Integrating Monolog into a WordPress Plugin Using the WPPB Boilerplate
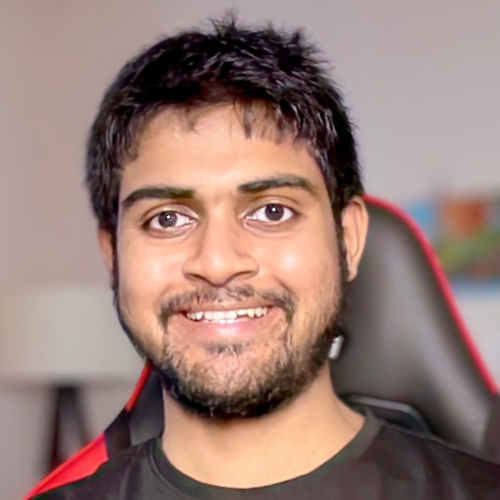
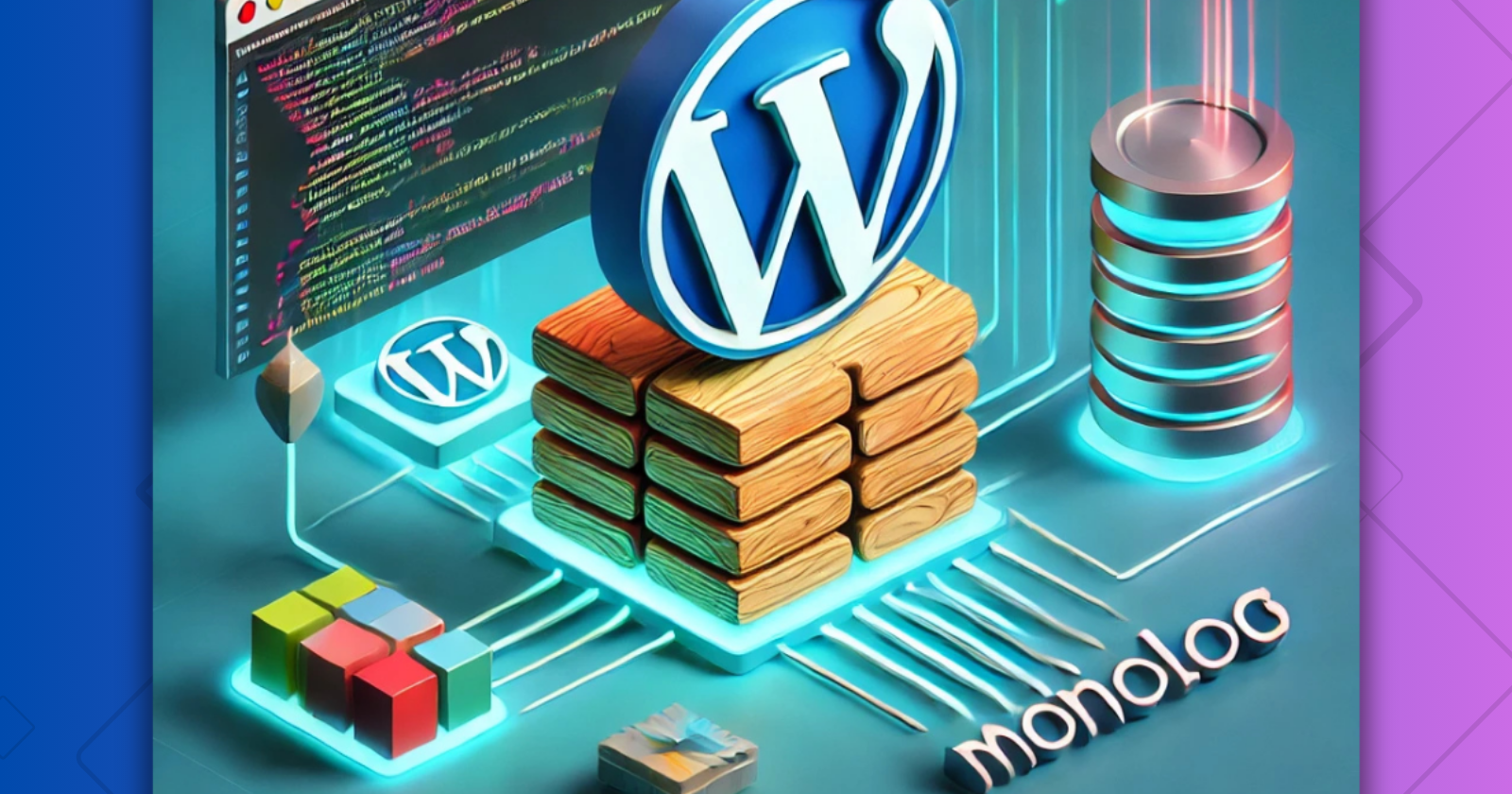
Logging is an essential part of any application or plugin. It helps developers track errors, debug issues, and monitor plugin behavior in real-time. When developing a WordPress plugin, using a robust logging library like Monolog can significantly enhance your debugging and monitoring capabilities. This tutorial walks you through integrating Monolog into a WordPress plugin using the WordPress Plugin Boilerplate (WPPB).
What is Monolog?
Monolog is a powerful PHP library that enables logging to various destinations, such as files, databases, cloud services, and more. It supports multiple logging levels, including DEBUG
, INFO
, WARNING
, and ERROR
, making it an excellent choice for WordPress plugin development.
