Building a Custom Dashboard UI for MS SQL: A Comprehensive Guide
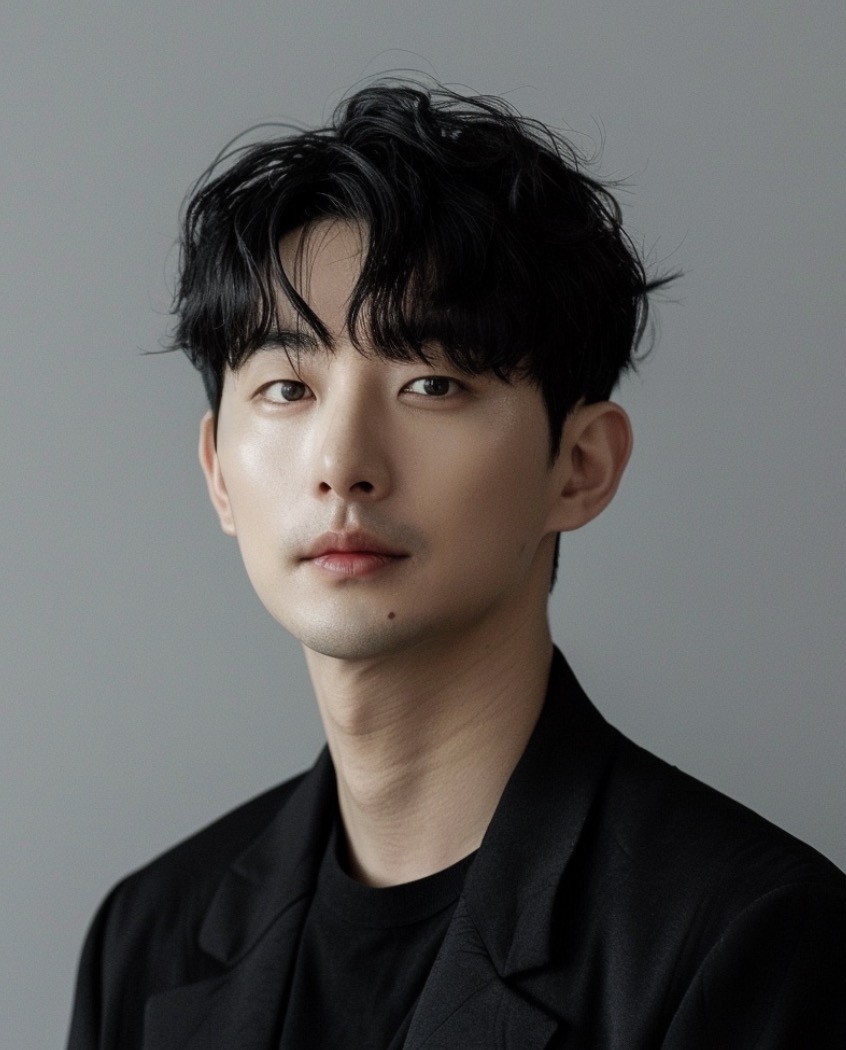

Learn how to design and develop a custom dashboard UI to effectively manage your SQL database. Explore popular technologies and frameworks for creating intuitive and functional dashboards.
The most effective way to create a Dashboard UI for SQL database management involves using modern web technologies and following established best practices. Here's a comprehensive guide:
Recommended Tech Stack
Frontend Framework
React with Next.js for robust UI development
Material-UI (MUI) or Shadcn for pre-built components
Chart.js or Nivo for data visualization
Backend Stack
Node.js with Express for API development
MongoDB or your existing SQL database
JWT for authentication
Key Features to Implement
Core Functionality
Data entry forms with input validation
Visual query builder for non-SQL users
Real-time data monitoring
Report generation capabilities
Essential UI Components
Responsive grid layout system
Light/dark mode theming
Interactive data tables
Customizable charts and graphs
Best Practices
Dashboard Organization
Place critical metrics at the top left
Group related cards and visualizations
Use consistent time granularity for data display
Break complex dashboards into multiple linked views
Performance Considerations
Implement data caching
Use pagination for large datasets
Optimize SQL queries for real-time updates
Consider data refresh intervals based on use case
Implementation Steps
// Example React component structure
import { useState, useEffect } from 'react';
import { DataGrid } from '@mui/x-data-grid';
import { Chart } from 'chart.js';
const Dashboard = () => {
const [data, setData] = useState([]);
useEffect(() => {
// Fetch data from your SQL database
const fetchData = async () => {
const response = await fetch('/api/data');
const result = await response.json();
setData(result);
};
fetchData();
}, []);
return (
<div className="dashboard-container">
<header>
<h1>Database Dashboard</h1>
</header>
<main>
<DataGrid
rows={data}
columns={columns}
pageSize={10}
/>
</main>
</div>
);
};
This approach provides a scalable, maintainable, and user-friendly dashboard interface for managing your SQL database while following modern development practices.
