API Design and Development: A Reference for Building Robust and Scalable Systems
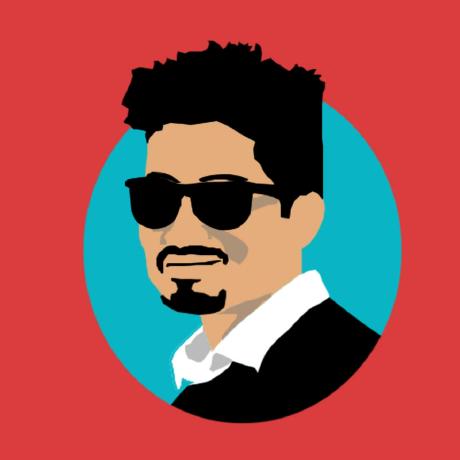

In the digital age, APIs (Application Programming Interfaces) have become the backbone of modern software development. They are the invisible threads that connect applications, enabling seamless communication and integration across diverse systems. From social media platforms and payment gateways to cloud-based services and IoT devices, APIs power the tools we use daily.
This guide is designed to be your comprehensive resource for mastering API design and development. Whether you're a seasoned developer or just starting, this guide covers every aspect of API creation—from foundational concepts to advanced techniques and real-world projects. With hands-on examples in Python, PHP, and Node.js, as well as insights into best practices, scalability, and lifecycle management, this is your ultimate reference for building APIs that are not only functional but also elegant, secure, and developer-friendly.
Prepare to embark on a journey where you will learn to craft APIs that stand the test of time, delight developers, and power robust, scalable systems.
Part 1: Conceptual Foundation
1. Introduction to APIs
What is an API?
An API, or Application Programming Interface, is a defined set of rules that enable different software applications to communicate with each other. It acts as a contract that specifies how two systems (a client and a server, for instance) will interact, detailing what requests can be made, how to make them, and the expected responses.
Real-world Analogy: Imagine a restaurant:
The menu is the API. It defines what the restaurant offers and how to order.
The customer (you) interacts with the menu, choosing what to request.
The kitchen is the server. It prepares the food (processes the request) and delivers it back to you.
Why APIs Matter:
Automation: APIs enable applications to perform tasks without human intervention. For example, an API can automate data fetching from a weather service.
Integration: APIs allow different systems to work together. For example, a payment gateway like Stripe integrates with e-commerce platforms to process payments.
Efficiency: Developers can reuse functionality exposed by APIs instead of building it from scratch. For instance, Google Maps APIs allow businesses to embed maps rather than creating mapping software.
Types of APIs
Web APIs (HTTP-based):
RESTful APIs: Most common for web applications.
GraphQL APIs: Flexible querying of data.
SOAP APIs: Uses XML; still in use for legacy systems.
Library APIs:
Expose functionality of a programming library or SDK.
Example: TensorFlow APIs for machine learning.
Operating System APIs:
Allow applications to interact with the OS.
Example: Windows API for accessing system features.
Hardware APIs:
Enable software to control hardware.
Example: Camera APIs in smartphones.
Real-world Examples
Google Maps API:
Use Case: Embed maps, geolocation, and route planning in apps.
Example:GET https://maps.googleapis.com/maps/api/geocode/json?address=New+York&key=YOUR_API_KEY
Twitter API:
Use Case: Fetch tweets, post programmatically, or analyze trends.
Example:GET https://api.twitter.com/2/tweets/1453489038376132611
Stripe API:
Use Case: Process payments and manage billing.
Example:POST https://api.stripe.com/v1/payment_intents
2. Principles of Good API Design
APIs are often judged by their usability and consistency. The design principles below are foundational for building APIs developers will love.
Simplicity and Intuitiveness
APIs should be simple enough for developers to guess their purpose and behavior without constantly referencing documentation.
Examples:
Good:
GET /users/123
Fetches user data for user ID 123.
Bad:
GET /getAllUserDataByID?id=123
Verbose and redundant.
Consistency and Predictability
Consistency reduces the cognitive load for developers. When all endpoints follow a uniform pattern, users quickly understand how to use the API.
Good Practices:
Use the same structure for similar actions.
GET /users/123 GET /products/456
Standardize response formats.
{ "status": "success", "data": { "id": 123, "name": "John Doe" } }
Developer Experience (DX)
APIs are products for developers, so their "user experience" matters. A positive developer experience can drive adoption.
Essential DX Features:
Clear, updated documentation.
Examples of common use cases.
SDKs or libraries for easy integration.
Sandbox environments for testing.
Security and Performance
Security:
Always use HTTPS to encrypt communication.
Implement authentication (OAuth, API keys, JWT).
Sanitize inputs to prevent injection attacks.
Performance:
Use caching (e.g.,
ETags
,Cache-Control
headers).Optimize queries and database access.
Minimize payload size (e.g., compress responses).
3. Understanding REST Architecture
REST (Representational State Transfer) is the most widely used API design architecture. It revolves around the concept of resources.
Key Concepts
Resources:
Everything exposed by the API is a resource.
Resources are identified by URIs.
Example:
/users
: A collection of users./users/123
: A specific user.
