Mastering C# Part 5.3 - Tuples
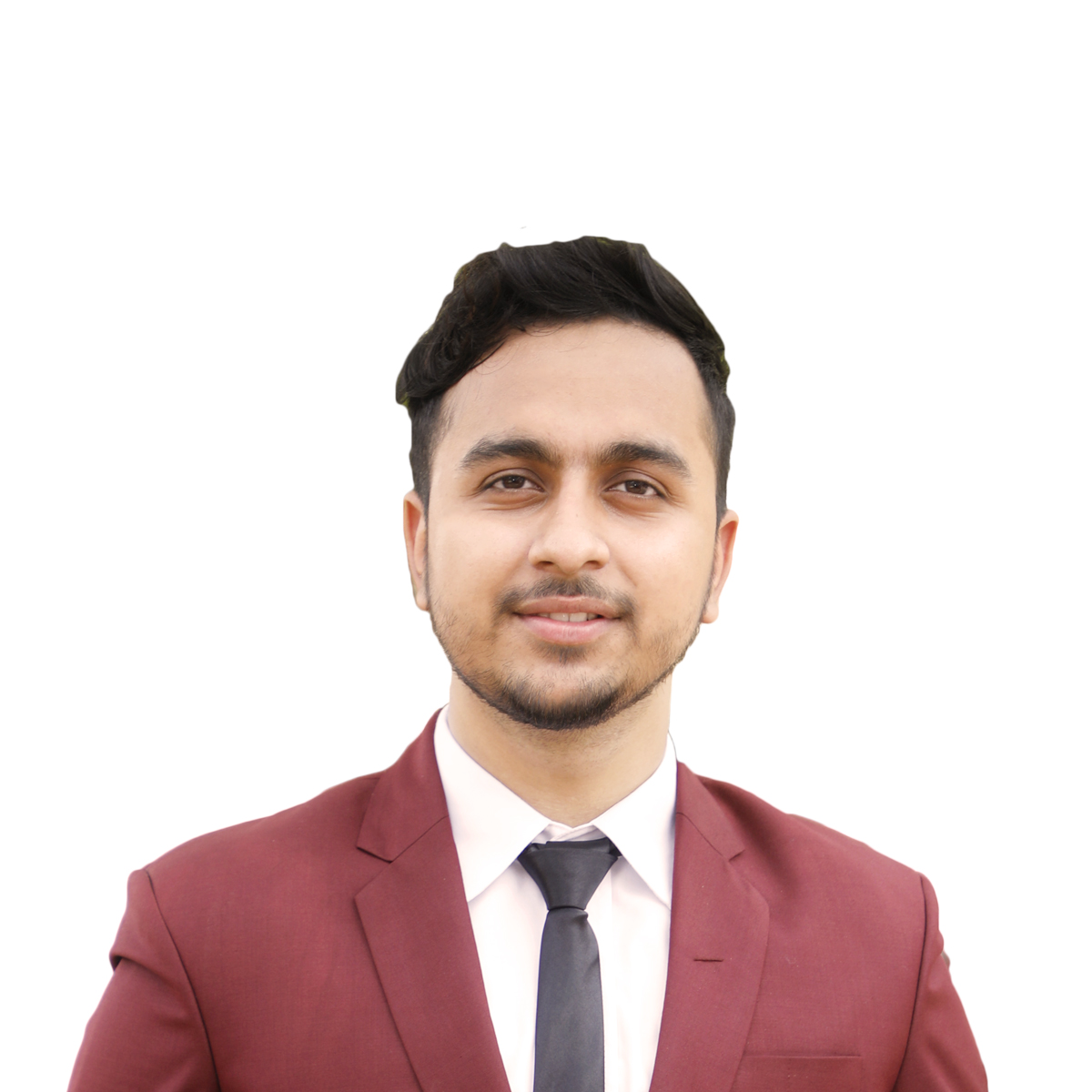
Table of contents
- Tuples in C#
- Key Concepts of Tuples
- Creating Tuples
- 1. Using Tuple<T1, T2, ..., T8> (Old-style Tuple)
- 2. Using ValueTuple (C# 7.0 and later)
- Creating ValueTuple
- Tuple with Multiple Elements
- Accessing Tuple Elements
- 1. Using Indexers (Position-based)
- 2. Using Named Properties
- Tuple Deconstruction
- Returning Tuples from Methods
- Tuple Equality and Comparisons
- Advantages of Tuples
- Limitations of Tuples
- Conclusion
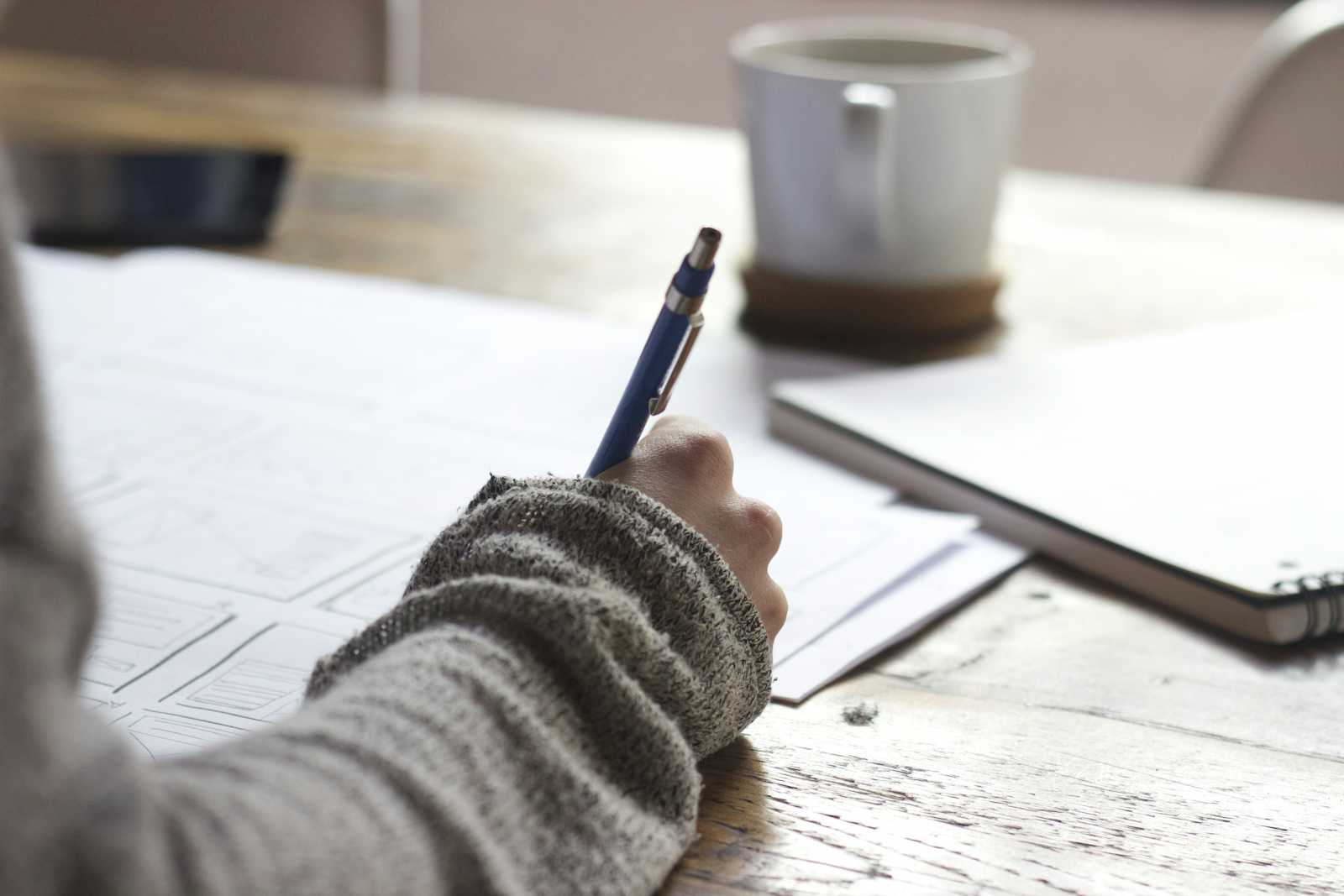
Tuples in C#
A tuple is a data structure that can hold a fixed number of elements, where each element can have a different type. In C#, tuples are typically used to return multiple values from a method without needing to create a custom class or struct. They can also be used when you want to group related values together temporarily.
C# 7.0 and later introduced ValueTuple, which provides a more lightweight, flexible way of using tuples as compared to the older Tuple
class (introduced in .NET Framework 4.0).
Key Concepts of Tuples
Fixed Size: A tuple has a fixed number of elements, and its size cannot change after creation.
Heterogeneous Types: Each element in the tuple can have a different type. For example, a tuple can store an integer, a string, and a boolean value.
