Unlocking the Power of xAI in C# and F#: A Developer's Guide to Intelligent Programming and RAG
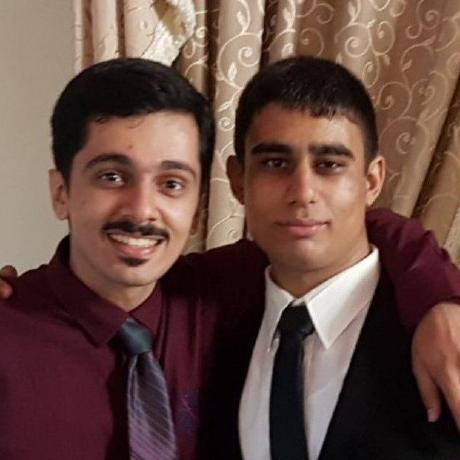

In the ever-evolving landscape of programming, where the quest for smarter, more efficient code never ceases, xAI Grok emerges as a beacon of innovation. For C# and F# developers, integrating xAI Grok isn't just about adding another tool to your arsenal; it's about redefining what's possible with AI-enhanced programming. This blog post is your gateway to understanding how xAI Grok can transform your C# and F# projects, making them not only more intelligent but also more intuitive.
Why Grok and Why Now?
The integration of artificial intelligence into programming isn't new, but with Grok, we're looking at a paradigm shift. Grok, developed by xAI, brings a level of understanding and interaction that feels almost human-like. For those of us in the dotnet community, this means we can now write code that not only performs tasks but also understands context, learns from data, and adapts to new information. Moreover, xAI has plans to add embedding models to their API, which promises to further enhance the capabilities of AI-driven development by allowing for more nuanced data processing and similarity searches.
Understanding RAG with Grok
Retrieval-Augmented Generation (RAG) is a technique that enhances the capabilities of large language models (LLMs) by integrating external knowledge bases into the generation process. RAG involves two main steps: retrieval, where relevant information is fetched from an external source based on a query, and generation, where this information is used to craft an answer or perform a task. Here's why Grok is particularly well-suited for RAG:
Real-Time Knowledge Access: Grok has the unique ability to access real-time data from the X platform, which means it can provide up-to-date responses by pulling the latest information into your code's decision-making process. This feature makes Grok particularly useful for applications needing current data, like financial market predictions or news analysis in real-time.
Enhanced Semantic Understanding: With Grok, the integration of RAG allows for deeper semantic understanding. For developers, this translates into writing code that can interpret and react to user queries with a nuanced understanding, leveraging external documents or databases for more accurate outputs. This is crucial for applications such as customer support bots or content summarization tools where context is key.
Adaptability and Learning: Grok's ability to learn and adapt based on new data or feedback is pivotal for RAG applications. In C# or F#, this means your applications can evolve with minimal retraining, simply by adjusting the knowledge bases or datasets Grok interacts with. This adaptability is beneficial for dynamic environments where data and requirements change frequently.
Efficiency in Development: Implementing RAG with Grok reduces the need for extensive model retraining when new information must be incorporated. Instead, developers can focus on expanding or refining data sources, which is less resource-intensive and allows for quicker iterations and updates in software development. The addition of embedding models will streamline this process even further by enabling more efficient data representation and similarity searches.
Ease of Integration: For C# and F# developers, Grok's API provides straightforward integration methods, making it less daunting to incorporate AI capabilities into existing projects. With the upcoming embedding models, developers will have even more tools at their disposal for complex semantic tasks, all accessible through a familiar API interface.
Conclusion
Grok by xAI stands out as an exemplary tool for RAG in the C# and F# ecosystems due to its real-time data access, semantic depth, adaptability, efficiency, and ease of integration. With plans to introduce embedding models to their API, xAI is set to enhance Grok's utility, promising developers a more robust toolkit for building intelligent, data-driven applications. Whether you're aiming to improve user experience through smarter applications or looking to automate complex data-driven tasks, Grok with RAG, and soon with embedding models, is your ally in making your code not just functional, but also intelligent and responsive to the ever-changing digital landscape.
The Journey Ahead
In this series, we will delve into:
How Grok API Works:
Understand the mechanics behind Grok's functionality.
Explore how Grok processes requests, interprets data, and responds to queries.