HTTP Methods:
GET
: Retrieve data.POST
: Create a new resource.PUT
: Update an existing resource.DELETE
: Remove a resource.
Example Resource Mapping:
Fetch all blog posts:
GET /posts
Fetch a specific post:
GET /posts/5
Create a new post:
POST /posts
Update a post:
PUT /posts/5
Delete a post:
DELETE /posts/5
Statelessness
In REST, each API request must contain all the information the server needs to fulfill it. The server does not store any client state between requests.
Benefits:
Scalable: Servers can handle requests independently.
Reliable: Reduces potential state-related errors.
Example (Stateless):
Request 1:
GET /cart
(Returns an empty cart)Request 2:
POST /cart
with product details to add to the cart.Server processes each independently.
4. Beyond REST: GraphQL and WebSockets
GraphQL
GraphQL provides a more flexible alternative to REST by allowing clients to specify the structure of the response.
Benefits:
Avoids over-fetching and under-fetching of data.
Single endpoint (
/graphql
) instead of multiple resource-specific endpoints.
Example Query:
query {
user(id: 1) {
name
email
orders {
id
total
}
}
}
Response:
{
"data": {
"user": {
"name": "John Doe",
"email": "john.doe@example.com",
"orders": [
{ "id": 101, "total": 250 },
{ "id": 102, "total": 75 }
]
}
}
}
WebSockets
WebSockets enable full-duplex communication channels over a single TCP connection. They are ideal for real-time applications like chat systems, stock tickers, or live notifications.
Example Use Case:
Stock Market Updates:
Client subscribes to a stock symbol.
Server pushes price updates in real-time.
Sample WebSocket Message:
Client:
{ "action": "subscribe", "symbol": "AAPL" }
Server:
{ "symbol": "AAPL", "price": 173.25 }
Part 2: Practical API Design
1. Planning and Designing an API
Understanding Requirements
Before writing any code, it’s essential to clearly define the purpose of your API and the needs of its users.
Steps to Define API Requirements:
Identify End Users:
Are they front-end developers, mobile developers, or third-party integrators?
Example: An E-commerce API might serve:
Frontend developers creating the shopping website.
Mobile app developers building the store's app.
Third-party developers integrating external inventory systems.
List Use Cases:
Example for E-commerce:
Customers need to browse and search products.
Admins need to add, update, and delete products.
Payment systems need to process transactions.
Define Resources:
- Resources represent entities within your system (e.g.,
products
,users
,orders
).
- Resources represent entities within your system (e.g.,
Document Actions and Interactions:
Example:
Products:
GET /products
: Retrieve all products.GET /products/{id}
: Retrieve a single product.POST /products
: Add a new product (admin only).PUT /products/{id}
: Update product details (admin only).DELETE /products/{id}
: Remove a product (admin only).
API Specifications
Using tools like OpenAPI/Swagger can formalize the API design and serve as a blueprint for development.
OpenAPI Example:
openapi: 3.0.0
info:
title: E-commerce API
version: 1.0.0
paths:
/products:
get:
summary: Retrieve all products
responses:
200:
description: A list of products
content:
application/json:
schema:
type: array
items:
$ref: '#/components/schemas/Product'
/products/{id}:
get:
summary: Retrieve a single product
parameters:
- name: id
in: path
required: true
schema:
type: string
responses:
200:
description: Details of a product
content:
application/json:
schema:
$ref: '#/components/schemas/Product'
components:
schemas:
Product:
type: object
properties:
id:
type: string
name:
type: string
price:
type: number
2. API Design Patterns
CRUD Operations
CRUD (Create, Read, Update, Delete) is the backbone of API design. RESTful APIs use HTTP methods to implement CRUD operations.
Example: Task Management API
Tasks Resource:
GET /tasks
: Retrieve all tasks.POST /tasks
: Create a new task.GET /tasks/{id}
: Retrieve a single task.PUT /tasks/{id}
: Update task details.DELETE /tasks/{id}
: Delete a task.
Sample Implementation:
Python (Django REST Framework):
from rest_framework.views import APIView
from rest_framework.response import Response
from rest_framework import status
tasks = []
class TaskView(APIView):
def get(self, request):
return Response(tasks)
def post(self, request):
task = request.data
tasks.append(task)
return Response(task, status=status.HTTP_201_CREATED)
PHP (Laravel):
use Illuminate\Http\Request;
Route::get('/tasks', function () {
return Task::all();
});
Route::post('/tasks', function (Request $request) {
return Task::create($request->all());
});
Node.js (Express):
const express = require('express');
const app = express();
app.use(express.json());
let tasks = [];
app.get('/tasks', (req, res) => {
res.json(tasks);
});
app.post('/tasks', (req, res) => {
const task = req.body;
tasks.push(task);
res.status(201).json(task);
});
Pagination, Filtering, and Sorting
APIs should handle large datasets efficiently by supporting pagination, filtering, and sorting.
Example:
Pagination:
Query Parameters:
?page=1&limit=10
Response:
{ "data": [...], "pagination": { "page": 1, "limit": 10, "total": 100 } }
Filtering:
Query Parameters:
?category=electronics&price[gte]=1000
Explanation:
category=electronics
: Filter by category.price[gte]=1000
: Filter products with price >= 1000.
