Angular Signals
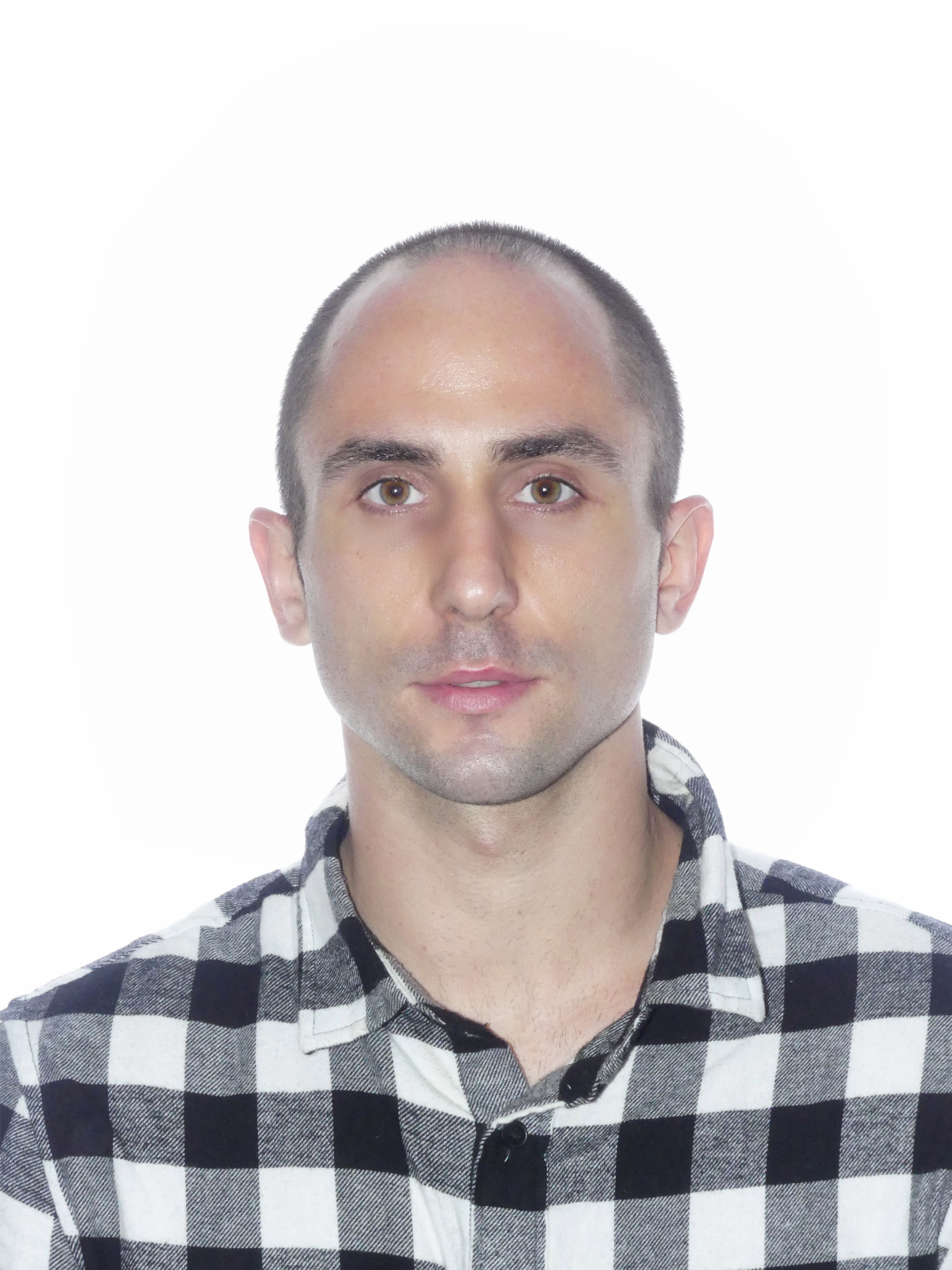
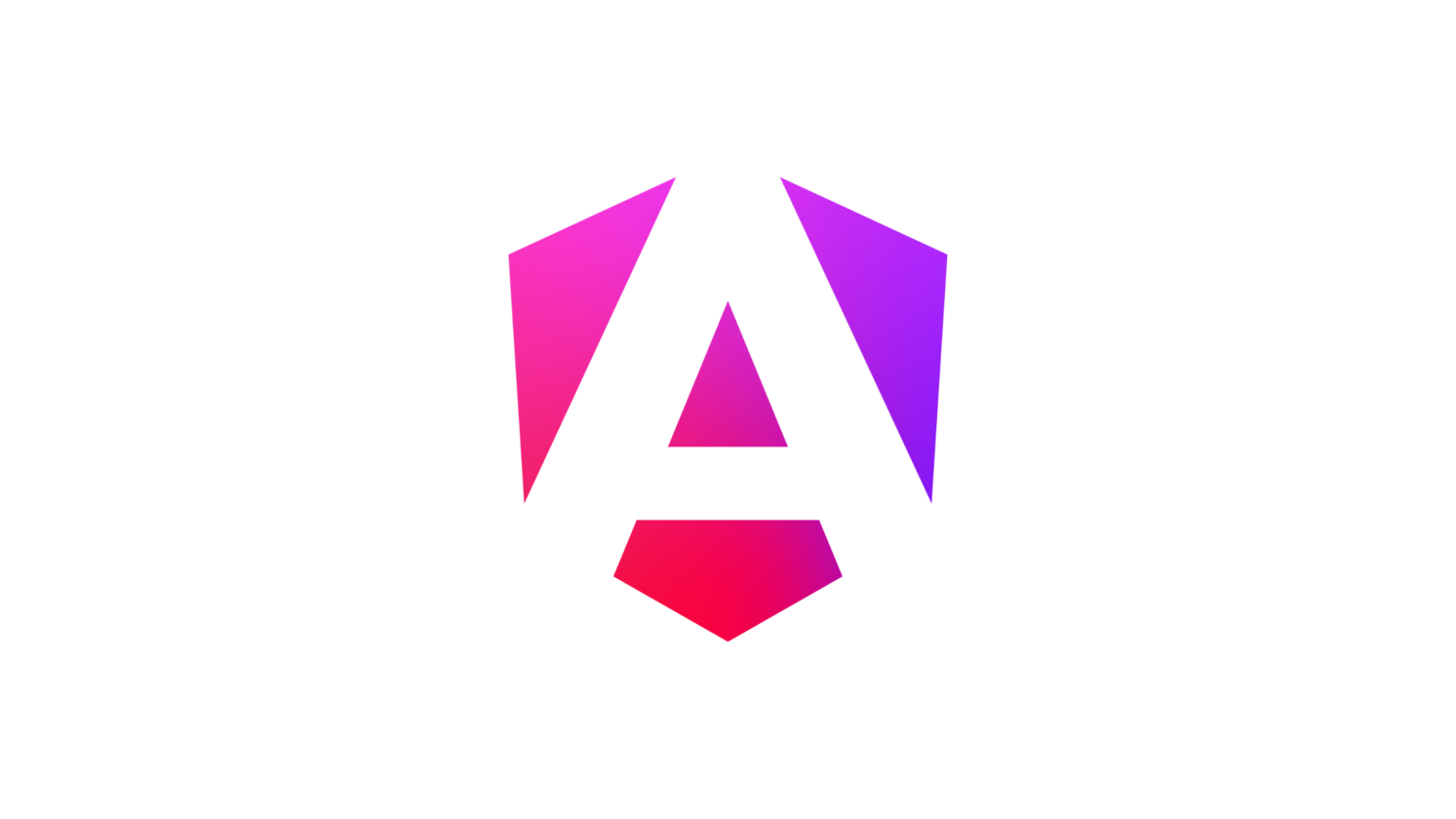
The next explanations are based on this project. To get the most of this article it is recommended to not only read it, but to practice creating a similar project.
This is a demo of the application functionality:
With the arrival of Angular Signals, achieving reactivity and handling change detection have become much simpler than when using Zone.js.