Introducing a Library for Grok:
Pure Way(Http client)
Present a dedicated C# library that simplifies the integration of Grok into your applications.
Discuss how this library can be used to manage API calls, handle responses, and maintain session states.
A Simple Example of RAG with C#:
Walk through a basic example of implementing Grok in a C# project.
From setup to execution, see how Grok can be utilized in a real-world scenario to enhance application functionality.
By the end of this series, you'll not only understand how Grok operates but also how to effectively incorporate it into your C# projects to create more dynamic and intelligent applications. Let's embark on this journey to elevate your programming with Grok.
How Grok API Works
How Grok API Works: Understand the mechanics behind Grok's functionality.
The Grok API is a sophisticated interface that leverages advanced natural language processing (NLP) and machine learning algorithms to interact with users. At its core, the API receives text inputs, which are then tokenized and analyzed for context, intent, and semantic meaning. This involves parsing the input to identify key elements like entities, actions, and relationships. Grok uses a combination of transformer models and custom-trained neural networks to understand complex queries, ensuring that the context of the conversation is maintained across multiple interactions. This process allows Grok to offer insightful, relevant, and often human-like responses by tapping into a vast database of knowledge and real-time web access.
Explore how Grok processes requests, interprets data, and responds to queries.
Once Grok receives a query, it initiates several steps to process the information. First, the query undergoes preprocessing to remove noise and normalize the text. Then, it's fed into the model where it's matched against learned patterns and data from its training. Grok interprets this data by applying layers of analysis, from basic syntactic understanding to deeper semantic interpretation. This includes sentiment analysis, entity recognition, and context-aware processing. After interpreting the data, Grok formulates a response by selecting and sometimes generating content that best fits the user's query. This response generation can involve pulling from pre-computed answers or dynamically creating new ones based on the latest information or user-specific data. The API then structures this response in a format that's easily consumable, whether it's simple text, JSON for more complex applications, or even multimedia content when applicable.
Introducing a Library for Grok
Http Client
Grok supports REST APIs, and you can use its REST API in C#. However, you should develop all API services and handle exceptions. You can see a sample code for these API calls. Next, we will introduce better ways to connect to Grok:
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
public class ApiService
{
private static readonly HttpClient client = new HttpClient
{
BaseAddress = new Uri("https://api.x.ai/v1/")
};
static ApiService()
{
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
// Configure other default headers as needed.
}
public async Task<MyResponseModel> CallApiAsync(string endpoint, object data)
{
var json = JsonConvert.SerializeObject(data);
var content = new StringContent(json, Encoding.UTF8, "application/json");
try
{
HttpResponseMessage response = await client.PostAsync(endpoint, content);
response.EnsureSuccessStatusCode();
var responseString = await response.Content.ReadAsStringAsync();
return JsonConvert.DeserializeObject<MyResponseModel>(responseString);
}
catch (HttpRequestException e)
{
// Log and rethrow or handle the exception as needed.
throw new Exception("Request failed: " + e.Message);
}
}
}
// A sample response
public class MyResponseModel
{
public string id { get; set; }
public string @object { get; set; }
public int created { get; set; }
public string model { get; set; }
public List<Choice> choices { get; set; }
public Usage usage { get; set; }
public string system_fingerprint { get; set; }
public class Choice
{
public int index { get; set; }
public Message message { get; set; }
public string finish_reason { get; set; }
}
public class Message
{
public string role { get; set; }
public string content { get; set; }
}
public class Usage
{
public int prompt_tokens { get; set; }
public int completion_tokens { get; set; }
public int total_tokens { get; set; }
}
}
Use 3rd party package
Grok does not have a dedicated library or package in any programming language; however, it illustrates how we can configure OpenAI's Python and JavaScript packages to access it. This method, with some modifications, can also be applied to the OpenAI package on NuGet, which I'll explain next. However, keep in mind that the APIs have some differences, and OpenAI currently offers more API endpoints than Grok. This discrepancy could lead to unexpected errors, so you should always test your code and data with Grok.