Sorting:
Query Parameters:
?sort=price:asc
Explanation:
price:asc
: Sort by price in ascending order.
Authentication and Authorization
Securing an API is critical. Authentication verifies who is accessing the API, while authorization ensures they have the correct permissions.
Authentication Options:
API Keys:
Simple but less secure.
Example:
GET /users?api_key=abcdef123456
OAuth2:
- Used for delegated access (e.g., "Login with Google").
JWT (JSON Web Tokens):
- Tokens include user information and are signed for integrity.
Authorization Levels:
Example Roles:
Admin: Full access.
User: Limited to their resources.
Error Handling
APIs should provide consistent, informative error messages to help clients debug effectively.
Example Error Response:
{
"error": "Resource not found",
"code": 404,
"details": "The requested product with ID 123 does not exist."
}
HTTP Status Codes:
200 OK
: Success.201 Created
: Resource successfully created.400 Bad Request
: Client error.401 Unauthorized
: Authentication required.403 Forbidden
: Permission denied.404 Not Found
: Resource doesn’t exist.500 Internal Server Error
: Unexpected server issue.
3. Versioning APIs
Versioning ensures that existing clients are not affected by changes to the API.
Techniques:
URI Versioning:
- Example:
/v1/products
,/v2/products
- Example:
Header Versioning:
- Example:
Accept: application/vnd.api+json;version=1.0
- Example:
Query Parameter:
- Example:
/products?version=1.0
- Example:
Best Practices:
Avoid breaking changes in the same version.
Deprecate older versions with clear communication.
4. Building APIs with Examples
Let’s consolidate our learnings by implementing an API for a Blog Application in Python, PHP, and Node.js.
Python (Django REST Framework):
from rest_framework.views import APIView
from rest_framework.response import Response
from rest_framework import status
blogs = []
class BlogView(APIView):
def get(self, request):
return Response(blogs)
def post(self, request):
blog = request.data
blogs.append(blog)
return Response(blog, status=status.HTTP_201_CREATED)
PHP (Laravel):
use Illuminate\Http\Request;
Route::get('/blogs', function () {
return Blog::all();
});
Route::post('/blogs', function (Request $request) {
return Blog::create($request->all());
});
Node.js (Express):
const express = require('express');
const app = express();
app.use(express.json());
let blogs = [];
app.get('/blogs', (req, res) => {
res.json(blogs);
});
app.post('/blogs', (req, res) => {
const blog = req.body;
blogs.push(blog);
res.status(201).json(blog);
});
Part 3: Advanced Topics
1. Securing APIs
Security is a critical aspect of API design. A poorly secured API can expose sensitive data, compromise user accounts, or even allow malicious actors to take over your system.
Authentication
Authentication verifies the identity of the client accessing the API.
Basic Authentication:
Sends credentials (
username:password
) encoded in Base64 in theAuthorization
header.Example:
Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=
Cons: Vulnerable if not used with HTTPS.
Token-Based Authentication:
Uses an API key or token instead of a password.
Example:
Authorization: Bearer YOUR_ACCESS_TOKEN
Tokens can be issued for specific scopes or time periods.
OAuth2:
Standard for delegated access.
Example Use Case: "Login with Google."
OAuth2 Flow:
Authorization Code Flow:
User logs into a third-party service (e.g., Google).
The service issues a temporary code.
The client exchanges the code for an access token.
Example Access Token Response:
{ "access_token": "ya29.a0AR...", "token_type": "Bearer", "expires_in": 3600 }
JWT (JSON Web Tokens):
Encodes claims (e.g., user ID, roles) in a signed token.
Example JWT:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyX2lkIjoxMjMsInJvbGUiOiJhZG1pbiJ9.cG
9F2RGsVfQHY12R-W8vIwEa9CvSthKz6Ix4W8c67ko
Verifiable using a secret or public/private keys.
Python Example (JWT Authentication with Django REST Framework):
pip install djangorestframework-simplejwt
from rest_framework_simplejwt.views import TokenObtainPairView, TokenRefreshView
urlpatterns = [
path('api/token/', TokenObtainPairView.as_view(), name='token_obtain_pair'),
path('api/token/refresh/', TokenRefreshView.as_view(), name='token_refresh'),
]
Authorization
Authorization determines what resources an authenticated client can access.
Role-Based Access Control (RBAC):
Assign roles to users (e.g.,
admin
,editor
,viewer
).Example Rule:
admin
: Full access.editor
: Modify content but not user data.viewer
: Read-only access.
Attribute-Based Access Control (ABAC):
Access is granted based on user attributes and resource properties.
Example:
- Allow users to edit their own data (
user_id
in the token matchesuser_id
in the resource).
- Allow users to edit their own data (
Node.js Example (RBAC Middleware):
const roles = {
admin: ['read', 'write', 'delete'],
editor: ['read', 'write'],
viewer: ['read']
};
function authorize(role, action) {
return (req, res, next) => {
if (roles[role] && roles[role].includes(action)) {
next();
} else {
res.status(403).json({ message: 'Forbidden' });
}
};
}
app.post('/posts', authorize('editor', 'write'), (req, res) => {
res.send('Post created');
});
Securing Data
Use HTTPS:
- Encrypts communication between client and server.
