[Python] How to Create Immutable Dictionaries & Sets in Python?
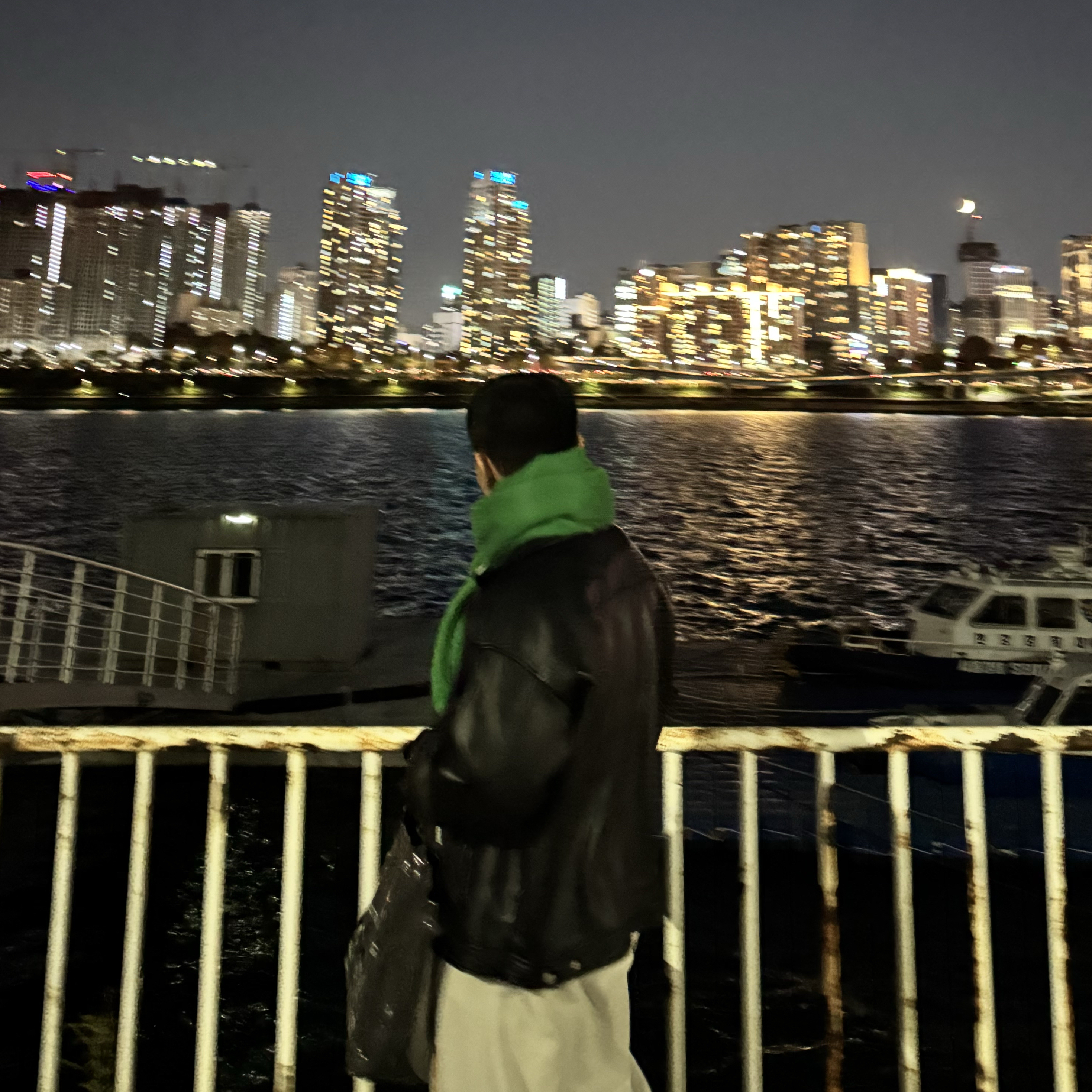

What are Immutable Dictionaries & Sets?
Before reading this article, you should have an understanding of mutable and immutable types in python. You can look at my article that explains them pretty easy: [CS fundamentals] Mutable vs. Immutable.
Dictotionaries and sets are mutable as we can change values. For example:
d = {'key1': 'value1'}
print(d) # {'key1': 'value1'}
d['key2'] = 'value2'
d.setdefault('key3', 'value3')
print(d) # {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
s = {'Apple', 'Orange', "Apple", "Orange", 'Kiwi'}
print(s) # {'Kiwi', 'Orange', 'Apple'}
s.add('Melon')
print(s) # {'Melon', 'Kiwi', 'Orange', 'Apple'}
As you may see, the values of d
and s
can be changed, which means they are mutable.
Immutable Dictionaries and Sets
Creating Immutable Dictionary:
from types import MappingProxyType
d = {'key1': 'value1'}
# Read-only
d_frozen = MappingProxyType(d)
print(d_frozen) # {'key1': 'value1'}
d_frozen['key2'] = 'value2' # error
You can make your dictionary as immutable by using
MappingProxyType
function in types module.
