Unlocking Hyper-Performance: Writing Efficient APIs with Golang's Fiber vs Node.js's Fastify
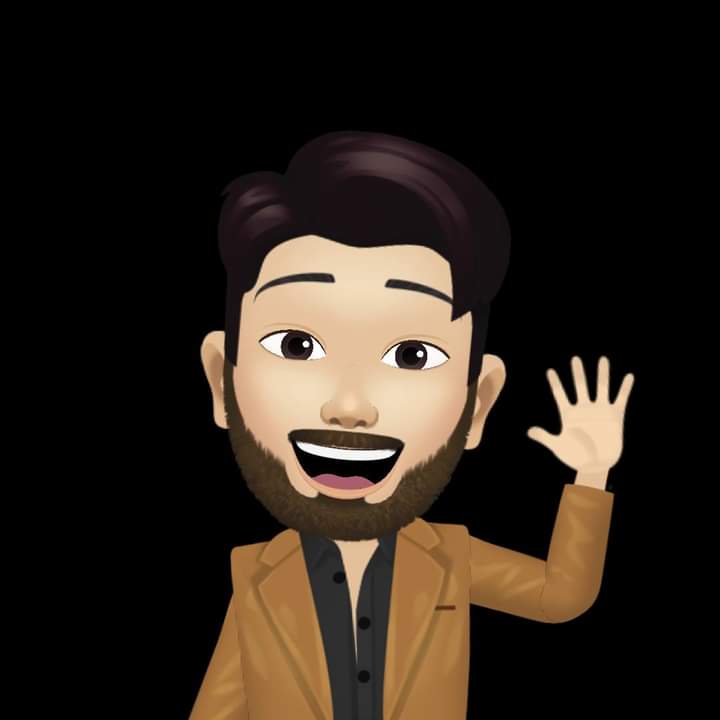

As modern applications demand ultra-fast performance, developers often find themselves choosing frameworks that can handle millions of requests per second. Two standout options for API development are Fiber (Golang) and Fastify (Node.js). Both promise high throughput, but their underlying architectures and ecosystems cater to different kinds of developers. This post explores these frameworks with practical examples, advanced optimizations, and tips to maximize performance.
Why Fiber and Fastify?
Fiber (Golang)
Fiber is built on top of fasthttp, the fastest HTTP engine for Golang. Its simplicity and performance make it an ideal choice for developers looking to squeeze every ounce of efficiency from their APIs.
Key Features:
Extremely low memory footprint.
Minimal overhead with fasthttp.
Built-in middleware and plugins for rapid development.
Fastify (Node.js)
Fastify boasts unparalleled speed and developer-friendliness in the Node.js ecosystem. It leverages asynchronous I/O and plugins to create high-performance APIs with minimal effort.
Key Features:
Schema-based validation for better performance and reliability.
Ecosystem rich with plugins for extending functionality.
Built-in request/response hooks for granular control.
Building a REST API: Fiber vs Fastify
Let’s build a simple REST API with both frameworks to compare their ease of use, performance, and scalability.
Fiber Example:
A basic GET
endpoint to retrieve users:
package main
import (
"github.com/gofiber/fiber/v2"
)
type User struct {
ID int `json:"id"`
Name string `json:"name"`
}
func main() {
app := fiber.New()
// Sample data
users := []User{
{ID: 1, Name: "John Doe"},
{ID: 2, Name: "Jane Smith"},
}
// Get all users
app.Get("/users", func(c *fiber.Ctx) error {
return c.JSON(users)
})
// Start the server
app.Listen(":3000")
}
Advanced Concept: Caching with Middleware
To optimize for high throughput, you can add a caching layer:
import "github.com/gofiber/fiber/v2/middleware/cache"
app.Use(cache.New(cache.Config{
Expiration: 10 * time.Second,
}))
Fastify Example:
The equivalent GET
endpoint in Fastify:
const fastify = require('fastify')({ logger: true });
// Sample data
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' },
];
// Get all users
fastify.get('/users', async (request, reply) => {
return users;
});
// Start the server
fastify.listen({ port: 3000 }, (err) => {
if (err) throw err;
});
Advanced Concept: Schema Validation
Fastify shines with built-in schema validation to enhance performance:
fastify.get('/users', {
schema: {
response: {
200: {
type: 'array',
items: {
type: 'object',
properties: {
id: { type: 'integer' },
name: { type: 'string' },
},
},
},
},
},
}, async (request, reply) => {
return users;
});
Advanced Concepts for Hyper-Performance
1. Concurrency and Worker Pools in Fiber
Fiber's concurrency model benefits from Golang’s goroutines. For CPU-bound tasks, use worker pools to prevent blocking the main thread.
