Building Dynamic React Forms: A Step-by-Step Guide
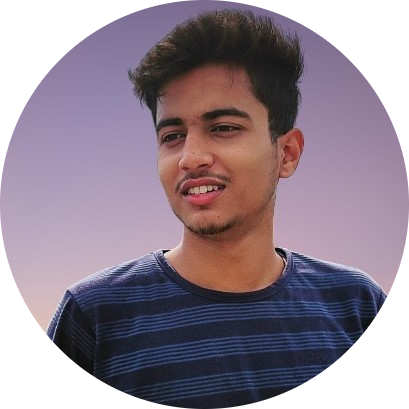

Dynamic forms are a powerful feature in web applications, enabling developers to create user interfaces that adapt to users' input and conditions. React, a popular JavaScript library, simplifies building such forms with its component-based architecture. This guide will walk you through creating dynamic forms in React that can change in real-time based on user interactions.
Why Use Dynamic Forms?
Dynamic forms provide flexibility and a better user experience by allowing content to be added, removed, or changed without needing to reload the page or navigate elsewhere. These forms are ideal for applications that require users to submit different sets of data or where the form fields are conditional based on previous inputs.
Key Benefits:
User-Friendliness: Dynamic forms simplify data input by showing relevant fields only.
Efficiency: Users complete forms faster as only the necessary fields are shown.
Scalability: Dynamic forms can handle complex data structures, making them great for enterprise-level applications.
Setting Up a Basic React App
To start, set up a new React project using create-react-app
:
npx create-react-app dynamic-form-demo
cd dynamic-form-demo
npm start
This creates a basic React environment to build your dynamic form.
Creating the Form Component
Define the Form State: Use React’s
useState
hook to manage form data and conditional rendering.Render Form Fields Dynamically: Use
map()
to iterate over an array of form fields and render them.
Here's a simple example:
import React, { useState } from 'react';
const DynamicForm = () => {
const [formData, setFormData] = useState([
{ name: 'field1', label: 'Field 1', type: 'text', value: '' },
{ name: 'field2', label: 'Field 2', type: 'number', value: '' },
]);
const handleInputChange = (e, index) => {
const updatedFormData = [...formData];
updatedFormData[index].value = e.target.value;
setFormData(updatedFormData);
};
return (
<form>
{formData.map((field, index) => (
<div key={field.name}>
<label>{field.label}</label>
<input
type={field.type}
value={field.value}
onChange={(e) => handleInputChange(e, index)}
/>
</div>
))}
</form>
);
};
export default DynamicForm;
Explanation:
State: The
formData
array holds form field objects with properties likename
,label
,type
, andvalue
.Input Handling: The
handleInputChange
function updates the state when a user types in the input fields.
Adding New Form Fields Dynamically
To make the form truly dynamic, add a button that appends new form fields when clicked:
const addField = () => {
setFormData([
...formData,
{ name: `field${formData.length + 1}`, label: `Field ${formData.length + 1}`, type: 'text', value: '' },
]);
};
// Add a button to your form JSX:
<button type="button" onClick={addField}>Add Field</button>
Conditional Rendering of Fields
Sometimes, showing certain fields only when specific criteria are met is necessary. This can be done using conditional logic inside the JSX:
<form>
{formData.map((field, index) => (
<div key={field.name}>
<label>{field.label}</label>
<input
type={field.type}
value={field.value}
onChange={(e) => handleInputChange(e, index)}
/>
{field.name === 'field2' && field.value > 10 && (
<div>
<label>Additional Field</label>
<input type="text" placeholder="Conditionally shown" />
</div>
)}
</div>
))}
</form>
Explanation:
- Conditional Check: If
field2
’s value is greater than 10, an additional input is displayed.
Validating Dynamic Form Fields
Form validation ensures that users provide the correct input. You can include simple validations directly in the handleInputChange
function or create separate validation logic:
const validateField = (field, value) => {
if (field.type === 'number' && value < 0) {
alert('Number cannot be negative');
}
};
Invoke validateField
inside handleInputChange
:
const handleInputChange = (e, index) => {
const updatedFormData = [...formData];
updatedFormData[index].value = e.target.value;
validateField(updatedFormData[index], e.target.value);
setFormData(updatedFormData);
};
Styling the Form
Add CSS to make your form look better:
form {
display: flex;
flex-direction: column;
gap: 10px;
}
label {
font-weight: bold;
}
input {
padding: 5px;
border: 1px solid #ccc;
border-radius: 4px;
}
Include the CSS in your component file or use styled-components for modular styling.
Conclusion
Dynamic forms in React offer an interactive user experience and simplify data collection by showing only relevant input fields. By using React’s state and hooks like useState
, you can build adaptable and user-friendly forms that respond to user actions seamlessly.
Subscribe to my newsletter
Read articles from Saurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
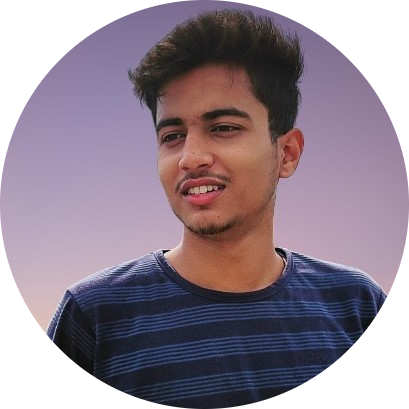
Saurav Kumar
Saurav Kumar
Build WebApps | Powered by Next.js, Node.js, Express.js, MongoDB & many more Styling, Auth, Db, Security, Deployment Tools & Techs