value — primitives and — non-primitives alike — as keys.
const map = new Map();
const key = { id: 1 };
// Using a non-primitive object as a key in a Map
map.set(key, 'value');
console.log(map.get(key)); // 'value'
Why it matters: Map
is a more flexible and predictable way of associating values to any kind of key, whether primitive or non-primitive. It preserves the type and order of keys, unlike plain objects, which convert keys to strings. This leads to more powerful and efficient handling of key-value pairs, especially when working with complex data or when you need fast lookups in larger collections.
Why is JavaScript’s Map better than Object?
JavaScript provides both Map and Object for storing key-value pairs, but Map offers significant advantages in many scenarios.
For more explanation, check out this article.
Use Map when:
Working with dynamic or complex key types
Performing frequent insertions or deletions.
Preserving insertion order
Quickly retrieving the number of entries
Avoiding prototype chain interference.
Use Object when:
Storing simple, static data.
Needing JSON serialization.
Preferring a more concise syntax for defining key-value pairs.
Symbols for hidden values
In JavaScript, objects are typically used to store key-value pairs. However, when you need to add “hidden“ or unique values to an object without risking name collisions with other properties, or you want to keep them somewhat private from external code, using Symbol can be very helpful. Symbols
create unique keys that are not accessible via normal enumeration or accidental property lookup.
const obj = { name: 'Alice' };
const hiddenKey = Symbol('hidden');
obj[hiddenKey] = 'Secret Value';
console.log(obj.name); // 'Alice'
console.log(obj[hiddenKey]); // 'Secret Value'
Advice: Use Symbol
when you want to add non-enumerable, hidden properties to an object. Symbols are not accessible during typical object operations like for…in
loops or Object.keys()
, making them perfect for internal or private data that shouldn’t be exposed accidentally.
const obj = { name: 'Alice' };
const hiddenKey = Symbol('hidden');
obj[hiddenKey] = 'Secret Value';
console.log(Object.keys(obj)); // ['name'] (Symbol keys won't appear)
console.log(Object.getOwnPropertySymbols(obj)); // [Symbol(hidden)] (accessible only if specifically retrieved)
Why it matters: Symbols allow you to safely add unique or “hidden“ properties to objects without worrying about key collisions or exposing internal details to other parts of the codebase. They can be especially useful in libraries or frameworks when you need to store metadata or internal states without affecting or interfering with other properties. This ensures better encapsulation and reduces the risk of accidental overwrites or misuse.
JavaScript Memory Leaks
JavaScript memory Leaks occur when allocated memory is not released after it is no longer needed. This can impact performance and potentially cause crashes. In JavaScript, memory management is handled by an automated garbage collector. The collector frees up memory by reclaiming it from unused objects. Automated management is helpful, but it’s not perfect. Memory leaks can still happen if objects are not properly cleared or released.
Over time, these leaks can slow down the application, reduce performance, or even cause it to crash.
Check out this article to explore how to identify, fix, and prevent these leaks.
AbortController
The fetch
API in JavaScript allows you to make HTTP requests. It is a modern alternative to the old XMLHttpRequest
, which is now mostly outdated.
fetch
works with promises, meaning it returns a promise that tells you when the request is completed, either successfully or with an error.
Example with fetch
:
fetch(url)
.then((response) => response.json()) // Convert response to JSON
.then((data) => console.log(data)) // Log the data
.catch((error) => console.error(error)); // Log any errors
This works fine for basic requests, but sometimes you need to cancel a request that has already started.
Searching for suggestions as you type
You have a search bar, and you want to show suggestions as the user types.
Each time the user types a character, a fetch request is made to get the suggestions based on the input. Here’s how that would look.
fetch(`https://api.example.com/search?q=${searchInput.value}`)
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
The fetch request is called every time the user types, even if the user types quickly.
This led to multiple API requests, which is inefficient because you only careabout the last requests when the user finishes typing.
Solution: Debounce
To fix this, you can use the debounce function, which delays the fetch request until the user stops typing for a specified amount of time (for example, 100ms). This way, you only run one request for the last typed value.
However, there’s still an issue: if the user starts typing right after the debounce is triggered, the request will be called again.
This is better than calling it every time a key is pressed, but we can improve it further.
Solution: AbortController
With AbortController
, you can cancel a request before it finishes. It's built into modern browsers.
AbortController: This object helps us control or cancel requests.
AbortController.signal: A signal you use to tell fetch to cancel the request.
AbortController.abort(): A method that cancels a request.
We have an input field where the user can search for posts. We want to cancel previous requests if a new one is made.
Basic HTML Example:
<!DOCTYPE html>
<html>
<body>
<input id="search" type="text" />
<script>
const search = document.getElementById("search");
let controller = new AbortController();
let signal = controller.signal;
const fetchPost = async (value, signal) => {
try {
const response = await fetch(
`https://jsonplaceholder.typicode.com/posts/${value}`,
{ signal }
);
const data = await response.json();
console.log("Success:", data);
} catch (error) {
if (error.name === "AbortError") {
console.log("Request canceled");
} else {
console.error("Something went wrong");
}
}
};
const onInputChange = () => {
const value = search.value;
if (value) {
controller.abort(); // Cancel the previous request
controller = new AbortController(); // Create a new controller
signal = controller.signal; // Get the new signal
fetchPost(value, signal); // Call the fetch function
}
};
search.oninput = onInputChange; // Trigger the function on input
</script>
</body>
</html>
AbortController
ensures that we cancel any ongoing requests before making a new one, improving performance, and preventing unnecessary API calls.
Advanced OOP Concepts
Object-Oriented Programming (OOP) is a paradigm that uses objects and classes to structure code. In JavaScript, OOP principles can help manage and organize code more efficiently, especially for complex applications.
What is Object-Oriented Programming?
Object-oriented programming is a programming paradigm based on the concept of “objects“, which can contain data (attributes) and code (methods). OOP helps in modeling real-world entities and interactions, making it easier to manage and maintain code.
Key Concepts of OOP:
Encapsulation: Bundling data and methods that operate on the data within one unit (i.e a class)
Abstraction: Hiding the complex implementation details and showing only the essential features of an object.
Inheritance: Mechanism by which one class can inherit the properties and methods of another class.
Polymorphism: The Ability of different objects to respond to the same method in different ways.
JavaScript and OOP
JavaScript is a versatile language that supports OOP concepts through its own syntax and features. Let’s explore how JavaScript implements these features.