What are the best practices for designing a user-friendly dashboard using MS SQL and Next.js on Vercel
Architecture Best Practices
Core Components
Implement Server-Side Rendering (SSR) for faster initial page loads and better SEO
Use Next.js API routes to handle database queries securely
Leverage Vercel Postgres SDK for protected SQL queries to prevent injections
Data Fetching
// Optimize multiple SQL queries using Promise.all
async function fetchDashboardData() {
const [metrics, trends, details] = await Promise.all([
sql`SELECT COUNT(*) FROM metrics`,
sql`SELECT * FROM trends LIMIT 10`,
sql`SELECT * FROM details WHERE status = 'active'`
]);
return { metrics, trends, details };
}
UI Implementation
Layout Structure
// pages/dashboard/layout.tsx
export default function DashboardLayout({ children }) {
return (
<div className="min-h-screen">
<nav className="h-16 border-b">
<DashboardNav />
</nav>
<div className="flex">
<Sidebar />
<main className="flex-1 p-6">{children}</main>
</div>
</div>
);
}
Data Visualization
Use Recharts or Chart.js for interactive data visualization
Implement responsive grid layouts using Tailwind CSS
Group related metrics in cards for better organization
Performance Optimization
Key Considerations
Implement data caching to reduce database load
Use dynamic imports for heavy components
Add pagination for large datasets
Real-time Updates
// Implement SWR for real-time data fetching
const { data, error } = useSWR('/api/metrics', fetcher, {
refreshInterval: 5000,
revalidateOnFocus: true
});
Security Measures
Database Access
Use parameterized queries to prevent SQL injection
Implement role-based access control
Sanitize all user inputs before database operations
By following these practices, you'll create a robust, performant, and secure dashboard that effectively visualizes your SQL data while maintaining good user experience and performance.
Backend Database Boilerplate for MS SQL
Database Schema Setup
Core Tables Structure
CREATE TABLE users (
user_id INT IDENTITY(1,1) PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
created_at DATETIME DEFAULT GETDATE(),
status TINYINT DEFAULT 1
);
CREATE TABLE audit_logs (
log_id INT IDENTITY(1,1) PRIMARY KEY,
action_type VARCHAR(50) NOT NULL,
table_name VARCHAR(50) NOT NULL,
record_id INT NOT NULL,
changed_by INT REFERENCES users(user_id),
changed_at DATETIME DEFAULT GETDATE()
);
Node.js Implementation
Basic Server Setup
const express = require('express');
const sql = require('mssql');
const app = express();
const config = {
user: process.env.SQL_USER,
password: process.env.SQL_PASSWORD,
server: process.env.SQL_SERVER,
database: process.env.SQL_DATABASE,
options: {
encrypt: true,
enableArithAbort: true
}
};
// Database connection pool
const pool = new sql.ConnectionPool(config);
const poolConnect = pool.connect();
app.use(express.json());
// Global error handler for database connections
pool.on('error', err => {
console.error('Database connection error:', err);
});
Query Helper Function
async function executeQuery(query, params = []) {
await poolConnect;
try {
const request = pool.request();
params.forEach(param => {
request.input(param.name, param.type, param.value);
});
return await request.query(query);
} catch (err) {
throw new Error(`Database query error: ${err.message}`);
}
}
API Routes Implementation
Basic CRUD Operations
// Get all records
app.get('/api/:table', async (req, res) => {
try {
const result = await executeQuery(`SELECT * FROM ${req.params.table}`);
res.json(result.recordset);
} catch (err) {
res.status(500).json({ error: err.message });
}
});
// Get single record
app.get('/api/:table/:id', async (req, res) => {
try {
const result = await executeQuery(
`SELECT * FROM ${req.params.table} WHERE id = @id`,
[{ name: 'id', type: sql.Int, value: req.params.id }]
);
res.json(result.recordset[0]);
} catch (err) {
res.status(500).json({ error: err.message });
}
});
Performance Optimization
Key Considerations
Implement connection pooling for better resource management
Use parameterized queries to prevent SQL injection
Add appropriate indexes on frequently queried columns
Implement query caching for frequently accessed data
This boilerplate provides a secure and scalable foundation for building a Node.js backend with MS SQL Server integration, following modern development practices and security standards.
