Catching up with Tests in Security lib
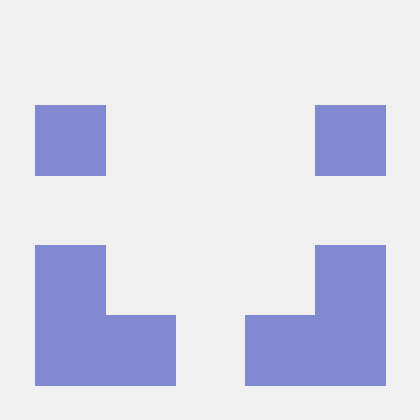
Hi. This is the twenty-second part of the diary about developing the “Programmers’ diary” blog. Previous part: https://hashnode.programmersdiary.com/catching-up-with-tests-in-programmers-diary-project. The open source code of this project is on https://github.com/TheProgrammersDiary. The first diary entry explains why this project was done: https://medium.com/@vievaldas/developing-a-website-with-microservices-part-1-the-idea-fe6e0a7a96b5.
Next entry:
—————
2024-01-14
Let’s continue catching up with tests, this time in Security lib.
Since Redis is used for token blacklisting we need an embedded Redis to test the functionality.
Let’s update pom.xml:
<dependency>
<groupId>it.ozimov</groupId>
<artifactId>embedded-redis</artifactId>
<version>0.7.3</version>
<scope>test</scope>
</dependency>
Also, we want to add jococo as before, to see the test coverage analysis:
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.11</version>
<configuration>
<excludes>
<exclude>com/evalvis/security/User.class</exclude>
</excludes>
</configuration>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>generate-code-coverage-report</id>
<phase>test</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
<execution>
<id>check</id>
<phase>test</phase>
<goals>
<goal>check</goal>
</goals>
<configuration>
<rules>
<rule>
<element>BUNDLE</element>
<limits>
<limit>
<counter>LINE</counter>
<value>COVEREDRATIO</value>
<minimum>0.86</minimum>
</limit>
<limit>
<counter>BRANCH</counter>
<value>COVEREDRATIO</value>
<minimum>0.86</minimum>
</limit>
</limits>
</rule>
</rules>
</configuration>
</execution>
</executions>
</plugin>
User.class does not have any relevant logic, therefore we don’t want it to count towards coverage. So we exclude it from jacoco analysis.
Let’s start by adding integration tests for login related functionality. Here is the ITTests class:
@SpringBootTest(classes = TestRedisConfig.class)
@ActiveProfiles("it")
public class ITTests {
@Autowired
private JwtKey key;
@Autowired
private BlacklistedJwtTokenRedisRepository blacklistedJwtTokenRedisRepository;
@Autowired
private JwtFilter jwtFilter;
@Test
void authenticates() {
JwtToken token = JwtToken.create(
new FakeAuthentication("tester1", null), key.value(), blacklistedJwtTokenRedisRepository
);
login(token);
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
assertEquals("tester1", ((User) authentication.getPrincipal()).getUsername());
assertTrue(authentication.isAuthenticated());
}
@Test
void blacklistsToken() {
JwtToken token = JwtToken.create(
new FakeAuthentication("tester2", null), key.value(), blacklistedJwtTokenRedisRepository
);
blacklistedJwtTokenRedisRepository.blacklistToken(token);
assertTrue(blacklistedJwtTokenRedisRepository.isTokenBlacklisted(token.value()));
login(token);
assertNull(SecurityContextHolder.getContext().getAuthentication());
}
private void login(JwtToken token) {
try {
jwtFilter.doFilterInternal(
new FakeHttpServletRequest(new Cookie[]{new Cookie("jwt", token.value())}),
new FakeHttpServletResponse(),
new FakeFilterChain()
);
} catch (ServletException | IOException e) {
throw new RuntimeException(e);
}
}
@Test
void doesNotRemoveValidBlacklistedTokens() {
JwtToken token = JwtToken.create(
new FakeAuthentication("tester", null), key.value(), blacklistedJwtTokenRedisRepository
);
blacklistedJwtTokenRedisRepository.blacklistToken(token);
blacklistedJwtTokenRedisRepository.removeExpiredTokens();
assertTrue(blacklistedJwtTokenRedisRepository.isTokenBlacklisted(token.value()));
}
}
First we test if by providing a valid JWT token the lib authenticates us.