Encrypt Sensitive Data:
Store passwords using algorithms like
bcrypt
.Example in Python:
from bcrypt import hashpw, gensalt hashed = hashpw(b"password", gensalt()) print(hashed)
Limit Data Exposure:
- Avoid exposing sensitive fields like passwords or internal IDs.
2. Optimizing Performance
APIs should be designed for scalability and speed, ensuring they can handle high traffic and large datasets.
Caching
Client-Side Caching:
Use HTTP headers (
Cache-Control
,ETag
) to enable browsers or clients to cache responses.Example Response Header:
Cache-Control: max-age=3600
Server-Side Caching:
Use Redis or Memcached to cache frequently accessed data.
Example in Python:
import redis cache = redis.StrictRedis(host='localhost', port=6379, db=0) cache.set('key', 'value')
Rate Limiting
Protect APIs from abuse by limiting the number of requests a client can make.
Example:
Limit: 100 requests per minute.
Response:
HTTP/1.1 429 Too Many Requests Retry-After: 60
Node.js Example with express-rate-limit
:
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 1 * 60 * 1000,
max: 100
});
app.use(limiter);
Load Balancing
Distribute traffic across multiple servers to prevent bottlenecks.
Example:
- Use AWS Elastic Load Balancer or NGINX to balance API traffic.
NGINX Load Balancer Configuration:
http {
upstream api_servers {
server api1.example.com;
server api2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://api_servers;
}
}
}
3. Real-time APIs
Real-time APIs are essential for applications requiring live updates, such as chat systems or stock tickers.
WebSockets
Enable bi-directional communication between client and server over a single TCP connection.
Python Example with websockets
:
import asyncio
import websockets
async def echo(websocket, path):
async for message in websocket:
await websocket.send(f"Received: {message}")
start_server = websockets.serve(echo, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
Node.js Example:
const WebSocket = require('ws');
const server = new WebSocket.Server({ port: 8080 });
server.on('connection', socket => {
socket.on('message', message => {
socket.send(`Received: ${message}`);
});
});
Server-Sent Events (SSE)
An alternative to WebSockets for one-way real-time updates.
Example Use Case: Stock Price Ticker
Node.js SSE Example:
app.get('/events', (req, res) => {
res.setHeader('Content-Type', 'text/event-stream');
setInterval(() => {
res.write(`data: ${JSON.stringify({ price: Math.random() * 100 })}\n\n`);
}, 1000);
});
4. Testing and Debugging APIs
Testing ensures APIs behave as expected and debugging helps identify issues during development or in production.
Unit Testing
Write tests for individual functions or endpoints.
Python Example (Pytest):
def test_get_users(client):
response = client.get('/users')
assert response.status_code == 200
assert len(response.json()) > 0
Integration Testing
Test how endpoints work together.
Postman:
Create a collection of API requests.
Automate tests using Postman scripts.
Debugging Tools
Python:
pdb
or Django Debug Toolbar.Node.js:
node-inspect
or VSCode Debugger.API Monitoring: Tools like Postman Monitor or New Relic.
Part 4: API Lifecycle Management
API development is not just about designing and coding; it's a continuous process involving deployment, monitoring, scaling, and improving. This chapter focuses on managing the entire lifecycle of an API.
1. Deployment Strategies
Efficient deployment ensures your API is accessible, stable, and scalable.
1.1. Containerization
What is Containerization?
Containers package applications and their dependencies, ensuring consistency across environments.
Popular tools: Docker and Kubernetes.
Example: Dockerizing an API
Create a
Dockerfile
:# Base image FROM python:3.9-slim # Set working directory WORKDIR /app # Copy project files COPY . . # Install dependencies RUN pip install -r requirements.txt # Expose the port EXPOSE 8000 # Start the API CMD ["gunicorn", "-w", "4", "-b", "0.0.0.0:8000", "app:app"]
Build and run:
docker build -t my-api . docker run -p 8000:8000 my-api
1.2. CI/CD Pipelines
CI/CD automates building, testing, and deploying code. Tools like GitHub Actions, GitLab CI/CD, and Jenkins make this process seamless.
Example: GitLab CI/CD for a Node.js API
stages:
- test
- deploy
test:
stage: test
script:
- npm install
- npm test
deploy:
stage: deploy
script:
- npm run build
- scp -r ./dist/ user@server:/var/www/api
1.3. Deployment Platforms
Cloud Providers:
AWS, Azure, Google Cloud.
Example: Deploying with AWS Elastic Beanstalk or Lambda for serverless APIs.
PaaS Providers:
Heroku, Netlify, Vercel.
Example: Hosting Node.js APIs on Vercel.
2. Monitoring and Observability
Monitoring APIs ensures they perform as expected and helps identify issues early.
2.1. Logging
Log requests, responses, and errors to track API usage and troubleshoot issues.
Best Practices:
Use structured logging (e.g., JSON).
Include metadata like timestamps, request IDs, and IP addresses.