To explore this assertion, we will configure our application to run without Zone.js—thereby removing its automatic change detection mechanism—and rely solely on Signals for reactivity:
import {
ApplicationConfig,
provideExperimentalZonelessChangeDetection,
} from '@angular/core';
export const appConfig: ApplicationConfig = {
providers: [provideExperimentalZonelessChangeDetection()],
};
Then, we will create the interface for our fetched data:
export interface Data {
imageUrl: string;
title: string;
}
Next, the we will create the service that fetches and modifies the data. We have asigned 1 second delay in fetchData method to simulate a request and we have added a reverseData method to modify data and see how the modification is shown instantly in the app, showing the reactive capabilities of signals (switching effect of images and titles, as shown in the video):
import { Injectable, signal } from '@angular/core';
import { Data } from '../models/data.interface';
@Injectable({
providedIn: 'root',
})
export class DataService {
private readonly data = signal<Data[]>([]);
get dataSignal() {
return this.data.asReadonly();
}
// request simulation
fetchData() {
const newData: Data[] = [
{
imageUrl:
'https://images-wixmp-ed30a86b8c4ca887773594c2.wixmp.com/f/a97cc6bd-3b7b-44aa-b03d-90717569e29b/dxnwwo-95a4dba9-a6a5-4c73-a567-4c3f00c24607.jpg/v1/fill/w_1024,h_768,q_75,strp/pupy_dogs__3_by_little_drunk_jesus_dxnwwo-fullview.jpg?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJzdWIiOiJ1cm46YXBwOjdlMGQxODg5ODIyNjQzNzNhNWYwZDQxNWVhMGQyNmUwIiwiaXNzIjoidXJuOmFwcDo3ZTBkMTg4OTgyMjY0MzczYTVmMGQ0MTVlYTBkMjZlMCIsIm9iaiI6W1t7ImhlaWdodCI6Ijw9NzY4IiwicGF0aCI6IlwvZlwvYTk3Y2M2YmQtM2I3Yi00NGFhLWIwM2QtOTA3MTc1NjllMjliXC9keG53d28tOTVhNGRiYTktYTZhNS00YzczLWE1NjctNGMzZjAwYzI0NjA3LmpwZyIsIndpZHRoIjoiPD0xMDI0In1dXSwiYXVkIjpbInVybjpzZXJ2aWNlOmltYWdlLm9wZXJhdGlvbnMiXX0.ShrBRJlt9X27CrEkj8EZr1s6tkjqhIAIMvV1fN8Kdwk',
title: 'Brown pupy',
},
{
imageUrl:
'https://images-wixmp-ed30a86b8c4ca887773594c2.wixmp.com/f/a97cc6bd-3b7b-44aa-b03d-90717569e29b/dxnwso-a8ad02ba-f373-470f-baeb-bebfda554aaa.jpg/v1/fill/w_1024,h_768,q_75,strp/pupy_dogs__2_by_little_drunk_jesus_dxnwso-fullview.jpg?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJzdWIiOiJ1cm46YXBwOjdlMGQxODg5ODIyNjQzNzNhNWYwZDQxNWVhMGQyNmUwIiwiaXNzIjoidXJuOmFwcDo3ZTBkMTg4OTgyMjY0MzczYTVmMGQ0MTVlYTBkMjZlMCIsIm9iaiI6W1t7ImhlaWdodCI6Ijw9NzY4IiwicGF0aCI6IlwvZlwvYTk3Y2M2YmQtM2I3Yi00NGFhLWIwM2QtOTA3MTc1NjllMjliXC9keG53c28tYThhZDAyYmEtZjM3My00NzBmLWJhZWItYmViZmRhNTU0YWFhLmpwZyIsIndpZHRoIjoiPD0xMDI0In1dXSwiYXVkIjpbInVybjpzZXJ2aWNlOmltYWdlLm9wZXJhdGlvbnMiXX0.xHFQ2oacJqg1pyqs9Ax4bQw7CtIt0f0Af3zxWWyUi1U',
title: '2 pupies',
},
];
setTimeout(() => {
this.data.set(newData);
}, 1000);
}
reverseData() {
this.data.update((data) => {
const reversedData = data.reverse();
return reversedData.slice();
});
}
}
And then we will create 2 components to reflect the changes in the UI
import { Component, computed, inject, Signal } from '@angular/core';
import { DataService } from '../../services/data.service';
@Component({
selector: 'app-image',
standalone: true,
imports: [],
templateUrl: './image.component.html',
styleUrl: './image.component.sass',
})
export class ImageComponent {
private readonly dataService = inject(DataService);
readonly imageUrls!: Signal<string[]>;
constructor() {
this.imageUrls = computed(() => {
return this.dataService.dataSignal().map((item) => item.imageUrl);
});
}
}
<h1>IMAGE</h1>
<ul>
@for (url of imageUrls(); track url) {
<div>
<img [src]="url" width="200" />
</div>
}
</ul>
import { Component, computed, inject, Signal } from '@angular/core';
import { DataService } from '../../services/data.service';
@Component({
selector: 'app-list',
standalone: true,
imports: [],
templateUrl: './list.component.html',
styleUrl: './list.component.sass',
})
export class ListComponent {
private readonly dataService = inject(DataService);
readonly titles!: Signal<string[]>;
constructor() {
this.titles = computed(() => {
return this.dataService.dataSignal().map((item) => item.title);
});
}
}
<h1>LIST</h1>
<ul>
@for (title of titles(); track title) {
<li>{{ title }}</li>
}
</ul>
As you can see using “root” services and Signals together, we can maintain a Signal holding the fetched data and create multiple computed Signals, each serving its own purpose in its respective component.
