A Guide to Go for Python, PHP, and Node.js Developers
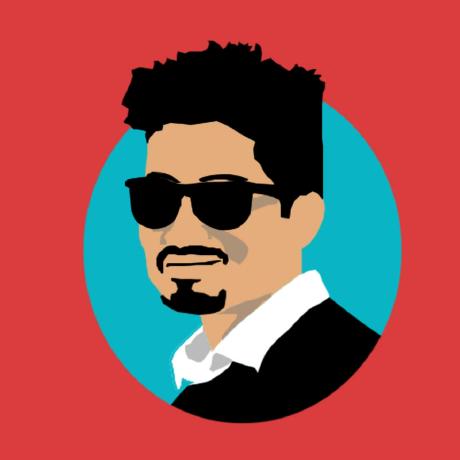
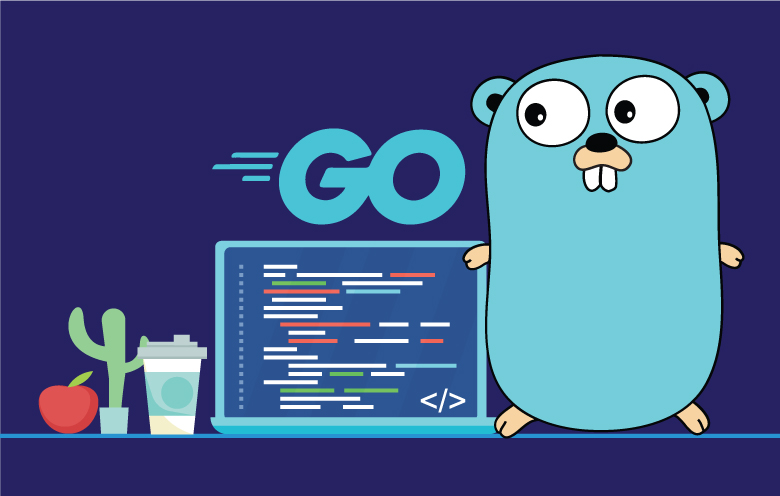
Why Go?
1. A Brief History
Go was created at Google in 2007 by a team led by Robert Griesemer, Rob Pike, and Ken Thompson. They designed it to address the challenges of large-scale software development, combining the simplicity and speed of dynamic languages like Python with the performance and type safety of statically typed languages like C++. Officially released in 2009, Go has since become one of the fastest-growing programming languages, renowned for its clean design, high performance, and ability to scale effortlessly.
2. Philosophy
The philosophy of Go is grounded in simplicity, readability, and productivity:
Simplicity: The language minimizes complexity, eliminating features like inheritance, implicit type conversions, and overloading, which can lead to subtle bugs in other languages.
Explicitness: Go avoids “magic” (hidden behaviors or implicit configurations). Developers write clear, predictable code.
Concurrency as a Core Feature: Unlike many languages that bolt on concurrency features, Go integrates goroutines and channels into its core.
Developer Productivity: Tools like
gofmt
ensure consistent code formatting, while the standard library provides robust out-of-the-box functionality.
3. Where Go Shines
Concurrency: Go's lightweight goroutines and channels simplify parallel programming, making it ideal for building high-performance, concurrent systems.
Speed: Its compiled nature and minimal runtime overhead deliver near-native performance.
Scalability: Built to handle modern, distributed systems, Go excels at building microservices and APIs.
Cross-Platform Binaries: Go generates standalone binaries, reducing deployment complexities.
Comparisons: Go vs. Python Django, PHP Laravel, Node.js Express
1. Language Design
Aspect | Go | Python Django | PHP Laravel | Node.js Express |
Typing | Statically typed | Dynamically typed | Dynamically typed | Dynamically typed |
Compilation | Compiled | Interpreted | Interpreted | Interpreted |
Concurrency Model | Goroutines and channels | Threads (limited async support) | Threads | Event loop (async by default) |
Error Handling | Explicit error return values | Exceptions | Exceptions | Exceptions |
Performance | High (compiled, low overhead) | Medium (interpreted) | Medium (interpreted) | Medium-High (non-blocking I/O) |
2. Development Workflow
Go emphasizes simplicity and minimalism, with fewer decisions about libraries or frameworks.
Django and Laravel come with batteries included, providing ORM, templating, and middleware out of the box.
Node.js Express offers flexibility but requires additional setup for routing, ORM, and other features.
3. Strengths
Go: Speed, concurrency, scalability, and a robust standard library for web development and networking.
Django: Rapid prototyping and development with a rich ecosystem.
Laravel: Elegant syntax and extensive tooling for web apps.
Node.js: Real-time apps with non-blocking I/O, such as chat and streaming services.
Use Cases and Industries Where Go is Prevalent
Web Development and APIs:
Go is a popular choice for building RESTful and gRPC APIs due to its performance and concurrency model.
Frameworks like Gin and Echo simplify web development, while Go's native
net/http
package provides low-level control.
Examples:
Netflix uses Go for high-performance, lightweight APIs.
Uber leverages Go for its geofencing and dispatch systems.
Cloud-Native and Microservices:
Designed for distributed systems, Go powers many cloud-native tools, including Docker, Kubernetes, and Prometheus.
Its speed and scalability make it ideal for microservice architectures.
Examples:
Kubernetes, the leading container orchestration tool, is written in Go.
DigitalOcean's internal services use Go for scalability and performance.
DevOps and Infrastructure:
- Go is widely used in DevOps tools due to its portability and performance. Standalone binaries reduce deployment dependencies.
Examples:
Terraform (infrastructure as code) and Vault (secrets management) are written in Go.
Docker’s core components are implemented in Go.
Networking and Real-Time Systems:
- Go’s efficient concurrency and low-latency networking capabilities make it a favorite for building real-time systems like chat apps, gaming servers, and financial applications.
Examples:
Discord uses Go for its real-time messaging backend.
Cloudflare employs Go for its high-performance web security services.
Data Processing and Streaming:
- Go’s simplicity and speed make it suitable for building scalable data pipelines and streaming applications.
Examples:
- InfluxDB (time-series database) and Telegraf (metric collection) are written in Go.
FinTech and Blockchain:
- The performance and efficiency of Go align well with the needs of financial systems and blockchain networks.
Examples:
Go is the core language behind Ethereum's implementation (Geth).
PayPal uses Go for parts of its payment systems.
Why Choose Go for Your Next Project?
Simplicity: Reduces cognitive load, making development fast and predictable.
Scalability: Handles millions of concurrent connections with minimal overhead.
Cross-Platform: Write once, compile anywhere.
Community and Ecosystem: A growing community and ecosystem provide extensive resources and libraries.
In this guide, you'll explore Go from every angle:
Basics: Learn Go’s syntax, core concepts, and idioms.
Advanced Features: Dive into concurrency, error handling, and memory management.
Ecosystem: Discover tools, libraries, and frameworks to supercharge your development.
Transition Tips: Map your existing knowledge to Go's features, ensuring a smooth learning curve.
Practical Applications: Build real-world projects to solidify your skills.
DevOps and Deployment: Learn how to take your Go applications from development to production.