Python Logging Example:
import logging
logging.basicConfig(level=logging.INFO)
logging.info("API started successfully")
2.2. Real-Time Monitoring
Real-time monitoring tools help track performance metrics like response time, request count, and error rates.
Popular Tools:
New Relic: Application performance monitoring.
Datadog: Full-stack observability.
Prometheus + Grafana: Open-source monitoring and visualization.
2.3. Alerts
Set up alerts for critical issues:
Latency exceeding a threshold.
High error rates (e.g., 5xx responses).
Example Alert in Prometheus:
groups:
- name: API_Alerts
rules:
- alert: HighErrorRate
expr: rate(http_requests_total{status=~"5.."}[5m]) > 0.05
for: 5m
labels:
severity: critical
annotations:
summary: "High error rate detected"
3. Scaling APIs
Scaling ensures APIs can handle increased traffic without compromising performance.
3.1. Vertical Scaling
Increase the resources (CPU, RAM) of the server hosting the API.
Pros: Simple to implement.
Cons: Limited by physical hardware.
3.2. Horizontal Scaling
Add more servers to distribute the load.
Implementation:
- Use a load balancer to distribute traffic across multiple servers.
AWS Example with Elastic Load Balancer:
Configure instances under an Auto Scaling Group.
Set target metrics (e.g., CPU utilization < 80%).
3.3. Database Scaling
Read Replicas:
Distribute read queries to replicas.
Example: Configure read replicas in MySQL or PostgreSQL.
Sharding:
Split data across multiple databases.
Example:
- Users A-M in
db1
, N-Z indb2
.
- Users A-M in
4. Maintenance and Continuous Improvement
Maintenance involves regularly updating, improving, and refining APIs.
4.1. Deprecation and Versioning
Plan for deprecating old APIs gracefully.
Best Practices:
Notify users well in advance (e.g., 6 months).
Offer support for migrations.
Example:
{ "message": "This API version will be deprecated on 2025-01-01. Please migrate to /v2." }
4.2. Feedback Loops
Gather feedback from API users to improve functionality.
Methods:
Surveys and user interviews.
Analyze support tickets and complaints.
4.3. Continuous Integration
Integrate updates and fixes frequently to keep APIs aligned with evolving requirements.
Use feature toggles to roll out features incrementally.
Conduct A/B testing for experimental features.
5. Case Study: Scaling a Multi-Tenant SaaS API
Scenario: You’re managing a SaaS platform for managing tasks across multiple teams. Each tenant (team) has its own data, and traffic increases as your client base grows.
Challenges:
Traffic Management:
Handle thousands of concurrent users.
Ensure response times remain low.
Data Isolation:
- Prevent tenant data from leaking into another tenant’s workspace.
Scaling Databases:
- Maintain performance while supporting tenant-specific data.
Solutions:
Traffic Management:
Use Kubernetes for auto-scaling API containers.
Deploy an NGINX load balancer to route traffic.
Data Isolation:
Implement row-level security (PostgreSQL).
Example: Use
tenant_id
in queries.SELECT * FROM tasks WHERE tenant_id = 'abc123';
Database Scaling:
Introduce sharding for tenants with heavy workloads.
Archive old data to reduce primary database load.
Part 5: Real-World API Projects
This chapter focuses on practical, end-to-end API implementations for real-world scenarios. These projects demonstrate how to apply the principles, techniques, and tools from earlier chapters in Python, PHP, and Node.js.
1. Building a Complete API Project
Scenario: Multi-Tenant Task Management API
A multi-tenant task management system where:
Each tenant (team) has isolated data.
Users can create, update, delete, and manage tasks within their team.
Admins can manage users and teams.
Features:
Authentication and Authorization:
JWT-based user authentication.
Role-based access control (Admin, Member).
Task Management:
CRUD operations for tasks.
Support for filtering and sorting.
Multi-Tenant Support:
- Tenant isolation using
tenant_id
.
- Tenant isolation using
Real-Time Notifications:
- WebSocket integration for live task updates.