What are the essential components of a backend database boilerplate for MS SQL
Core Components
Database Connection Setup
const sql = require('mssql');
const config = {
user: process.env.DB_USER,
password: process.env.DB_PWD,
server: process.env.DB_SERVER,
database: process.env.DB_NAME,
pool: {
max: 10,
min: 0,
idleTimeoutMillis: 30000
},
options: {
encrypt: true,
trustServerCertificate: false
}
}
Error Handling Wrapper
const executeQuery = async (query, params) => {
try {
await sql.connect(config);
const request = new sql.Request();
const result = await request.query(query);
return result;
} catch (error) {
throw new Error(`Database Error: ${error.message}`);
} finally {
sql.close();
}
}
Data Management Layer
Base Repository Pattern
class BaseRepository {
async create(entity) {
const pool = await sql.connect(config);
const request = pool.request();
return request
.input('param1', sql.VarChar, entity.param1)
.query('INSERT INTO table_name (column1) VALUES (@param1)');
}
async getById(id) {
const pool = await sql.connect(config);
return pool.request()
.input('id', sql.Int, id)
.query('SELECT * FROM table_name WHERE id = @id');
}
}
Security Features
Authentication Middleware
const authMiddleware = async (req, res, next) => {
try {
const token = req.headers.authorization;
if (!token) {
throw new Error('No token provided');
}
// Verify token and set user context
next();
} catch (error) {
res.status(401).json({ error: 'Unauthorized' });
}
}
Performance Optimizations
Connection Pooling
Implement maximum pool size of 10 connections
Set idle timeout to 30 seconds
Configure minimum pool size to 0 for resource efficiency
These components provide a solid foundation for building a secure and scalable MS SQL database backend while following modern development practices and maintaining good performance characteristics.
What are the best practices to develop a Dashboard UI backend for MS SQL Database with Node.js server
Database Connection Setup
Connection Pool Management
const sql = require('mssql');
const config = {
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
server: process.env.DB_SERVER,
database: process.env.DB_NAME,
pool: {
max: 10,
min: 0,
idleTimeoutMillis: 30000
},
options: {
encrypt: true,
trustServerCertificate: true
}
}
Query Management Layer
Base Query Handler
class DatabaseService {
async executeQuery(query, params = []) {
try {
const pool = await sql.connect(config);
const request = pool.request();
params.forEach(param => {
request.input(param.name, param.type, param.value);
});
return await request.query(query);
} catch (error) {
throw new Error(`Database Error: ${error.message}`);
}
}
}
API Structure
Route Organization
const express = require('express');
const router = express.Router();
// Dashboard metrics endpoint
router.get('/metrics', async (req, res) => {
try {
const result = await executeQuery(`
SELECT
COUNT(*) as total_records,
SUM(value) as total_value
FROM your_table
WHERE created_at >= DATEADD(day, -7, GETDATE())
`);
res.json(result.recordset);
} catch (err) {
res.status(500).json({ error: err.message });
}
});
Performance Optimization
Key Practices
Implement connection pooling for efficient resource management
Use parameterized queries to prevent SQL injection
Cache frequently accessed data
Implement proper error handling and logging
Use stored procedures for complex queries
Security Implementation
Authentication Layer
const authMiddleware = async (req, res, next) => {
try {
const token = req.headers.authorization;
if (!token) {
return res.status(401).json({ error: 'No token provided' });
}
// Verify token logic here
next();
} catch (error) {
res.status(401).json({ error: 'Authentication failed' });
}
}
These practices ensure a robust, secure, and performant backend system for your dashboard UI while maintaining good development practices and security standards.