Encapsulation
In JavaScript, encapsulation is achieved using classes and objects. A class is a blueprint for creating objects, and an object is an instance of a class
class Person {
// Constructor to initialize object properties
constructor(name, age) {
this.name = name;
this.age = age;
}
// Method to display person's details
greet() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
// Creating an instance of the Person class
const person1 = new Person('Alice', 30);
person1.greet(); // Output: Hello, my name is Alice and I am 30 years old.
In this example, Person
is a class with a constructor and methods. The greet
method encapsulates the behavior of greeting and the name
and age
properties encapsulate the data.
Abstraction
Abstraction is about hiding complex implementation details and showing only the necessary features. In JavaScript, abstraction is achieved by using classes and methods that abstract away complex functionalities.
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
// Abstract method
startEngine() {
console.log('Engine started.');
}
}
class ElectricCar extends Car {
startEngine() {
console.log('Electric engine started silently.');
}
}
const myCar = new ElectricCar('Tesla', 'Model S');
myCar.startEngine(); // Output: Electric engine started silently.
Here, the Car
class provides an abstract method startEngine
. The ElectricCar
subclass provides a specific implementation of startEngine
, hiding the complexity of starting an electric engine.
Inheritance
Inheritance allows one class to inherit properties and methods from another class. In JavaScript, this is achieved by using extends
keyword.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const myDog = new Dog('Rex');
myDog.speak(); // Output: Rex barks.
In this example, Dog
inherits from Animal
and overrides the speak method to provide the specific implementation for dogs.
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. In JavaScript, this is achieved through method overriding and dynamic method dispatch.
class Shape {
draw() {
console.log('Drawing a shape.');
}
}
class Circle extends Shape {
draw() {
console.log('Drawing a circle.');
}
}
class Square extends Shape {
draw() {
console.log('Drawing a square.');
}
}
const shapes = [new Circle(), new Square()];
shapes.forEach(shape => shape.draw());
// Output:
// Drawing a circle.
// Drawing a square.
Here, the draw
method is polymorphic: different shapes provide different implementations of draw
, but they can be used interchangeably.
JavaScript OOP Features and Syntax
JavaScript provides several features to support OOP:
Classes: Introduced in ES6, classes provide a clear syntax for creating objects and handling inheritance.
Constructors: Special methods used to initialize new objects.
Getters and Setters: Special methods for defining how to set and get object properties.
Static methods: Methods that belong to the class rather than any instance of the class.
class Rectangle {
constructor(width, height) {
this.width = width;
this.height = height;
}
// Getter for area
get area() {
return this.width * this.height;
}
// Setter for width
set width(value) {
if (value > 0) {
this._width = value;
} else {
console.log('Width must be positive.');
}
}
// Static method
static describe() {
console.log('A rectangle is a four-sided shape.');
}
}
const myRectangle = new Rectangle(10, 5);
console.log(myRectangle.area); // Output: 50
Rectangle.describe(); // Output: A rectangle is a four-sided shape.
In this example:
area
is a getter that computes the area of the rectangle.width
is a setter that validates the width before setting it.describe
is a static method that provides information about rectangles.
this
Keyword
In JavaScript, the this
keyword refers to the current execution context or the object that a function is a method of. The value of this
is determined by how a function is called. Understanding this
is crucial for writing object-oriented and functional JavaScript code. Here are the main scenarios in which the value of this
is determined, along with examples:
Global Context:
When used outside of any function, this
refers to the global object(e.g, window
in browser or global
in Node.js):
console.log(this); // Output: Window (in a browser environment)
Function Context:
A function
this
can have different values depending on how the function is invoked.In a regular function,
this
is determined by the calling context. If the function is not a method of an object or is not called withcall
,apply
, orbind
, it defaults to the global object (orundefined
in strict mode).
function showThis() {
console.log(this);
}
showThis(); // Output: Window (in a browser environment)
Method Context
When a function is a method of an object, this
refers to the objects on which the method is called.
var person = {
name: 'John',
sayHello: function() {
console.log("Hello, my name is " + this.name);
}
};
person.sayHello(); // Output: Hello, my name is John
Constructor Context
When a function is used as a constructor with the new
keyword, this
refers to the newly created object.
function Dog(name) {
this.name = name;
}
var myDog = new Dog('Buddy');
console.log(myDog.name); // Output: Buddy
Event Handler Context
In event handlers, this
often refers to the element that triggered the event.
<button id="myButton">Click me</button>
<script>
document.getElementById('myButton').addEventListener('click', () => {
console.log(this); // Output: <button> element });
</script>
Arrow Function Context
Arrow functions do not have their this
context. Instead, they inherit this
from enclosing scope (lexical scoping).
function outerFunction() {
return () => {
console.log(this);
};
}
var arrowFunction = outerFunction();
arrowFunction(); // Output: The value of `this` from outerFunction
Advanced JavaScript Functions To Improve Code Quality
There are some built-in features to create some of the most powerful functions to boost your performance and make your code look much more beautiful. I will cover Debounce, Throttle, Once, Memoize, Curry, Partial, Pipe, Compose, Pick, Omit, and Zip, which you can save in the utility file/class to optimize your code quality as a JavaScript developer.
Although the functions are explained using JavaScript, they could be easily implemented in any programming language. Once the concept of the different functions is understood, it can be applied everywhere.
Furthermore, the functions (or concepts) described in this post are often asked in technical interviews.
Whether you are a beginner or an experienced senior developer, these functions will optimize your code and coding experience. They will make working with JavaScript more enjoyable and efficient.
Debounce, Throttle
Check out my previous article on this link.
Once
The Once function is a method that will prevent execution if already been called. This is especially useful when working with event listeners, where you often encounter functions that should only run once. Instead of removing event listeners every time, you can use the Once function in JavaScript.
function once(func) {
let ran = false;
let result;
return function() {
if (ran) return result;
result = func.apply(this, arguments);
ran = true;
return result;
};
}
For example, you can have a function that sends a request to the server to load some data. With the once()
function, you could ensure that the request is not called multiple times if the user keeps clicking the button. This will avoid performance issues.
Without the once()
function, you would remove the click listener instantly after the request is sent to prevent sending the request again.
Applying the once()
function to any code will look like this:
// Define the function that sends the request
function requestSomeData() {
// Send the request...