1.1 API Design and Endpoints
Base URL: https://api.example.com
Method | Endpoint | Description |
POST | /auth/register | Register a new user |
POST | /auth/login | Authenticate user |
GET | /tasks | Fetch tasks for the tenant |
POST | /tasks | Create a new task |
PUT | /tasks/{id} | Update a task |
DELETE | /tasks/{id} | Delete a task |
GET | /users | Fetch users in the tenant |
POST | /users | Invite a user to the tenant |
DELETE | /users/{id} | Remove a user from the tenant |
1.2 Database Schema
Users Table:
CREATE TABLE users (
id UUID PRIMARY KEY,
email VARCHAR(255) UNIQUE NOT NULL,
password_hash TEXT NOT NULL,
role VARCHAR(50) DEFAULT 'member',
tenant_id UUID NOT NULL,
FOREIGN KEY (tenant_id) REFERENCES tenants(id)
);
Tasks Table:
CREATE TABLE tasks (
id UUID PRIMARY KEY,
title VARCHAR(255) NOT NULL,
description TEXT,
status VARCHAR(50) DEFAULT 'pending',
tenant_id UUID NOT NULL,
created_by UUID NOT NULL,
FOREIGN KEY (tenant_id) REFERENCES tenants(id),
FOREIGN KEY (created_by) REFERENCES users(id)
);
Tenants Table:
CREATE TABLE tenants (
id UUID PRIMARY KEY,
name VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
1.3 Implementation
Python (Django REST Framework)
Setup and Authentication: Install dependencies:
pip install djangorestframework djangorestframework-simplejwt
Define authentication views:
from rest_framework_simplejwt.views import TokenObtainPairView, TokenRefreshView urlpatterns = [ path('auth/login/', TokenObtainPairView.as_view(), name='token_obtain_pair'), path('auth/refresh/', TokenRefreshView.as_view(), name='token_refresh'), ]
Task Management API:
from rest_framework import viewsets, permissions from .models import Task from .serializers import TaskSerializer class TaskViewSet(viewsets.ModelViewSet): serializer_class = TaskSerializer permission_classes = [permissions.IsAuthenticated] def get_queryset(self): return Task.objects.filter(tenant_id=self.request.user.tenant_id) def perform_create(self, serializer): serializer.save(tenant_id=self.request.user.tenant_id, created_by=self.request.user)
PHP (Laravel)
Setup and Middleware: Install dependencies:
composer require tymon/jwt-auth php artisan jwt:secret
Middleware for tenant-based isolation:
public function handle($request, Closure $next) { $request->merge(['tenant_id' => auth()->user()->tenant_id]); return $next($request); }
Task Controller:
use App\Models\Task; class TaskController extends Controller { public function index() { return Task::where('tenant_id', auth()->user()->tenant_id)->get(); } public function store(Request $request) { $request->validate([ 'title' => 'required', 'description' => 'nullable', ]); return Task::create([ 'title' => $request->title, 'description' => $request->description, 'tenant_id' => $request->tenant_id, 'created_by' => auth()->id(), ]); } }
Node.js (Express)
Setup: Install dependencies:
npm install express jsonwebtoken bcryptjs mongoose
Authentication Middleware:
const jwt = require('jsonwebtoken'); function authenticateToken(req, res, next) { const token = req.header('Authorization').split(' ')[1]; if (!token) return res.status(401).send('Access Denied'); jwt.verify(token, process.env.JWT_SECRET, (err, user) => { if (err) return res.status(403).send('Invalid Token'); req.user = user; next(); }); }
Task Routes:
const express = require('express'); const router = express.Router(); const Task = require('../models/Task'); router.get('/tasks', authenticateToken, async (req, res) => { const tasks = await Task.find({ tenant_id: req.user.tenant_id }); res.json(tasks); }); router.post('/tasks', authenticateToken, async (req, res) => { const task = new Task({ title: req.body.title, description: req.body.description, tenant_id: req.user.tenant_id, created_by: req.user.id, }); await task.save(); res.status(201).json(task); }); module.exports = router;
2. Integrating Third-Party APIs
Use Case 1: Payment Gateway (Stripe)
Scenario: Enable tenants to subscribe to premium plans.
Integration Steps:
Create a Stripe account and get an API key.
Implement payment endpoints.
Node.js Example:
const stripe = require('stripe')(process.env.STRIPE_SECRET_KEY);
app.post('/payments', async (req, res) => {
const session = await stripe.checkout.sessions.create({
payment_method_types: ['card'],
line_items: [{
price_data: {
currency: 'usd',
product_data: { name: 'Premium Plan' },
unit_amount: 5000,
},
quantity: 1,
}],
mode: 'payment',
success_url: 'https://example.com/success',
cancel_url: 'https://example.com/cancel',
});
res.json({ url: session.url });
});
Use Case 2: Social Login (Google OAuth)
- Scenario: Allow users to log in using Google accounts.
Python Example:
Install OAuth library:
pip install social-auth-app-django
Configure settings:
AUTHENTICATION_BACKENDS = [ 'social_core.backends.google.GoogleOAuth2', ] SOCIAL_AUTH_GOOGLE_OAUTH2_KEY = '<client-id>' SOCIAL_AUTH_GOOGLE_OAUTH2_SECRET = '<client-secret>'
3. Error Handling and Troubleshooting
- Centralized Error Handling: Define a middleware for catching and formatting errors.
Node.js Example:
app.use((err, req, res, next) => {
res.status(err.status || 500).json({ error: err.message });
});
- Monitoring API Errors: Integrate monitoring tools like Sentry or New Relic to track errors in production.
Part 6: Best Practices
This chapter focuses on the lessons learned from years of API development, the patterns that have stood the test of time, and the best practices that ensure your API is developer-friendly, scalable, and maintainable.
1. Developer-Centric API Design
APIs are products, and developers are your primary users. A well-designed API should be intuitive, predictable, and enjoyable to use.
Key Characteristics of Developer-Friendly APIs:
Consistency:
Uniform endpoint naming, response structures, and HTTP methods.
Example:
- Consistent verbs for actions:
POST /tasks
(create),GET /tasks
(read).
- Consistent verbs for actions:
Clear Documentation:
Use tools like Swagger or Postman to generate interactive documentation.