Chapter 1: Getting Started with Go
1.1 Setting Up the Go Environment
Installation:
Download and install Go from golang.org.
Verify installation:
go version
Add Go to your PATH and set up
GOPA
TH
(if necessary).
IDEs and Editors:
Recommended editors:
VS Code: Popular for Go development. Install the Go plugin for enhanced features.
GoLand: A robust IDE for Go by JetBrains.
Lite IDE: Lightweight alternative specific to Go.
Setting up linting and formatting:
Install extensions like
go
langci-lin
t
for linting.Enable
gofmt
orgoimports
to format your code on save.
Testing Your Environment:
Create a
hello.go
file:package main import "fmt" func main() { fmt.Println("Hello, World!") }
Run it:
go run hello.go
1.2 Understanding Go's Workspace
GOPATH and GOROOT:
GOROOT
: The location where Go is installed.GOPATH
: Your workspace directory. Contains:src
: Your Go source code.bin
: Compiled binaries.pkg
: Package object files.
Check:
go env
Using Modules:
1.3 Writing Your First Go Program
Basics of the
main
Package:Every Go program starts with the
main
package.
Your First Web Server:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, %s!", r.URL.Path[1:]) } func main() { http.HandleFunc("/", handler) fmt.Println("Server starting at http://localhost:8080") http.ListenAndServe(":8080", nil) }
Run with:
go run main.go
Access at
http://localhost:8080
.
1.4 The Go CLI
Key Commands:
Run your Go file:
go run filename.go
Build an executable:
go build filename.go
Test your application:
go test ./...
Install binaries:
go install
Managing Dependencies:
Fetching dependencies:
go get module_name
Tidy up unused dependencies:
go mod tidy
Creating a Binary:
Chapter 2: Language Basics
2.1 Variables and Constants
Theory:
Variables in Go are explicitly typed or inferred. Variables declared but not used result in a compile-time error, which enforces clean code.
Constants are immutable and evaluated at compile time. They can only be of basic types (
bool
,string
, or numeric types).
Practical Examples:
Variable Declaration:
// Explicit declaration var x int = 10 // Inferred type y := "Hello, Go!" // Zero values var z int // Defaults to 0 var name string // Defaults to an empty string
Constants:
const ( Pi = 3.14159 Language = "Go" ) fmt.Printf("Pi: %.2f, Language: %s\n", Pi, Language)
Enumerated Constants:
const ( Red = iota Green Blue ) fmt.Println(Red, Green, Blue) // Outputs: 0 1 2
2.2 Data Types
Theory:
Go has simple and powerful type systems with no implicit conversions. This prevents accidental loss of data or unexpected behaviors.
Composite types like slices and maps are designed for efficient memory usage and safety.
Practical Examples:
Basic Types:
var age int = 30 var weight float64 = 72.5 var isEmployed bool = true fmt.Printf("Age: %d, Weight: %.1f, Employed: %t\n", age, weight, isEmployed)
Strings:
name := "John Doe" fmt.Printf("Name: %s, Length: %d\n", name, len(name))
Composite Types:
Arrays:
nums := [3]int{10, 20, 30} fmt.Println(nums)
Slices:
nums := []int{1, 2, 3} nums = append(nums, 4) fmt.Println(nums) // Outputs: [1 2 3 4]
Maps:
scores := map[string]int{"Alice": 95, "Bob": 90} scores["Charlie"] = 85 fmt.Println(scores)
2.3 Operators
Theory:
- Operators are concise tools to manipulate data. Arithmetic, comparison, and logical operators perform operations, while bitwise operators manipulate individual bits.
Practical Examples:
Arithmetic Operators:
a, b := 10, 3 fmt.Println("Add:", a+b) fmt.Println("Sub:", a-b) fmt.Println("Mul:", a*b) fmt.Println("Div:", a/b) fmt.Println("Mod:", a%b)
Logical Operators:
a, b := true, false fmt.Println("AND:", a && b) fmt.Println("OR:", a || b) fmt.Println("NOT:", !a)
2.4 Control Structures
Theory:
Go has no
while
ordo-while
loops; instead, thefor
loop is used universally.The
switch
statement avoids cascading by default, reducing bugs.
Practical Examples:
For Loop:
for i := 0; i < 5; i++ { fmt.Println(i) }
Infinite Loop:
count := 0 for { count++ if count == 5 { break } } fmt.Println("Count reached:", count)
Switch with Multiple Conditions:
day := "Tuesday" switch day { case "Monday", "Tuesday", "Wednesday": fmt.Println("Weekday") case "Saturday", "Sunday": fmt.Println("Weekend") default: fmt.Println("Invalid day") }
2.5 Functions and Error Handling
Theory:
Functions are first-class citizens in Go. They support closures, variadic parameters, and multiple return values.
Error handling is explicit. Go emphasizes handling errors locally.
Practical Examples:
Named Return Values:
func rectangleProperties(length, width int) (area, perimeter int) { area = length * width perimeter = 2 * (length + width) return } a, p := rectangleProperties(5, 3) fmt.Printf("Area: %d, Perimeter: %d\n", a, p)
Variadic Functions:
func sum(nums ...int) int { total := 0 for _, n := range nums { total += n } return total } fmt.Println("Sum:", sum(1, 2, 3, 4, 5)) // Outputs: Sum: 15
Error Handling:
func divide(a, b int) (int, error) { if b == 0 { return 0, fmt.Errorf("cannot divide by zero") } return a / b, nil } result, err := divide(10, 2) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Result:", result) }
2.6 Structs and Interfaces
Theory:
Structs are custom data types that group fields together. They enable object-like programming.
Interfaces define behavior and enable polymorphism. They are satisfied implicitly, reducing boilerplate.
Practical Examples:
Structs:
type Car struct { Brand string Model string Year int } car := Car{Brand: "Toyota", Model: "Corolla", Year: 2022} fmt.Printf("%s %s (%d)\n", car.Brand, car.Model, car.Year)
Interfaces:
type Shape interface { Area() float64 } type Rectangle struct { Length, Width float64 } func (r Rectangle) Area() float64 { return r.Length * r.Width } var s Shape = Rectangle{Length: 5, Width: 3} fmt.Println("Area:", s.Area())
2.7 Pointers
Theory:
- Pointers in Go hold the memory address of a value. They are a powerful way to share data between functions.
Practical Examples:
Passing by Reference:
func doubleValue(x *int) { *x = *x * 2 } value := 10 doubleValue(&value) fmt.Println("Doubled value:", value) // Outputs: 20
Nil Checks:
var ptr *int if ptr == nil { fmt.Println("Pointer is nil") }
Chapter 3: Advanced Language Features
3.1 Goroutines and Concurrency Patterns
Theory:
Go's concurrency model is based on goroutines and channels, following the Communicating Sequential Processes (CSP) paradigm.
Goroutines are lightweight threads managed by the Go runtime. They share memory by communicating rather than sharing directly.
