A Comprehensive Guide to Popular PHPUnit Assertions
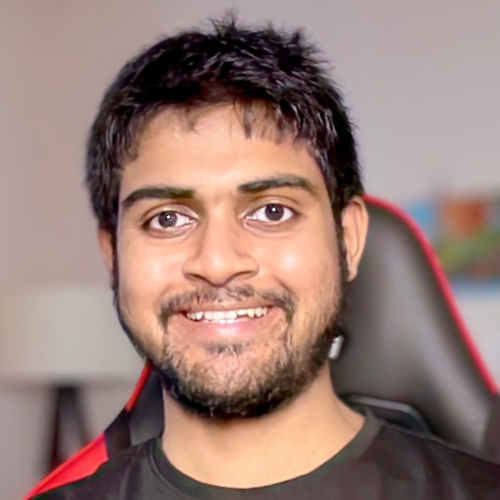
Table of contents
- 1. assertEquals
- 2. assertNotEquals
- 3. assertTrue
- 4. assertFalse
- 5. assertNull
- 6. assertNotNull
- 7. assertSame
- 8. assertNotSame
- 9. assertContains
- 10. assertNotContains
- 11. assertCount
- 12. assertEmpty
- 13. assertNotEmpty
- 14. assertGreaterThan
- 15. assertLessThan
- 16. assertInstanceOf
- 17. assertStringContainsString
- 18. assertStringNotContainsString
- 19. assertMatchesRegularExpression
- 20. assertDoesNotMatchRegularExpression
- 21. assertFileExists
- 22. assertFileDoesNotExist
- 23. assertDirectoryExists
- 24. assertDirectoryDoesNotExist
- 25. assertIsReadable

Assertions are the backbone of any testing framework, and PHPUnit is no exception. In PHPUnit, assertions are methods used to verify that the behavior of the code under test meets expectations. Understanding and using the right assertions is crucial for writing effective and maintainable tests.
This blog covers the most popular and widely used PHPUnit assertions, providing examples to help you master them.
1. assertEquals
The assertEquals
method checks if two values are equal. This is one of the most commonly used assertions.
Usage:
$this->assertEquals(5, 2 + 3);
Example:
public function testAddition() {
$result = 2 + 3;
$this->assertEquals(5, $result, 'The addition result should be 5.');
}
2. assertNotEquals
The assertNotEquals
method verifies that two values are not equal.
Usage:
$this->assertNotEquals(5, 2 * 3);
Example:
public function testSubtraction() {
$result = 10 - 3;
$this->assertNotEquals(5, $result, 'The result should not be 5.');
}
3. assertTrue
The assertTrue
method ensures that a given condition evaluates to true
.
Usage:
$this->assertTrue(5 > 3);
Example:
public function testBooleanCondition() {
$this->assertTrue(5 > 3, 'The condition should evaluate to true.');
}
4. assertFalse
The assertFalse
method ensures that a given condition evaluates to false
.
Usage:
$this->assertFalse(3 > 5);
Example:
public function testBooleanCondition() {
$this->assertFalse(3 > 5, 'The condition should evaluate to false.');
}
5. assertNull
The assertNull
method checks if a variable is null
.
Usage:
$this->assertNull(null);
Example:
public function testNullValue() {
$value = null;
$this->assertNull($value, 'The value should be null.');
}
6. assertNotNull
The assertNotNull
method verifies that a variable is not null
.
Usage:
$this->assertNotNull('Hello World');
Example:
public function testNotNullValue() {
$value = 'Hello World';
$this->assertNotNull($value, 'The value should not be null.');
}
7. assertSame
The assertSame
method ensures that two variables reference the same object or have the same value and type.
Usage:
$this->assertSame('5', '5');
Example:
public function testSame() {
$this->assertSame('5', '5', 'The values and types should match.');
}
8. assertNotSame
The assertNotSame
method ensures that two variables do not reference the same object or do not have the same value and type.
Usage:
$this->assertNotSame(5, '5');
Example:
public function testNotSame() {
$this->assertNotSame(5, '5', 'The value and type should not match.');
}
9. assertContains
The assertContains
method verifies that a value exists within a specified array or string.
Usage:
$this->assertContains('foo', ['foo', 'bar']);
Example:
public function testContains() {
$array = ['apple', 'banana', 'cherry'];
$this->assertContains('banana', $array, 'The array should contain "banana".');
}
10. assertNotContains
The assertNotContains
method ensures that a value does not exist within a specified array or string.
Usage:
$this->assertNotContains('pear', ['apple', 'banana', 'cherry']);
Example:
public function testNotContains() {
$array = ['apple', 'banana', 'cherry'];
$this->assertNotContains('pear', $array, 'The array should not contain "pear".');
}
11. assertCount
The assertCount
method verifies the number of elements in an array or countable object.
Usage:
$this->assertCount(3, ['apple', 'banana', 'cherry']);
Example:
public function testCount() {
$array = ['apple', 'banana', 'cherry'];
$this->assertCount(3, $array, 'The array should have 3 elements.');
}
12. assertEmpty
The assertEmpty
method checks if a variable is empty.
Usage:
$this->assertEmpty([]);
Example:
public function testEmpty() {
$array = [];
$this->assertEmpty($array, 'The array should be empty.');
}
13. assertNotEmpty
The assertNotEmpty
method checks if a variable is not empty.