}
// Create a version of the function that can only be called once
const sendRequestOnce = once(sendRequest);
// Listen for clicks on a button and call the "once" function
const button = document.querySelector("button");
button.addEventListener("click", sendRequestOnce);
In this example, the requestSomeData
function will be called once, even if the user clicks the button multiple times.
Memoize
Memoize is a JavaScript function that is used to cache the results of a given function to prevent calling computationally expensive routines multiple times with the same arguments.
function memoize(func) {
const cache = new Map();
return function() {
const key = JSON.stringify(arguments);
if (cache.has(key)) {
return cache.get(key);
}
const result = func.apply(this, arguments);
cache.set(key, result);
return result;
};
}
This memoize()
function will cache the result of a given function and uses the arguments as key to retrieve the result if called again with the same arguments.
Now, if you have a function that performs a complex calculation that is based on an input variable, you can use memoize()
function to cache the results and retrieve them instantly if called multiple times with the same input.
To see the benefits of the memoize()
function, you can use it to calculate the Fibonacci numbers:
// Define the function that performs the calculation
function fibonacci(n) {
if (n < 2)
return 1;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Create a memoized version of the function
const memoizedFibonacci = memoize(fibonacci);
// Call the memoized function with multiple input values
console.time('total')
console.time('sub1')
const result1 = memoizedFibonacci(30);
console.timeEnd('sub1')
console.time('sub2')
const result2 = memoizedFibonacci(29);
console.timeEnd('sub2')
console.time('sub3')
const result3 = memoizedFibonacci(30);
console.timeEnd('sub3')
console.timeEnd('total')
In this example, the Fibonacci()
function will be converted into a memoizedFibonacci
function. Then the memoized()
function will be called, and the execution time will be logged to the console.
The output will look like this:
Although the second call only calculated the Fibonacci number of 29 it took much longer than calculating the Fibonacci number of 30 a second time because it was cached by the memoize()
function.
Curry
The Curry function (also known as Currying) is an advanced JavaScript function used to create a new function from an existing one by “pre-filling“ some of its arguments. Currying is often used when working with functions that take multiple arguments and transform them into functions that take some arguments because the other will always stay the same.
Using the Curry function has several benefits:
It helps to avoid using the same variable again and again
It makes the code more readable
It divides functions into multiple smaller functions that can handle one responsibility
function curry(func, arity = func.length) {
return function curried(...args) {
if (args.length >= arity) return func(...args);
return function(...moreArgs) {
return curried(...args, ...moreArgs);
};
};
}
This Curry function takes another function (func
) and an optional argument arity
that defaults to the length of func
’s arguments. It returns a new function (curried
) that can be called with a arity
number of arguments. If not all arguments have been supplied, it returns a new function that can be called with more arguments until all required arguments have been provided. When all arguments are supplied, the original function (func
) is called, and its result will be returned.
To understand the benefits of the Curry function, you could think of a method to calculate the distance between two points in a plane. Using a Curry function, you can create a new function that will only require one of these points, making it easier to use.
The following snippet will show how the previously defined curry function is used to optimize the reliability of the implementation:
// Define the function that calculates the distance between two points
function distance(x1, y1, x2, y2) {
return Math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2);
}
// Create a curried version of the function that only requires one of the points
const distanceFromOrigin = curry(distance, 3)(0, 0);
// Call the curried function with the other point
const d1 = distanceFromOrigin(1, 1);
const d2 = distanceFromOrigin(2, 2);
In this example, a curried version of the distance
function is created (distanceFromOrigin
) by using the curry
function and passing distance
as the first argument and 3 as the second argument (arity
). Also, it will call the curried function with 0,0
as the first two arguments.
The resulting function distanceFromOrigin
is now a new function that needs only two arguments, and will always use 0,0
as the first point.
Partial
The Partial function in JavaScript is similar to the Curry function. The significant difference between Curry and Partial is that a call to a Partial function returns the result instantly instead of returning another function down the currying chain.
function partial(func, ...args) {
return function partiallyApplied(...moreArgs) {
return func(...args, ...moreArgs);
}
}
The partial
function in JavaScript typically takes an existing function, one or more input arguments, and returns a new function that calls the original function with the additional arguments passed in when the new function is called.
In the following use case, a calculate
function will be pre-filled with the first two arguments to generate a new function with a more readable name.
// Define the function that calculates something
function calculate(x, y, z) {
return (x + y) * z
}
// Create a partially applied version of the function the last argument
const multiply10By = partial(calculate, 8, 2);
// Call the partially applied function with the number of iterations
const result = multiply10By(5);
In this example, the multiply10By
function is created by partially applying the generic calculate
function and pre-filling the first arguments with 8 and 2. This will create a new function multiply10By
that only requires one argument, specifying the amount of 10 multiplication that has to be done. Also, it will make the code more readable and understandable.
Pipe
The Pipe function is a utility function used to chain multiple functions and pass the output of one to the next one in the chain. It’s similar to the Unix pipe operator and will apply all functions left to right by using the JavaScript reduce()
function.
function pipe(...funcs) {
return function piped(...args) {
return funcs.reduce((result, func) => [func.call(this, ...result)], args)[0];
};
}
To understand the pipe function, imagine you have three functions:
Add a Prefix to a String
Add a Suffix to a String
Convert a String to Uppercase
Then you can use the pipe function to create a new function that will apply every single one from left to right to a String.
// Define the functions that add to the string
function addPrefix(str) {
return "prefix-" + str;
}
function addSuffix(str) {
return str + "-suffix";
}
function toUppercase(str) {
return str.toUpperCase()
}
// Create a piped function that applies the three functions in the correct order
const decorated1 = pipe(addPrefix, addSuffix, toUppercase);
const decorated2 = pipe(toUppercase, addPrefix, addSuffix);
// Call the piped function with the input string
const result1 = decorated1("hello"); // PREFIX-HELLO-SUFFIX
const result2 = decorated2("hello"); // prefix-HELLO-suffix
In this example, the decorated1 and decorated2 functions are created by piping the addPrefix
, addSuffix
, and toUppercase
functions in different orders. The new functions, that are created can be called with the input string to apply the three original ones in the given order. The resulting output strings will be different because the order provided in the pipe function is different.
Compose
The Compose function is the same as the Pipe function, but it will use reduceRight
to apply all functions:
function compose(...funcs) {
return function composed(...args) {
return funcs.reduceRight((result, func) => [func.call(this, ...result)], args)[0];
};
}
This will result in the same functionality, but the functions are applied from right to left.