Practical Examples:
Creating a Goroutine:
package main import ( "fmt" "time" ) func sayHello() { for i := 0; i < 5; i++ { fmt.Println("Hello") time.Sleep(100 * time.Millisecond) } } func main() { go sayHello() fmt.Println("Main function running") time.Sleep(1 * time.Second) // Wait to observe goroutine output }
Waiting for Goroutines with
sync.WaitGroup
:package main import ( "fmt" "sync" ) func worker(id int, wg *sync.WaitGroup) { defer wg.Done() fmt.Printf("Worker %d started\n", id) fmt.Printf("Worker %d finished\n", id) } func main() { var wg sync.WaitGroup for i := 1; i <= 3; i++ { wg.Add(1) go worker(i, &wg) } wg.Wait() fmt.Println("All workers done") }
3.2 Channels and Select Statements
Theory:
Channels are conduits for communication between goroutines.
select
allows goroutines to wait on multiple channel operations.
Practical Examples:
Basic Channel Usage:
package main import "fmt" func main() { messages := make(chan string) go func() { messages <- "Hello from Goroutine" }() msg := <-messages fmt.Println(msg) }
Buffered Channels:
package main import "fmt" func main() { messages := make(chan string, 2) messages <- "Buffered" messages <- "Channel" fmt.Println(<-messages) fmt.Println(<-messages) }
Select Statement:
package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(1 * time.Second) ch1 <- "Message from channel 1" }() go func() { time.Sleep(2 * time.Second) ch2 <- "Message from channel 2" }() for i := 0; i < 2; i++ { select { case msg1 := <-ch1: fmt.Println(msg1) case msg2 := <-ch2: fmt.Println(msg2) } } }
3.3 The sync
and context
Packages
Theory:
The
sync
package provides tools for synchronization, such as mutexes and wait groups.The
context
package allows you to control and cancel goroutines across API boundaries.
Practical Examples:
Using Mutexes:
package main import ( "fmt" "sync" ) var counter int var mutex sync.Mutex func increment(wg *sync.WaitGroup) { defer wg.Done() mutex.Lock() counter++ mutex.Unlock() } func main() { var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go increment(&wg) } wg.Wait() fmt.Println("Final Counter:", counter) }
Using Context for Cancellation:
package main import ( "context" "fmt" "time" ) func main() { ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second) defer cancel() go func(ctx context.Context) { for { select { case <-ctx.Done(): fmt.Println("Goroutine stopped") return default: fmt.Println("Working...") time.Sleep(500 * time.Millisecond) } } }(ctx) time.Sleep(3 * time.Second) fmt.Println("Main function done") }
3.4 Reflection and Type Assertions
Theory:
Reflection allows inspecting and manipulating variables at runtime. It's useful for generic programming but should be used sparingly due to complexity.
Type assertions enable extracting concrete types from interfaces.
Practical Examples:
Reflection:
package main import ( "fmt" "reflect" ) func main() { var x float64 = 3.14 t := reflect.TypeOf(x) v := reflect.ValueOf(x) fmt.Println("Type:", t) fmt.Println("Value:", v) }
Type Assertion:
package main import "fmt" func describe(i interface{}) { if v, ok := i.(string); ok { fmt.Println("String:", v) } else { fmt.Println("Not a string") } } func main() { describe("Hello") describe(42) }
3.5 Error Handling Best Practices
Theory:
Go encourages explicit error handling, avoiding exceptions and panics for recoverable conditions.
Custom error types enhance clarity and debugging.
Practical Examples:
Custom Error Types:
package main import ( "fmt" ) type CustomError struct { Code int Message string } func (e CustomError) Error() string { return fmt.Sprintf("Error %d: %s", e.Code, e.Message) } func doSomething(flag bool) error { if !flag { return CustomError{Code: 404, Message: "Resource not found"} } return nil } func main() { err := doSomething(false) if err != nil { fmt.Println(err) } }
Chapter 4: Ecosystem Overview
4.1 Essential Libraries and Frameworks for Web Development
Theory:
The
net/http
package is part of the Go standard library and provides the fundamental tools to build HTTP servers and clients.Third-party frameworks like
Gin
,Echo
, andFiber
offer additional abstractions, such as routing, middleware, and JSON handling, making it easier to build robust APIs.Choosing a framework:
Gin
: Lightweight, high performance, and ideal for REST APIs.Echo
: Similar to Gin but with more comprehensive features like built-in support for middleware.Fiber
: Inspired by Express.js, making it a great fit for developers transitioning from Node.js.
Practical Examples:
Using
net/http
:Building a simple HTTP server:
package main import ( "fmt" "net/http" ) func helloHandler(w http.ResponseWriter, r *http.Request) { w.Header().Set("Content-Type", "application/json") fmt.Fprintf(w, `{"message": "Hello, %s!"}`, r.URL.Path[1:]) } func main() { http.HandleFunc("/", helloHandler) fmt.Println("Server running at http://localhost:8080") http.ListenAndServe(":8080", nil) }
Pros: Fine-grained control.
Cons: Manual handling of routing, middleware, and response formatting.
Using Gin for a REST API:
Installing Gin:
go get -u github.com/gin-gonic/gin
Example REST API:
package main import ( "github.com/gin-gonic/gin" ) type User struct { ID int `json:"id"` Name string `json:"name"` Email string `json:"email"` } var users = []User{ {ID: 1, Name: "Alice", Email: "alice@example.com"}, {ID: 2, Name: "Bob", Email: "bob@example.com"}, } func main() { router := gin.Default() router.GET("/users", func(c *gin.Context) { c.JSON(200, users) }) router.POST("/users", func(c *gin.Context) { var newUser User if err := c.ShouldBindJSON(&newUser); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } newUser.ID = len(users) + 1 users = append(users, newUser) c.JSON(201, newUser) }) router.Run(":8080") }
Pros: Clear syntax and built-in JSON parsing.
Cons: Dependency on third-party libraries.
4.2 Database Integration
Theory:
Go's
database/sql
package is database-agnostic, relying on drivers for specific databases (e.g., PostgreSQL, MySQL).ORMs like
GORM
simplify database interactions by abstracting SQL queries into high-level methods.
Practical Examples:
Using
database/sql
with PostgreSQL:package main import ( "database/sql" "fmt" _ "github.com/lib/pq" ) func main() { connStr := "user=username password=password dbname=mydb sslmode=disable" db, err := sql.Open("postgres", connStr) if err != nil { panic(err) } defer db.Close() query := "INSERT INTO users (name, email) VALUES ($1, $2)" _, err = db.Exec(query, "Alice", "alice@example.com") if err != nil { panic(err) } rows, err := db.Query("SELECT id, name, email FROM users") if err != nil { panic(err) } defer rows.Close() for rows.Next() { var id int var name, email string rows.Scan(&id, &name, &email) fmt.Printf("ID: %d, Name: %s, Email: %s\n", id, name, email) } }
Using GORM for Simplified Queries:
package main import ( "gorm.io/driver/sqlite" "gorm.io/gorm" ) type Product struct { ID uint Name string Price float64 } func main() { db, err := gorm.Open(sqlite.Open("test.db"), &gorm.Config{}) if err != nil { panic("failed to connect database") } db.AutoMigrate(&Product{}) db.Create(&Product{Name: "Laptop", Price: 1500.00}) var product Product db.First(&product, 1) fmt.Println(product) }
4.3 Testing and Debugging
Theory:
Testing in Go is built into the language, using the
testing
package.Debugging tools like
delve
allow breakpoints and step execution.Profiling with
pprof
helps optimize performance.