Include:
Endpoint descriptions.
Request/response examples.
Authentication details.
Ease of Integration:
Provide SDKs for popular languages (Python, JavaScript, PHP).
Example: Stripe SDKs for seamless integration.
Error Messages:
Error responses should be specific and actionable.
Example:
{ "error": "Invalid Parameter", "message": "The 'email' field is required.", "code": 400 }
2. Common Pitfalls to Avoid
Over-Engineering:
Avoid building overly generic or abstract APIs.
Example: Don't attempt to design a one-size-fits-all endpoint (
/generic-endpoint
).
Inconsistent Response Formats:
Avoid returning different response structures for similar actions.
Bad Example:
GET /tasks
returns a list.GET /users
returns a dictionary.
Ignoring Performance:
Example:
- An API that fetches all user data without pagination will quickly degrade as the dataset grows.
Neglecting Versioning:
Breaking changes without proper versioning can alienate users.
Always maintain backward compatibility for existing versions.
3. Advanced Best Practices
3.1. Design for Scale
Pagination:
Always paginate large datasets using query parameters:
GET /users?page=2&limit=50
Rate Limiting:
Protect your API from abuse:
HTTP/1.1 429 Too Many Requests Retry-After: 30
Caching:
Cache responses for commonly requested resources.
Example:
Use
Cache-Control
headers:Cache-Control: max-age=3600
3.2. Security
Authentication:
- Use OAuth2, JWT, or API keys for authentication.
Authorization:
- Implement role-based or attribute-based access control (RBAC/ABAC).
Input Validation:
Sanitize inputs to prevent injection attacks.
Example:
const sanitize = require('sanitize-html'); const safeInput = sanitize(userInput);
Secure Communication:
- Enforce HTTPS for all API communications.
3.3. Observability
Logging:
Log incoming requests, responses, and errors.
Structured logging in JSON format simplifies parsing.
Monitoring:
Use tools like Prometheus, Datadog, or ELK Stack to monitor:
Request latency.
Error rates.
Throughput.
Alerting:
Configure alerts for:
High error rates (e.g., 5xx responses).
Latency spikes.
4. Tips for Scaling API Teams
As teams grow, maintaining consistency and collaboration becomes challenging.
Coding Standards:
- Define and enforce guidelines for API design, naming conventions, and code formatting.
Collaboration Tools:
- Use tools like Postman Teams or SwaggerHub for collaborative API development.
Code Reviews:
- Peer reviews ensure quality and adherence to best practices.
5. Future-Proofing APIs
5.1. Emerging Trends
Serverless APIs:
- Use services like AWS Lambda or Azure Functions for lightweight, on-demand APIs.
Edge Computing:
- Shift processing closer to users with tools like Cloudflare Workers.
GraphQL Federation:
- Combine multiple GraphQL APIs into a unified schema for complex applications.
5.2. Evolving Standards
AsyncAPI:
- A specification for defining asynchronous APIs (e.g., WebSocket, Kafka).
OpenAPI 3.1:
- Enhanced support for JSON Schema and reusability.
6. Case Study: Crafting a Developer-Friendly API
Scenario: You are designing an API for a travel booking platform that aggregates flight, hotel, and car rental data. Your users are developers building integrations for travel apps and websites.
Challenges:
Complex relationships between resources (e.g., flights, hotels, and cars).
High volume of queries during peak booking seasons.
Real-time price updates.
Solutions:
Endpoint Design:
Flights:
GET /flights?origin=NYC&destination=SFO&date=2023-01-10
Hotels:
GET /hotels?location=SFO&checkin=2023-01-10&checkout=2023-01-15
Response Format:
{ "flights": [ { "id": "AA123", "departure": "2023-01-10T08:00:00", "arrival": "2023-01-10T11:30:00", "price": 350 } ] }
Caching and Rate Limiting:
Cache search results for 5 minutes.
Limit queries to 100 requests per minute.
Documentation:
- Provide Swagger-based interactive docs with example requests.
Monitoring:
- Track response times and alert for latencies above 1 second.
Part 7: Appendices
This section serves as a reference for quick lookups and additional resources. It includes glossaries, cheat sheets, recommended tools, and real-world examples.