Then we check if blacklisting works, and if non-expired tokens are not accidentally removed by functionality which removes expired tokens (removing blacklisted non-expired tokens (which indicates the user of that token logged out previously) poses a security risk, since then a logged out user would be logged in again allowing an intruder having that token to manipulate user data).
We need to add embedded redis host and port in application-it.properties:
spring.main.banner-mode=off
spring.data.redis.host=localhost
spring.data.redis.port=6370
jwt512=b936cee86c9f87aa5d3c6f2e84cb5a4239a5fe50480a6ec66b70ab5b1f4ac6730c6c515421b327ec1d69402e53dfb49ad7381eb067b338fd7b0cb22247225d47
We also need to provide a JWT key value for JwtKey class.
We also need a config for testing TestRedisConfig:
@Configuration
@ComponentScan("com.evalvis.security")
public class TestRedisConfig {
@Value("${spring.data.redis.port}") String port;
private RedisServer redisServer;
@Bean
public LettuceConnectionFactory redisConnectionFactory() {
RedisStandaloneConfiguration redisConfiguration = new RedisStandaloneConfiguration(
"localhost", Integer.parseInt(port)
);
return new LettuceConnectionFactory(redisConfiguration);
}
@Bean
public StringRedisTemplate stringRedisTemplate() {
return new StringRedisTemplate(redisConnectionFactory());
}
@PostConstruct
public void postConstruct() {
redisServer = RedisServer.builder().port(Integer.parseInt(port)).setting("maxmemory 128M").build();
redisServer.start();
}
@PreDestroy
public void preDestroy() {
redisServer.stop();
}
}
By using this config we are able to setup a fake redis server.
We are done with integration tests.
Let’s also improve unit tests in JwtTokenTest:
public class JwtTokenTest {
private static final SecretKey key = Keys.hmacShaKeyFor(
Decoders.BASE64.decode(
"b936cee86c9f87aa5d3c6f2e84cb5a4239a5fe50480a6ec66b70ab5b1f4ac6730c6c51542" +
"1b327ec1d69402e53dfb49ad7381eb067b338fd7b0cb22247225d47"
)
);
private final BlacklistedJwtTokenRepository blacklistedJwtTokenRepository = new BlacklistedJwtTokenFakeRepository();
@Test
void createsToken() {
JwtToken token = JwtToken.create(
new FakeAuthentication("tester", null), key, blacklistedJwtTokenRepository
);
assertAll(
() -> assertEquals("tester", token.username()),
() -> assertTrue(token.expirationDate().after(new Date(System.currentTimeMillis()))),
() -> assertTrue(token.tokenIsValid())
);
}
@Test
void readsExistingTokenFromAuthorizationHeader() {
HttpServletRequest httpRequest = new FakeHttpServletRequest(
Map.of(
"Authorization",
"Bearer " +
JwtToken.create(
new FakeAuthentication("tester", null),
key,
blacklistedJwtTokenRepository
).value()
)
);
Optional<JwtToken> token = JwtToken.existing(httpRequest, key, blacklistedJwtTokenRepository);
assertTrue(token.isPresent());
assertEquals("tester", token.get().username());
assertTrue(token.get().expirationDate().after(new Date(System.currentTimeMillis())));
assertTrue(token.get().tokenIsValid());
}
@Test
void readsExistingTokenFromCookies() {
HttpServletRequest httpRequest = new FakeHttpServletRequest(
new Cookie[]{
new Cookie(
"jwt",
JwtToken.create(
new FakeAuthentication("tester", null),
key,
blacklistedJwtTokenRepository
).value()
)
}
);
Optional<JwtToken> token = JwtToken.existing(httpRequest, key, blacklistedJwtTokenRepository);
assertTrue(token.isPresent());
assertEquals("tester", token.get().username());
assertTrue(token.get().expirationDate().after(new Date(System.currentTimeMillis())));
assertTrue(token.get().tokenIsValid());
}
@ParameterizedTest
@ValueSource(strings = {
"",
"short",
"eyJhbGciOiJIUzUxMiJ9.eyJzdWIiOiJ0ZXN0ZXIiLCJpYXQiOjE3MDUxNjg1OTQsI" +
"mV4cCI6MTcwNTE2OTE5NH0.0BKl5k3IJ7PlfpuuPjRO0qqb7_IVal6IqqjvkPLm3Yhk" +
"rqsn5SMYtkNqN321ChofuxvhhfdfJdROuh10_hyflg" // expired token
})
void rejectsInvalidToken(String jwt) {
// Token is neither in Auth header nor cookies.