Practical Examples:
Unit Testing:
Example test file (
main_test.go
):package main import "testing" func Add(a, b int) int { return a + b } func TestAdd(t *testing.T) { result := Add(2, 3) if result != 5 { t.Errorf("expected 5, got %d", result) } }
Run tests:
go test ./...
Debugging with Delve:
Start a debug session:
dlv debug main.go
Profiling with
pprof
:Enable profiling:
import _ "net/http/pprof" go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }()
Run:
go tool pprof http://localhost:6060/debug/pprof/profile
4.4 Common Tools in the Go Ecosystem
Theory:
Go’s ecosystem thrives on simplicity. Key tools include:
go mod
for dependency management.gofmt
for code formatting.golangci-lint
for linting.staticcheck
for code analysis.
Practical Examples:
Dependency Management:
go mod init myproject go get github.com/gin-gonic/gin
Code Formatting:
go fmt ./...
Static Code Analysis:
staticcheck ./...
4.5 Building APIs with OpenAPI/Swagger
Theory:
OpenAPI simplifies API documentation, making it machine-readable.
Tools like
swaggo
automatically generate OpenAPI specifications from annotated Go code.
Practical Examples:
Installing Swaggo:
go install github.com/swaggo/swag/cmd/swag swag init
Annotated Code:
// @Summary Add a new user // @Description Add a new user to the system // @Accept json // @Produce json // @Param user body User true "User data" // @Success 200 {object} User // @Failure 400 {object} Error // @Router /users [post]
Chapter 5: Building a Web Application in Go
5.1 Project Structure
Theory:
A structured project layout is critical for scalability, maintainability, and readability.
Following conventions like the Standard Go Project Layout simplifies onboarding for new developers.
Practical Example:
Recommended Project Layout:
myapp/ ├── cmd/ # Entry points │ └── main.go # Main application file ├── config/ # Configuration and environment files │ └── config.go ├── internal/ # Private application code │ ├── handlers/ # HTTP handlers │ │ ├── home.go │ │ └── user.go │ ├── middleware/ # Middleware functions │ │ └── auth.go │ ├── models/ # Database models │ │ └── user.go │ ├── repositories/ # Database queries │ │ └── user_repo.go │ ├── services/ # Business logic │ │ └── user_service.go │ └── utils/ # Helper functions ├── pkg/ # Public reusable components ├── test/ # Unit and integration tests ├── web/ # Frontend assets (if applicable) ├── go.mod # Dependency management file └── README.md # Project documentation
Example Config File (
config/config.go
):package config import ( "log" "os" ) type Config struct { DBUser string DBPassword string DBName string } func LoadConfig() *Config { dbUser := os.Getenv("DB_USER") if dbUser == "" { log.Fatal("DB_USER is not set") } return &Config{ DBUser: dbUser, DBPassword: os.Getenv("DB_PASSWORD"), DBName: os.Getenv("DB_NAME"), } }
5.2 Routing
Theory:
Routing defines how incoming requests are matched to specific handlers.
A robust routing system supports path parameters, query strings, and complex URL structures.
Practical Examples:
Using
net/http
:package main import ( "fmt" "net/http" ) func main() { mux := http.NewServeMux() mux.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Welcome to MyApp!") }) mux.HandleFunc("/users", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "User List") }) fmt.Println("Server running on :8080") http.ListenAndServe(":8080", mux) }
Advanced Routing with
httprouter
:package main import ( "fmt" "net/http" "github.com/julienschmidt/httprouter" ) func UserDetail(w http.ResponseWriter, r *http.Request, ps httprouter.Params) { id := ps.ByName("id") fmt.Fprintf(w, "User ID: %s\n", id) } func main() { router := httprouter.New() router.GET("/users/:id", UserDetail) fmt.Println("Server running on :8080") http.ListenAndServe(":8080", router) }
Using
Gin
for Cleaner Syntax:r := gin.Default() r.GET("/users", handlers.GetUsers) r.POST("/users", handlers.CreateUser) r.Run(":8080")
5.3 Middleware
Theory:
Middleware processes requests and responses, adding functionality such as:
Logging.
Authentication and authorization.
CORS (Cross-Origin Resource Sharing) headers.
Rate limiting.
Practical Examples:
Basic Logging Middleware:
func LoggingMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { log.Printf("Request: %s %s", r.Method, r.URL.Path) next.ServeHTTP(w, r) }) }
Authentication Middleware:
func AuthMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { token := r.Header.Get("Authorization") if token != "valid-token" { http.Error(w, "Forbidden", http.StatusForbidden) return } next.ServeHTTP(w, r) }) }
Applying Middleware in Gin:
func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { token := c.GetHeader("Authorization") if token != "valid-token" { c.AbortWithStatusJSON(http.StatusUnauthorized, gin.H{"error": "unauthorized"}) return } c.Next() } } r := gin.Default() r.Use(AuthMiddleware()) r.GET("/secure", secureHandler)
5.4 Interacting with Databases
Theory:
A robust application should have clear layers:
Repository: Handles database queries.
Service: Encapsulates business logic.
Use transactions for complex operations to ensure consistency.
Practical Examples:
Repository Pattern with
sqlx
:type UserRepository struct { db *sqlx.DB } func (ur *UserRepository) GetUserByID(id int) (*User, error) { var user User err := ur.db.Get(&user, "SELECT id, name, email FROM users WHERE id = ?", id) return &user, err }
Service Layer Example:
type UserService struct { repo *UserRepository } func (us *UserService) GetUserDetails(id int) (*User, error) { return us.repo.GetUserByID(id) }
5.5 Building RESTful APIs
Theory:
Follow REST principles:
Use HTTP verbs (
GET
,POST
,PUT
,DELETE
).Use proper status codes (
200 OK
,404 Not Found
).Serialize responses as JSON.
Practical Examples:
CRUD Operations with Gin:
r.GET("/users", func(c *gin.Context) { users := []User{{ID: 1, Name: "Alice"}} c.JSON(http.StatusOK, users) }) r.POST("/users", func(c *gin.Context) { var user User if err := c.ShouldBindJSON(&user); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } user.ID = 2 c.JSON(http.StatusCreated, user) })
5.6 Testing the Application
Theory:
Tests ensure your application meets requirements and prevent regressions.
Types of tests:
Unit Tests: Test individual functions.
Integration Tests: Test multiple components together.
Practical Examples:
Testing Handlers:
req := httptest.NewRequest("GET", "/users", nil) w := httptest.NewRecorder() handlers.GetUsers(w, req) if w.Code != http.StatusOK { t.Fatalf("Expected status OK, got %v", w.Code) }
5.7 Deployment
Theory:
Use Docker for consistent deployment across environments.
CI/CD pipelines automate testing, building, and deployment.