Indexed Access: Elements of a tuple can be accessed using indices (starting from 0) or using named properties (for
ValueTuple
).
Creating Tuples
1. Using Tuple<T1, T2, ..., T8>
(Old-style Tuple)
In C#, tuples were originally provided by the System.Tuple
class. This class allows you to create tuples with up to 8 elements.
// Using Tuple<T1, T2> class
Tuple<int, string> tuple = new Tuple<int, string>(1, "Hello");
Console.WriteLine($"Item1: {tuple.Item1}, Item2: {tuple.Item2}");
Here, Item1
and Item2
are the default names of the tuple items.
2. Using ValueTuple
(C# 7.0 and later)
ValueTuple
is the recommended way of using tuples in C#. It is a more lightweight version compared to Tuple<T>
and offers the ability to name the tuple elements.
Creating ValueTuple
// Using ValueTuple
var person = (Id: 1, Name: "Alice");
Console.WriteLine($"Id: {person.Id}, Name: {person.Name}");
In this example, the tuple contains two elements: an int
(Id
) and a string
(Name
). The elements are named for better readability.
Tuple with Multiple Elements
Tuples can hold more than two elements. In fact, you can create tuples with up to 8 elements using ValueTuple
.
// Tuple with multiple elements
var product = (Id: 101, Name: "Laptop", Price: 999.99, InStock: true);
Console.WriteLine($"Id: {product.Id}, Name: {product.Name}, Price: {product.Price}, InStock: {product.InStock}");
In this case, the tuple holds an int
, a string
, a double
, and a bool
. You can add more fields by extending the tuple.
Accessing Tuple Elements
1. Using Indexers (Position-based)
You can access the elements of a tuple using index-based access, where the index starts from 0.
var point = (x: 10, y: 20);
// Accessing by position (Index starts at 0)
Console.WriteLine($"x: {point.Item1}, y: {point.Item2}");
2. Using Named Properties
For better readability, it’s often recommended to use named properties when dealing with ValueTuple
.
var person = (Id: 1, Name: "John");
// Accessing by name
Console.WriteLine($"Id: {person.Id}, Name: {person.Name}");
Tuple Deconstruction
You can deconstruct a tuple into individual variables, making it easier to work with tuple elements.
var person = (Id: 1, Name: "Alice");
// Deconstructing the tuple
var (id, name) = person;
Console.WriteLine($"Id: {id}, Name: {name}");
This is particularly useful when you want to work with the tuple's values directly in variables.
Returning Tuples from Methods
Tuples are often used in C# methods to return multiple values in a single return statement.
// Method returning a tuple
public static (int, string) GetPersonDetails()
{
return (1, "Alice");
}
var result = GetPersonDetails();
Console.WriteLine($"Id: {result.Item1}, Name: {result.Item2}");
Alternatively, you can use named items to make the method more readable.
public static (int Id, string Name) GetPersonDetails()
{
return (1, "Bob");
}
var result = GetPersonDetails();
Console.WriteLine($"Id: {result.Id}, Name: {result.Name}");
Tuple Equality and Comparisons
ValueTuple
provides built-in support for comparisons. You can compare two tuples directly:
var tuple1 = (1, "John");
var tuple2 = (1, "John");
var tuple3 = (2, "Alice");
Console.WriteLine(tuple1 == tuple2); // True
Console.WriteLine(tuple1 == tuple3); // False
Tuples of the same type and structure can be compared for equality, and the comparison is based on the values.
Advantages of Tuples
Lightweight:
ValueTuple
is a lightweight structure and does not require the overhead of creating a class or struct.Multiple Returns: Allows you to return multiple values from a function without creating complex return types.
Named Values: In
ValueTuple
, you can give names to each element, making the code more readable.Improved Performance:
ValueTuple
is more memory efficient compared to theTuple
class.
Limitations of Tuples
Fixed Size: Tuples have a fixed size, so if you need a variable-sized collection, you should use a list or array.
Limited to 8 Elements:
ValueTuple
can only store up to 8 elements. For more than 8 elements, you would need to use a custom class or struct.Not Ideal for Complex Data Models: For complex models, using a class or struct is more appropriate than tuples because they provide better readability and maintainability.
Conclusion
Tuples in C# offer a simple and efficient way to group data of different types. ValueTuple
is the preferred approach due to its performance advantages and support for named fields. It's especially useful when you need to return multiple values from a method without creating custom classes or structs. However, for more complex scenarios, custom data structures may be more appropriate.