Subscribe to my newsletter
Read articles from Jon Andoni Castelo Meléndez directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
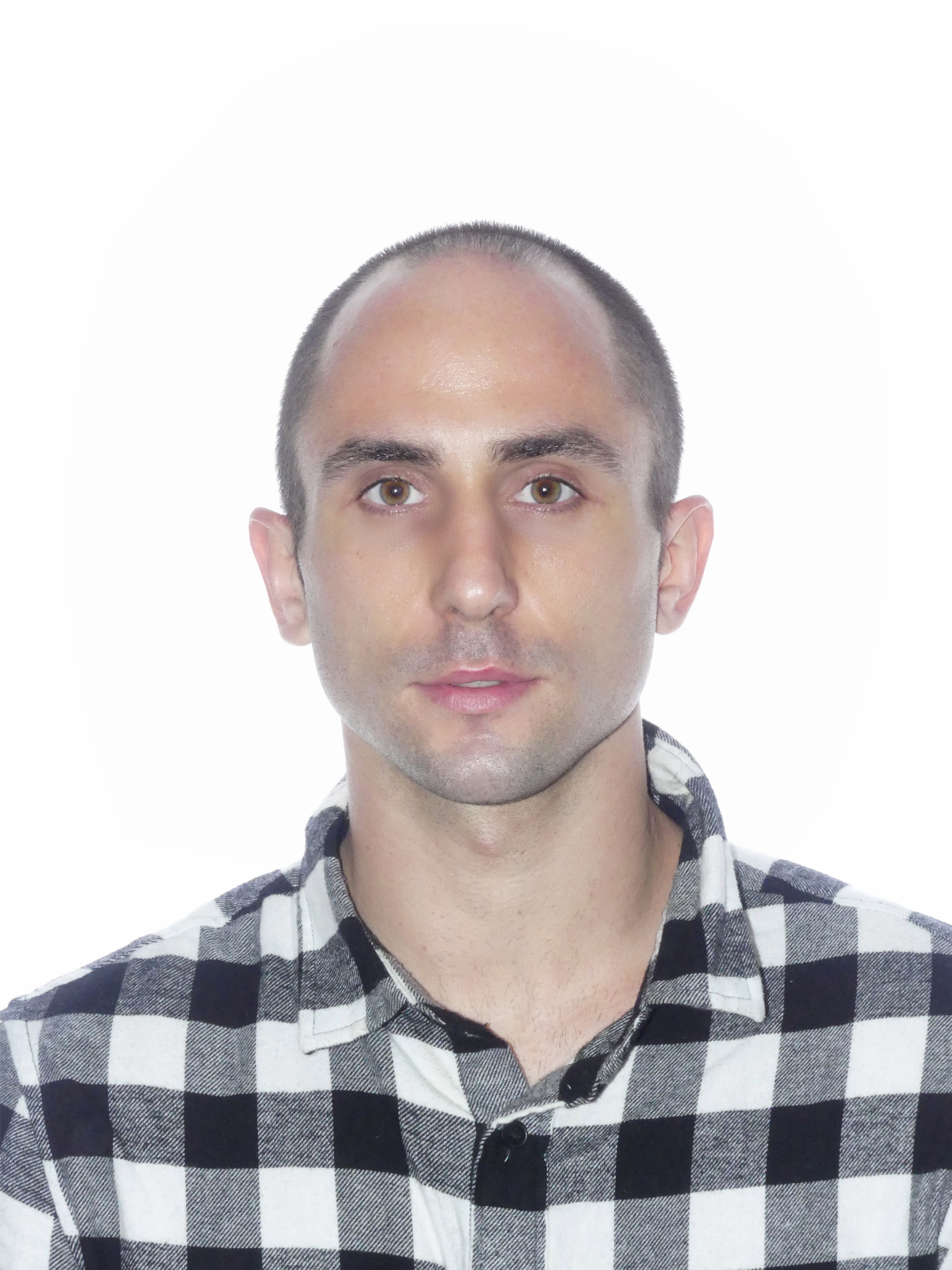
Jon Andoni Castelo Meléndez
Jon Andoni Castelo Meléndez
I'm a full-stack and DevOps developer with four years of experience. I usually work with Angular, Nodejs, MongoDB, and Kubernetes