Practical Examples:
Dockerizing a Go Application:
FROM golang:1.20 WORKDIR /app COPY go.mod go.sum ./ RUN go mod download COPY . . RUN go build -o main . CMD ["./main"]
Deploying with Kubernetes:
apiVersion: apps/v1 kind: Deployment metadata: name: myapp spec: replicas: 2 template: spec: containers: - name: myapp image: myapp:latest ports: - containerPort: 8080
Chapter 6: Performance and Optimization
This chapter dives into optimizing Go applications for performance, scalability, and efficient resource utilization. Go’s lightweight design and concurrency primitives make it ideal for high-performance applications, but leveraging these effectively requires thoughtful design and practices.
6.1 Profiling and Benchmarking
Theory:
Profiling identifies bottlenecks in CPU, memory, and goroutine usage.
Benchmarking measures the performance of specific functions or code blocks under controlled conditions.
Tools:
Built-in:
pprof
,testing
.External:
benchstat
,go-torch
.
Practical Examples:
Benchmarking a Function:
package main import ( "math/rand" "testing" ) func BubbleSort(nums []int) { n := len(nums) for i := 0; i < n; i++ { for j := 0; j < n-i-1; j++ { if nums[j] > nums[j+1] { nums[j], nums[j+1] = nums[j+1], nums[j] } } } } func BenchmarkBubbleSort(b *testing.B) { for i := 0; i < b.N; i++ { nums := rand.Perm(1000) BubbleSort(nums) } }
Profiling with
pprof
:Enable profiling:
import _ "net/http/pprof" go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }()
Run the profiler:
go tool pprof http://localhost:6060/debug/pprof/profile
Visualizing with
go-torch
:Install:
go install github.com/uber/go-torch
Generate flame graphs:
go-torch -u http://localhost:6060/debug/pprof/profile
6.2 Writing Efficient Goroutines
Theory:
Goroutines are lightweight but can lead to high memory usage and context-switching overhead if mismanaged.
Best practices:
Limit the number of goroutines based on available CPU cores.
Avoid unbounded goroutines (e.g., in infinite loops).
Use worker pools to manage concurrency effectively.
Practical Examples:
Using Worker Pools:
package main import ( "fmt" "sync" ) func worker(id int, jobs <-chan int, results chan<- int, wg *sync.WaitGroup) { defer wg.Done() for job := range jobs { fmt.Printf("Worker %d processing job %d\n", id, job) results <- job * 2 } } func main() { const numWorkers = 3 jobs := make(chan int, 10) results := make(chan int, 10) var wg sync.WaitGroup for i := 1; i <= numWorkers; i++ { wg.Add(1) go worker(i, jobs, results, &wg) } for j := 1; j <= 10; j++ { jobs <- j } close(jobs) wg.Wait() close(results) for result := range results { fmt.Println("Result:", result) } }
Preventing Goroutine Leaks:
Ensure all goroutines have proper exit conditions.
Use
context.Context
for cancellation.
6.3 Memory Management
Theory:
Go uses garbage collection (GC), but developers should still minimize unnecessary memory allocation and retention.
Tools like
pprof
andheapdump
help identify memory issues.
Practical Examples:
Avoiding Memory Leaks:
package main import "fmt" func main() { ch := make(chan int) go func() { for i := 0; i < 10; i++ { ch <- i } close(ch) }() for val := range ch { fmt.Println(val) } }
Using Sync Pools:
import "sync" var bufferPool = sync.Pool{ New: func() interface{} { return make([]byte, 1024) }, } func main() { buf := bufferPool.Get().([]byte) defer bufferPool.Put(buf) // Use buf }
6.4 Optimizing I/O Operations
Theory:
Reduce disk and network latency by:
Using buffered readers and writers.
Caching frequently accessed data.
Using asynchronous I/O where possible.
Practical Examples:
Buffered File Reading:
package main import ( "bufio" "fmt" "os" ) func main() { file, err := os.Open("largefile.txt") if err != nil { panic(err) } defer file.Close() scanner := bufio.NewScanner(file) for scanner.Scan() { fmt.Println(scanner.Text()) } if err := scanner.Err(); err != nil { panic(err) } }
Caching with
sync.Map
:evar cache sync.Map func GetFromCache(key string) (string, bool) { if value, ok := cache.Load(key); ok { return value.(string), true } return "", false } func SetToCache(key, value string) { cache.Store(key, value) }
6.5 Avoiding Common Pitfalls
Blocking Channels:
Always ensure channels have consumers before sending data.
Use buffered channels to avoid blocking producers.
Global State:
Avoid mutable global variables to prevent race conditions.
Use
sync.Mutex
orsync.RWMutex
for safe concurrent access.
Excessive Use of Reflection:
- Reflection is slow and should be avoided in performance-critical code.
6.6 Observability and Monitoring
Theory:
Observability tools help track application health and performance in production.
Use tools like
Prometheus
,Grafana
, andElastic Stack
for monitoring metrics and logs.
Practical Examples:
Prometheus Metrics with
prometheus/client_golang
:package main import ( "net/http" "github.com/prometheus/client_golang/prometheus" "github.com/prometheus/client_golang/prometheus/promhttp" ) var opsProcessed = prometheus.NewCounter(prometheus.CounterOpts{ Name: "ops_processed_total", Help: "The total number of processed events", }) func main() { prometheus.MustRegister(opsProcessed) http.Handle("/metrics", promhttp.Handler()) go func() { for { opsProcessed.Inc() time.Sleep(1 * time.Second) } }() http.ListenAndServe(":8080", nil) }
Structured Logging with
logrus
:import log "github.com/sirupsen/logrus" func main() { log.WithFields(log.Fields{ "user_id": 1234, "action": "login", }).Info("User action logged") }
Chapter 7: Deployment and DevOps with Go
This chapter provides a guide to deploying Go applications, integrating DevOps practices, and ensuring applications are production-ready.
7.1 Building Production-Ready Binaries
Theory:
Go’s static compilation produces self-contained binaries, simplifying deployment across platforms.
Cross-compilation allows generating binaries for different operating systems and architectures from any host machine.
Hands-On Examples:
Basic Binary Compilation:
go build -o myapp main.go ./myapp
- Produces a
myapp
executable for your local OS and architecture.
- Produces a
Cross-Compiling for Multiple Targets:
# Compile for Linux on AMD64 GOOS=linux GOARCH=amd64 go build -o myapp-linux main.go # Compile for Windows on AMD64 GOOS=windows GOARCH=amd64 go build -o myapp-windows.exe # Compile for macOS on ARM64 GOOS=darwin GOARCH=arm64 go build -o myapp-macos main.go
- Useful when building on a CI/CD pipeline for diverse deployment targets.
Optimized Compilation for Smaller Binaries:
go build -ldflags="-s -w" -o myapp main.go
-s
: Strips the symbol table.-w
: Excludes debug information.
Embedding Build Information:
go build -ldflags="-X 'main.version=1.0.0' -X 'main.buildTime=$(date -u)'"
Code to read these values:
package main import "fmt" var version string var buildTime string func main() { fmt.Printf("Version: %s, Build Time: %s\n", version, buildTime) }
7.2 Dockerizing a Go Application
Theory:
Docker simplifies packaging and deployment by creating consistent environments.
