How to Build a Windows Application with React Native
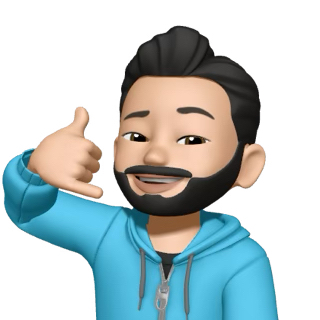
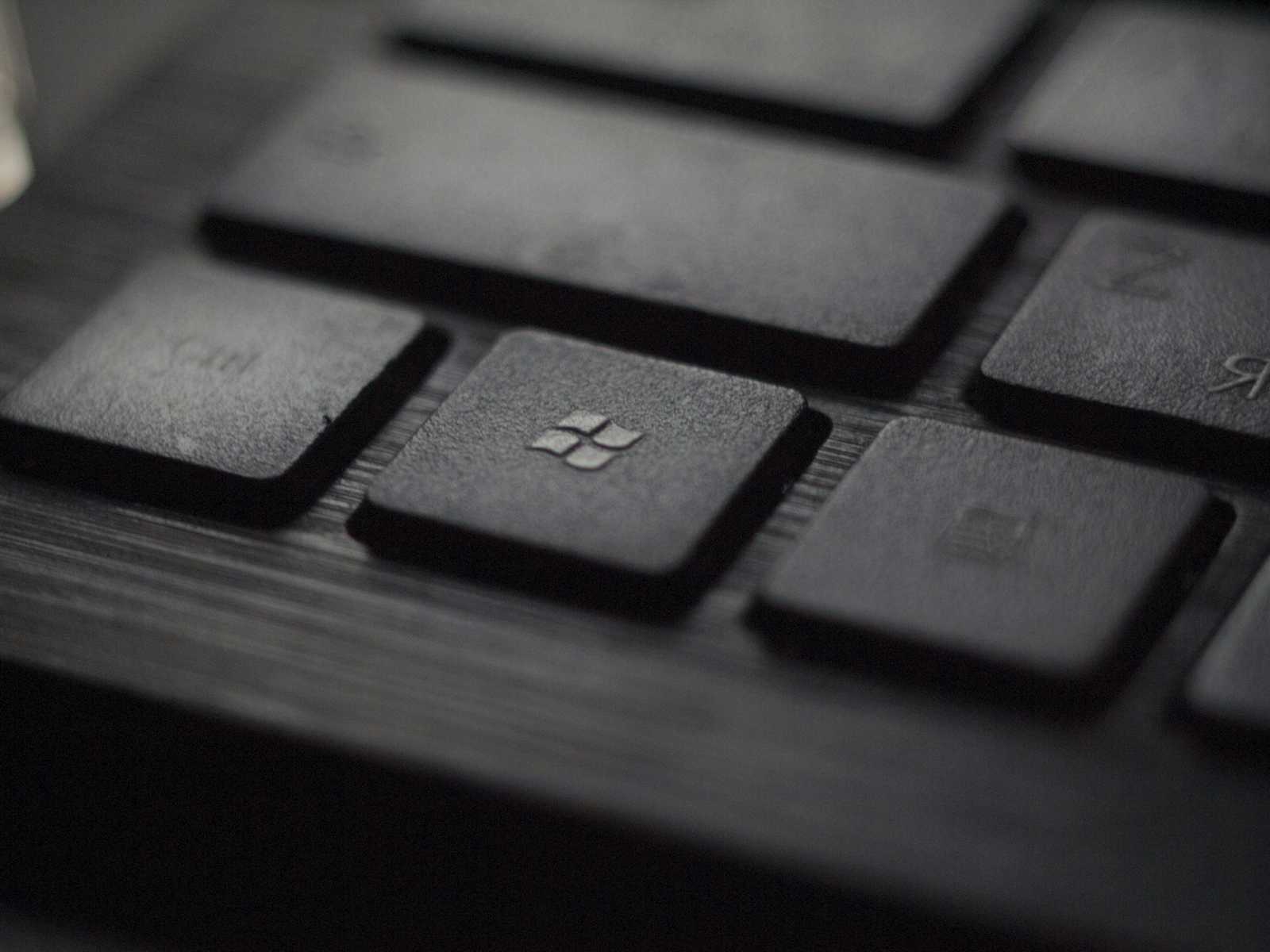
React Native is known for its ability to build mobile applications, but with the React Native Windows extension, you can also create desktop applications for Windows. In this guide, we'll walk you through the steps to set up, build, and run a React Native app for Windows.