Why Use Monolog in WordPress Plugins?
Advanced Logging Features: Log to multiple destinations, add context to logs, and format logs.
Debugging Made Easy: Quickly identify and resolve issues during development.
Scalability: Handle large-scale applications with ease.
Reusability: Create a logging utility you can reuse across projects.
Step-by-Step Integration
Step 1: Install Monolog Using Composer
Start by installing Monolog in your plugin directory using Composer.
Navigate to your plugin directory:
cd /path/to/your/plugin
Install Monolog:
composer require monolog/monolog
This creates a vendor
directory and an autoload.php
file inside your plugin folder.
Step 2: Include Composer’s Autoloader
You need to load the Composer autoloader to use Monolog in your plugin. Open the main class file of your WPPB plugin (e.g., includes/class-your-plugin-name.php
) and add the following code:
// Include the Composer autoloader if it exists
if ( file_exists( plugin_dir_path( __FILE__ ) . '../vendor/autoload.php' ) ) {
require plugin_dir_path( __FILE__ ) . '../vendor/autoload.php';
}
This ensures the Monolog library is available for your plugin.
Step 3: Create a Logger Utility Class
To keep your code organized, create a separate class for logging functionality. Add a new file in the includes/
folder, e.g., includes/class-your-plugin-logger.php
.
Logger Class Code:
<?php
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
if ( ! defined( 'ABSPATH' ) ) {
exit; // Exit if accessed directly.
}
class Your_Plugin_Logger {
private static $logger = null;
/**
* Get the Monolog Logger instance
*
* @return Logger
*/
public static function get_logger() {
if ( self::$logger === null ) {
self::$logger = new Logger( 'your_plugin' );
// Log to a file in the plugin directory
$log_file = plugin_dir_path( __FILE__ ) . '../logs/your-plugin.log';
self::$logger->pushHandler( new StreamHandler( $log_file, Logger::DEBUG ) );
}
return self::$logger;
}
/**
* Log info messages
*
* @param string $message
* @param array $context
*/
public static function info( $message, $context = [] ) {
self::get_logger()->info( $message, $context );
}
/**
* Log error messages
*
* @param string $message
* @param array $context
*/
public static function error( $message, $context = [] ) {
self::get_logger()->error( $message, $context );
}
}
Step 4: Log Messages in Your Plugin
You can now use the Your_Plugin_Logger
class to log messages anywhere in your plugin.
Example: Logging in an Action Hook
Add the following code in your plugin's main class or any other appropriate location:
add_action( 'init', function() {
Your_Plugin_Logger::info( 'Plugin initialized', [ 'time' => current_time( 'mysql' ) ] );
Your_Plugin_Logger::error( 'An error occurred', [ 'error_code' => 1234 ] );
});
Step 5: Create a Logs Directory
Monolog writes logs to a file. Ensure a logs/
directory exists in your plugin folder:
Create the directory:
mkdir logs
Set proper permissions:
chmod 755 logs
Step 6: Add a Log Cleanup Option
Over time, your log file might grow large. Add a cleanup mechanism to delete old logs periodically or on-demand via an admin option.
Example: Deleting Logs
add_action( 'admin_init', function() {
if ( isset( $_GET['delete_logs'] ) ) {
$log_file = plugin_dir_path( __FILE__ ) . 'logs/your-plugin.log';
if ( file_exists( $log_file ) ) {
unlink( $log_file ); // Delete the log file
}
}
});
Step 7: Advanced Features
Monolog supports additional advanced features like:
Logging to external services (e.g., Slack, Sentry).
Adding custom formatters and processors for more detailed logs.
Handling asynchronous log writes for performance.
Example: Logging to Slack
use Monolog\Handler\SlackWebhookHandler;
$slackHandler = new SlackWebhookHandler('your-slack-webhook-url', '#logs', 'LoggerBot', true, null, Logger::ERROR);
Your_Plugin_Logger::get_logger()->pushHandler($slackHandler);
Your_Plugin_Logger::error('An error occurred that requires attention');
Best Practices
Log Only When Necessary: Avoid excessive logging in production environments.
Secure Logs: Ensure sensitive data isn’t exposed in log files.
Monitor Log File Size: Regularly clean up or rotate logs to prevent bloating.
Conclusion
Integrating Monolog into your WordPress plugin using the WPPB boilerplate allows you to manage logging efficiently and keep your plugin's debugging and monitoring structured. With its rich feature set, Monolog ensures you can focus on improving your plugin’s functionality while keeping potential issues in check.
Start logging smarter today, and let Monolog simplify your development process!
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
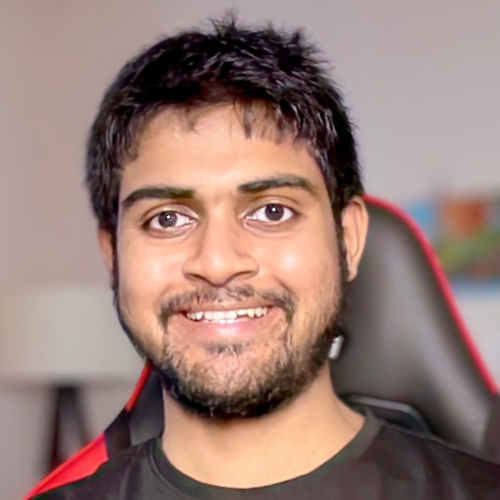
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.