Multi-stage builds reduce the image size and improve runtime performance.
Hands-On Examples:
Basic Dockerfile:
FROM golang:1.20 as builder WORKDIR /app COPY . . RUN go build -o myapp FROM alpine:latest WORKDIR /root/ COPY --from=builder /app/myapp . CMD ["./myapp"]
Running the Docker Image:
docker build -t myapp . docker run -p 8080:8080 myapp
Multi-Stage Build to Include Dependencies:
FROM golang:1.20 as builder WORKDIR /app COPY go.mod go.sum ./ RUN go mod download COPY . . RUN go build -o myapp FROM alpine:latest RUN apk --no-cache add ca-certificates WORKDIR /root/ COPY --from=builder /app/myapp . CMD ["./myapp"]
Using Docker Compose:
version: "3.8" services: app: build: . ports: - "8080:8080" environment: - PORT=8080 - ENV=production
Start with:
docker-compose up --build
7.3 Cloud Deployment
Theory:
Cloud platforms provide scalability, managed services, and global availability.
Popular platforms:
AWS: EC2, Elastic Beanstalk, Fargate.
GCP: App Engine, Cloud Run.
Heroku: Simplified deployment for small apps.
DigitalOcean: Affordable hosting with Kubernetes support.
Hands-On Examples:
Deploying on AWS EC2:
Launch an EC2 instance with Docker installed:
sudo yum update -y sudo yum install docker -y sudo service docker start
Copy your Docker image or pull it from Docker Hub:
docker pull yourdockerhubusername/myapp:latest docker run -p 8080:8080 myapp
Using AWS Elastic Beanstalk:
Create an Elastic Beanstalk application.
Zip your application directory:
zip app.zip Dockerfile main.go
Deploy using the AWS CLI:
eb init -p docker myapp eb create myapp-env
Deploying on Heroku:
Initialize Heroku for your project:
heroku create
Add a
Procfile
:web: ./myapp
Push to Heroku:
git push heroku main
7.4 CI/CD Pipelines
Theory:
CI/CD pipelines automate the testing, building, and deployment of applications.
Popular tools:
GitHub Actions: Integrated with GitHub repositories.
GitLab CI/CD: Highly customizable.
CircleCI: Easy integration with Docker and Kubernetes.
Hands-On Examples:
GitHub Actions Pipeline:
name: Go CI/CD on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up Go uses: actions/setup-go@v2 with: go-version: 1.20 - name: Build run: go build -o myapp - name: Test run: go test ./... - name: Docker Build and Push uses: docker/build-push-action@v3 with: push: true tags: yourdockerhubusername/myapp:latest
GitLab CI/CD Pipeline:
stages: - build - test - deploy build: stage: build script: - go build -o myapp test: stage: test script: - go test ./... deploy: stage: deploy script: - docker build -t yourdockerhubusername/myapp . - docker push yourdockerhubusername/myapp
7.5 Observability and Monitoring
Theory:
Observability tools help monitor performance, trace errors, and identify bottlenecks in production.
Popular tools:
Prometheus: Metrics collection.
Grafana: Visualization.
OpenTelemetry: Distributed tracing.
Hands-On Examples:
Using Prometheus for Metrics:
import ( "github.com/prometheus/client_golang/prometheus" "github.com/prometheus/client_golang/prometheus/promhttp" ) var requests = prometheus.NewCounterVec( prometheus.CounterOpts{ Name: "http_requests_total", Help: "Total HTTP requests", }, []string{"method", "endpoint"}, ) func main() { prometheus.MustRegister(requests) http.Handle("/metrics", promhttp.Handler()) http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { requests.WithLabelValues(r.Method, "/").Inc() w.Write([]byte("Hello, Prometheus!")) }) http.ListenAndServe(":8080", nil) }
Setting Up Logging with
logrus
:import log "github.com/sirupsen/logrus" func main() { log.SetFormatter(&log.JSONFormatter{}) log.WithFields(log.Fields{ "user_id": 1234, "action": "login", }).Info("User logged in") }
7.6 Security Best Practices
Theory:
Secure your application and deployment by:
Using HTTPS for all communication.
Protecting sensitive data with environment variables.
Implementing rate limiting to prevent abuse.
Restricting Docker container privileges.
Hands-On Examples:
Enabling HTTPS in Go:
http.ListenAndServeTLS(":8443", "server.crt", "server.key", nil)
Securing Docker Images:
FROM golang:1.20 AS builder USER nonroot:nonroot
Using Rate Limiting with Middleware:
import "golang.org/x/time/rate" var limiter = rate.NewLimiter(1, 5) // 1 request/sec with a burst of 5 func RateLimit(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { if !limiter.Allow() { http.Error(w, "Too many requests", http.StatusTooManyRequests) return } next.ServeHTTP(w, r) }) }
Chapter 8: Transition Tips for Python, PHP, and Node.js Developers
This chapter provides resources for transitioning from Python Django, PHP Laravel, and Node.js Express to Go. It provides a smooth learning curve for adapting to Go's ecosystem while leveraging your existing knowledge.
8.1 Understanding Go’s Philosophy
Minimalism and Explicitness:
Go avoids “magic.” Explicit code enhances maintainability and predictability.
Unlike Django or Laravel, where configurations and functionality are abstracted, Go encourages explicit declarations and modular design.
Example:
Django Query:
user = User.objects.get(id=1)
Go Equivalent:
var user User err := db.First(&user, 1).Error if err != nil { fmt.Println("Error fetching user:", err) }
Concurrency as a Core Feature:
Go treats concurrency as a built-in feature with lightweight goroutines and channels.
In Python or Node.js, concurrency relies on frameworks (
asyncio
,express
).
Example:
Node.js Promise:
fetch('/api/users') .then(res => res.json()) .then(data => console.log(data));
Go Goroutine:
go func() { resp, err := http.Get("/api/users") if err == nil { body, _ := ioutil.ReadAll(resp.Body) fmt.Println(string(body)) } }()
Static Typing vs. Dynamic Typing:
Python and PHP allow flexible types but can lead to runtime errors.
Go’s static typing ensures errors are caught during compilation, not execution.
