Python Lists and Tuples: A Comprehensive Guide for DevOps Engineers
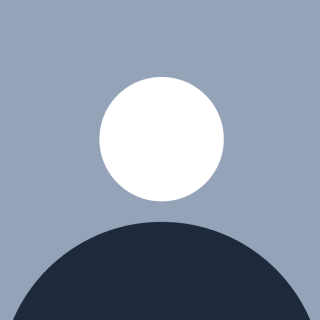
Introduction
In the world of Python programming, lists and tuples are fundamental sequence data types that every developer and DevOps engineer should master. This guide will walk you through the basics of lists and tuples, their differences, and practical use cases.
What are Lists?
A list in Python is a mutable, ordered collection of elements that can store different types of data. Lists are created using square brackets []
.
Key Characteristics of Lists:
Mutable (can be modified after creation)
Can store elements of different data types
Supports dynamic resizing
Zero-indexed
Basic List Operations
Creating a List
students = ['Abhishek', 'Ram', 'Tim', 'John']
Adding Elements
students.append('New Student')
Removing Elements
students.remove('Ram')
Accessing Elements
first_student = students[0] # Accesses first element
Understanding Tuples
A tuple is an immutable, ordered collection of elements created using parentheses ()
.
Key Characteristics of Tuples:
Immutable (cannot be changed after creation)
More memory-efficient
Useful for storing constant collections
Creating a Tuple
admin_users = ('Abhishek', 'John')
When to Use Lists vs Tuples
Use Lists When:
You need a collection that can change
Storing dynamic data like S3 buckets, EC2 instances
Elements may be added or removed
Use Tuples When:
You want an unchangeable collection
Storing fixed information like admin users
Need better memory performance
DevOps Use Cases
Lists in DevOps
Storing S3 bucket names
Managing EC2 instance IDs
Tracking EKS clusters
Tuples in DevOps
Storing fixed configuration parameters
Representing unchangeable credentials or access keys
Advanced List Techniques
Heterogeneous Lists
random_list = [1, 'Ramu', 7.5, True]
List Slicing
subset = original_list[0:3] # Creates a new list from index 0 to 2
Sorting
numbers = [10, 1, 15]
numbers.sort() # Sorts in-place
Best Practices
Use lists for dynamic collections
Use tuples for fixed, sensitive data
Choose the right data structure based on your use case
Conclusion
Understanding the nuances between lists and tuples is crucial for writing efficient and clear Python code, especially in DevOps scenarios. Practice these concepts to become more proficient in Python programming.
Next Steps
Practice creating and manipulating lists and tuples
Explore advanced list operations
Apply these concepts in real-world DevOps scenarios
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by