Pick
The Pick function in JavaScript is used to select specific values from an object. It is a way to create a new object by selecting certain properties from a provided object. It is a functional programming technique that allows extracting a subset of properties from any object if the properties are available.
Here’s the implementation of the Pick function:
function pick(obj, keys) {
return keys.reduce((acc, key) => {
if (obj.hasOwnProperty(key)) {
acc[key] = obj[key];
}
return acc;
}, {});
}
This function takes two parameters:
obj
: Original object which the new object will be createdkeys
: Array of keys to select into the new object.
To create a new object, the function will use the reduce()
method to iterate over the keys and compare them to the original object’s properties. If a value is present, it will be added to the accumulator object in the reduce function, which was initialized with {}
.
At the end of the reduce function, the accumulator object will be the new object and will contain only the specified properties that were in keys
array.
This function is useful if you want to avoid over-fetching data. With the Pick function, you can retrieve any objects from the database and then only pick()
needed properties and return them to the caller.
const obj = {
id: 1,
name: 'Paul',
password: '82ada72easd7',
role: 'admin',
website: 'https://www.paulsblog.dev',
};
const selected = pick(obj, ['name', 'website']);
console.log(selected); // { name: 'Paul', website: 'https://www.paulsblog.dev' }
This function will use the pick()
function to create a new object only containing name
and website
which can be returned to the caller without exposing the role
, and password
, or the id
.
Omit
The Omit function is the opposite of the Pick function, as it will remove certain properties from an existing object. That means you can avoid overfetching by hiding properties. It can be used as a replacement for the Pick function if the number of properties to hide is smaller than the number of properties to pick.
function omit(obj, keys) {
return Object.keys(obj)
.filter(key => !keys.includes(key))
.reduce((acc, key) => {
acc[key] = obj[key];
return acc;
}, {});
}
This function takes two parameters:
obj
: Original object from which the new object will be created fromkeys
: An array of keys that won’t be in the new object.
To create a new object and remove the properties the Object.keys()
function is used to create an array of keys for the original object. Then Javascript filter()
function will remove every key that was specified in the keys
argument. With the reduce
function, the remaining keys will be iterated, and a new object is returned that only consists of every key to provided in the keys
array.
In practice, you can use it if you have a large user object where you only want to remove the ID:
const obj = {
id: 1,
name: 'Paul',
job: 'Senior Engineer',
twitter: 'https://www.twitter.com/paulknulst',
website: 'https://www.paulsblog.dev',
};
const selected = omit(obj, ['id']);
console.log(selected); // {name: 'Paul', job: 'Senior Engineer', twitter: 'https://www.twitter.com/paulknulst', website: 'https://www.paulsblog.dev'}
In this example, the omit()
function is used to remove the id property and retrieve an object which will make your code more readable than using a for loop, setting obj.id = undefined
or using pick()
and supplying every attribute to pick.
Zip
The Zip function is a JavaScript function that matches each passed array of elements to another array element and is used to combine multiple arrays into a single array of tuples. The resulting array will contain the corresponding elements from each array. Often, this functionality is used when working with data from multiple sources that need to be merged or correlated in some way.
Unlike Python, JavaScript doesn't provide the Zip function out of the box. But the implementation is easy:
function zip(...arrays) {
const maxLength = Math.max(...arrays.map(array => array.length));
return Array.from({ length: maxLength }).map((_, i) => {
return Array.from({ length: arrays.length }, (_, j) => arrays[j][i]);
});
}
This JavaScript snippet will create a new array of arrays when every subarray is composed of the elements of the provided arrays. This means that every element of the original array will be mapped to another element from another original array in the same index.
For example, you could have three arrays that:
contains the x coordinate
contains the y coordinate
contains the z coordinate'
Without a zip function, you would manually loop through the arrays and pair the x,y, and z elements. But, by using the zip function, you can pass the original arrays and generate a new array of (x,y,z) tuples.
// Define the arrays that contain the coordinates
const xCoordinates = [1, 2, 3, 4];
const yCoordinates = [5, 6, 7, 8];
const zCoordinates = [3, 6, 1, 7];
// Create a zipped array of points
const points = zip(xCoordinates, yCoordinates, zCoordinates);
// Use the zipped array of points
console.log(points); // [[1, 5, 3], [2, 6, 6], [3, 7, 1], [4, 8, 7]]
In this example, the zip
function is used to combine the xCoordinates
, yCoordinates
, and zCoordinates
arrays into a single array of tuples.
8 Modern Javascript Reactive Patterns
Reactivity is essentially about how a system reacts to data changes and there are different types of reactivity. Our focus is on reactivity in terms of taking actions in response to data changes.
As a front-end engineer, I have to face it every single day. That’s because the browser itself is a fully asynchronous environment. Modern web interfaces must react quickly to user actions, and this includes updating the UI, sending network requests, managing navigation, and various other tasks.
While people often associate reactivity with frameworks, I believe that we can learn a lot by implementing it in pure Js. So, we are going to code patterns ourselves and also study some native browser APIs that are based on reactivity.
PubSub or Publish-Subscribe
PubSub is one of the most commonly used and fundamental reactivity patterns. The Publisher is responsible for notifying Subscribers about the updates and the Subscriber receives those updates and can react in response.
class PubSub {
constructor() {
this.subscribers = {};
}
subscribe(event, callback) {
if (!this.subscribers[event]) {
this.subscribers[event] = [];
}
this.subscribers[event].push(callback);
}
// Publish a message to all subscribers of a specific event
publish(event, data) {
if (this.subscribers[event]) {
this.subscribers[event].forEach((callback) => {
callback(data);
});
}
}
}
const pubsub = new PubSub();
pubsub.subscribe('news', (message) => {
console.log(`Subscriber 1 received news: ${message}`);
});
pubsub.subscribe('news', (message) => {
console.log(`Subscriber 2 received news: ${message}`);
});
// Publish a message to the 'news' event
pubsub.publish('news', 'Latest headlines: ...');
// console logs are:
// Subscriber 1 received news: Latest headlines: ...
// Subscriber 2 received news: Latest headlines: ...
One popular example of its usage is Redux. This popular state management library is based on this pattern (or more specifically, the Flux architecture). Thing works pretty simple in the context of Redux:
Publisher: The store acts as the publisher. When an action is dispatched, the store notifies all the subscribed components about the state change.