Example:
Python:
def add(x, y): return x + y print(add(2, "3")) # Runtime error
Go:
func add(x int, y int) int { return x + y } // Compile-time error if mismatched types are used fmt.Println(add(2, "3"))
8.2 Conceptual Mappings: Side-by-Side Comparisons
Feature | Python Django | PHP Laravel | Node.js Express | Go Equivalent |
Routing | Defined in urls.py | Defined in web.php | app.get('/path', ...) | net/http or Gin Router |
ORM | Django ORM | Eloquent ORM | Sequelize, Mongoose | gorm or sqlx |
Middleware | Middleware in settings | Middleware in HTTP Kernel | app.use() | Custom Middleware or Gin Middleware |
Background Jobs | Celery | Laravel Queues | bull , agenda | Goroutines with Worker Pools |
Template Engine | Django Template Engine | Blade Templates | EJS, Pug | html/template |
Dependency Injection | Django Service Layer | Service Providers | DI Libraries | wire or manual struct injection |
Error Handling | Exceptions | Try-Catch Blocks | Try-Catch | Explicit error handling (error ) |
8.3 Adapting Familiar Concepts with Go
Routing and Middleware:
Python Django Example:
from django.urls import path from .views import home, users urlpatterns = [ path("", home), path("users/", users), ]
Go Equivalent with
net/http
:package main import ( "net/http" "log" ) func homeHandler(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Welcome to Home!")) } func usersHandler(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Users List")) } func main() { http.HandleFunc("/", homeHandler) http.HandleFunc("/users", usersHandler) log.Println("Server running on http://localhost:8080") http.ListenAndServe(":8080", nil) }
ORM and Database Access:
PHP Laravel Example:
$user = DB::table('users')->where('id', 1)->first();
Go Equivalent with GORM:
var user User db.First(&user, 1) fmt.Println(user)
Error Handling:
Node.js Example:
app.get('/users', async (req, res) => { try { const users = await fetchUsers(); res.json(users); } catch (err) { res.status(500).send(err.message); } });
Go Equivalent:
func usersHandler(w http.ResponseWriter, r *http.Request) { users, err := fetchUsers() if err != nil { http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } json.NewEncoder(w).Encode(users) }
8.4 Adopting Go’s Ecosystem
Dependency Management:
Python:
pip
, PHP: Composer, Node.js:npm
.Go:
go mod
.
Example:
Python:
pip install requests
Go:
go get github.com/gin-gonic/gin
Testing:
Django:
pytest
, Laravel: PHPUnit, Node.js: Jest.Go: Built-in
testing
package.
Example:
package main
import "testing"
func TestAdd(t *testing.T) {
result := add(2, 3)
if result != 5 {
t.Errorf("Expected 5, got %d", result)
}
}
Templating:
Python Example:
<h1>Hello, {{ user.name }}!</h1>
Go Equivalent:
<h1>Hello, {{ .Name }}!</h1>
8.5 Hands-On Transition Project
Objective: Build a RESTful API with CRUD operations.
Step-by-Step Guide:
Initialize Project:
mkdir go-rest-api cd go-rest-api go mod init go-rest-api
Set Up Router:
package main import ( "github.com/gin-gonic/gin" ) type User struct { ID int `json:"id"` Name string `json:"name"` } var users = []User{{ID: 1, Name: "Alice"}, {ID: 2, Name: "Bob"}} func main() { r := gin.Default() r.GET("/users", func(c *gin.Context) { c.JSON(200, users) }) r.POST("/users", func(c *gin.Context) { var user User if err := c.ShouldBindJSON(&user); err == nil { user.ID = len(users) + 1 users = append(users, user) c.JSON(201, user) } else { c.JSON(400, gin.H{"error": err.Error()}) } }) r.Run(":8080") }
Run the API:
go run main.go
Test Endpoints:
Fetch Users:
curl http://localhost:8080/users
Add a User:
curl -X POST http://localhost:8080/users -H "Content-Type: application/json" -d '{"name":"Charlie"}'
8.6 Advanced Transition Tips
Concurrency Mastery:
Replace
async
andawait
with goroutines.Use worker pools for controlled concurrency.
Adopt Go Idioms:
Embrace Go's error-handling approach.
Avoid over-engineering; focus on simplicity.
Explore Libraries:
Gin for web frameworks.
GORM for ORMs.
Zap for structured logging.
Chapter 9: Reference Section
This chapter serves as a complete and detailed reference for Go's features, standard library, idioms, and best practices. It’s a resource for understanding Go’s ecosystem and includes cheat sheets, commonly used patterns, and curated resources.
9.1 Core Syntax Cheat Sheet
Feature | Syntax Example |
Variable Declaration | var x int = 10 |
y := 20
|
| Constants | const Pi = 3.14
|
| Functions | func add(a int, b int) int { return a + b }
|
| Loops | for i := 0; i < 10; i++ { fmt.Println(i) }
|
| Conditionals | if x > 0 { fmt.Println("Positive") }
|
| Switch | switch x { case 1: fmt.Println("One") }
|
| Structs | type User struct { Name string; Age int }
|
| Pointers | x := 10; p := &x; fmt.Println(*p)
|
| Interfaces | type Shape interface { Area() float64 }
|
| Error Handling | if err != nil { log.Fatal(err) }
|
| Concurrency | go func() { fmt.Println("Hello") }()
|
| Channels | ch := make(chan int); ch <- 42; fmt.Println(<-ch)
|
9.2 Standard Library Highlights
Commonly Used Packages:
fmt
: Formatting and printing.net/http
: HTTP servers and clients.io
: File and stream I/O.os
: Operating system interaction.encoding/json
: JSON marshaling/unmarshaling.sync
: Concurrency primitives likeMutex
.
Code Examples:
Reading a File:
data, err := os.ReadFile("example.txt") if err != nil { log.Fatal(err) } fmt.Println(string(data))
Creating an HTTP Server:
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, World!")) }) log.Fatal(http.ListenAndServe(":8080", nil))
Parsing JSON:
var user struct { Name string `json:"name"` Age int `json:"age"` } jsonData := `{"name":"Alice","age":25}` if err := json.Unmarshal([]byte(jsonData), &user); err != nil { log.Fatal(err) } fmt.Println(user.Name, user.Age)
9.3 Idiomatic Go Patterns
Error Wrapping:
import "fmt" func readFile(filename string) error { _, err := os.ReadFile(filename) if err != nil { return fmt.Errorf("failed to read file %s: %w", filename, err) } return nil }
Defer for Cleanup:
func writeFile(filename string) { file, err := os.Create(filename) if err != nil { log.Fatal(err) } defer file.Close() file.WriteString("Hello, World!") }
Using
context.Context
:import ( "context" "time" ) func main() { ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second) defer cancel() select { case <-time.After(1 * time.Second): fmt.Println("Operation completed") case <-ctx.Done(): fmt.Println("Timeout") } }
9.4 Tools and Ecosystem
Essential Tools:
go mod
: Dependency management.gofmt
: Code formatting.golangci-lint
: Linting.pprof
: Profiling.
Third-Party Libraries:
Web Frameworks:
Gin
,Echo
.Database:
GORM
,sqlx
.Logging:
logrus
,zap
.
Install Tools:
go install golang.org/x/tools/cmd/goimports@latest go install github.com/golangci/golangci-lint/cmd/golangci-lint@latest
9.5 Best Practices
Code Organization:
Keep packages small and focused.
Use
internal/
for private code andpkg/
for reusable components.
Error Handling:
- Always check errors and return meaningful messages.
Concurrency:
Avoid goroutine leaks by ensuring proper exit conditions.
Use
sync.WaitGroup
orcontext.Context
to manage goroutines.
9.6 Curated Resources
Books:
The Go Programming Language by Alan Donovan and Brian Kernighan.
Go in Action by William Kennedy.
Online Courses:
A Tour of Go: Interactive introduction.
Go by Example: Real-world examples.
Communities:
Gophers Slack.
Go Forum.
