Flask-RESTful API Data
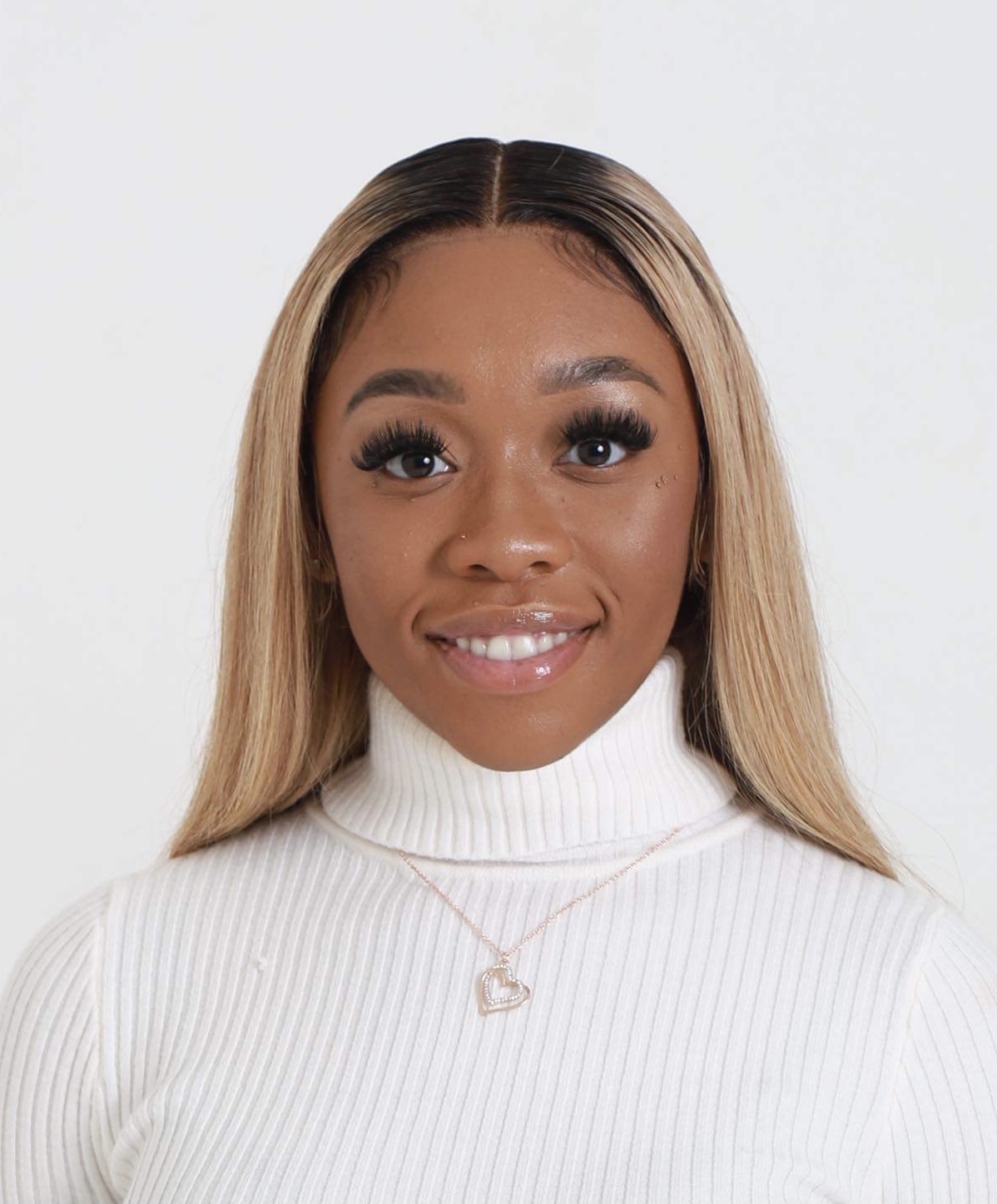

REST is short for Representational State Transfer, which is a convention that makes HTTP more readable and understandable. API is short for Application Programming Interface, which is an application that allows other applications to communicate with each other. Using Flask is a simpler way to create a RESTful API.
Routes and Actions
There are several actions that can be performed within a RESTful app to navigate and manipulate the application. We can create with a POST request, read with a GET request to either show all or just show one, update with a PATCH or PUT request or delete with a DELETE request.
GET Request
For example, if i wanted to create an application for people to shop for groceries we would need to first get access to all the products that the customer can choose from using a GET request.
from flask import Flask
from flask_restful import Api, Resource
from models import Product
app = Flask(__name__)
api = Api(app)
class Products(Resource):
def get(self):
products =[product.to_dict() for product in Product.query.all()]
return products, 200
api.add_resource(Products, '/products')
if __name__ == '__main__':
app.run(port=5555, debug=True)
Start by adding all the necessary imports. Api
is the constructor that is initialized with an instance and populated with resources that come from the Resource
class. The resource is added to the Api
via add_resource()
. Since we are utilizing the Resource
class the @app.route
decorator is not needed since the Resource
class basically handles the same job that the decorator does.
Once we finish with our imports we move on to retrieving the data, we can run a query on the imported Product
class to retrieve all the data for the products and use the data to return the response with the necessary status code. In this case the response needs to be successful so the status code will be 200.