What are the best libraries for interacting with MS SQL in Node.js
Based on the search results, here are the most effective libraries for interacting with MS SQL in Node.js:
Primary Libraries
node-mssql
Built on top of Tedious driver
Provides promise-based API
Includes built-in connection pooling
Offers robust transaction support
Easy-to-use interface for database operations
Tedious
Pure JavaScript implementation
Works across Windows, macOS, and Linux
Default TDS driver for Node.js
Provides low-level control over database operations
Suitable for specialized SQL Server applications
ORM Solutions
Prisma
Modern database toolkit with TypeScript support
Type-safe API with auto-generated query builder
Strong maintenance and community support
Excellent for microservices architecture
Includes efficient database migrations
Sequelize
Mature and established ORM
Comprehensive feature set
Supports multiple database systems
Includes migrations and data validation
Large community and extensive documentation
Performance Considerations
Connection Management
const config = {
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
server: process.env.DB_SERVER,
database: process.env.DB_NAME,
pool: {
max: 10,
min: 0,
idleTimeoutMillis: 30000
},
options: {
encrypt: true,
trustServerCertificate: true
}
}
This configuration ensures optimal performance through connection pooling and proper security settings.
Subscribe to my newsletter
Read articles from Ewan Mak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
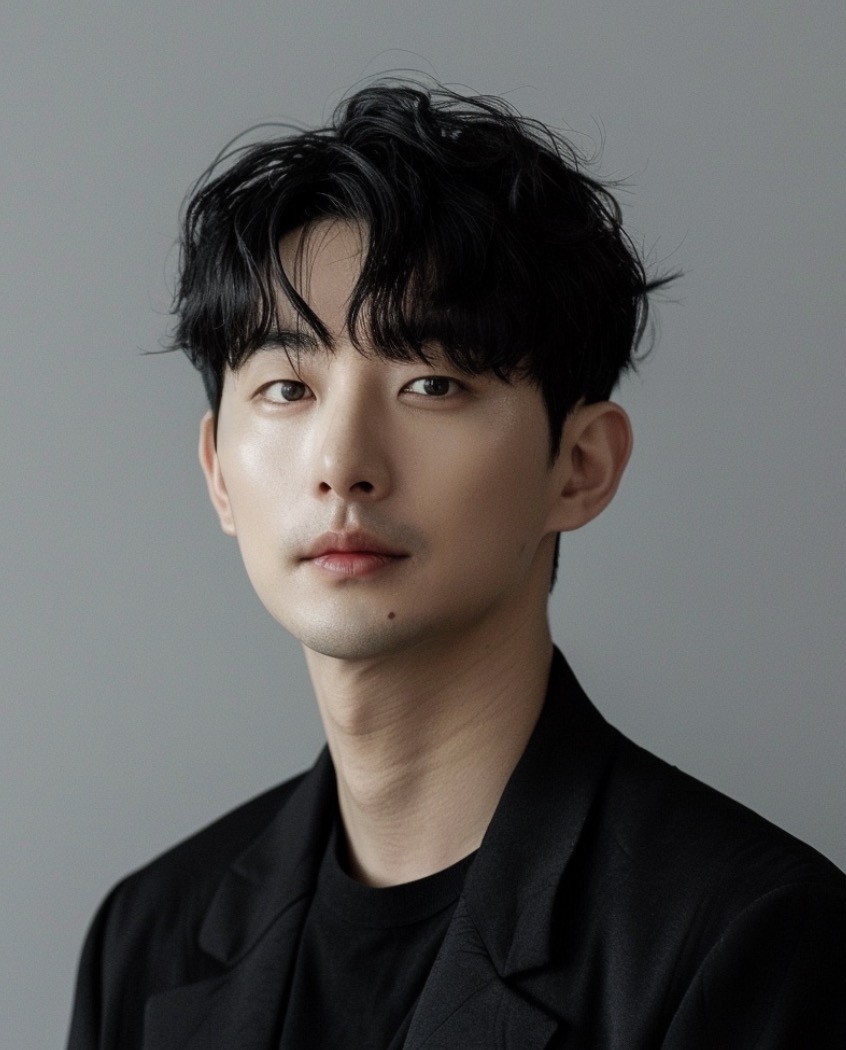
Ewan Mak
Ewan Mak
Crafting seamless user experiences with a passion for headless CMS, Vercel deployments, and Cloudflare optimization. I'm a Full Stack Developer with expertise in building modern web applications that are blazing fast, secure, and scalable. Let's connect and discuss how I can help you elevate your next project!