You are making your dictionary as read-only, so that your co-workers cannot edit the sensitive data stored in your immutable dictionary.
If you try to assign a new key-value pair, it will print an error.
Traceback (most recent call last):
File "your_file", line 14, in <module>
d_frozen['key2'] = 'value2'
TypeError: 'mappingproxy' object does not support item assignment
Creating Immutable Set:
s = frozenset(['Apple', 'Orange', "Apple", "Orange", 'Kiwi'])
s.add('Melon') # error
It’s very simple. You can use frozenset()
to make it immutable. Just like a dictionary, immutable set is also read-only, so that the data are not changed by someone.
If you try to modify it, it will print an error:
Traceback (most recent call last):
File "your_file", line 29, in <module>
s6.add('Melon')
AttributeError: 'frozenset' object has no attribute 'add'
Subscribe to my newsletter
Read articles from Lim Woojae directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
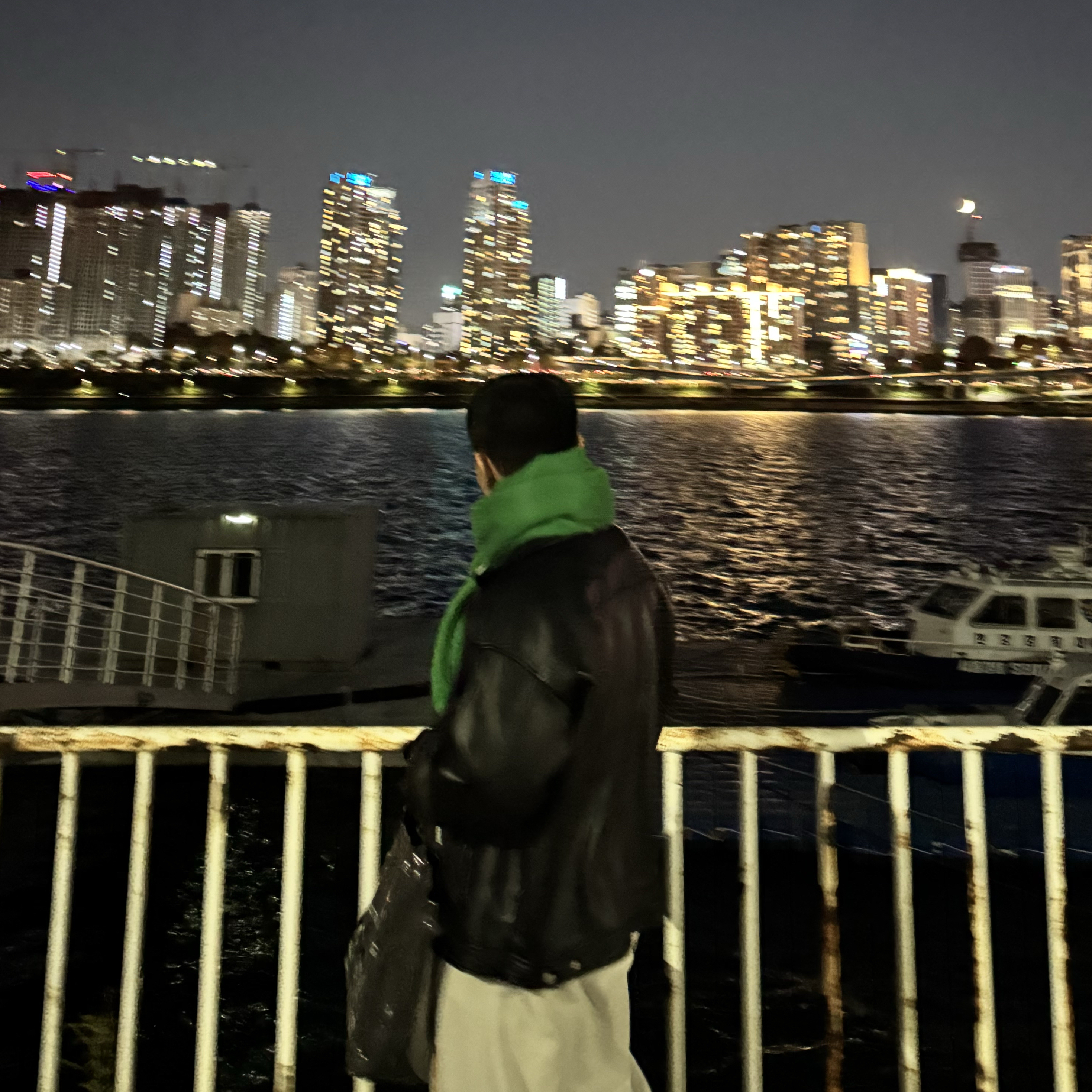
Lim Woojae
Lim Woojae
Computer Science Enthusiast with a Drive for Excellence | Data Science | Web Development | Passionate About Tech & Innovation