Subscribe to my newsletter
Read articles from Nishant Banjade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
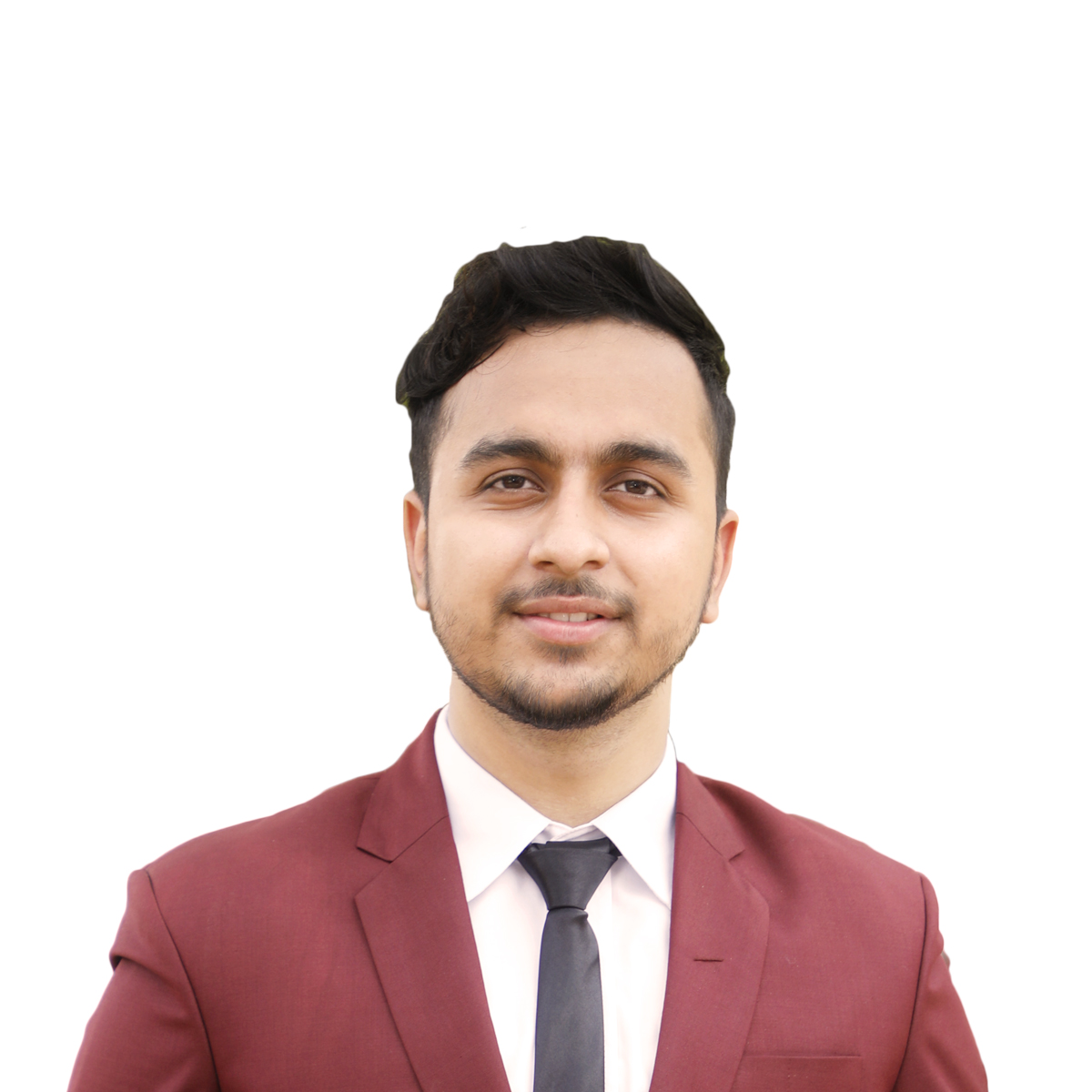
Nishant Banjade
Nishant Banjade
Hi, I'm Nishant Banjade, a Software Engineer at Ellucian, committed to transforming education through technology. With expertise in developing CRM systems, plugins, workflows, APIs, and customized solutions for Higher Education, I bring a strong focus on innovation and efficiency. Currently, I'm honing my skills in full-stack software development, system design, data structures, algorithms, and Microsoft Dynamics 365. I am a skilled software developer with expertise in C#/.NET, D365, JavaScript, TypeScript, ReactJS, SQL, and cloud technologies like AWS, along with experience in RabbitMQ, Docker, MUI, Tailwind, JIRA, and Git.