Subscriber: Ui components in the application are the subscribers. They subscribe to the redux store and receive updates whenever the state changes.
Custom Events as a Browser Version of PubSub
The browser offers an API for triggering or subscribing to custom events through the CustomEvent class and the dispatchEvent method. The latter provides us with the ability not only to trigger an event but also to attach any desired data to it.
const customEvent = new CustomEvent('customEvent', {
detail: 'Custom event data', // Attach desired data to the event
});
const element = document.getElementById('.element-to-trigger-events');
element.addEventListener('customEvent', (event) => {
console.log(`Subscriber 1 received custom event: ${event.detail}`);
});
element.addEventListener('customEvent', (event) => {
console.log(`Subscriber 2 received custom event: ${event.detail}`);
});
// Trigger the custom event
element.dispatchEvent(customEvent);
// console logs are:
// Subscriber 1 received custom event: Custom event data
// Subscriber 2 received custom event: Custom event data
Custom Event Targets
If you prefer not to dispatch an event globally on the window object, you can create your own event target.
By extending the native EventTarget class, you can dispatch events to the new instance. This ensures that your event is triggered only on the new class itself, avoiding global propagation. Moreover, you have the flexibility to attach handlers directly to this specific instance.
class CustomEventTarget extends EventTarget {
constructor() {
super();
}
// Custom method to trigger events
triggerCustomEvent(eventName, eventData) {
const event = new CustomEvent(eventName, { detail: eventData });
this.dispatchEvent(event);
}
}
const customTarget = new CustomEventTarget();
// Add an event listener to the custom event target
customTarget.addEventListener('customEvent', (event) => {
console.log(`Custom event received with data: ${event.detail}`);
});
// Trigger a custom event
customTarget.triggerCustomEvent('customEvent', 'Hello, custom event!');
// console log is:
// Custom event received with data: Hello, custom event!
Observer
The Observer pattern is really similar to PubSub. You subscribe to the subject and then it notifies its subscribers (Observers) about changes, allowing them to react accordingly. This pattern plays a significant role in building decoupled and flexible architecture.
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
// Remove an observer from the list
removeObserver(observer) {
const index = this.observers.indexOf(observer);
if (index !== -1) {
this.observers.splice(index, 1);
}
}
// Notify all observers about changes
notify() {
this.observers.forEach((observer) => {
observer.update();
});
}
}
class Observer {
constructor(name) {
this.name = name;
}
// Update method called when notified
update() {
console.log(`${this.name} received an update.`);
}
}
const subject = new Subject();
const observer1 = new Observer('Observer 1');
const observer2 = new Observer('Observer 2');
// Add observers to the subject
subject.addObserver(observer1);
subject.addObserver(observer2);
// Notify observers about changes
subject.notify();
// console logs are:
// Observer 1 received an update.
// Observer 2 received an update.
Reactive Properties With Proxy
if you want to react to changes in objects, Proxy is a way to go. It lets us achieve reactivity when setting or getting values of the object field.
const person = {
name: 'Pavel',
age: 22,
};
const reactivePerson = new Proxy(person, {
// Intercept set operation
set(target, key, value) {
console.log(`Setting ${key} to ${value}`);
target[key] = value;
// Indicates if setting value was successful
return true;
},
// Intercept get operation
get(target, key) {
console.log(`Getting ${key}`);
return target[key];
},
});
reactivePerson.name = 'Sergei'; // Setting name to Sergei
console.log(reactivePerson.name); // Getting name: Sergei
reactivePerson.age = 23; // Setting age to 23
console.log(reactivePerson.age); // Getting age: 23
Individual Object Properties and Reactivity
If you don’t need to track all the fields in the objects, you can choose the specific one using Object.defineProperty or group of them with Object.defineProperties.
const person = {
_originalName: 'Pavel', // private property
}
Object.defineProperty(person, 'name', {
get() {
console.log('Getting property name')
return this._originalName
},
set(value) {
console.log(`Setting property name to value ${value}`)
this._originalName = value
},
})
console.log(person.name) // 'Getting property name' and 'Pavel'
person.name = 'Sergei' // Setting property name to value Sergei
Reactive HTML Attributes With MutationObserver
One way to achieve reactivity in the DOM is by using MutationObserver. Its API allows us to observe changes in attributes and also in the text content of the target element and its children.
function handleMutations(mutationsList, observer) {
mutationsList.forEach((mutation) => {
// An attribute of the observed element has changed
if (mutation.type === 'attributes') {
console.log(`Attribute '${mutation.attributeName}' changed to '${mutation.target.getAttribute(mutation.attributeName)}'`);
}
});
}
const observer = new MutationObserver(handleMutations);
const targetElement = document.querySelector('.element-to-observe');
// Start observing the target element
observer.observe(targetElement, { attributes: true });
Reactive Scrolling With IntersectionObserver
The IntersectionObserver API enables reacting to the intersection of a target element with another element or the viewport area.
function handleIntersection(entries, observer) {
entries.forEach((entry) => {
// The target element is in the viewport
if (entry.isIntersecting) {
entry.target.classList.add('visible');
} else {
entry.target.classList.remove('visible');
}
});
}
const observer = new IntersectionObserver(handleIntersection);
const targetElement = document.querySelector('.element-to-observe');
// Start observing the target element
observer.observe(targetElement);
The Regular Expressions
You might see this written as regular expressions, regex, or RegExp, but all refer to the same thing.
Regex is a sequence of characters for matching a part of a string or the whole string. Matching strings with regular expressions might require more than just “characters“. Many times, you will need to use a special set of characters called “metacharacters“ and “quantifiers”.
Because regular expressions are a powerful tool, you can use them to do much more than just “matching strings“ when you combine regex with programming languages.
Almost all the main programming languages of the modern era have built-in support for regular expressions. Some programming languages might even have specific libraries that help you work more conveniently with regex.
Apart from using regular expressions in programming languages, other tools that let you use regular expressions are.
Text Editors and IDEs: These are for search and replacement in VS Code, Visual Studio, Notepad++, Sublime Text, and others.
Browser Developer Tools: These are mostly in-browser search (with extensions or add-ons) and search within the developer tools.
Database Tools: for data mining.
RegEx Testers: you can paste in text and write the regular expressions to match them – which is a very good way to learn regular expressions. This book explores that option quite a bit.
