Kubernetes Service and Types of Services
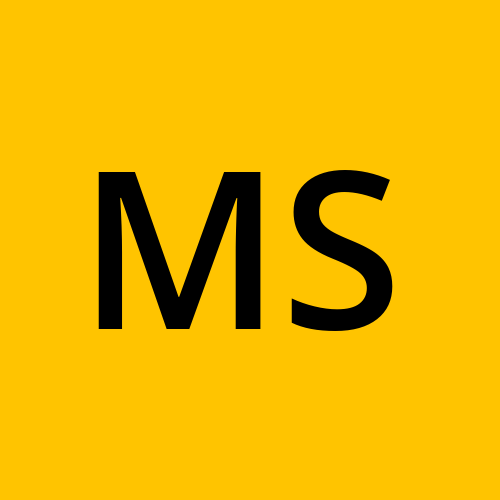
Table of contents

👉YouTube Link: https://youtu.be/S_IXfq6HHgA?si=Ck_AXS8AdzFHJjuG
Kubernetes Services: A Complete Guide
A Kubernetes Service provides a stable, reliable way to connect and communicate with Pods, abstracting away their dynamic nature.
Why Do We Need Kubernetes Services?
Kubernetes, at its core, is all about managing containerized applications. While Pods serve as the execution units for these applications, they’re ephemeral, meaning they can be replaced or moved by Kubernetes at any time. This is because Pods are designed to be lightweight and short-lived, often tied to a specific node or container instance. If a node fails or an application is updated, Kubernetes ensures high availability by rescheduling Pods on healthy nodes, making their IP addresses and locations dynamic. This dynamic behavior, central to Kubernetes' design principles, makes direct communication with Pods challenging. That’s where Services come in.
Imagine you have an application running across multiple Pods. Each Pod has its own IP address, but these addresses change whenever a Pod is recreated. If another application or user wants to interact with these Pods, it becomes chaotic to keep track of constantly changing IPs. Services solve this problem by acting as a single stable access point for a group of Pods.
Core Concepts in Services
Stable Access: Services provide a consistent IP address and DNS name to access Pods, regardless of their lifecycle changes. The DNS name acts as a human-readable alias for the Service, simplifying communication by eliminating the need to track dynamic IP addresses. This abstraction ensures that applications can reliably connect to Pods without worrying about their underlying infrastructure changes. Here, the targetPort concept also comes into play. While port defines the Service's exposed port for communication, targetPort is the fixed port on the Pod where the Service redirects traffic. This decouples the internal Pod configuration from the Service interface, simplifying platform management. For example, a Service may expose port 80, while the targetPod uses port 8080 internally, enabling flexibility.
Discovery and Load Balancing: Services distribute incoming traffic evenly across multiple Pods, ensuring no single Pod is overwhelmed.
Selector Labels: Services use label selectors to identify which Pods they manage, ensuring communication with the correct application group. For example, a Service with the label selector app: backend will route traffic only to Pods with the label app: backend. This ensures that the Service targets the correct set of Pods without ambiguity.
Types of Kubernetes Services
Kubernetes supports four primary types of Services, each tailored for different use cases. Let’s dive into them.
1. ClusterIP
What: The default Service type, accessible only within the cluster.
Why: Ideal for internal communication between Pods or applications within the same Kubernetes cluster.
Example: A backend service communicating with a database service.
How It Works:
Assigns an internal cluster-wide IP.
Pods in the cluster use this IP to communicate without needing external exposure.
Code Example:
apiVersion: v1 kind: Service metadata: name: backend-service spec: type: ClusterIP selector: app: backend ports: - port: 80 targetPort: 8080
Port and TargetPort:
port
: The Service port accessible to other Pods.targetPort
: The port on the Pod the Service forwards traffic to.
2. NodePort
What: Exposes the Service on a port of each cluster node.
Why: Useful for accessing the application externally without needing a LoadBalancer.
How It Works:
Opens a static port (default range: 30000–32767) on each node.
Requests to the node's IP at the NodePort are routed to the Service, then to the Pods.
Key Considerations:
NodePorts are limited to a specific range (30000–32767) to ensure consistency and avoid conflicts with well-known ports used by other applications. This range helps Kubernetes reserve a dedicated space for external access, but it can pose limitations in scenarios where many NodePorts are required, as the range is relatively small. For large-scale production environments, LoadBalancer or Ingress is often preferred to overcome this constraint.
Additionally, NodePorts expose ports directly to external traffic, creating security risks. Unauthorized access or attacks can occur as NodePorts make all cluster nodes accessible through the exposed port. For production environments, LoadBalancer or Ingress is preferred to ensure better security and traffic management.
Code Example:
apiVersion: v1 kind: Service metadata: name: nodeport-service spec: type: NodePort selector: app: my-app ports: - port: 80 targetPort: 8080 nodePort: 30007
- NodePort Specifics: The node gets a port (e.g.,
30007
), which users or applications use to access the Service.