assertFalse(
JwtToken.existing(
new FakeHttpServletRequest(Map.of(), null), key, blacklistedJwtTokenRepository
).isPresent()
);
// Token is in Auth header.
assertFalse(
JwtToken.existing(
new FakeHttpServletRequest(Map.of("Authorization", jwt)),
key,
blacklistedJwtTokenRepository
).isPresent()
);
// Token is in cookies.
assertFalse(
JwtToken.existing(
new FakeHttpServletRequest(new Cookie[]{new Cookie("jwt", jwt)}),
key,
blacklistedJwtTokenRepository
)
.get()
.tokenIsValid()
);
}
}
New tests to strengthen security are added (if JWT is malformed, non-existent, the lib should not authenticate the user). Also note, that these tests cover the integration tests: if there is a bug and unit test can catch it we will know the exact location of the bug and we won’t need running ITs.
You can check the whole code in commit: https://github.com/TheProgrammersDiary/Security/commit/55fdaf9f15855333e1bbf13ad72ce2a7dac5f914. Also, since changes were made the lib version was updated from 2.2.1 to 2.2.2 (since tests were added - there is no new functionality, let’s consider this update as patching).
Security lib tests updates are done.
Now let’s add more tests to blog (our monolith).
We add the same jacoco plugin (with different excluded classes).
First let’s add unit tests:
@ExtendWith({SnapshotExtension.class})
public class CommentTests {
private Expect expect;
private final CommentController controller;
private final CommentMother mother;
public CommentTests() {
this.controller = new CommentController(new FakeCommentRepository());
this.mother = new CommentMother(this.controller);
}
@Test
void createsComment() {
CommentRepository.CommentEntry comment = mother.create();
expect.toMatchSnapshot(jsonWithMaskedProperties(comment, "id"));
}
private <T> ObjectNode jsonWithMaskedProperties(
T object, String... properties
) {
ObjectNode node = new ObjectMapper().valueToTree(object);
Arrays.stream(properties).forEach(property -> node.put(property, "#hidden#"));
return node;
}
@Test
void findsPostComments() {
List<CommentRepository.CommentEntry> savedComments = mother.createPostComments("postId");
List<CommentRepository.CommentEntry> foundComments = new ArrayList(
controller.listCommentsOfPost("postId").getBody()
);
Assertions.assertThat(foundComments)
.extractingResultOf("toString")
.containsExactlyInAnyOrderElementsOf(
savedComments.stream().map(CommentRepository.CommentEntry::toString).toList()
);
}
}
Each endpoint is tested. Note that our unit tests are large scope: the entry point is the controller. That abstracts us from the implementation details and reduces the time we will need to update the tests when implementation details change. Controller is less likely to change than the implementation classes.
Now, the new IT tests:
@SpringBootTest(classes = ITUserTestConfig.class)
@ActiveProfiles("it")
@ExtendWith({SnapshotExtension.class})
public class ITUserTests {
private Expect expect;
private final UserController controller;
private final UserRepository repository;
private final BlacklistedJwtTokenRepository blacklistedJwtTokenRepository;
private final OAuth2AuthorizationSuccessHandler oAuth2AuthorizationSuccessHandler;
private final UserMother mother;
@Autowired
public ITUserTests(
UserController controller, UserRepository repository,
BlacklistedJwtTokenRepository blacklistedJwtTokenRepository,
OAuth2AuthorizationSuccessHandler oAuth2AuthorizationSuccessHandler
) {
this.controller = controller;
this.repository = repository;
this.blacklistedJwtTokenRepository = blacklistedJwtTokenRepository;
this.oAuth2AuthorizationSuccessHandler = oAuth2AuthorizationSuccessHandler;
this.mother = new UserMother(this.controller);
}
@AfterEach
void cleanUp() {
SecurityContextHolder.getContext().setAuthentication(null);
SecurityContextHolder.clearContext();
}
@Test
void signsUp() {
mother.signUp("tester");
assertTrue(repository.findByUsername("tester").isPresent());
}
@Test
void logsIn() {
mother.loginNewUser();
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
assertNotNull(authentication);
assertTrue(authentication.isAuthenticated());
}
@Test
void logsOut() {
String jwt = mother
.loginNewUser()
.getHeader(HttpHeaders.SET_COOKIE)
.split("jwt=")[1]
.split(";")[0];
controller.logout(new FakeHttpServletRequest(Map.of("Authorization", "Bearer " + jwt)));
assertTrue(blacklistedJwtTokenRepository.isTokenBlacklisted(jwt));
}
@Test
void logsInViaOAuth2() throws IOException {
String username = UUID.randomUUID().toString();
DefaultOidcUser user = new DefaultOidcUser(
Collections.emptyList(),
new OidcIdToken(
"fakeToken", Instant.now(), Instant.now().plus(Duration.ofMinutes(10)),
Map.of("email", UUID.randomUUID().toString(), "name", username, "sub", username)
)
);
We test signIn/login/logOut/loginViaOAuth2 scenarios. We also add necessary fakes.