For more details, check out this article.
Stop Using .forEach: Transform your code with for…of Loop
In the realm of Javascript and Typescript, iterating over arrays is a common task. Many developers default to using .forEach
for its simplicity and familiarity.
However, there is a more powerful and versatile alternative: the for…of
loop.
Understanding the Basics: .forEach
vs. for...of
🔧
The .forEach
method is a straightforward way to iterate over an array. Here is a basic example:
The for…of
loop, introduced in ES6, offers a more flexible way to iterate over iterable objects (including arrays):
Why prefer for…of
?
While .forEach
is concise and easy to use, for…of
provides several advantages that can enhance your coding practice.
Better Asynchronous Handling
When dealing with asynchronous operations, for…of
shines. The .forEach
method doesn't work well with async/await
because it doesn't handle promises natively. Consider the following example:
This code does not wait for each fetch operation to complete before starting the next one, which can lead to race operations and unexpected results.
The main downstream of iterating arrays with forEach loop is that it can be postponed until the previous call is fulfilled.
This is very crucial is the real world when you work with the messive amount of assets that should be fetched one after another.
In this example, each fetch operation waits for the previous one to complete, ensuring sequential execution and more predictable behavior.
Breaking and Continuing Loops
The .forEach
method does not support the continue
and break
statements, which can limit its flexibility in certain scenarios.
Using for…of
to break a loop
Using for…of
to continue a loop
This feature makes for…of
more powerful and versatile for complex iteration logic.
Making Good Use of Console
Use case: Making good logging for debugging complex objects. Console methods like console.table
, console.group
, and console.time
can provide more structured and informative debug information.
// Basic logging
console.log('Simple log');
console.error('This is an error');
console.warn('This is a warning');
// Logging tabular data
const users = [
{ name: 'John', age: 30, city: 'New York' },
{ name: 'Jane', age: 25, city: 'San Francisco' },
];
console.table(users);
// Grouping logs
console.group('User Details');
console.log('User 1: John');
console.log('User 2: Jane');
console.groupEnd();
// Timing code execution
console.time('Timer');
for (let i = 0; i < 1000000; i++) {
// Some heavy computation
}
console.timeEnd('Timer');
Why Use It: Enhances the visibility and organization of debugging information, making it easier to diagnose and fix issues. Propper use of console methods can significantly improve the efficiency of your debugging process by providing clear, organized, and detailed logs.
Structured Cloning With structuredClone()
Deep clone objects using the new structuredClone
. Unlike the traditional shallow copy, structured cloning creates a deep copy of the object, and ensures that nested objects are also copied. This method avoids the limitation of JSON.parse(JSON.stringify(obj))
, which can not handle certain data types like functions, undefined
, and circular references.
Use case: Creating a deep copy of complex objects. This is useful when you need to duplicate objects for operations that should not mutate the original data.
const obj = {
a: 1,
b: { c: 2 },
date: new Date(),
arr: [1, 2, 3],
nestedArr: [{ d: 4 }]
};
const clonedObj = structuredClone(obj);
console.log(clonedObj);
// { a: 1, b: { c: 2 }, date: 2023-06-08T00:00:00.000Z, arr: [1, 2, 3], nestedArr: [{ d: 4 }] }
console.log(clonedObj === obj); // false
console.log(clonedObj.b === obj.b); // false
console.log(clonedObj.date === obj.date); // false
console.log(clonedObj.arr === obj.arr); // false
console.log(clonedObj.nestedArr[0] === obj.nestedArr[0]); // false
Why Use It: Provides a built-in, efficient way to perform deep cloning of objects, avoiding the pitfalls and complexities of manual deep copy implementations. This method is more reliable and handles complex data structures better than alternatives like JSON.parse(JSON.stringify(obj))
.
Self-Invoking Functions
Self-invoking functions, also known as Immediately Invoked Function Expressions(IIEF), are executed immediately after they are created. They are useful for encapsulating code to avoid polluting the global scope, or in scenarios where immediate execution is needed for initialization logic.
(function() {
const privateVar = 'This is private';
console.log('Self-invoking function runs immediately');
// Initialization code here
})();
// Private variables are not accessible from outside
// console.log(privateVar); // ReferenceError: privateVar is not defined
Why Use It: Helps maintain clean code by avoiding global variables and executing initialization code without leaving traces in the global scope. This approach can prevent conflicts in larger codebases and ensure better encapsulation of functionality, improving code maintainability and avoiding side effects.
Tagged Template Literals
Tagged template literals allow you to customize the way template literals are processed. They are useful for creating specialized templates, such as for internationalization, sanitizing HTML, or generating dynamic SQL queries.
Use Case: Sanitizing user input in HTML templates to prevent XSS attacks. This technique ensures that user-generated content is safely inserted into the DOM without executing any malicious scripts.
function sanitize(strings, ...values) {
return strings.reduce((result, string, i) => {
let value = values[i - 1];
if (typeof value === 'string') {
value = value.replace(/&/g, '&')
.replace(/</g, '<')
.replace(/>/g, '>')
.replace(/"/g, '"')
.replace(/'/g, ''');
}
return result + value + string;
});
}
const userInput = '<script>alert("xss")</script>';
const message = sanitize`User input: ${userInput}`;
console.log(message); // User input: <script>alert("xss")</script>
Why Use It: Provides a powerful mechanism to control and customize the output of template literals, enabling safer and more flexible template creation. Tagged template literals can be used to enforce security, format strings, and generate dynamic content, enhancing the robustness and versatility of your code.
12 Javascript Web APIs to build Futuristic Websites You didn’t know
With the rapidly changing technologies, developers are being provided with incredible new tools and APIs. However, it has been seen that out of the 100+ APIs, only 5% of them are actively used by developers.
Let’s take a look at some of the useful Web APIs that can help you skyrocket your website to noon.
Check out the list in this article.
The Mysteries of bind()
, call()
, and apply()
Ever been deep in a Javascript rabbit hole and come across bind()
, call()
, and apply()
? if you have ever scratched your head wondering what is the deal with these, you are not alone. Let’s chat about them like we are catching up over a cup of coffee.