Usage:
$this->assertNotEmpty(['apple']);
Example:
public function testNotEmpty() {
$array = ['apple'];
$this->assertNotEmpty($array, 'The array should not be empty.');
}
14. assertGreaterThan
The assertGreaterThan
method ensures that a value is greater than another value.
Usage:
$this->assertGreaterThan(5, 10);
Example:
public function testGreaterThan() {
$this->assertGreaterThan(5, 10, '10 should be greater than 5.');
}
15. assertLessThan
The assertLessThan
method ensures that a value is less than another value.
Usage:
$this->assertLessThan(10, 5);
Example:
public function testLessThan() {
$this->assertLessThan(10, 5, '5 should be less than 10.');
}
16. assertInstanceOf
The assertInstanceOf
method checks if an object is an instance of a specified class.
Usage:
$this->assertInstanceOf(MyClass::class, $object);
Example:
public function testInstanceOf() {
$object = new MyClass();
$this->assertInstanceOf(MyClass::class, $object, 'The object should be an instance of MyClass.');
}
17. assertStringContainsString
The assertStringContainsString
method ensures that a string contains a specific substring.
Usage:
$this->assertStringContainsString('world', 'Hello, world!');
Example:
public function testStringContainsString() {
$string = 'Hello, world!';
$this->assertStringContainsString('world', $string, 'The string should contain "world".');
}
18. assertStringNotContainsString
The assertStringNotContainsString
method ensures that a string does not contain a specific substring.
Usage:
$this->assertStringNotContainsString('error', 'All systems are operational.');
Example:
public function testStringNotContainsString() {
$string = 'All systems are operational.';
$this->assertStringNotContainsString('error', $string, 'The string should not contain "error".');
}
19. assertMatchesRegularExpression
The assertMatchesRegularExpression
method verifies that a string matches a given regular expression.
Usage:
$this->assertMatchesRegularExpression('/^[a-z]+$/', 'hello');
Example:
public function testRegexMatch() {
$string = 'hello';
$this->assertMatchesRegularExpression('/^[a-z]+$/', $string, 'The string should match the pattern.');
}
20. assertDoesNotMatchRegularExpression
The assertDoesNotMatchRegularExpression
method ensures that a string does not match a given regular expression.
Usage:
$this->assertDoesNotMatchRegularExpression('/^[0-9]+$/', 'hello');
Example:
public function testRegexNoMatch() {
$string = 'hello';
$this->assertDoesNotMatchRegularExpression('/^[0-9]+$/', $string, 'The string should not match the pattern.');
}
21. assertFileExists
The assertFileExists
method checks if a file exists.
Usage:
$this->assertFileExists('/path/to/file.txt');
Example:
public function testFileExists() {
$filePath = '/tmp/test-file.txt';
touch($filePath); // Create the file
$this->assertFileExists($filePath, 'The file should exist.');
}
22. assertFileDoesNotExist
The assertFileDoesNotExist
method verifies that a file does not exist.
Usage:
$this->assertFileDoesNotExist('/path/to/nonexistent-file.txt');
Example:
public function testFileDoesNotExist() {
$filePath = '/tmp/nonexistent-file.txt';
$this->assertFileDoesNotExist($filePath, 'The file should not exist.');
}
23. assertDirectoryExists
The assertDirectoryExists
method checks if a directory exists.
Usage:
$this->assertDirectoryExists('/path/to/directory');
Example:
public function testDirectoryExists() {
$dirPath = '/tmp/test-dir';
mkdir($dirPath); // Create the directory
$this->assertDirectoryExists($dirPath, 'The directory should exist.');
}
24. assertDirectoryDoesNotExist
The assertDirectoryDoesNotExist
method verifies that a directory does not exist.
Usage:
$this->assertDirectoryDoesNotExist('/path/to/nonexistent-directory');
Example:
public function testDirectoryDoesNotExist() {
$dirPath = '/tmp/nonexistent-dir';
$this->assertDirectoryDoesNotExist($dirPath, 'The directory should not exist.');
}
25. assertIsReadable
The assertIsReadable
method ensures that a file or directory is readable.
Usage:
$this->assertIsReadable('/path/to/readable-file.txt');
Example:
public function testIsReadable() {
$filePath = '/tmp/readable-file.txt';
touch($filePath); // Create the file
chmod($filePath, 0444); // Set it to be readable
$this->assertIsReadable($filePath, 'The file should be readable.');
}
Conclusion
With these 25 PHPUnit assertions, you'll have a comprehensive toolkit to test various conditions effectively. Use these assertions in your PHPUnit test cases to ensure the reliability and robustness of your code.
Let me know if there are additional edits or further clarifications required! 😊
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
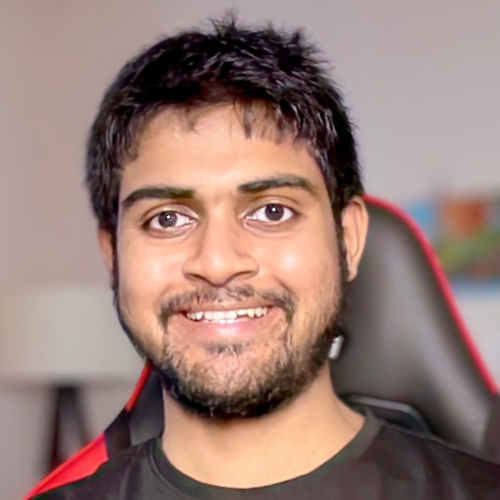
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.