Building a Dockerfile on Docker for Desktop (Windows) to Run Python Script and Capture Output in a File

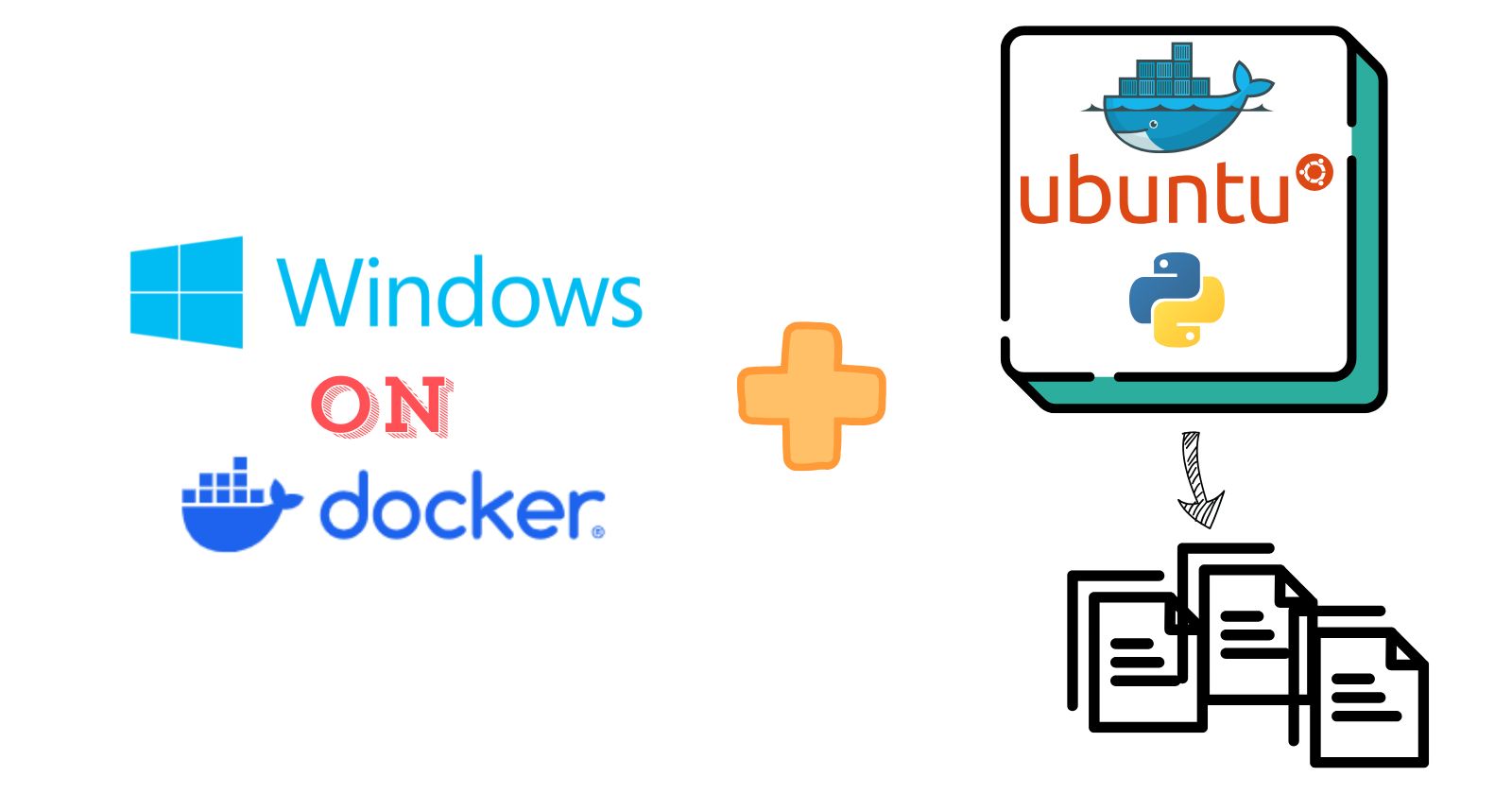
Let’s break down each section step by step
Build Docker file
1. FROM ubuntu:
FROM ubuntu
2. Install Python and pip:
RUN apt-get update && apt-get install -y python3 python3-venv
apt-get update
: Updates the package list to make sure we get the latest versions of the packages available.apt-get install -y python3 python3-venv
: Installs Python 3 and the virtual environment (python3-venv
).-y
flag is used to automatically confirm installations without user input.
3. Create and set up the virtual environment:
RUN python3 -m venv /opt/venv
python3 -m venv /opt/venv
: Creates a virtual environment at/opt/venv/
inside the container.A virtual environment helps isolate dependencies from the host system, ensuring that only the packages necessary for the application are available.
4. Set environment PATH:
ENV PATH="/opt/venv/bin:$PATH"
This ensures that the Python virtual environment is added to the system's
PATH
.Any command that runs within the container now uses the virtual environment Python (
/opt/venv/bin/python
) rather than the system's default Python.
5. Set working directory:
WORKDIR /opt
Sets
/opt
as the working directory.Any subsequent instructions that copy files or run commands will execute in this directory.
6. Copy the application file (hello-world.py
):
COPY hello-world.py /opt/
- Copies the
hello-world.py
file from the host into the/opt/
directory inside the container.
7. Set entry point to run the script using the virtual environment:
ENTRYPOINT ["python", "hello-world.py"]
This specifies that when the container starts, the
python
interpreter from the virtual environment is used to runhello-world.py
.No need to specify full paths to Python as the virtual environment is included in the PATH environment variable.
Create a file named Dockerfile in any folder on your Windows system and paste the following code into it.
FROM ubuntu
# Update and install Python and pip
RUN apt-get update && apt-get install -y python3 python3-venv
# Create a virtual environment
RUN python3 -m venv /opt/venv
# Activate the virtual environment (set PATH for subsequent layers)
ENV PATH="/opt/venv/bin:$PATH"
# Copy application files
WORKDIR /opt
COPY hello-world.py /opt/hello-world.py
# Use the virtual environment for the ENTRYPOINT
ENTRYPOINT ["/opt/venv/bin/python", "hello-world.py"]
Create python script
Create a sample python script and some code in it and place the file where the Dockerfile is kept
Build Docker file
Open Docker for desktop application on Windows and click on terminal. It will open.
Go to directory where docker file and python script is kept
Run below comamnd in terminal and you will be in that directory
cd C:\Users\Admin\Desktop\DevOps\DockerHello
Run build command to build docker
docker build . -t run_my_python_script
Build completed
Run the container
After running the container the script output will be written to file which will be at current directory where Docker file and Python script is kept
docker run run_my_python_script > output.log
As run successfully
Output file created
Output is written to file
If you found my post helpful, feel free to show your support by buying me a coffee! ☕
Subscribe to my newsletter
Read articles from vikas bhaskar vooradi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

vikas bhaskar vooradi
vikas bhaskar vooradi
In my free time, I enjoy coding, blogging, and exploring technology-related content on the internet.