Here is our line-up:
bind(): Think of it like giving a function a backpack filled with stuff(context), it will always carry around, no matter where it goes.
call(): Imagine telling a function, “Hey, I need you to do this right now, with these items.“. You hand over the items one by one.
apply(): Same as
call()
, but instead of handling items individually, you give a function a whole box (array) of items.
bind() - The backpack method
Here is a simple way to understand bind()
. Imagine you have got a little script that talks about someone.
function introduce() {
return `Hey, I’m ${this.name}!`;
}
const person = { name: “Alex” };
const sayHi = introduce.bind(person);
console.log(sayHi()); // “Hey, I’m Alex!”
With bind()
, it’s like giving the introduce function a backpack with a name tag that says “Alex.“
call() - The Immediate Action
call()
is like being at a burger joint. You need to tell them what exactly toppings you want and expect the burger right away.
function orderBurger(topping1, topping2) {
console.log(`I’d like a burger with ${topping1} and ${topping2}, please!`);
}
orderBurger.call(null, “cheese”, “lettuce”); // I’d like a burger with cheese and lettuce, please!
See? You immediately tell the function what you want, and it serves it up right then
apply() — The Boxed Lunch
apply()
is pretty similar to call()
, but instead of listing out toppings for your burger, you hand over the lunch box with everything inside.
function makePizza(topping1, topping2) {
console.log(`Whipping up a pizza with ${topping1} and ${topping2}!`);
}
const toppings = [“pepperoni”, “mushrooms”];
makePizza.apply(null, toppings); // Whipping up a pizza with pepperoni and mushrooms!
You are still getting your pizza, but this time, all the ingredients came in a tidy little box.
Explore the hidden power of Javascript Generators
Javascript Generators were first introduced in ES6. They are just normal functions with a little bit of strange behavior. They can stop their execution in the middle of the function and resume it further at the same point.
How do they differ from normal functions?
In the normal Javascript function, we expect the code inside will execute until we reach a return statement, an error, or the end of the function.
With a generator function, we are changing that behavior with the yield
keyword. When we encounter yield
in our function we are expressing that we would like to pause the execution, allowing us to get the value out or into the function.
Syntax
They are declared like a normal function plus one *
added to it. Here is the simplest example. A function that returns the numbers from 1 to 5.
function *example() {
yield 1;
yield 2;
yield 3;
yield 4;
return 5;
}
const func = example();
console.log(func.next()); // { value:1, done:false }
console.log(func.next()); // { value:2, done:false }
console.log(func.next()); // { value:3, done:false }
console.log(func.next()); // { value:4, done:false }
console.log(func.next()); // { value:5, done:true }
The most important thing about generators is the yield
keyword. It is called an yield expression
, because when we restart the generator, we will send the value back in, and whatever we send will be the computed result of that expression. To iterate on the generator we should use the method .next()
over it. It actually returns the response of a type object with two properties: value and done. The value property is the yield
ed-out value, and done is a boolean that indicates if the generator has completed or not.
What are the advantages?
Memory Efficient: Generators are memory efficient, which means that the only values that are generated are those that are needed. With normal functions, values should be generated and kept to be used later on. Only those data and computations that are necessary, are used.
Lazy Evaluation: The evaluation of an expression is not calculated until its value is needed. If it is not needed, it won’t exist. It is calculated on demand.
Use Cases: You may ask yourself, why do I need that? Well, there are plenty of good practical examples of where and how we can use generators.
Unique ID Generator: First the basic one is a ID generator. You should want everyone to have a unique ID so instead of using closure, you can do it with generators.
function* idGenerator() { let i = 1; while (true) { yield i++; } } const ids = idGenerator(); console.log(ids.next().value); // 1 console.log(ids.next().value); // 2 console.log(ids.next().value); // 3
Using With Promises
Here is an example of promise. The entire structure might look complicated, but if we focus on *main(), we can see that we are calling the API and getting results in data as if it were a synchronous call. There is only the addition of the yield
in it.
const URL = 'https://someAPI?name=';
function callAPI(name) {
const url = `${URL}${name}`;
return new Promise(function(resolve, reject) {
$.ajax({
url,
success: function(data) {
resolve(data);
}
});
});
}
function* main() {
try {
const data = yield callAPI('Svetli');
console.log('Data: ' + data);
} catch (err) {
console.error(err);
}
}
const it = main();
const res = it.next();
res.value.then(data => it.next(data));
If we want to write great code it should be easily maintained by other developers and generators give us exactly that: a clean and understandable structure that is easy to follow.
Advanced Uses Of Promises
The Promise object implements eventual completion (or failure) of an asynchronous operation and its resulting values.
A Promise is always in one of the following states:
Pending: The initial state, neither fulfilled or rejected.
Fulfilled: The operation was completed successfully.
Rejected: The operation failed.
Unlike “old-style“ callbacks, using Promises has the following conventions:
Callback functions will not be called until the current event loop completes.
Even if the asynchronous operation completes (successfully or unsuccessfully), callbacks added via
then()
afterward will still be called.You can add callbacks by calling
then()
multiple times, and they will be executed in the order they were added.
The characteristic feature of Promises is chaining.
Usages
- Promise.all([])
When all Promise instances in the array succeed, it returns an array of success results in the order they were requested. If any Promise fails, it enters the failure callback.
const p1 = new Promise((resolve) => {
resolve(1);
});
const p2 = new Promise((resolve) => {
resolve(1);
});
const p3 = Promise.resolve('ok');
// If all promises succeed, result will be an array of 3 results.
const result = Promise.all([p1, p2, p3]);
// If one fails, the result is the failed promise's value.
- Promise.allSettled([])
The execution will not fail; it returns an array corresponding to the status of each Promise instance in the input array.
const p1 = Promise.resolve(1);
const p2 = Promise.reject(-1);
Promise.allSettled([p1, p2]).then(res => {
console.log(res);
});
// Output:
/*
[
{ status: 'fulfilled', value: 1 },
{ status: 'rejected', reason: -1 }
]
*/
- Promise.any([])
If any Promise in the input array fulfills, the returned instance will become fulfilled and return the value of the first fulfilled promise. If all are rejected, it will become rejected.
const p1 = new Promise((resolve, reject) => {
reject(1);
});
const p2 = new Promise((resolve, reject) => {
reject(2);
});
const p3 = Promise.resolve("ok");
Promise.any([p1, p2, p3]).then(
(r) => console.log(r), // Outputs 'ok'
(e) => console.log(e)
);
- Promise.race([])
As soon as any Promise in the array changes state, the state of race
method will change accordingly; the value of the first changed Promise will be passed to the race
method’s callback.
const p1 = new Promise((resolve) => {
setTimeout(() => {
resolve(10);
}, 3000);
});
const p2 = new Promise((resolve, reject) => {
setTimeout(() => {
throw new Error("I encountered an error");
}, 2000);
});
Promise.race([p1, p2]).then(
(v) => console.log(v), // Outputs 10
(e) => console.log(e)
);
Throwing an exception does not change the race
state; it is still determined by p1
.