package main
import (
"sync"
"github.com/gofiber/fiber/v2"
)
var wg sync.WaitGroup
func worker(id int, jobs <-chan int) {
for job := range jobs {
// Process the job
}
wg.Done()
}
func main() {
app := fiber.New()
// Worker pool example
jobs := make(chan int, 100)
for i := 1; i <= 5; i++ {
wg.Add(1)
go worker(i, jobs)
}
app.Post("/process", func(c *fiber.Ctx) error {
job := c.FormValue("job")
jobs <- job // Send job to worker pool
return c.SendStatus(202)
})
wg.Wait()
app.Listen(":3000")
}
2. Async Hooks and Middlewares in Fastify
Fastify’s hooks allow for advanced request/response lifecycle management. Use them to log, modify requests, or even manage authentication tokens.
fastify.addHook('onRequest', async (request, reply) => {
console.log(`Incoming request: ${request.method} ${request.url}`);
});
fastify.addHook('onResponse', async (request, reply) => {
console.log(`Response sent for: ${request.method} ${request.url}`);
});
3. Benchmarking with Artillery
Both Fiber and Fastify claim to handle millions of requests per second. Use tools like Artillery for load testing:
Artillery Config Example:
config:
target: "http://localhost:3000"
phases:
- duration: 60
arrivalRate: 1000
scenarios:
- flow:
- get:
url: "/users"
Run the test and compare the results!
Key Takeaways
Fiber outshines when raw performance is crucial, thanks to Go’s efficient concurrency model and fasthttp. It’s ideal for low-latency APIs and CPU-intensive tasks.
Fastify delivers impressive performance within the Node.js ecosystem, offering unmatched developer experience with features like schema validation and hooks.
Both frameworks benefit from optimizations like caching, middleware, and proper load balancing.
Which framework suits your next API project? Share your experience and benchmarks in the comments below! 🚀
Subscribe to my newsletter
Read articles from JealousGx directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
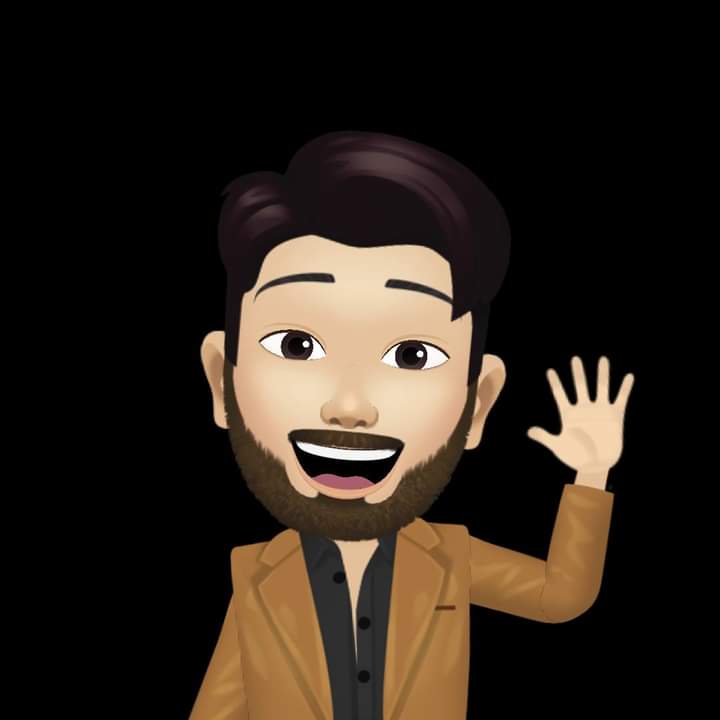
JealousGx
JealousGx
Hello, I'm a highly skilled full stack web developer with a rich background in creating and maintaining dynamic websites that drive online engagement and brand awareness. My expertise extends beyond WordPress to include a versatile skill set.