Why React Native for Windows?
React Native for Windows extends React Native to support Windows 10 and 11. You can leverage your existing React Native knowledge to build high-performance native desktop apps. Key benefits include:
- Shared codebase across platforms.
- Access to native Windows APIs.
- Integration with Windows features like touch, pen input, and Fluent UI.
Prerequisites
Before we begin, make sure you have the following:
- Node.js (LTS version recommended)
- React Native CLI installed globally:
npm install -g react-native-cli
- Visual Studio 2022 with the following workloads:
- Universal Windows Platform development.
- Desktop development with C++.
- Visual Studio 2022 with the following workloads:
- Windows 10/11 Development Environment enabled:
- Enable Developer Mode in Windows Settings.
Step 1: Create a React Native Project
Run the following command to create a new React Native project:
npx react-native init MyWindowsApp
Navigate to your project directory:
cd MyWindowsApp
Step 2: Add Windows Support
Add React Native Windows to your project:
npx react-native-windows-init --overwrite
This will configure your project for Windows development and add a new windows
folder.
Step 3: Build the Windows Application
- Open the
windows/MyWindowsApp.sln
file in Visual Studio. - Set the solution configuration to
Debug
and platform tox64
. - Press F5 or click Start to build and run the application.
Step 4: Write Your React Native Code
You can now start building your application by editing the App.js
file. For example:
import React from 'react';
import { Text, View, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, Windows with React Native!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f0f0f0',
},
text: {
fontSize: 24,
color: '#333',
},
});
export default App;
Step 5: Customize for Windows
You can add platform-specific code for Windows using the Platform
module:
import { Platform } from 'react-native';
if (Platform.OS === 'windows') {
console.log('This is running on Windows!');
}
Step 6: Debugging and Testing
React Native for Windows supports hot reloading, so changes you make to your code will reflect immediately in the running app. Use the React Native Debugger to inspect and debug your application.
Conclusion
With React Native for Windows, you can create versatile desktop applications with a shared codebase for web, mobile, and desktop. Whether you're building productivity tools, entertainment apps, or enterprise software, React Native for Windows provides a robust platform for development.
Resources
Happy coding!
Subscribe to my newsletter
Read articles from Valliappan Periannan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
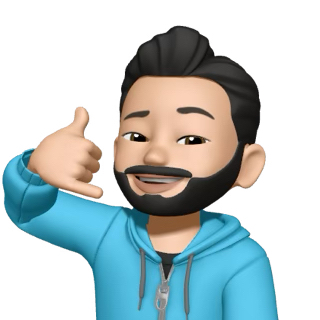
Valliappan Periannan
Valliappan Periannan
Started my career as a mobile app intern in 2017 at a dynamic startup. Specialized in Android Kotlin app development, later expanded skills to Swift and React Native. ๐ฑ๐ป Passionate about creating seamless user experiences and staying at the forefront of mobile technology trends. I thrive on challenges and love turning innovative ideas into reality through code. Experienced in collaborating with cross-functional teams and translating complex requirements into elegant solutions. Excited about the potential of technology to make a positive impact on people's lives. Always up for a coding challenge or exploring the next big thing in tech. When I'm not coding, you can find me immersed in a good book, capturing moments through my lens, or conquering new trails. Let's embark on this coding journey together! โจ๐