POST Request
Let’s use the same example but this time we have new products in stock, this means that we now need to create a new product to be added to our database via a form on the front end.
def post(self):
new_record = Product(
name=request.form['name'],
price=request.form['price'],
)
db.session.add(new_record)
db.session.commit()
response_dict = new_record.to_dict()
return response_dict, 201
With this request we are creating a new record by retrieving data from the form that will be placed on the frontend of the application and using that data to add a new product to our session. We will then return the response with a 201 status code which indicates a created record.
PATCH Request
What if I mistakenly added the wrong price for the new product that i added or there is a typo in the name of the product? I will need to update the product with a PATCH request via a form of some sort as well.
def patch(self, id)
product = Product.query.filter(Product.id == id).first()
for attr in request.form:
setattr(product, attr, request.form.get(attr))
db.session.add(product)
db.session.commit()
response_dict = product.to_dict()
return response_dict, 200
We will need to first, target the ID of the product that needs to be updated and use the ID to filter all of the products in the Product class to get the first product that has a matching ID. Once we have found the correct product we can update the attribute in the form on the frontend of the application that was updated and return our response.
DELETE Request
I can also just delete the entire record to save time and create a new one later.
def patch(self, id)
product = Product.query.filter(Product.id == id).first()
db.session.delete(product)
db.session.commit()
response_body = {
"delete_successful": True,
"message": "Review deleted."
}
return response_body
A delete request is the simplest because the only thing we are doing is find the product we want to delete and simply deleting it from the database and returning the response.
Achieving each one of these will give you the ability to be able to manipulate and retrieve data from your RESTful API as you please so that we can use it to create a functional client side frontend.
Subscribe to my newsletter
Read articles from Dalecia Peterson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
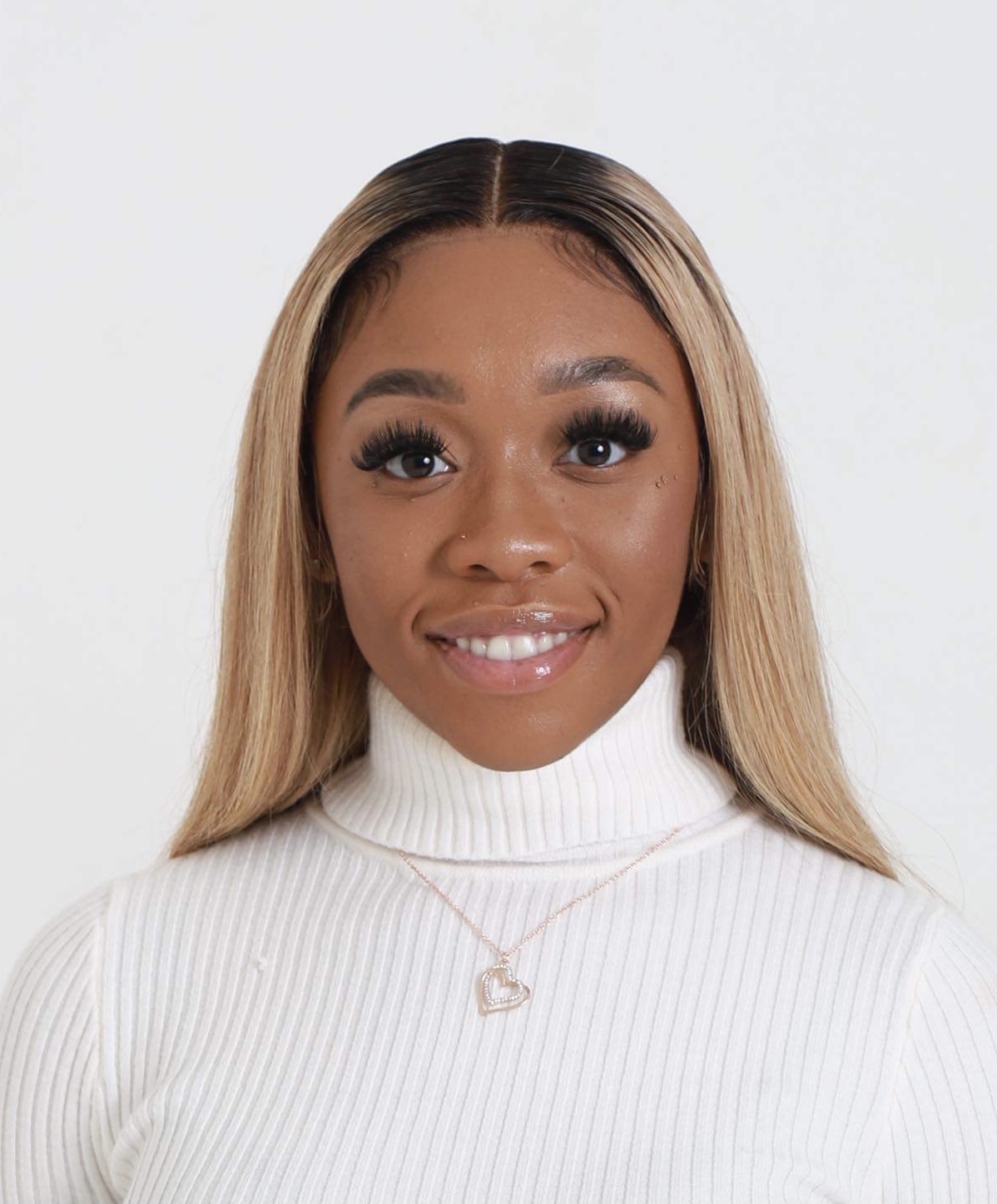
Dalecia Peterson
Dalecia Peterson
UNDER CONSTRUCTION