We also need to provide a jwt key in application-it.properties:
spring.main.banner-mode=off
jwt512=b936cee86c9f87aa5d3c6f2e84cb5a4239a5fe50480a6ec66b70ab5b1f4ac6730c6c515421b327ec1d69402e53dfb49ad7381eb067b338fd7b0cb22247225d47
We need Test config to simulate repositories in unit tests:
@Configuration
@EnableAutoConfiguration(exclude = DataSourceAutoConfiguration.class)
@ComponentScan("com.evalvis.security")
@Import(value = {
UserController.class, SecurityConfig.class, UserDetailsServiceImpl.class, HttpLoggingFilter.class, JwtKey.class
})
public class ITUserTestConfig {
@Bean
public UserRepository fakeUserRepository() {
return new FakeUserRepository();
}
@Bean
public ClientRegistrationRepository fakeClientRegistrationRepository() {
return new FakeClientRegistrationRepository();
}
@Bean
@Primary
public BlacklistedJwtTokenRepository fakeBlacklistedJwtTokenRepository() {
return new FakeBlacklistedJwtTokenRepository();
}
}
Since we are importing security lib and it has production blacklisted jwt token repository implementation, we overwrite it with a fake one by using @Primary annotation (another possibility would be to exclude the production class from component scan). We don’t need to do the same with blog repos since we are importing only specific classes as beans with @Import annotation.
The whole code is available on commit: https://github.com/TheProgrammersDiary/Blog/commit/e89f871688299046d55a74d3c673297b23b16a1a.
I have spent lots of time doing this tests. All because I was lazy to write them earlier, wanting to ship my code quicker. In book Pragmatic programmer, it is said (not exact quote): if you postpone testing later you will never test the code. The rephrased line lives inside my code:
createsPost=[
{
"author": "Human",
"content": "You either test first, test along coding, or don't test at all.",
"id": "#hidden#",
"title": "Testing matters"
}
]
And I understand why: testing after code is written sucks: it’s boring and hard. It’s hard to write good tests after you have written the code. That’s why disciplines like TDD were born: writing tests first allows us to decouple from implementation detail and write good code later.
Yet better to pay the debt by testing after than regret not testing at all later. To not repeat the mistake of testing the code after, jacoco code coverage rules will come in handy - I will have to test extensively before the code is written or packaged.
—————
Thanks for reading.
The project logged in this diary is open source, so if you would like to code or suggest changes, please visit https://github.com/TheProgrammersDiary.
Next part: https://hashnode.programmersdiary.com/implement-https-in-microservices-and-fe.
Subscribe to my newsletter
Read articles from Evaldas Visockas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
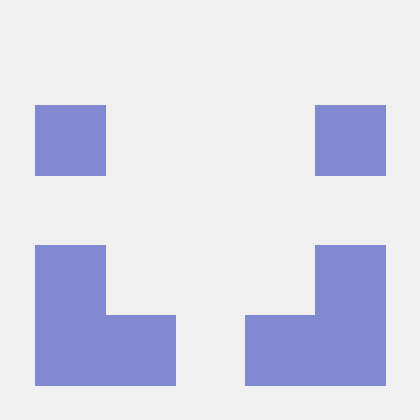