- NodePort Specifics: The node gets a port (e.g.,
3. LoadBalancer
What: Extends NodePort by provisioning a cloud provider's load balancer to expose the Service externally.
Why: Automatically balances traffic across nodes and routes traffic to healthy Pods.
How It Works:
Integrates with cloud providers like AWS or GCP to set up a load balancer.
Useful for high-traffic applications requiring robust fault tolerance.
Code Example:
apiVersion: v1 kind: Service metadata: name: loadbalancer-service spec: type: LoadBalancer selector: app: frontend ports: - port: 80 targetPort: 8080
- The cloud provider assigns an external IP or DNS name for the LoadBalancer.
4. ExternalName
What: Maps a Service to an external DNS name.
Why: Useful for integrating external services like third-party APIs.
How It Works:
No Pods or selectors are required.
The Service acts as an alias for the external resource.
Code Example:
apiVersion: v1 kind: Service metadata: name: external-service spec: type: ExternalName externalName: example.com
The Role of kube-proxy
kube-proxy is the traffic manager for Kubernetes Services. Here’s how it integrates:
What It Does: Monitors the Kubernetes API for Service and Endpoint changes and updates routing rules.
How It Works:
Manages network rules using
iptables
orIPVS
.Ensures traffic reaches the right Pods based on Service configuration.
Example: When a request is made to a Service IP, kube-proxy forwards it to one of the Pods behind the Service.
Key Differences Between Service Types
Feature | ClusterIP | NodePort | LoadBalancer | ExternalName |
Access Scope | Internal (cluster-wide) | External via node IP | External via load balancer | External DNS name |
Use Case | Pod-to-Pod communication | User-facing or debugging | Production-level traffic | External services/APIs |
IP Stability | Stable | Node-specific | Cloud-provided | External |
Example Use Case | Backend DB communication | Debugging an app locally | Public-facing applications | Connecting to external APIs |
Why Ports Matter in Services
port: Exposed by the Service and used by clients to communicate with it.
targetPort: The port on the Pod the traffic is directed to. It must match the container's port unless explicitly defined differently.
nodePort (for NodePort and LoadBalancer): The port assigned on each node for external access.
Common Challenges and Best Practices
Challenges with NodePort:
Limited port range and potential security vulnerabilities.
Not suitable for high-traffic production environments.
Best Practices:
Use
ClusterIP
for internal communication.Prefer
LoadBalancer
orIngress
for external traffic in production.Define labels and selectors clearly to avoid misrouting.
Real-World Use Cases for Kubernetes Services:
ClusterIP:
- Example Use Case: Using
ClusterIP
for internal microservice communication.
Scenario: A backend API service communicates with a Redis cache to manage session data within the same Kubernetes cluster.
- Example Use Case: Using
NodePort:
- Example Use Case: Using
NodePort
for exposing applications during development or testing.
Scenario: A QA team tests a new feature by exposing a Flask web application on a specific NodePort for external access.
- Example Use Case: Using
LoadBalancer:
- Example Use Case: Using
LoadBalancer
for a public-facing e-commerce website.
Scenario: A company hosts its online store frontend on Kubernetes and exposes it to customers globally with automatic load balancing across multiple replicas.
- Example Use Case: Using
ExternalName:
- Example Use Case: Using
ExternalName
to integrate with a third-party payment gateway.
Scenario: A SaaS application connects to PayPal's API by mapping the Kubernetes Service to the external DNS name of the PayPal API server.
- Example Use Case: Using
Summary
Kubernetes Services act as the backbone for managing communication between applications in a cluster. Each Service type has its unique role:
ClusterIP: For internal communication.
NodePort: For exposing Services externally without cloud integration.
LoadBalancer: For robust, scalable, and fault-tolerant external exposure.
ExternalName: For integrating external resources.
Understanding these concepts, alongside kube-proxy's role, ensures seamless application deployment and traffic management in Kubernetes clusters.
Amazing Resources:
Follow me on linkedin: Md. Musfikur Rahman Sifar | LinkedIn
YouTube: Md Musfikur Rahman Sifar - YouTube
Subscribe to my newsletter
Read articles from Md. Musfikur Rahman Sifar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
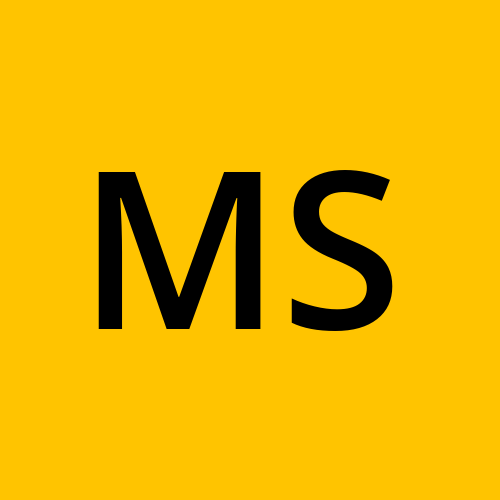