Blogs:
Dave Cheney's Blog.
Tools:
GopherJS: Compile Go to JavaScript.
GoReleaser: Simplifies release automation.
9.7 Appendix: Go Glossary
Goroutines:
- Lightweight threads managed by the Go runtime.
Channels:
- Communication mechanism between goroutines.
Interfaces:
- Define behavior without enforcing structure.
Defer:
- Ensures a function call is executed at the end of its containing function.
Zero Value:
- Default value assigned to variables when not explicitly initialized.
Chapter 10: Appendices
This chapter serves as a supplementary resource for further exploration and mastery of Go. It includes practical appendices, advanced exercises, FAQs, troubleshooting tips, and a roadmap for learning Go effectively.
10.1 Advanced Exercises and Challenges
Concurrency Challenge:
Problem: Write a program that concurrently calculates the sum of all numbers in a list using multiple goroutines.
Hints:
Split the list into chunks.
Use goroutines to process each chunk.
Aggregate results using a channel.
Solution:
package main import ( "fmt" ) func sum(nums []int, ch chan int) { total := 0 for _, n := range nums { total += n } ch <- total } func main() { nums := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10} ch := make(chan int, 2) mid := len(nums) / 2 go sum(nums[:mid], ch) go sum(nums[mid:], ch) total := <-ch + <-ch fmt.Println("Total Sum:", total) }
HTTP Server with Rate Limiting:
Problem: Create an HTTP server that limits incoming requests to 5 per second using a token bucket.
Hints:
- Use
golang.org/x/time/rate
for rate limiting.
- Use
Solution:
package main import ( "net/http" "golang.org/x/time/rate" ) var limiter = rate.NewLimiter(5, 5) func rateLimitMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { if !limiter.Allow() { http.Error(w, "Too Many Requests", http.StatusTooManyRequests) return } next.ServeHTTP(w, r) }) } func main() { mux := http.NewServeMux() mux.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, World!")) }) http.ListenAndServe(":8080", rateLimitMiddleware(mux)) }
File Processing Pipeline:
Problem: Implement a pipeline to read lines from a file, process them, and write results to another file concurrently.
Hints:
Use channels to pass data through the pipeline stages.
Use
bufio
for reading and writing files.
10.2 Frequently Asked Questions
Why doesn’t Go have generics?
- Go added generics in version 1.18 to balance simplicity and flexibility. Prior to this, Go relied on interfaces and type assertions for generic behavior.
How is Go different from other languages like Python or Java?
Python: Dynamically typed, interpreted, extensive libraries.
Java: Statically typed, verbose, strong object orientation.
Go: Statically typed, compiled, lightweight concurrency.
Is Go suitable for machine learning or data analysis?
- While Go has libraries like
gonum
andgorgonia
, Python remains the preferred language for ML and data analysis due to its mature ecosystem.
- While Go has libraries like
Why does Go enforce strict error handling?
- Explicit error handling improves code clarity and reliability, making it easier to debug and maintain.
10.3 Troubleshooting Tips
Compilation Errors:
Common Issue: Undefined variables or unused imports.
Solution: Remove unused variables or imports, as Go enforces code cleanliness.
Race Conditions:
Common Issue: Concurrent goroutines accessing shared resources.
Solution: Use
sync.Mutex
orsync.RWMutex
to prevent data races.
Example:
var mu sync.Mutex
func safeIncrement(counter *int) {
mu.Lock()
*counter++
mu.Unlock()
}
Dependency Issues:
Common Issue: Module not found during
go build
.Solution:
- Run
go mod tidy
to clean up and download missing dependencies.
- Run
10.4 Roadmap for Learning Go
Beginner Level:
Learn the basics: variables, control flow, functions, structs, and slices.
Build a simple CLI application using
flag
package.
Intermediate Level:
Explore concurrency: goroutines, channels, and the
sync
package.Build a RESTful API with Gin or Echo.
Use GORM for database interactions.
Advanced Level:
Master error handling and context management.
Dive into Go’s internals: garbage collection, memory management, and runtime optimizations.
Contribute to an open-source Go project.
Expert Level:
Build and deploy distributed systems using Kubernetes.
Explore Go for WebAssembly or IoT projects.
Write a custom Go toolchain plugin.
10.5 Appendix: Common Libraries and Frameworks
Web Frameworks:
Gin
: High-performance web framework.Echo
: Lightweight and extensible.
Database Libraries:
GORM
: ORM for relational databases.sqlx
: SQL extension library.
Logging:
logrus
: Structured logging.zap
: Fast logging library.
Testing:
testify
: Assertions and mock frameworks.
Utilities:
cobra
: Command-line application builder.viper
: Configuration management.
10.6 Suggested Projects
To-Do List Application:
- Build a simple CRUD app using Gin and SQLite.
Concurrency-Based Crawler:
- Scrape multiple websites concurrently using goroutines and channels.
Real-Time Chat Application:
- Use WebSockets for real-time communication.
API Gateway:
- Implement rate limiting, authentication, and proxying for multiple APIs.
10.7 Glossary of Key Go Terms
Goroutines:
- Lightweight threads for concurrent execution.
Channels:
- Communication mechanism between goroutines.
Interfaces:
- Contract for behavior implementation.
Zero Value:
- Default value for uninitialized variables.
Defer:
- Executes a function call at the end of its enclosing function.
Congratulations on completing your journey through this guide to Go programming! By now, you've acquired a robust understanding of Go's syntax, idioms, and ecosystem. You've explored everything from the fundamentals to advanced topics like concurrency, deployment, and performance optimization.
The skills you’ve learned here are just the beginning. Go is a continuously evolving language with an active and vibrant community. As you apply your knowledge in real-world projects, you'll uncover even more opportunities to innovate, optimize, and create.
Here’s what you can do next:
Build Projects: Apply your skills to solve real problems—start small, then scale up to complex systems.
Contribute to Open Source: Join the Go community by contributing to libraries or frameworks.
Explore Advanced Topics: Dive into areas like WebAssembly, IoT, or distributed systems with Go.
Stay Updated: Follow Go’s release notes and community blogs to stay ahead of new features and best practices.
Remember, Go’s simplicity and performance give you the power to create clean, efficient, and maintainable code. As you continue to grow in your Go journey, always strive for code that embodies clarity, reliability, and scalability.
Thank you for choosing this guide as your companion. Happy coding!
For more such indepth guides, ebooks, visit AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
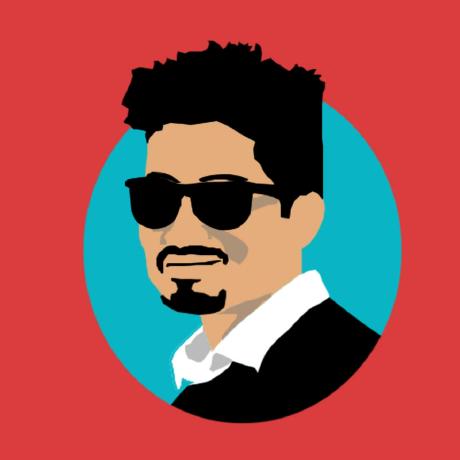