1. Glossary of API Terms
Term | Definition |
API (Application Programming Interface) | A set of rules and tools for building and interacting with software applications. |
Endpoint | A specific URL where an API resource can be accessed. |
Resource | An object or entity exposed by an API (e.g., users, products). |
HTTP Methods | Standard verbs like GET , POST , PUT , and DELETE used to interact with API endpoints. |
JWT (JSON Web Token) | A compact, URL-safe token used for securely transmitting information between parties. |
Rate Limiting | Restricting the number of API requests a client can make within a specific timeframe. |
Stateless | The property that ensures each API request contains all the information needed to process it. |
Versioning | Managing API updates by assigning versions (e.g., /v1 , /v2 ). |
Payload | The data sent in an API request or response. |
2. HTTP Status Codes Cheat Sheet
Code | Category | Meaning |
200 | Success | The request was successful. |
201 | Created | A new resource was successfully created. |
204 | No Content | The request was successful, but there is no response body. |
400 | Bad Request | The server could not understand the request due to invalid syntax. |
401 | Unauthorized | Authentication is required. |
403 | Forbidden | The client does not have access rights to the resource. |
404 | Not Found | The requested resource could not be found. |
429 | Too Many Requests | The user has sent too many requests in a given timeframe. |
500 | Internal Server Error | The server encountered an unexpected condition. |
3. Common API Design Patterns
Pattern | Description |
CRUD | Basic operations for resources: Create, Read, Update, Delete. |
Pagination | Divide large datasets into smaller chunks using query parameters like page and limit . |
Filtering | Allow clients to filter data using query parameters (e.g., ?status=active ). |
Sorting | Enable sorting data (e.g., ?sort=price:asc ). |
Authentication | Secure the API using mechanisms like OAuth2, JWT, or API keys. |
Versioning | Introduce backward-compatible updates with versions (/v1 , /v2 ). |
HATEOAS | Include links in API responses to guide the client through possible actions. |
4. API Design Tools
Tool/Library | Purpose | Example Usage |
Postman | API testing and collaboration. | Create test collections and automate workflows. |
Swagger/OpenAPI | API documentation and design. | Generate interactive docs from OpenAPI specifications. |
Insomnia | API testing and debugging. | Test REST and GraphQL APIs with a user-friendly interface. |
API Gateway (AWS) | API management and scaling. | Deploy, monitor, and secure APIs on AWS infrastructure. |
Kong | API gateway for microservices. | Manage authentication, rate limiting, and analytics for APIs. |
5. Real-World Examples
Example 1: E-commerce API
Feature: Product Management
Endpoints:
GET /products
: Fetch all products.POST /products
: Add a new product (Admin only).PUT /products/{id}
: Update a product.DELETE /products/{id}
: Delete a product.
Sample Response:
{
"id": "12345",
"name": "Laptop",
"price": 999.99,
"status": "in stock"
}
Example 2: Social Media API
Feature: Post Management
Endpoints:
GET /posts
: Fetch all posts.POST /posts
: Create a new post.PUT /posts/{id}
: Update a post.DELETE /posts/{id}
: Delete a post.
Sample Response:
{
"id": "98765",
"content": "Hello World!",
"author": {
"id": "54321",
"name": "John Doe"
},
"created_at": "2023-01-01T12:00:00Z"
}
6. Learning Resources
Books:
API Design for Developers by Mark Richards.
Designing APIs with Swagger and OpenAPI by Josh Ponelat.
RESTful Web APIs by Leonard Richardson.
Courses:
Udemy: "REST API Design, Development & Management" by Sanjay Patel.
Coursera: "API Design and Fundamentals of Google Cloud's Apigee API Platform."
Documentation and Blogs:
Stripe API Docs: An example of excellent API documentation.
Postman Blog: API design, testing, and management tips.
7. Code Repositories
Python (Django REST Framework):
- GitHub: django-rest-framework-example
PHP (Laravel):
- GitHub: laravel-api-example
Node.js (Express):
- GitHub: node-express-api
GraphQL:
- GitHub: graphql-js
8. Cheat Sheet: HTTP Methods
Method | Purpose | Idempotent? | Safe? |
GET | Retrieve data | Yes | Yes |
POST | Create new resources | No | No |
PUT | Update existing resources | Yes | No |
PATCH | Partially update resources | No | No |
DELETE | Remove resources | Yes | No |
9. API Testing Checklist
Functional Testing:
Ensure endpoints return the correct data and status codes.
Example: Does
POST /tasks
return201 Created
?
Load Testing:
Test how the API performs under heavy traffic.
Tools: JMeter, K6.
Security Testing:
- Test for vulnerabilities (e.g., SQL injection, unauthorized access).
Integration Testing:
- Verify how different endpoints work together.
By working through this guide, you’ve gained a thorough understanding of the art and science of API design and development. From the fundamentals of RESTful architecture and advanced GraphQL queries to scaling strategies, security best practices, and real-world projects, you now possess the tools to tackle any API challenge.
API development is more than just a technical skill—it’s a craft that balances creativity, user empathy, and engineering rigor. The APIs you build are not just endpoints; they are bridges that connect ideas, businesses, and users. Whether you’re designing an API for your startup, enhancing an existing platform, or contributing to open-source projects, your work has the potential to shape the way applications interact and evolve.
Happy coding! 🚀
For more ebooks and guides, visit AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
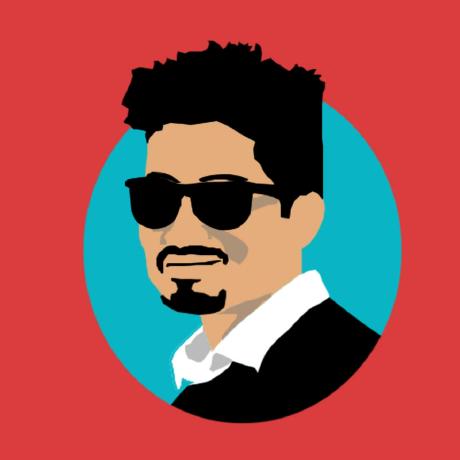