Advanced Uses
Here are 9 advanced uses that help developers handle asynchronous operations more efficiently and elegantly.
- Concurrency Control
Using Promise.all
allows for parallel executions of multiple Promises, but to control the number of simultaneous requests, you can implement a concurrency control function.
const concurrentPromises = (promises, limit) => {
return new Promise((resolve, reject) => {
let i = 0;
let result = [];
const executor = () => {
if (i >= promises.length) {
return resolve(result);
}
const promise = promises[i++];
Promise.resolve(promise)
.then(value => {
result.push(value);
if (i < promises.length) {
executor();
} else {
resolve(result);
}
})
.catch(reject);
};
for (let j = 0; j < limit && j < promises.length; j++) {
executor();
}
});
};
- Promise Timeout
Sometimes, you may want to a Promise to automatically reject if it does not resolve in a certain time frame. This can be implemented as follows.
const promiseWithTimeout = (promise, ms) =>
Promise.race([
promise,
new Promise((resolve, reject) =>
setTimeout(() => reject(new Error('Timeout after ' + ms + 'ms')), ms)
)
]);
- Cancelling Promises
Native Javascript Promises can not be cancelled, but you can simulate cancellation by introducing controllable interrupt logic.
const cancellablePromise = promise => {
let isCanceled = false;
const wrappedPromise = new Promise((resolve, reject) => {
promise.then(
value => (isCanceled ? reject({ isCanceled, value }) : resolve(value)),
error => (isCanceled ? reject({ isCanceled, error }) : reject(error))
);
});
return {
promise: wrappedPromise,
cancel() {
isCanceled = true;
}
};
};
- Sequential Execution with Promises Array
Sometimes, you need to execute the series of Promises in order, ensuring that the previous Promise operation completes before starting the next.
const sequencePromises = promises =>
promises.reduce(
(prev, next) => prev.then(() => next()),
Promise.resolve()
);
- Retry Logic for Promises
When a Promise is rejected due to temporary errors, you may want to retry its execution.
const retryPromise = (promiseFn, maxAttempts, interval) => {
return new Promise((resolve, reject) => {
const attempt = attemptNumber => {
if (attemptNumber === maxAttempts) {
reject(new Error('Max attempts reached'));
return;
}
promiseFn().then(resolve).catch(() => {
setTimeout(() => {
attempt(attemptNumber + 1);
}, interval);
});
};
attempt(0);
});
};
- Ensuring a Promise only resolves only once
In some cases, you may want to ensure that a Promise resolves only once, even if resolve
is called multiple times.
const onceResolvedPromise = executor => {
let isResolved = false;
return new Promise((resolve, reject) => {
executor(
value => {
if (!isResolved) {
isResolved = true;
resolve(value);
}
},
reject
);
});
};
- Using Promises Instead of Callbacks
Promises provide a more standardized and convenient way to handle asynchronous operations by replacing callback functions.
const callbackToPromise = (fn, ...args) => {
return new Promise((resolve, reject) => {
fn(...args, (error, result) => {
if (error) {
reject(error);
} else {
resolve(result);
}
});
});
};
- Dynamically Generating a Promise Chain
In some situations, you may need to dynamically create a series of Promise chains based on different conditions.
const tasks = [task1, task2, task3]; // Array of asynchronous tasks
const promiseChain = tasks.reduce((chain, currentTask) => {
return chain.then(currentTask);
}, Promise.resolve());
- Using Promises to Implement a Simple Asynchronous Lock
In a multi-threaded environment, you can use Promises to implement a simple asynchronous lock, ensuring that only one task can access shared resources at a time.
let lock = Promise.resolve();
const acquireLock = () => {
let release;
const waitLock = new Promise(resolve => {
release = resolve;
});
const tryAcquireLock = lock.then(() => release);
lock = waitLock;
return tryAcquireLock;
};
This code creates and resolves Promises continuously, implementing a simple FIFO queue to ensure that only one task can access shared resources. The lock
variable represents whether there is a task currently executing, always pointing to the Promise of the task in progress. The acquireLock
function requests permission to execute and creates a new Promise to wait for the current task to finish.
Conclusion
JavaScript is a language rich with features that can help you write cleaner, more efficient code. By mastering these advanced concepts, you can improve your productivity and enhance the readability of your code.
Happy coding !!!
References
https://medium.com/version-1/cloning-an-object-in-javascript-shallow-copy-vs-deep-copy-fa8acd6681e9
https://www.freecodecamp.org/news/javascript-let-and-const-hoisting/
https://blog.jetbrains.com/webstorm/2024/10/javascript-best-practices-2024/
https://www.paulsblog.dev/advanced-javascript-functions-to-improve-code-quality/
https://levelup.gitconnected.com/8-modern-javascript-reactive-patterns-0b5698fdb46e
https://medium.com/@bjprajapati381/10-advanced-javascript-tricks-you-dont-know-f1929e40703d
https://medium.com/@obrm770/javascript-under-the-hood-8cec84bbfd64
https://blog.stackademic.com/9-must-know-advanced-uses-of-promises-a6d1ab195dfc
Subscribe to my newsletter
Read articles from Tuan Tran Van directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
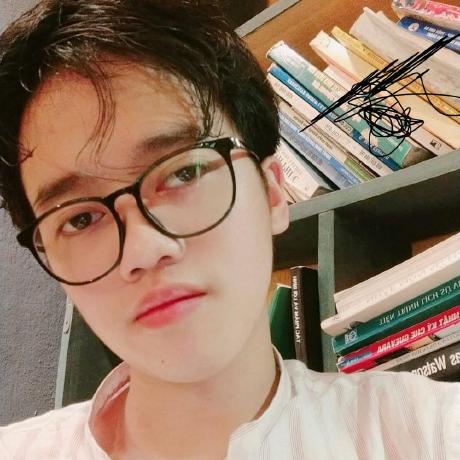
Tuan Tran Van
Tuan Tran Van
I am a developer creating open-source projects and writing about web development, side projects, and productivity.