JavaScript vs. JSX: Understanding Their Role in Modern Web Development

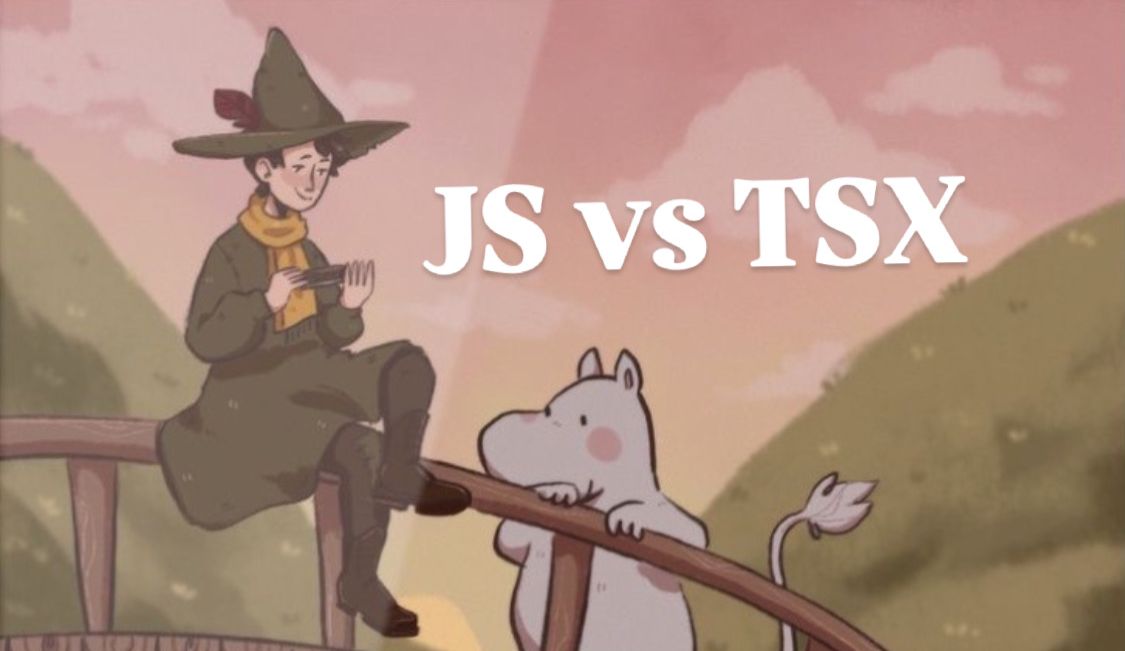
JavaScript has been the backbone of web development for decades, powering the interactivity and logic of web applications. Its evolution gave birth to JSX, an HTML-like syntax embedded within JavaScript, which transformed how developers build dynamic user interfaces. To understand how we arrived at JSX, it’s helpful to explore similar server-side templating concepts like Jinja in Flask and how they compare to JSX’s client-side rendering approach.
JavaScript: The Core of Interactivity
JavaScript has always been the language for adding behavior to web pages. In the early days, developers used JavaScript to manually manipulate the DOM, creating and appending HTML elements dynamically. For example, creating a list dynamically in plain JavaScript would look like this:
const items = ["JavaScript", "DOM", "Web"];
const ul = document.createElement("ul");
items.forEach(item => {
const li = document.createElement("li");
li.textContent = item;
ul.appendChild(li);
});
document.body.appendChild(ul);
This approach works, but it gets verbose and harder to manage as your UI becomes more complex. JavaScript alone doesn’t provide an easy way to structure user interfaces declaratively, which is where newer technologies come in.
Jinja in Flask: Server-Side Rendering
Before client-side rendering gained popularity, server-side templating engines like Jinja were the primary way to build dynamic web pages. Jinja, used with Flask in Python, allows developers to generate HTML on the server by embedding Python logic directly into templates.
For instance, a Flask route might pass a list of items to a Jinja template like this:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
items = ["Flask", "Jinja", "Templates"]
return render_template("home.html", items=items)
The corresponding Jinja template would handle rendering:
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
When the user requests the page, the server processes the template, replaces placeholders like {{ item }}
, and sends the fully-rendered HTML to the browser. This server-driven approach simplifies rendering for static or mostly-static pages, but it doesn’t scale well for highly interactive web apps. Every change or update requires a full-page reload or AJAX calls.
JSX: A Declarative, Component-Based Approach
React introduced a new way to think about building user interfaces with JSX. Instead of rendering HTML on the server and sending it to the client, React manages rendering on the client. JSX allows developers to write HTML-like code directly within JavaScript, creating components that describe the UI in a declarative way.
Take the same list example from before, but in React with JSX:
function App() {
const items = ["React", "JSX", "Components"];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
This syntax blends HTML and JavaScript seamlessly. React handles rendering in the browser, updating the DOM dynamically without requiring a full-page reload. JSX eliminates the need for manual DOM manipulation, making it easier to focus on the structure of the UI.
JSX is not HTML—it’s syntactic sugar for JavaScript. During the build process, tools like Babel compile JSX into regular JavaScript.
The example above compiles into something like this:
React.createElement(
"ul",
null,
items.map((item, index) =>
React.createElement("li", { key: index }, item)
)
);
Comparing Jinja and JSX
Jinja and JSX both aim to simplify dynamic content creation, but they operate in very different environments. Jinja works on the server, injecting data into templates before sending them to the browser. JSX works on the client, dynamically rendering and updating the UI in response to user interactions.
For example, updating a list with Jinja would require a new server request to re-render the page, while JSX can instantly update the UI in the browser by modifying the virtual DOM. Jinja keeps the logic and rendering tied to the backend, whereas JSX shifts the rendering responsibility to the frontend, making it ideal for single-page applications (SPAs).
TSX: Adding Type Safety
As web apps grew more complex, the need for predictability and type safety led to the rise of TypeScript. TSX is JSX with TypeScript support, offering static typing to catch potential errors during development. Here’s how the same React example might look in TSX:
type Item = {
id: number;
name: string;
};
function App() {
const items: Item[] = [
{ id: 1, name: "React" },
{ id: 2, name: "TSX" }
];
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
TSX ensures the items
array and its elements adhere to the Item
type, reducing runtime errors and making the code easier to understand and maintain.
JSX and its evolution into TSX showcase the shift from server-driven rendering to client-side interactivity. While technologies like Jinja in Flask excel in generating static or semi-dynamic pages on the server, JSX thrives in building highly interactive, component-based UIs. Both serve their purposes, but JSX has become essential for creating modern, seamless web applications.
Subscribe to my newsletter
Read articles from Prajjwal Datir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Prajjwal Datir
Prajjwal Datir
bequeath a treasure