You must modify the endpoint configuration of OpenAI's package to access the xAI model, as demonstrated below. This involves changing the Endpoint and ensuring that the model parameter is set to use xAI's model name or identifier.
new OpenAIClient(new(openai_key), new() { Endpoint= new("https://api.x.ai/v1") })
// Get a xAI model
(new OpenAIClient(new(openai_key), new() { Endpoint= new("https://api.x.ai/v1") }))
.GetChatClient("grok-beta")
Compare this two method
The HTTP client method can be slow and cumbersome because you need to develop and manage all aspects of the connection yourself, including error handling for various scenarios. Alternatively, using the OpenAI Package is designed for OpenAI's services, and there's always the possibility that OpenAI might alter their APIs in ways that xAI does not support. However, we must acknowledge that the most straightforward and efficient approach would be for xAI to introduce a dedicated package for them on NuGet, which they have not done yet.
A Simple Example RAG with C#
I will not go into detailed explanations for each line of the sample code because I believe this would be tedious for my advanced and knowledgeable readers. However, if anyone wishes to understand it further, please do not hesitate to leave a comment.
A High-Level Explanation:
I loaded xAI_key from user secrets. This is a good practice as it ensures you never accidentally expose your secrets in the code.
Create an OpenAIClient with the xAI endpoint configuration and pass the xAI_key to it.
Get the 'grok-beta' model as a chat client.
Create a messages list and load messages from text files.
Send the request to Grok.
Save the response in 'grok-response.txt'.
using Microsoft.Extensions.Configuration;
using OpenAI.Chat;
using OpenAI;
var xAI_key = new ConfigurationBuilder().AddUserSecrets<Program>().Build()
.GetValue<string>("xAI-key") ?? "";
var client = (new OpenAIClient(new(xAI_key), new() { Endpoint = new("https://api.x.ai/v1") }))
.GetChatClient("grok-beta");
List<ChatMessage> messages =
[
new SystemChatMessage(File.ReadAllText("systemIntialMessage.txt")),
new UserChatMessage(File.ReadAllText("userMessage.txt")),
];
ChatCompletion completion = client.CompleteChat(messages);
File.WriteAllText("grok-response.txt",completion.Content[0].Text);
You can find the full code in this repository. In this sample, I instructed Grok to act as a Persian phone salesman, comparing the Samsung Galaxy A54 with the Xiaomi Poco M3.
You can watch a full view of it in the Youtube:
In the end
As we've journeyed through the innovative landscape of xAI Grok and its integration into C# and F# programming, it's clear that we're on the cusp of a new era in software development. Grok isn't just another AI tool; it's a transformative force that brings a level of intelligence, adaptability, and real-time interaction previously unseen in the dotnet ecosystem.
By harnessing the power of Retrieval-Augmented Generation (RAG), Grok allows developers to craft applications that not only understand and respond to user queries with remarkable precision but also evolve with the ever-changing data landscape. The upcoming integration of embedding models promises to further enhance this capability, making semantic understanding and data processing even more intuitive and efficient.
For C# and F# developers, Grok represents more than just an API to call; it's a partner in the creative process, enabling you to build applications that are not only functional but also intelligent, dynamic, and deeply connected to the real world. The examples and insights we've explored illustrate the potential to revolutionize how we think about software development, pushing the boundaries of what's possible with AI.
As you continue to explore Grok's capabilities, remember that this is just the beginning. The potential applications are vast, from enhancing user experiences in customer service to powering sophisticated data analysis tools. The journey with Grok is an ongoing adventure, one where your creativity as a developer can truly shine, making your code not just perform tasks but also understand, learn, and adapt in real-time.
Embrace Grok, engage with the community, and let's redefine programming together, making our applications smarter, more responsive, and infinitely more capable. The future of programming with AI isn't just on the horizon; it's here, and it's Grok.
If you have any questions about it you can ask them in the comments.
Subscribe to my newsletter
Read articles from behrooz bozorgchamy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
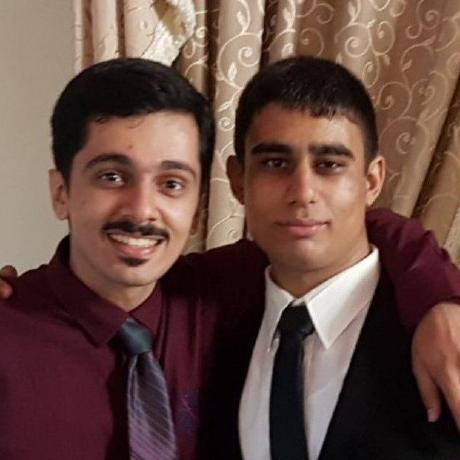